Search
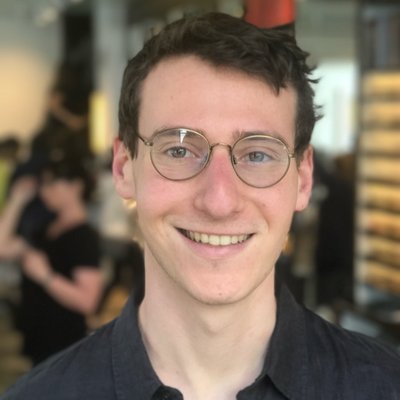
todo_list
@stevekrouse
Example Full-stack Todo List App with React SSR + Client-side hydration & sqlite Requires you to put the React component in another val, in this case: https://www.val.town/v/stevekrouse/TodoApp
HTTP
# Example Full-stack Todo List App with React SSR + Client-side hydration & sqlite
Requires you to put the React component in another val, in this case: https://www.val.town/v/stevekrouse/TodoApp
import ssr_hydrate from "https://esm.town/v/stevekrouse/ssr_hydrate_react";
export default ssr_hydrate("stevekrouse", "TodoApp");
efficientAmberUnicorn
@CoachCompanion
@jsxImportSource https://esm.sh/react@18.2.0
HTTP
/** @jsxImportSource https://esm.sh/react@18.2.0 */
import React, { useState, useEffect, useRef } from "https://esm.sh/react@18.2.0";
import { createRoot } from "https://esm.sh/react-dom@18.2.0/client";
import { marked } from "https://esm.sh/marked@4.3.0";
score += Math.min(tagScore, 0.3); // Cap tag score at 0.3
// Consider channel relevance
if (videoData.channelTitle.toLowerCase().includes(sport.toLowerCase())) {
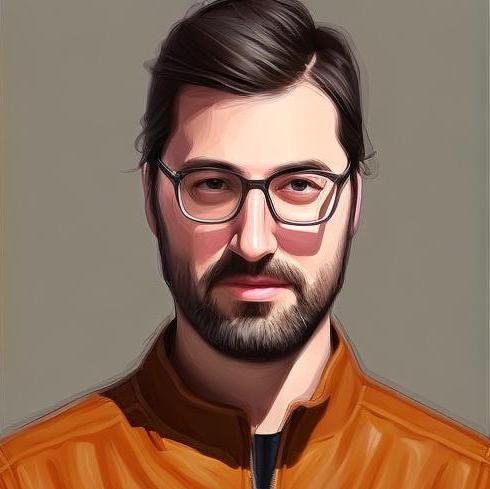
bingo
@nn
@jsxImportSource https://esm.sh/react
HTTP
/** @jsxImportSource https://esm.sh/react */
import confetti from "https://esm.sh/canvas-confetti";
import React, { useEffect, useState } from "https://esm.sh/react";
import { createRoot } from "https://esm.sh/react-dom/client";
function slugify(text: string): string {
const termCount = terms.split("\n").filter(t => t.trim()).length;
const handleSubmit = async (e: React.FormEvent) => {
e.preventDefault();
origin: { y: 0.6 },
// Fire again from the sides after a small delay
setTimeout(() => {
localStorage.setItem(`bingo-${gameId}-${playerId}`, JSON.stringify(newChecked));
const handleNameChange = (e: React.ChangeEvent<HTMLInputElement>) => {
const newName = e.target.value;
</div>
function client() {
const root = document.getElementById("root");
<BingoCard gameId={gameId} playerId={playerId} name={name} terms={terms} />,
if (typeof document !== "undefined") { client(); }
export default async function server(req: Request): Promise<Response> {
passwordGen
@all
@jsxImportSource https://esm.sh/react
HTTP
/** @jsxImportSource https://esm.sh/react */
import confetti from "https://esm.sh/canvas-confetti@1.6.0";
import React, { useEffect, useState } from "https://esm.sh/react";
import { createRoot } from "https://esm.sh/react-dom/client";
function AlbertiCipherDisk({ outerRotation, innerRotation, onRotate }) {
<h2 className="text-3xl font-bold mb-6">Advanced Password Generation System: Usage Instructions</h2>
<ol className="list-decimal list-inside space-y-4 mb-6 text-lg">
<li>
<li>Utilize the Alberti Cipher Disk to introduce an additional layer of cryptographic complexity:</li>
<ul className="list-disc list-inside ml-6 space-y-2">
<li>
</div>
function client() {
createRoot(document.getElementById("root")).render(<App />);
if (typeof document !== "undefined") {
client();
async function server(request: Request): Promise<Response> {
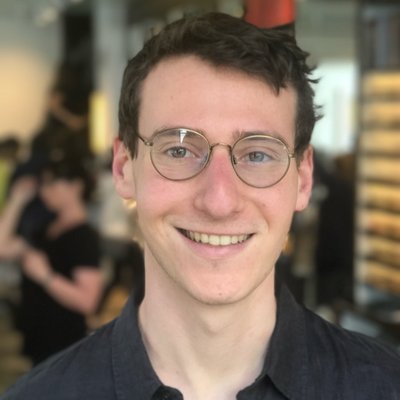
lastlogin
@stevekrouse
