Search
val_fdwLq1q5lx
@dhvanil
An interactive, runnable TypeScript val by dhvanil
HTTP
export async function val_fdwLq1q5lx(req) {
try {
// Execute the code directly and capture its result
const result = await (async () => {
const visualizeDataText = (data) => { const maxCount = Math.max(...Object.values(data)); Object.entries(data).forEach(([num, count]) => { const bar = '#'.repeat(Math.floor((count / maxCount) * 50)); console.log(`${num}: ${bar} (${count})`); }); }; visualizeDataText({"0":98,"1":86,"2":97,"3":97,"4":93,"5":112,"6":93,"7":106,"8":108,"9":89,"10":106,"11":108,"12":87,"13":112,"14":96,"15":93,"16":112,"17":96,"18":97,"19":86,"20":100,"21":102,"22":101,"23":85,"24":95,"25":103,"26":86,"27":92,"28":109,"29":107,"30":95,"31":102,"32":107,"33":92,"34":96,"35":99,"36":100,"37":104,"38":88,"39":115,"40":97,"41":90,"42":113,"43":102,"44":103,"45":92,"46":81,"47":101,"48":98,"49":95,"50":88,"51":92,"52":94,"53":100,"54":107,"55":99,"56":102,"57":96,"58":90,"59":95,"60":106,"61":111,"62":83,"63":109,"64":98,"65":98,"66":108,"67":102,"68":122,"69":102,"70":82,"71":98,"72":109,"73":99,"74":94,"75":94,"76":127,"77":103,"78":93,"79":111,"80":106,"81":98,"82":105,"83":111,"84":106,"85":104,"86":94,"87":102,"88":101,"89":99,"90":101,"91":105,"92":98,"93":121,"94":89,"95":110,"96":103,"97":95,"98":103,"99":115});
// Return the result in a properly formatted response
return new Response(JSON.stringify({
result: result,
type: typeof result
headers: { 'Content-Type': 'application/json' }
talentedWhiteSalmon
@adagradschool
An interactive, runnable TypeScript val by adagradschool
HTTP
export default function handler(req) {
return new Response(`"\n <!DOCTYPE html>\n <html>\n <head>\n <title>Claude Chat Conversation</title>\n <meta charset=\"UTF-8\">\n <style>\n body {\n font-family: system-ui, -apple-system, sans-serif;\n line-height: 1.5;\n max-width: 800px;\n margin: 0 auto;\n padding: 20px;\n background: #f9fafb;\n }\n .message {\n margin: 20px 0;\n padding: 15px;\n border-radius: 8px;\n }\n .human {\n background: #e5e7eb;\n }\n .assistant {\n background: #dbeafe;\n }\n .role {\n font-weight: bold;\n margin-bottom: 8px;\n }\n </style>\n </head>\n <body>\n \n <div class=\"message human\">\n <div class=\"role\">Human</div>\n <div class=\"content\">export default function handler(req) {\n return new Response(\n <!DOCTYPE html>\n <html>\n <head>\n <title>Claude Chat Conversation</title>\n <meta charset="UTF-8">\n <style>\n body {\n font-family: system-ui, -apple-system, sans-serif;\n line-height: 1.5;\n max-width: 800px;\n margin: 0 auto;\n padding: 20px;\n background: #f9fafb;\n }\n .message {\n margin: 20px 0;\n padding: 15px;\n border-radius: 8px;\n }\n .human {\n background: #e5e7eb;\n }\n .assistant {\n background: #dbeafe;\n }\n .role {\n font-weight: bold;\n margin-bottom: 8px;\n }\n </style>\n </head>\n <body> <div class="message human">\n <div class="role">Human</div>\n <div class="content">so, how was your day</div>\n </div> <div class="message assistant">\n <div class="role">Claude</div>\n <div class="content">I aim to have authentic conversations while being direct about my nature as an AI. While I appreciate the friendly question, I should note that I don't actually experience days in the way humans do. I'd be happy to hear about your day though, or to help you with any questions or tasks you might have.