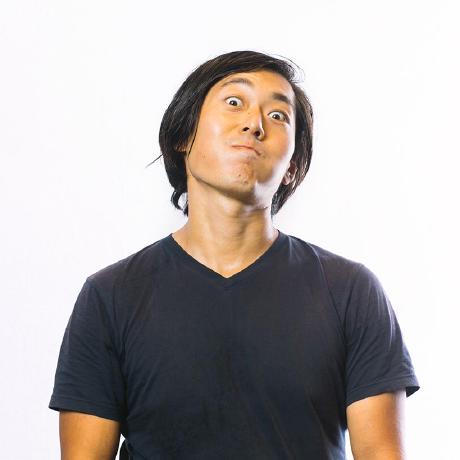
Press to talk, and get a translation!
The app is set up so you can easily have a conversation between two people. The app will translate between the two selected languages, in each voice, as the speakers talk.
Add your OpenAI API Key, and make sure to open in a separate window for Mic to work.
An http and class wrapper for Vercel's AI SDK
Usage:
- Groq:
https://yawnxyz-ai.web.val.run/generate?prompt="tell me a beer joke"&provider=groq&model=llama3-8b-8192
- Perplexity:
https://yawnxyz-ai.web.val.run/generate?prompt="what's the latest phage directory capsid & tail article about?"&provider=perplexity
Example of using Hono to stream OpenAI's streamed chat responses
Unfortunately this doesn't work on val.town — use Deno Deploy instead
Val.town somehow doesn't run multi-line code. Does work with "3+3" but not more complex stuff.
Basic demo of getting reactive Alpine.js working on a hono/jsx "backend"
- For server <> frontend interaction, do form POST submissions (or potentially POST json to the public val address?)
Doesn't seem to work on val.town, should work in principle
markdown.download
Handy microservice/library to convert various data sources into markdown. Intended to make it easier to consume the web in ereaders
https://jsr.io/@tarasglek/markdown-download
Features
- Apply readability
- Further convert article into markdown to simplify it
- Allow webpages to be viewable as markdown via curl
- Serve markdown converted to html to browsers
- Extract youtube subtitles
Source
https://github.com/tarasglek/markdown-download
https://www.val.town/v/taras/markdown_download
License: MIT
Usage: https://markdown.download/ + URL
Dev: https://val.markdown.download/ + URL