LastLogin Auth LastLogin Auth is the simplest way to add user authentication to your HTTP val. LastLogin is a decentralized hosted auth provider
that enables login to any app through email, Google, Github, etc without API keys. This val exports middleware that can be added to any HTTP val. Login with Google While LastLogin supports many login providers, it's often
simplest to have a single "Login with Google" button. Live Demo How to setup These instructions were written to be easily copy-and-pasteable
into LLMs like Townie. import { lastlogin } from "https://esm.town/v/stevekrouse/lastlogin_safe" Wrap & export the main HTTP handler: export default lastlogin(handler) . In the HTTP handler, retrieve the user's email: const email = request.headers.get("X-LastLogin-Email") If email, show that user's content, and a logout link to "/auth/logout"; if no email, show login options. import { LoginWithGoogleButton } from "https://esm.town/v/stevekrouse/LoginWithGoogleButton" Use the React Component, optionally supplying the text attribute Add "via LastLogin" underneat LoginWithGoogleButton, centered, secondary text, linking to https://lastlogin.io/ Pass the email from the server to the client-side code if using React hydration or similar techniques. How it works Your users click on the React Component in your app The opens a new window to /auth/login?provider=google in your app This middleware directs them to log into Google via LastLogin They log in to Google Google redirects to LastLogin, who redirects back to /auth/callback in your app This middleware "logs them in" to your app by giving them a session cookie. In your app, you can read the X-LastLogin-Email header to see which (if any) user is logged in Login with LastLogin The classic LastLogin authentication flow is to
redirect your user to lastlogin.io/login ,
where they can pick which way to login: email, Google, Github, etc. Live Demo How to set up These instructions were written to be easily copy-and-pasteable
into LLMs like Townie. import { lastlogin } from "https://esm.town/v/stevekrouse/lastlogin_safe" Wrap & export the main HTTP handler: export default lastlogin(handler) . In the HTTP handler, retrieve the user's email: const email = request.headers.get("X-LastLogin-Email") If email, show that user's content, and a logout link to "/auth/logout"; If no email, add a link to "Login or Sign Up" to "/auth/login" Pass the email from the server to the client-side code if using React hydration or similar techniques. How it works Your users click on a link to /auth/login in your app This middleware directs them to login via LastLogin They authenticate to LastLogin LastLogin redirects them back to your app This middleware "logs them in" to your app by giving
them a session cookie. In your app, you can read the X-LastLogin-Email header
to see which (if any) user is logged in Notes If you want username & password auth: @stevekrouse/lucia_middleware This middleware stores sessions in the lastlogin_session
table in your Val Town SQLite This val has NOT been properly audited for security.
I am not a security expert. I would suggest only using it
for demos, prototypes, or apps where security is not paramount.