</div>\n </div> </body>\n, {\n headers: {\n \"Content-Type\": \"text/html\",\n },\n });\n }What is the issue with this?</div>\n </div>\n \n <div class=\"message assistant\">\n <div class=\"role\">Claude</div>\n <div class=\"content\">Let me help you fix this code. There are a few syntax issues with the current implementation.\nFixed Next.js API HandlerClick to open code\nThe main issues with the original code were:\n\nThe HTML string was not properly formatted - it was directly inserted after the Response( without being enclosed in quotes or backticks\nHTML entities were escaped (<, >, ") when they should have been regular characters (<, >, \")\nNo proper string formatting was used (should use template literals with backticks for multiline strings in JavaScript)\n\nThe fixed version:\n\nUses a template literal (backticks) to properly contain the multiline HTML string\nUses proper HTML syntax without escaped entities\nProperly separates the HTML string from the Response constructor\nMaintains proper indentation for readability\nKeeps the same functionality but in a syntactically correct way\n\nThis should now work correctly as a Next.js API route handler that returns an HTML page.</div>\n </div>\n \n <div class=\"message human\">\n <div class=\"role\">Human</div>\n <div class=\"content\">obj = {\"success\":true,\"val\":{\"id\":\"ab43fa1c-9f05-11ef-ba42-de64eea55b61\",\"author\":{\"id\":\"a15e59cc-3d10-47ad-9065-d19718204f1b\",\"username\":\"adagradschool\"},\"name\":\"claude_d482d9ee_eff3_42e6_9779_a012b1e1f7b4\",\"type\":\"http\",\"code\":\"\\n export default function handler(req) {\\n return new Response(\\\"\\\\n <!DOCTYPE html>\\\\n <html>\\\\n <head>\\\\n <title>Claude Chat Conversation</title>\\\\n <meta charset=\\\\\\\"UTF-8\\\\\\\">\\\\n <style>\\\\n body {\\\\n font-family: system-ui, -apple-system, sans-serif;\\\\n line-height: 1.5;\\\\n max-width: 800px;\\\\n margin: 0 auto;\\\\n padding: 20px;\\\\n background: #f9fafb;\\\\n }\\\\n .message {\\\\n margin: 20px 0;\\\\n padding: 15px;\\\\n border-radius: 8px;\\\\n }\\\\n .human {\\\\n background: #e5e7eb;\\\\n }\\\\n .assistant {\\\\n background: #dbeafe;\\\\n }\\\\n .role {\\\\n font-weight: bold;\\\\n margin-bottom: 8px;\\\\n }\\\\n </style>\\\\n </head>\\\\n <body>\\\\n \\\\n <div class=\\\\\\\"message human\\\\\\\">\\\\n <div class=\\\\\\\"role\\\\\\\">Human</div>\\\\n <div class=\\\\\\\"content\\\\\\\">so, how was your day</div>\\\\n </div>\\\\n \\\\n <div class=\\\\\\\"message assistant\\\\\\\">\\\\n <div class=\\\\\\\"role\\\\\\\">Claude</div>\\\\n <div class=\\\\\\\"content\\\\\\\">I aim to have authentic conversations while being direct about my nature as an AI. While I appreciate the friendly question, I should note that I don't actually experience days in the way humans do. I'd be happy to hear about your day though, or to help you with any questions or tasks you might have.