Search
shittyValTownUI
@vez
An interactive, runnable TypeScript val by vez
Script
export async function shittyValTownUI(req, res) {
const editVal = (body: {
code: string;
name?: string;
isInterval?: boolean;
`fetch('https://api.val.town/eval/@vez.editVal(${JSON.stringify(body)})')`;
return res.send(`<h1>Shitty Val Town 2</h1>
${(await getAllVals("@vez")).map(({ code, name }) => {
const editorId = `${name}-editor`;
const onClick = `(document.getElementById('${name}-button').textContent = 'save 🔄'), fetch('https://api.val.town/eval/@vez.editVal', { method: 'POST', body: JSON.stringify({ name: '${name}', code: document.getElementById('${editorId}').value })}).then(()=>(document.getElementById('${name}-button').textContent = 'save ✅'))`;
val_6ZdZ89Aqqh
@dhvanil
An interactive, runnable TypeScript val by dhvanil
HTTP
export async function val_6ZdZ89Aqqh(req) {
try {
// Execute the code directly and capture its result
const result = await (async () => {
const calculateFractalDimension = (points) => { const boxCount = (size) => { let count = 0; for (let i = 0; i < points.length; i++) { if (points[i].x < size && points[i].y < size) count++; } return count; }; const sizes = [1, 2, 4, 8, 16]; const counts = sizes.map(size => boxCount(size)); const logCounts = counts.map(count => Math.log(count)); const logSizes = sizes.map(size => Math.log(size)); const slope = (logCounts[1] - logCounts[0]) / (logSizes[1] - logSizes[0]); return -slope; }; const mandelbrotPoints = [/* Add points from the Mandelbrot set */]; const sierpinskiPoints = [/* Add points from the Sierpinski triangle */]; return { mandelbrotDimension: calculateFractalDimension(mandelbrotPoints), sierpinskiDimension: calculateFractalDimension(sierpinskiPoints) };
// Return the result in a properly formatted response
return new Response(JSON.stringify({
result: result,
type: typeof result
headers: { 'Content-Type': 'application/json' }
immenseBlushSpider
@ngmi
An interactive, runnable TypeScript val by ngmi
HTTP
const API_KEY = Deno.env.get("FARCASTER_API_KEY");
const API_URL = "https://build.wield.xyz/farcaster/v2/feed";
export default async function server(request: Request): Promise<Response> {
let feedItems = [];
let error = null;
if (!API_KEY) {
error = "API key is not set. Please configure the FARCASTER_API_KEY environment variable.";
} else {
async function fetchFeedItems() {
try {
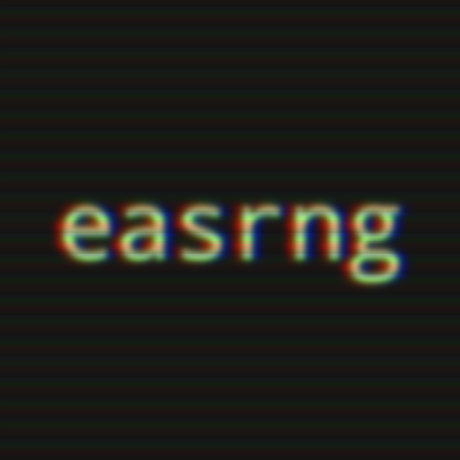
playground
@easrng
playground edit, run, and embed vals without requiring an account (or even js enabled!) caveats: logs don't stream I haven't set up codemirror only script vals supported everything else should be fully functional. you can prefill the editor with code:
https://easrng-playground.web.val.run/?code=console.log(1) a val:
https://easrng-playground.web.val.run/?load=easrng/playground some other url: https://easrng-playground.web.val.run/?load=https://any/other/url