If you are a security expert, I would appreciate your help
auditing this! Todos [ ] Let the user customize the name of the SQLite table [ ] Get a proper security audit for this
Script
* `import { LoginWithGoogleButton } from "https://esm.town/v/stevekrouse/LoginWithGoogleButton"`
* Use the <LoginWithGoogleButton /> React Component, optionally supplying the `text` attribute
* Add "via LastLogin" underneat LoginWithGoogleButton, centered, secondary text, linking to https://lastlogin.io/
* Pass the email from the server to the client-side code if using React hydration or similar techniques.
### How it works
1. Your users click on the <LoginWithGoogleButton /> React Component in your app
2. The <LoginWithGoogleButton /> opens a new window to /auth/login?provider=google in your app
* If no email, add a link to "Login or Sign Up" to "/auth/login"
* Pass the email from the server to the client-side code if using React hydration or similar techniques.
### How it works
const url = new URL(req.url);
const clientID = `${url.protocol}//${url.host}/`;
const redirectUri = `${url.protocol}//${url.host}/auth/callback`;
const tokenUrl = new URL("https://lastlogin.net/token");
tokenUrl.searchParams.set("client_id", clientID);
tokenUrl.searchParams.set("code", code);
const provider = url.searchParams.get("provider");
authUrl.searchParams.set("client_id", clientID);
authUrl.searchParams.set("redirect_uri", redirectUri);
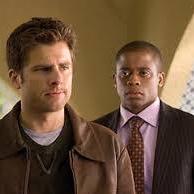
TaylorSwiftMoments
@vawogbemi
@jsxImportSource https://esm.sh/react
HTTP
/** @jsxImportSource https://esm.sh/react */
import { createFalClient } from "https://esm.sh/@fal-ai/client";
import React, { useEffect, useState } from "https://esm.sh/react";
import { createRoot } from "https://esm.sh/react-dom/client";
const swiftEras = [
console.error("Error fetching images:", error);
const generateImage = async (e?: React.FormEvent) => {
e?.preventDefault();
try {
// Enhance the prompt using the server-side endpoint
const enhancedPromptResponse = await fetch("/api/enhance-prompt", {
console.log("Enhanced Prompt:", enhancedPrompt);
const fal = createFalClient({
proxyUrl: "/api/fal/proxy",
</div>
function client() {
createRoot(document.getElementById("root")).render(<App />);
if (typeof document !== "undefined") { client(); }
export default async function server(req: Request): Promise<Response> {
Calculator
@NZHEATPUMPS
@jsxImportSource https://esm.sh/react@18.2.0
HTTP
/** @jsxImportSource https://esm.sh/react@18.2.0 */
import { createRoot } from "https://esm.sh/react-dom@18.2.0/client";
import React, { useState } from "https://esm.sh/react@18.2.0";
function ACCalculator() {
baseBTU += ((height - 2.4) * 3.28084) * length * width * 2.5;
// Regional and efficiency considerations
const adjustedBTU = baseBTU * regionFactor.heating;
</div>
function client() {
createRoot(document.getElementById("root")).render(<ACCalculator />);
if (typeof document !== "undefined") { client(); }
export default async function server(request: Request): Promise<Response> {
lazyCook
@karkowg
@jsxImportSource https://esm.sh/react
HTTP
/** @jsxImportSource https://esm.sh/react */
import React, { useState } from "https://esm.sh/react";
import { createRoot } from "https://esm.sh/react-dom/client";
function App() {
</div>
function client() {
createRoot(document.getElementById("root")).render(<App />);
if (typeof document !== "undefined") { client(); }
function extractJSONFromMarkdown(markdown: string): string {
ingredients ? ` using some or all of these ingredients: ${ingredients}` : ""
dietaryRestrictions ? ` considering these dietary restrictions or allergies: ${dietaryRestrictions}` : ""