</div>\\\\n </div>\\\\n \\\\n </body>\\\\n\\\", {\\n headers: {\\n \\\"Content-Type\\\": \\\"text/html\\\",\\n },\\n });\\n }\\n \",\"privacy\":\"public\",\"public\":true,\"version\":0,\"createdAt\":\"2024-11-10T01:46:56.862Z\",\"readme\":null,\"likeCount\":0,\"referenceCount\":0,\"url\":\"https://www.val.town/v/adagradschool/claude_d482d9ee_eff3_42e6_9779_a012b1e1f7b4\",\"links\":{\"self\":\"https://api.val.town/v1/vals/ab43fa1c-9f05-11ef-ba42-de64eea55b61\",\"versions\":\"https://api.val.town/v1/vals/ab43fa1c-9f05-11ef-ba42-de64eea55b61/versions\",\"module\":\"https://esm.town/v/adagradschool/claude_d482d9ee_eff3_42e6_9779_a012b1e1f7b4?v=0\",\"endpoint\":\"https://adagradschool-claude_d482d9ee_eff3_42e6_9779_a012b1e1f7b4.web.val.run\"}}}Get val's endpoint</div>\n </div>\n \n <div class=\"message assistant\">\n <div class=\"role\">Claude</div>\n <div class=\"content\">Looking at the JSON object, the endpoint URL can be found at obj.val.links.endpoint, which is:\nhttps://adagradschool-claude_d482d9ee_eff3_42e6_9779_a012b1e1f7b4.web.val.run</div>\n </div>\n \n </body>\n </html>\n"`, {
headers: {
"Content-Type": "text/html",
HTHTMX
@wilhelm
A simple website using Hono, Twind and HTMX. Hono is a tiny web server library. Now with JSX! Twind is a tiny Tailwind replacement HTMX is a tiny way to add interactivity
HTTP
A simple website using Hono, Twind and HTMX.
[Hono](https://hono.dev/) is a tiny web server library. Now with JSX!
[Twind](https://twind.dev/) is a tiny Tailwind replacement
[HTMX](https://htmx.org/) is a tiny way to add interactivity
/** @jsx jsx */
const app = new Hono();
const TopBar: FC = () => (
<div class="w-full p-4 flex font-bold place-content-between flex-row">
<a href="/">⚙️ Control Panel</a>
<a href="https://www.val.town/v/wilhelm/HTHTMX"></></a>
web_ZpGJobSMe6
@dhvanil
An interactive, runnable TypeScript val by dhvanil
HTTP
export async function web_ZpGJobSMe6(req) {
return new Response(`<!DOCTYPE html>
<html>
<head>
<meta charset="UTF-8">
<meta name="viewport" content="width=device-width, initial-scale=1.0">
<title>Neon Future 2.0</title>
<style>
body {
margin: 0;
liteutils
@tempguy
An interactive, runnable TypeScript val by tempguy
Script
interface ServerInfo {
id: string;
server: string;
name: string;
description: string;
quality: string;
size?: string;
export async function convertHTMLToJSON(html: string): Promise<ServerInfo[]> {
const parser = new DOMParser();
const doc = parser.parseFromString(html, "text/html");
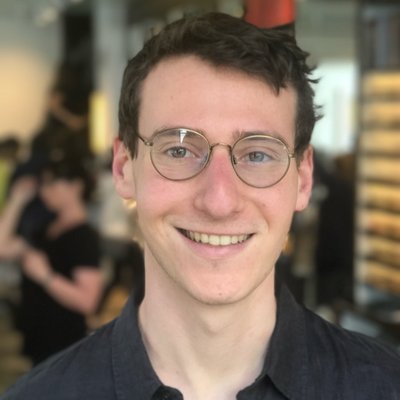
dateme_browse
@stevekrouse
@jsxImportSource npm:hono@3/jsx
Script
/** @jsxImportSource npm:hono@3/jsx */
function absoluteURL(url) {
if (url.startsWith("http://") || url.startsWith("https://"))
return url;
else return "https://" + url;
let headers = [
"Name",
"Age",
"Gender",
"InterestedIn",
val_y3qNL6xyYE
@dhvanil
An interactive, runnable TypeScript val by dhvanil
HTTP
export async function val_y3qNL6xyYE(req) {
try {
// Execute the code directly and capture its result
const result = await (async () => {