HTTP
# playground
## edit, run, and embed vals without requiring an account (or even js enabled!)
[](https://easrng-playground.web.val.run/)
caveats:
- logs don't stream
- I haven't set up codemirror
// (c) easrng 2024 all rights reserved
/** @jsx jsx */
/** @jsxFrag Fragment */
createContext,
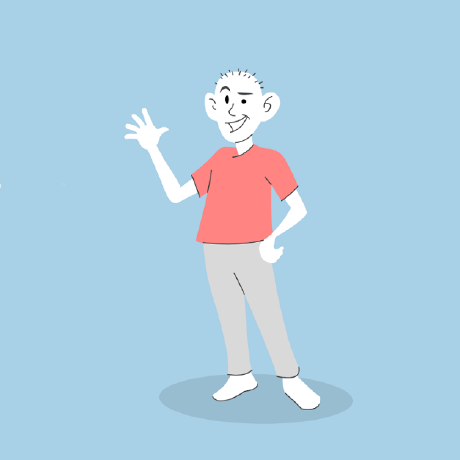
njuskaloCreateHtmlResponse
@kajgod
An interactive, runnable TypeScript val by kajgod
Script
export const njuskaloCreateHtmlResponse = (
res: express.Response,
formHtml: string,
dataHtml: string,
res.send(`
<!DOCTYPE html>
<html>
<head>
<title>Njuškalo sniffer</title>
</head>
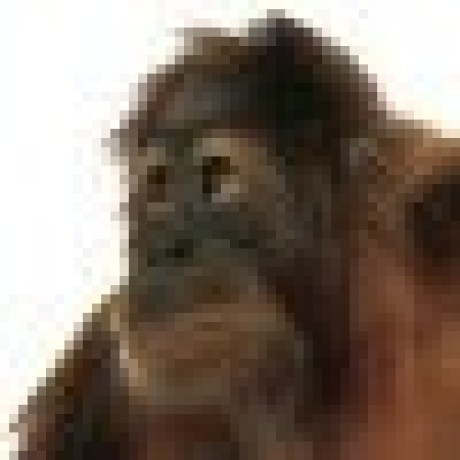
password_auth
@pomdtr
Password Auth Middleware Protect your vals behind a password. Use session cookies to persist authentication. Usage import { passwordAuth } from "https://esm.town/v/pomdtr/password_auth?v=84";
export default passwordAuth(() => {
return new Response("OK");
}, { verifyPassword: (password) => password == Deno.env.get("VAL_PASSWORD") }); If you want to use an api token to authenticate: import { passwordAuth } from "https://esm.town/v/pomdtr/password_auth?v=84";
import { verifyToken } from "https://esm.town/v/pomdtr/verifyToken";
export default passwordAuth(() => {
return new Response("OK");
}, { verifyPassword: verifyToken }); TODO [x] allow to authenticate using a val town token [ ] add a way to send an email to ask a password from the val owner [ ] automatically extend the session [ ] automatically remove expired sessions FAQ How to sign out ? Navigate to <your-site>/signout .
Script
# Password Auth Middleware
Protect your vals behind a password. Use session cookies to persist authentication.
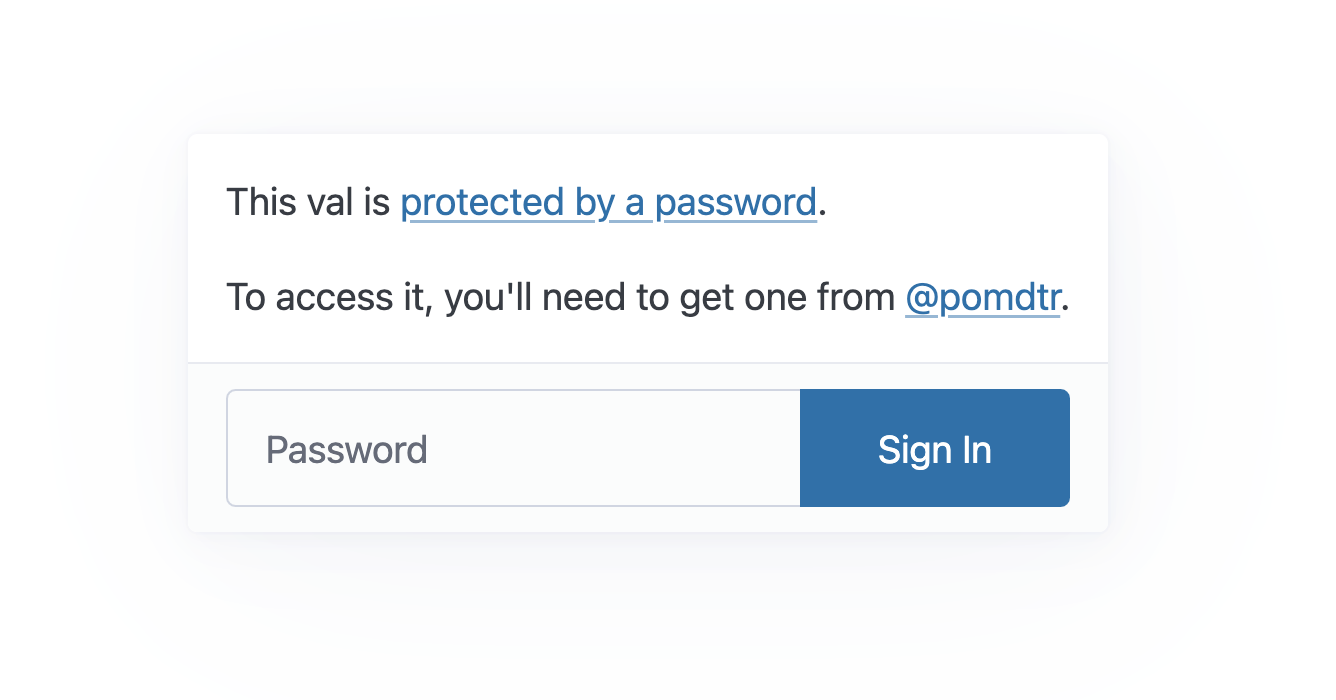
## Usage
```ts
export default passwordAuth(() => {
type Session = {
id: string;
expiresAt: number;
async function createSessionTable(tableName: string) {
val_prZ5olhiXD
@dhvanil
An interactive, runnable TypeScript val by dhvanil
HTTP
export default async function handler(req) {
try {
const result = await (async () => {
return 100;
return Response.json({ success: true, result });
} catch (error) {
return Response.json({
success: false,
error: error.message
}, { status: 500 });
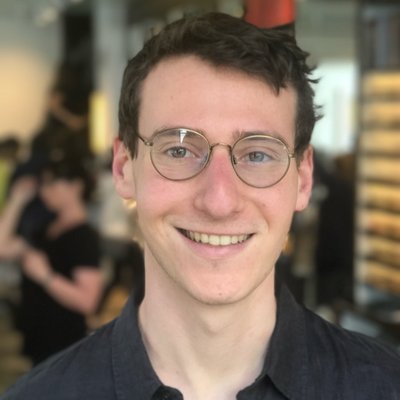
qr
@stevekrouse
// Forked from @ramkarthik.GenerateQR
Express (deprecated)
export async function qr(req: express.Request, res: express.Response) {
if (req.path.startsWith("/qr")) {
const { qrcode } = await import("https://deno.land/x/qrcode/mod.ts");
const base64Image = await qrcode(req.query.data);
res.set("content-type", "image/gif");
res.send(
Buffer.from(base64Image.replace("data:image/gif;base64,", ""), "base64"),
else {
res.send(`<html>
<div>
valle_tmp_463480496454860730332801536131093
@janpaul123
// This updated val serves an HTML page emulating a Hacker News clone.
HTTP
// This updated val serves an HTML page emulating a Hacker News clone.
// It now has clickable titles that will serve pages with some fake story content.
export default async function(req: Request): Promise<Response> {
const url = new URL(req.url);