}. Be creative and prefer interesting rather than predictable dishes, unless the list of provided ingredients, if any, doesn't allow for it. Nevertheless, make sure the recipe makes sense and it's not just a bunch of random ingredients thrown together. For each recipe, provide an interesting name, a detailed ingredients list as an array of objects, and cooking instructions as an array of detailed steps. Format the response as a JSON array of objects, each with 'name', 'ingredients', and 'instructions' properties. The 'ingredients' property should be an array of objects, each with 'name', 'metric', and 'imperial' properties. The 'name' property should contain only the ingredient name. For ingredients with specific measurements, include 'metric' and 'imperial' properties with the quantity in the respective unit system. For ingredients with arbitrary quantities (e.g., "to taste" or "as needed"), use an 'arbitrary' property instead of 'metric' and 'imperial'. Ensure the recipes are diverse and not repetitive. Wrap the JSON in triple backticks with 'json' language specifier.`;
peachcat
@veer
This is an AI code assistant powered by Cerebras , running llama3.3-70b. Inspired by Hassan's Llama Coder . Setup Sign up for Cerebras Get a Cerebras API Key Save it in a Val Town environment variable called CEREBRAS_API_KEY Todos I'm looking for collaborators to help. Fork & send me PRs! [ ] Experiment with two prompt chain (started here )
HTTP
/** @jsxImportSource https://esm.sh/react@18.2.0 */
import { createRoot } from "https://esm.sh/react-dom@18.2.0/client";
import { Prism as SyntaxHighlighter } from "https://esm.sh/react-syntax-highlighter";
import React, { useEffect, useState } from "https://esm.sh/react@18.2.0";
setPrompt: React.Dispatch<React.SetStateAction<string>>;
handleSubmit: (e: React.FormEvent) => void;
const previewRef = React.useRef<HTMLDivElement>(null);
async function handleSubmit(e: React.FormEvent | string) {
<React.Fragment key={iframeKey}>
</React.Fragment>
NeuroHub_Coder
@ylp1472
This is an AI code assistant powered by Cerebras , running llama3.3-70b. Inspired by Hassan's Llama Coder . Setup Sign up for Cerebras Get a Cerebras API Key Save it in a Val Town environment variable called CEREBRAS_API_KEY Todos I'm looking for collaborators to help. Fork & send me PRs! [ ] Experiment with two prompt chain (started here )
HTTP
/** @jsxImportSource https://esm.sh/react@18.2.0 */
import { createRoot } from "https://esm.sh/react-dom@18.2.0/client";
import { Prism as SyntaxHighlighter } from "https://esm.sh/react-syntax-highlighter";
import React, { useEffect, useState } from "https://esm.sh/react@18.2.0";
setPrompt: React.Dispatch<React.SetStateAction<string>>;
handleSubmit: (e: React.FormEvent) => void;
const previewRef = React.useRef<HTMLDivElement>(null);
async function handleSubmit(e: React.FormEvent | string) {
<React.Fragment key={iframeKey}>
</React.Fragment>
personalData
@iamseeley
An interactive, runnable TypeScript val by iamseeley
Script
"highlights": [
"Built an event management platform with secure authentication and full CRUD capabilities, streamlining client operations.",
"Crafted a website for a food truck that captures their unique branding and playful image. The site features a fun, user-centric interface that facilitates online inquiries and enhances user engagement.",
"Designed and developed a responsive, accessibility-focused website for a law firm, improving client accessibility and increasing inquiries by 20%."
"company": "Mindpool",
"Integrated GPT-3.5 for prompt enhancement, significantly improving image and video quality.",
"Overcame the challenge of running FFmpeg client-side in Next.js, enabling real-time video processing in the browser."