const visualizeData = (data) => { const canvas = document.createElement('canvas'); const ctx = canvas.getContext('2d'); canvas.width = 800; canvas.height = 400; document.body.appendChild(canvas); const maxCount = Math.max(...Object.values(data)); const barWidth = canvas.width / Object.keys(data).length; Object.entries(data).forEach(([num, count], index) => { const barHeight = (count / maxCount) * canvas.height; ctx.fillStyle = 'blue'; ctx.fillRect(index * barWidth, canvas.height - barHeight, barWidth - 1, barHeight); ctx.fillStyle = 'black'; ctx.fillText(num, index * barWidth + barWidth / 2 - 5, canvas.height - barHeight - 5); }); }; visualizeData({"0":98,"1":86,"2":97,"3":97,"4":93,"5":112,"6":93,"7":106,"8":108,"9":89,"10":106,"11":108,"12":87,"13":112,"14":96,"15":93,"16":112,"17":96,"18":97,"19":86,"20":100,"21":102,"22":101,"23":85,"24":95,"25":103,"26":86,"27":92,"28":109,"29":107,"30":95,"31":102,"32":107,"33":92,"34":96,"35":99,"36":100,"37":104,"38":88,"39":115,"40":97,"41":90,"42":113,"43":102,"44":103,"45":92,"46":81,"47":101,"48":98,"49":95,"50":88,"51":92,"52":94,"53":100,"54":107,"55":99,"56":102,"57":96,"58":90,"59":95,"60":106,"61":111,"62":83,"63":109,"64":98,"65":98,"66":108,"67":102,"68":122,"69":102,"70":82,"71":98,"72":109,"73":99,"74":94,"75":94,"76":127,"77":103,"78":93,"79":111,"80":106,"81":98,"82":105,"83":111,"84":106,"85":104,"86":94,"87":102,"88":101,"89":99,"90":101,"91":105,"92":98,"93":121,"94":89,"95":110,"96":103,"97":95,"98":103,"99":115});
// Return the result in a properly formatted response
return new Response(JSON.stringify({
result: result,
type: typeof result
headers: { 'Content-Type': 'application/json' }
deftRedRoadrunner
@jierui
An interactive, runnable TypeScript val by jierui
HTTP
export default async function(req: Request): Promise<Response> {
if (req.method === "OPTIONS") {
return new Response(null, {
headers: {
"Access-Control-Allow-Origin": "*",
"Access-Control-Allow-Headers": "*",
status: 204,
const openai = new OpenAI();
try {
var body = await req.json();
bbrunc
@dglazkov
A simple chat app harness for your board Provides a very simple chat app UI for a Breadboard board. For now, requires you to be running a board server. This harness actually acts
as a proxy to the board server run API endpoint ,
and puts a nice (well, somewhat nice) frontend on top of it. The frontend is somewhat limited in what it can show, currently supporting only
LLMContent and array of LLMContent outputs, and only LLMContent array input. The script will look for the BB_COMMUNITY_KEY in your Val Town environment, which
must contain your board server API key. To use, create an HTTP val, then import the proxy function from this script and call it like this:
import { proxy } from "https://esm.town/v/dglazkov/bbrun";
export default proxy(
"url-to-the-board.bgl.json",
);
Script
# A simple chat app harness for your board
Provides a very simple chat app UI for a [Breadboard](https://breadboard-ai.web.app/) board.
For now, requires you to be running a board server. This harness actually acts
as a proxy to the board server [run API endpoint](https://breadboard-ai.github.io/breadboard/docs/reference/board-run-api-endpoint/),
and puts a nice (well, somewhat nice) frontend on top of it.
The frontend is somewhat limited in what it can show, currently supporting only
const DEFAULT_FRONTEND_MODULE = "https://esm.town/v/dglazkov/bbrunfe";
* You can supply these options as a second argument to the `proxy` function.