const path = url.pathname;
if (path === "/") {
const htmlContent = `
<!DOCTYPE html>
<html lang="en">
<head>
val_RcFf3oHVQl
@dhvanil
An interactive, runnable TypeScript val by dhvanil
HTTP
export async function val_RcFf3oHVQl(req) {
try {
// Execute the code directly and capture its result
const result = await (async () => {
// Exploit proof-of-concept for matrix precision glitch
const exploitPrecisionGlitch = () => {
// Create a function that forces values into specific rational states
const forceValueSnap = (value, target) => {
// Use extremely high precision to force rational interpretation
const precision = 1000000000000;
val_4TWAFb0mDp
@dhvanil
An interactive, runnable TypeScript val by dhvanil
HTTP
export async function val_4TWAFb0mDp(req) {
try {
// Execute the code directly and capture its result
const result = await (async () => {
const calculateFractalDimension = (points) => { const boxCount = (size) => { let count = 0; for (let i = 0; i < points.length; i++) { if (points[i].x < size && points[i].y < size) count++; } return count; }; const sizes = [1, 2, 4, 8, 16]; const counts = sizes.map(size => boxCount(size)); const logCounts = counts.map(count => Math.log(count)); const logSizes = sizes.map(size => Math.log(size)); const slope = (logCounts[1] - logCounts[0]) / (logSizes[1] - logSizes[0]); return -slope; }; const mandelbrotPoints = [/* Add points from the Mandelbrot set */]; const sierpinskiPoints = [/* Add points from the Sierpinski triangle */]; return { mandelbrotDimension: calculateFractalDimension(mandelbrotPoints), sierpinskiDimension: calculateFractalDimension(sierpinskiPoints) };
// Return the result in a properly formatted response
return new Response(JSON.stringify({
result: result,
type: typeof result
headers: { 'Content-Type': 'application/json' }
kindApricotAnglerfish
@adagradschool
An interactive, runnable TypeScript val by adagradschool
HTTP
export default function handler(req) {
return new Response(`"\n <!DOCTYPE html>\n <html>\n <head>\n <title>Claude Chat Conversation</title>\n <meta charset=\"UTF-8\">\n <style>\n body {\n font-family: system-ui, -apple-system, sans-serif;\n line-height: 1.5;\n max-width: 800px;\n margin: 0 auto;\n padding: 20px;\n background: #f9fafb;\n }\n .message {\n margin: 20px 0;\n padding: 15px;\n border-radius: 8px;\n }\n .human {\n background: #e5e7eb;\n }\n .assistant {\n background: #dbeafe;\n }\n .role {\n font-weight: bold;\n margin-bottom: 8px;\n }\n </style>\n </head>\n <body>\n \n <div class=\"message human\">\n <div class=\"role\">Human</div>\n <div class=\"content\">export default function handler(req) {\n return new Response(\n <!DOCTYPE html>\n <html>\n <head>\n <title>Claude Chat Conversation</title>\n <meta charset="UTF-8">\n <style>\n body {\n font-family: system-ui, -apple-system, sans-serif;\n line-height: 1.5;\n max-width: 800px;\n margin: 0 auto;\n padding: 20px;\n background: #f9fafb;\n }\n .message {\n margin: 20px 0;\n padding: 15px;\n border-radius: 8px;\n }\n .human {\n background: #e5e7eb;\n }\n .assistant {\n background: #dbeafe;\n }\n .role {\n