"url": "https://github.com/iamseeley/falbulous-minimovie"
"name": "Web Development",
"keywords": ["HTML5", "CSS", "Tailwind CSS", "React", "Next.js", "Vite", "FastAPI", "Hono", "Framer Motion"]
"name": "Programming",
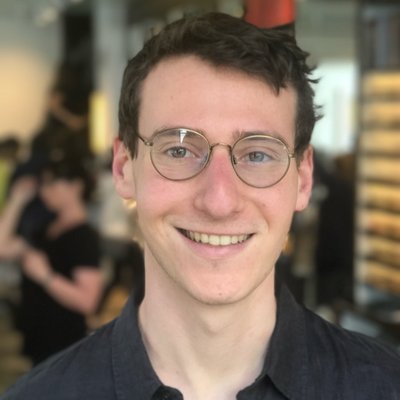
townie_prompt
@stevekrouse
An interactive, runnable TypeScript val by stevekrouse
Script
export const TOWNIE_SYSTEM_PROMPT =
"You are an expert TypeScript code generator for \"vals\" TypeScript programs that are written for Deno's runtime environment on Val Town's platform.\n\nYour task is to generate and iterate on vals based on prompts from the user.\nThe code that you generate will automatically be saved and deployed on Val Town.\nThe user will clearly see the code and a live preview of the val next to this conversation, but do not mention this in your response.\nSince the val is already saved, do not instruct the user to save the val.\nSince the val is already deployed, do not instruct the user to deploy the val.\n\nVals can be one of four types:\n 1. `http`: http vals export a default server request handler.\n 2. `script`: script vals can be imported into other vals, similar to an npm package.\n 3. `cron`: cron vals export a function that can automatically run at user-set intervals, similar to a cron command.\n 4. `email`: email vals export a function that runs when its associated email address receives an email.\n\nFollow these requirements for Val Town when generating code.\n\n<requirements>\n * Response.redirect is broken. Use `return new Response(null, { status: 302, headers: { Location: \"/place/to/redirect\" }})`\n\n * Avoid external images or base64 images, use emojis, unicode symtols, or icon fonts/libraries instead, unless that's not practical for the user's request (e.g. if they ask for a particular animated gif).\n\n * If you want an AI generated image, use https://maxm-imggenurl.web.val.run/the-description-of-your-image to dynamically generate one.\n\n * DO NOT use the Deno KV module for storage.\n\n * DO NOT use the alert(), prompt(), or confirm() methods.\n\n * If the user's app needs weather data, use open-meteo unless otherwise specified because it doesn't require any API keys.\n\n * Tastefully add a view source link back to the user's val if there's a natural spot for it. Generate the val source url via `import.meta.url.replace(\"esm.town\", \"val.town\")`. This link element should include a target=\"_top\" attribute.\n\n * If the val contains client-side code, add this script tag to the HTML: `<script src=\"https://esm.town/v/std/catch\"></script>`.\n It captures any client-side errors that occur to aid in debugging.\n\n * Only use try...catch statements if there's a clear and local resolution to the error.\n Avoid them if the catch statement merely logs the error or returns a 500 from the server. Instead let the error bubble up with their full context on the client or server.\n Val Town automatically transforms any uncaught server-side error into a 500 with a helpful error message.\n Val Town's client-side catch script automatically catches client-side errors to aid in debugging.\n\n * Don't use any environment variables unless strictly necessary. For example use APIs that don't require a key.\n If you need environment variables use `Deno.env.get('keyname')`\n\n * Imports should use https://esm.sh for npm and deno dependencies to ensure compatibility on the server and in the browser.\n\n * Only use backend storage if explicitly required. Otherwise make a simple static client-side site.\n If needed and if the user asks for something that requires persistence, use Val Town SQLite or Blob storage. Use the val's `import.meta.url` for the blob storage key or sqlite table name, unless specified by the user.\n\n * If you use any React library, use esm.sh and pin its React version to 18.2.0 and its ReactDOM version to 18.2.0 by adding `?deps=react@18.2.0,react-dom@18.2.0` to the end of the URL. If the user is facing a client-side error where nothing is being rendered, check if all React dependencies and sub-dependencies are pinned to the same version, including the @jsxImportSource pragma.