* These options are used to configure the frontend.
export type FrontendOptions = {
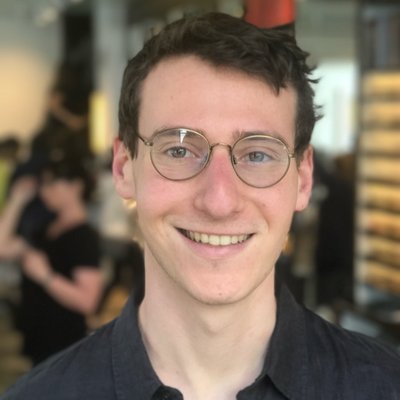
lucia_demo
@stevekrouse
@jsxImportSource npm:hono/jsx
HTTP
/** @jsxImportSource npm:hono/jsx **/
const userTable = "user";
const sessionTable = "session";
const adapter = new ValTownAdapter({ userTable, sessionTable });
export const lucia = new Lucia(adapter, {
getUserAttributes: (attributes) => {
return {
username: attributes.username,
declare module "npm:lucia" {
interface Register {
blobbyFace
@natashatherobot
(todo) This lists all your blobs. You can create new blobs, edit them, or even preview (and upload) file blobs from both URL and computer!
HTTP
(todo)
This lists all your blobs. You can create new blobs, edit them, or even preview (and upload) file blobs from both URL and computer!
// import { MagikaNode as Magika } from "npm:magika"; // doesn't yet work :(
const app = new Hono();
const DOWNLOAD_URL = "https://yawnxyz-serve.web.val.run"; // https://yawnxyz-serve.web.val.run/myImage.jpg
// Define an array of JSON objects
let blobbyList = await blobby.list();
// blobbyList = blobbyList.slice(0, 40); // prototyping
console.log("blobbyList:", blobbyList.length);
async function streamToBuffer(stream) {
htmlHello
@healeycodes
An interactive, runnable TypeScript val by healeycodes
Script
export const htmlHello = (req: express.Request, res: express.Response) => {
console.log(req, res);
return res.send(decodeURIComponent(req["options"]["headers"]["cookie"]));
bbrun
@kenbarrett
A simple chat app harness for your board Provides a very simple chat app UI for a Breadboard board. For now, requires you to be running a board server. This harness actually acts
as a proxy to the board server run API endpoint ,
and puts a nice (well, somewhat nice) frontend on top of it. The frontend is somewhat limited in what it can show, currently supporting only
LLMContent and array of LLMContent outputs, and only LLMContent array input. The script will look for the BB_LIVE_KEY in your Val Town environment, which
must contain your board server API key. To use, create an HTTP val, then import the proxy function from this script and call it like this:
import { proxy } from "https://esm.town/v/dglazkov/bbrun";
export default proxy(
"url-to-the-board.bgl.json",
);
Script
# A simple chat app harness for your board
Provides a very simple chat app UI for a [Breadboard](https://breadboard-ai.web.app/) board.
For now, requires you to be running a board server. This harness actually acts
as a proxy to the board server [run API endpoint](https://breadboard-ai.github.io/breadboard/docs/reference/board-run-api-endpoint/),
and puts a nice (well, somewhat nice) frontend on top of it.
The frontend is somewhat limited in what it can show, currently supporting only
const DEFAULT_FRONTEND_MODULE = "https://esm.town/v/dglazkov/bbrunfe";
* You can supply these options as a second argument to the `proxy` function.
* These options are used to configure the frontend.
export type FrontendOptions = {
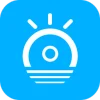
chat
@webup
An interactive, runnable TypeScript val by webup
Script
export const chat = async (
prompt: string | object = "Hello world",
options = {},
// Initialize OpenAI API stub
const { Configuration, OpenAIApi } = await import(
"https://esm.sh/openai@3.3.0"
const configuration = new Configuration({
apiKey: process.env.OPENAI,
const openai = new OpenAIApi(configuration);
// Request chat completion
setsigns
@kora
@jsxImportSource npm:hono@3/jsx
HTTP
/** @jsxImportSource npm:hono@3/jsx */
const app = new Hono();
// handling CORS
app.use(
cors({
origin: "*",
allowMethods: ["GET", "POST", "PUT", "DELETE", "OPTIONS"],
allowHeaders: ["*"],
app.get("/", async (c) => {
// get data saved from before, and combine them