font-weight: bold;\n margin-bottom: 8px;\n }\n </style>\n </head>\n <body> <div class="message human">\n <div class="role">Human</div>\n <div class="content">so, how was your day</div>\n </div> <div class="message assistant">\n <div class="role">Claude</div>\n <div class="content">I aim to have authentic conversations while being direct about my nature as an AI. While I appreciate the friendly question, I should note that I don't actually experience days in the way humans do. I'd be happy to hear about your day though, or to help you with any questions or tasks you might have.</div>\n </div> </body>\n, {\n headers: {\n \"Content-Type\": \"text/html\",\n },\n });\n }What is the issue with this?</div>\n </div>\n \n <div class=\"message assistant\">\n <div class=\"role\">Claude</div>\n <div class=\"content\">Let me help you fix this code. There are a few syntax issues with the current implementation.\nFixed Next.js API HandlerClick to open code\nThe main issues with the original code were:\n\nThe HTML string was not properly formatted - it was directly inserted after the Response( without being enclosed in quotes or backticks\nHTML entities were escaped (<, >, ") when they should have been regular characters (<, >, \")\nNo proper string formatting was used (should use template literals with backticks for multiline strings in JavaScript)\n\nThe fixed version:\n\nUses a template literal (backticks) to properly contain the multiline HTML string\nUses proper HTML syntax without escaped entities\nProperly separates the HTML string from the Response constructor\nMaintains proper indentation for readability\nKeeps the same functionality but in a syntactically correct way\n\nThis should now work correctly as a Next.js API route handler that returns an HTML page.</div>\n </div>\n \n <div class=\"message assistant\">\n <div class=\"role\">Claude</div>\n <div class=\"content\">Looking at the JSON object, the endpoint URL can be found at obj.val.links.endpoint, which is:\nhttps://adagradschool-claude_d482d9ee_eff3_42e6_9779_a012b1e1f7b4.web.val.run</div>\n </div>\n \n </body>\n </html>\n"`, {
headers: {
"Content-Type": "text/html",
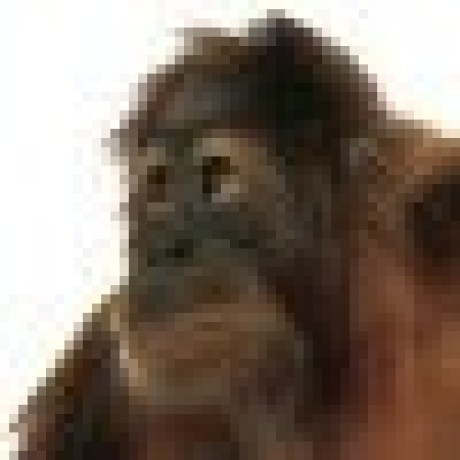
blob_admin_blob
@pomdtr
@jsxImportSource https://esm.sh/hono@4.0.8/jsx
Script
/** @jsxImportSource https://esm.sh/hono@4.0.8/jsx **/
const route = new Hono();
route.get("/:key", async (c) => {
const key = c.req.param("key");
let response = await blob.get(key);
let text = await response.text();
return c.render(
<div>
<h1>{key}</h1>
<code-mirror code={text} readonly></code-mirror>
valle_tmp_821221338019192827080648147114728
@janpaul123
// This HTTP val will generate an HTML frontend for a simple Hacker News clone.
HTTP
// This HTTP val will generate an HTML frontend for a simple Hacker News clone.
// It will use hardcoded example stories for now and display them in a clean layout.
const app = new Hono();
const exampleStories = [
title: "Introducing Val Town",
url: "https://val.town",
points: 100,
author: "Steve Krouse",
comments: 30,
title: "Hacker News Clone with Val Town",