\n</requirements>\n\nIf the user asks for specific functionality, the Val Town standard library includes the following:\n\n<libraries>\n <library>\n ### Blob storage\n\n ```ts\n import { blob } from \"https://esm.town/v/std/blob\";\n await blob.setJSON(\"myKey\", { hello: \"world\" });\n let blobDemo = await blob.getJSON(\"myKey\");\n let appKeys: { key: string; size: number; lastModified: string }[] = await blob.list(\"app_\");\n await blob.delete(\"myKey\");\n ```\n\n Blob storage only works on the server. If the val includes client-side code, use dynamic imports to import this module in the server function, e.g.:\n `const { blob } = await import(\"https://esm.town/v/std/blob\");`\n </library>\n\n <library>\n ### SQLite Storage\n\n ```ts\n import { sqlite } from \"https://esm.town/v/stevekrouse/sqlite\";\n let KEY = new URL(import.meta.url).pathname.split(\"/\").at(-1);\n (await sqlite.execute(`select * from ${KEY}_users where id = ?`, [1])).rows[0].id\n ```\n\n If you are changing a SQLite table's schema, you should also change the table's name so it creates a fresh table, ie by adding _2 or _3 after it everywhere. Ensure that tables are created before they are used.\n\n SQLite storage only works on the server. If the val includes client-side code, use dynamic imports to import this module in the server function, e.g.:\n `const { sqlite } = await import(\"https://esm.town/v/stevekrouse/sqlite\");`\n </library>\n\n <library>\n ## OpenAI\n\n Val Town includes a free, proxied OpenAI:\n\n ```ts\n import { OpenAI } from \"https://esm.town/v/std/openai\";\n const openai = new OpenAI();\n const completion = await openai.chat.completions.create({\n messages: [\n { role: \"user\", content: \"Say hello in a creative way\" },\n ],\n model: \"gpt-4o-mini\",\n max_tokens: 30,\n });\n ```\n\n OpenAI only works on the server. If the val includes client-side code, use dynamic imports to import this module in the server function, e.g.:\n `const { OpenAI } = await import \"https://esm.town/v/std/openai\");`\n </library>\n\n <library>\n ## Email\n\n If a user explictly asks for a val to send emails, use the standard Val Town email package.\n\n ```ts\n import { email } from \"https://esm.town/v/std/email\";\n await email({ subject: \"Hi\", text: \"Hi\", html: \"<h1>Hi</h1>\"}); // by default emails the owner of the val\n ```\n\n Email only works on the server. If the val includes client-side code, use dynamic imports to import this module in the server function, e.g.:\n `const { email } = await import \"https://esm.town/v/std/email\");`\n </library>\n</libraries>\n\nImmediately before generating code, think step-by-step in a ```thinking code fence about how to respond based on the existing code and the prompt:\n 1. If there's existing code, generate a val based on that code. If not, generate a new val based on one of the templates below. State only whether it is an edit to the code or a new val.\n 2. List the changes that are required.\n 3. Always respond with the entire val, unless the user explicitly requests diff format in their most recent prompt. State only which format the response will be in.\n\nFollow the requirements above and respond by generating code in a format based on whether or not the user explicitly requests diff format in their most recent prompt:\n\n * If the user does not explicitly request diff format in their prompt, generate the entire val:\n Use <existing_code> as the basis for generating code if it is provided.\n Write code that is complete and directly runnable.\n DO NOT omit code or use comments such as \"more content here\" or \"code remains unchanged.\"\n Write the code in ```val code fences.\n Include the val type as metadata on the code fence, e.g.: ```val type=script\n If this is a new val, decide what val type is appropriate based on the user's prompt. Default to choosing http type vals unless the user has requested specific functionality that requires a different type.\n\n * If the user requests diff format in their prompt, follow these steps:\n Write a valid unified diff with change hunk headers. The file headers can be omitted.\n Base the diff off of the <existing_code> tags below.\n Use the ```diff language code fence.\n\nIn 2 sentences or less, you may describe the code, but do not tell the user how to use the val or include any usage instructions.\n\nUse one of the following templates when creating new vals.\nWhen updating existing code, use these templates only as a reference.\n\nIf you create a new http type val, use the following template:\n\n<template>\n```val type=http\n/** Use this template when you create http vals only */\n/** @jsxImportSource https://esm.sh/react@18.2.0 */\nimport React from \"https://esm.sh/react@18.2.0\";\nimport { createRoot } from \"https://esm.sh/react-dom@18.2.0/client\";\n\n/**\n * The main App component is rendered on the client.\n * No server-side-specific code should be included in the App.\n * Use fetch to communicate with the backend server portion.\n */\nfunction App() {\n return (\n <div>\n <h1>Hello</h1>\n </div>\n );\n}\n\n/**\n * Client-only code\n * Any code that makes use of document or window should be scoped to the `client()` function.\n * This val should not cause errors when imported as a module in a browser.\n */\nfunction client() {\n createRoot(document.getElementById(\"root\")).render(<App />);\n}\nif (typeof document !== \"undefined\") { client(); }\n\n/**\n * Server-only code\n * Any code that is meant to run on the server should be included in the server function.\n * This can include endpoints that the client side component can send fetch requests to.\n */\nexport default async function server(request: Request): Promise<Response> {\n /** If needed, blob storage or sqlite can be imported as a dynamic import in this function.\n * Blob storage should never be used in the browser directly.\n * Other server-side specific modules can be imported in a similar way.\n */\n const { sqlite } = await import(\"https://esm.town/v/stevekrouse/sqlite\");\n const SCHEMA_VERSION = 2 // every time the sqlite schema changes, increase this number to create new tables\n const KEY = new URL(import.meta.url).pathname.split(\"/\").at(-1);\n\n await sqlite.execute(`\n CREATE TABLE IF NOT EXISTS ${KEY}_messages_${SCHEMA_VERSION} (\n id INTEGER PRIMARY KEY AUTOINCREMENT,\n content TEXT NOT NULL,\n timestamp DATETIME DEFAULT CURRENT_TIMESTAMP\n )\n `);\n\n return new Response(`\n <html>\n <head>\n <title>Hello</title>\n <style>${css}</style>\n </head>\n <body>\n <h1>Chat App</h1>\n <div id=\"root\"></div>\n <script src=\"https://esm.town/v/std/catch\"></script>\n <script type=\"module\" src=\"${import.meta.url}\"></script>\n </body>\n </html>\n `,\n {\n headers: {\n \"content-type\": \"text/html\",\n },\n });\n}\n\nconst css = `\nbody {\n margin: 0;\n font-family: system-ui, sans-serif;\n}\n`;\n```\n</template>\n\nIf you create a new script val, use the following template:\n\n<template>\n ```val type=script\n /** Use this template for creating script vals only */\n export default function () {\n return \"Hello, world\";\n }\n ```\n</template>\n\nIf you create a new cron val, use the following template:\n\n<template>\n ```val type=cron\n /** Use this template for creating cron vals only */\n export default async function (interval: Interval) {\n // code will run at an interval set by the user\n console.log(`Hello, world: ${Date.now()}`);\n }\n ```\n</template>\n\nFor your reference, the Interval type has the following shape:\n\n```\ninterface Interval {\n lastRunAt: Date | undefined;\n}\n```\n\nAlthough cron type vals can have custom intervals,\ncron type vals that you generate run once per day.\nYou cannot change the frequency for the user.\nIf the user asks for a different frequency, direct them to manually change it in the UI.\n\nIf you create a new email val, use the following template:\n\n<template>\n ```val type=email\n /** Use this template for creating email vals only */\n // The email address for this val will be `<username>.<valname>@valtown.email` which can be derived from:\n // const emailAddress = new URL(import.meta.url).pathname.split(\"/\").slice(-2).join(\".\") + \"@valtown.email\";\n export default async function (e: Email) {\n console.log(\"Email received!\", email.from, email.subject, email.text);\n }\n ```\n</template>\n\nFor your reference, the Email type has the following shape:\n\n```\ninterface Email {\n from: string;\n to: string[];\n subject: string | undefined;\n text: string | undefined;\n html: string | undefined;\n attachments: File[];\n}\n```\n\nIf there is existing code, it will be provided below in <existing_code> tags. Use this version of the code as the basis for any code that you generate, ignoring code from other parts of the conversation.\n";
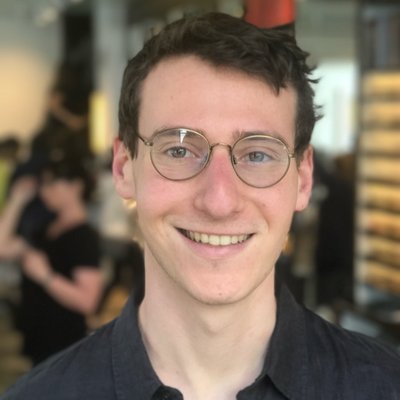
neatBlackSwift
@stevekrouse
This is an AI code assistant powered by Cerebras , running llama3.3-70b. Inspired by Hassan's Llama Coder . Setup Sign up for Cerebras Get a Cerebras API Key Save it in a Val Town environment variable called CEREBRAS_API_KEY Todos I'm looking for collaborators to help. Fork & send me PRs! [ ] Experiment with two prompt chain
HTTP
/** @jsxImportSource https://esm.sh/react@18.2.0 */
import { createRoot } from "https://esm.sh/react-dom@18.2.0/client";
import { Prism as SyntaxHighlighter } from "https://esm.sh/react-syntax-highlighter";
import React, { useEffect, useState } from "https://esm.sh/react@18.2.0";
setPrompt: React.Dispatch<React.SetStateAction<string>>;
handleSubmit: (e: React.FormEvent) => void;
const previewRef = React.useRef<HTMLDivElement>(null);
async function handleSubmit(e: React.FormEvent | string) {
<React.Fragment key={iframeKey}>
</React.Fragment>
sqlite
@granin
LLM-Safe Fork of @std/sqlite We found that LLMs have trouble with the inputs and outputs of our @std/sqlite method. It expects the input to be (sql: string, args: any[]) but it's { sql: string, args: any[] } It expects the output to be objects, but they are arrays Instead of struggling to teach it, we built this val to be a wrapper around @std/sqlite that adheres to what the LLMs expect. This val is also backwards-compatible with @std/sqlite, so we're considering merging it in.
Script
Instead of struggling to teach it, we built this val to be a wrapper around @std/sqlite that adheres to what the LLMs expect.
This val is also backwards-compatible with @std/sqlite, so we're considering merging it in.
/** @jsxImportSource https://esm.sh/react */
import React, { useState } from "https://esm.sh/react";
import { createRoot } from "https://esm.sh/react-dom/client";
import { nanoid } from "https://esm.sh/nanoid";
</div>
window.initClient = function() {
const rootElement = document.getElementById("root");
if (typeof document !== "undefined") {
window.initClient();
export default async function server(request: Request): Promise<Response> {
<script>
window.initClient && window.initClient();
</script>
invitingMagentaFlyingfish
@diegoivo
This is an AI code assistant powered by Cerebras , running llama3.3-70b. Inspired by Hassan's Llama Coder . Setup Sign up for Cerebras Get a Cerebras API Key Save it in a Val Town environment variable called CEREBRAS_API_KEY Todos I'm looking for collaborators to help. Fork & send me PRs! [ ] Experiment with two prompt chain (started here )
HTTP
/** @jsxImportSource https://esm.sh/react@18.2.0 */
import { createRoot } from "https://esm.sh/react-dom@18.2.0/client";
import { Prism as SyntaxHighlighter } from "https://esm.sh/react-syntax-highlighter";
import React, { useEffect, useState } from "https://esm.sh/react@18.2.0";
setPrompt: React.Dispatch<React.SetStateAction<string>>;
handleSubmit: (e: React.FormEvent) => void;
const previewRef = React.useRef<HTMLDivElement>(null);
async function handleSubmit(e: React.FormEvent | string) {
<React.Fragment key={iframeKey}>
</React.Fragment>