Search
scrapecapfriendly
@brianleroux
An interactive, runnable TypeScript val by brianleroux
HTTP
export async function handler(request: Request) {
let url = "https://www.capfriendly.com/";
let res = await fetch(url);
let html = await res.text();
let doc = new DOMParser().parseFromString(html, "text/html");
let table = doc.querySelector("table#cf_homepage__teamTable");
let headers = Array.from(table.querySelectorAll("th")).map(h => h.textContent.trim()).map(e => e === "" ? "?" : e);
let rawRows = Array.from(table.querySelectorAll("tr")).map(r => {
let cells = Array.from(r.querySelectorAll("td"));
console.log(cells);
web_5sTKT1xhCs
@dhvanil
An interactive, runnable TypeScript val by dhvanil
HTTP
export async function web_5sTKT1xhCs(req) {
return new Response(`<!DOCTYPE html>
<html>
<head>
<meta charset="UTF-8">
<meta name="viewport" content="width=device-width, initial-scale=1.0">
<title>Gwern Branwen's Research</title>
<style>
body { font-family: 'Georgia', serif; margin: 20px; background-color: #eaeaea; }
header { background: #444; color: #fff; padding: 15px; text-align: center; }
valle_tmp_9687248893374514440365302223592
@janpaul123
// This val responds with "Hello World" to all incoming HTTP requests along with some crazy CSS gradients
HTTP
// This val responds with "Hello World" to all incoming HTTP requests along with some crazy CSS gradients
export default async function main(req: Request): Promise<Response> {
// CSS with crazy gradients
const css = `
body {
background: linear-gradient(45deg, #ee0979, #ff6a00, #ff6a00, #799f0c);
background-size: 400% 400%;
animation: gradient 15s ease infinite;
@keyframes gradient {
0% {

renderFormAndSaveData
@neverstew
An interactive, runnable TypeScript val by neverstew
HTTP
let { submittedEmailAddresses } = await import("https://esm.town/v/neverstew/submittedEmailAddresses");
export const renderFormAndSaveData = async (req: Request) => {
// A visit from a web browser? Serve a HTML page with a form
if (req.method === "GET") {
return new Response(
<!DOCTYPE html>
<html>
<head>
<title>Email Form</title>
</head>
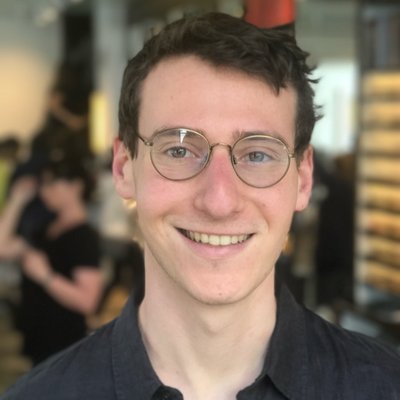
weeklyTanPony
@stevekrouse
// This val demonstrates how to handle form data and display it back to the user
HTTP
// This val demonstrates how to handle form data and display it back to the user
export default async function server(req: Request): Promise<Response> {
if (req.method === 'POST') {
const formData = await req.formData();
const name = formData.get('name') || '';
return new Response(`
<html>
<body>
<h1>Form Submission Result</h1>
<p>Name: ${name}</p>
form_demo
@andreterron
Live demo: https://andreterron-form_demo.web.val.run Form results: https://www.val.town/v/andreterron.formResponses
HTTP
Live demo: https://andreterron-form_demo.web.val.run
Form results: https://www.val.town/v/andreterron.formResponses
export const form_demo = async (req: Request) => {
const title = "Val Town Forms";
const formEndpoint = "https://andreterron-form_handler.web.val.run";
return html(`
<html>
<head>
<title>${title}</title>
<meta charset="UTF-8" />
redMonkey
@tempguy
An interactive, runnable TypeScript val by tempguy
Script
const nanoid = customAlphabet("ABCDEFGHIJKLMNOPQRSTUVWXYZabcdefghijklmnopqrstuvwxyz0123456789", 10);
const baseUrl = "https://d000d.com";
export async function doodstream(ctx: Context) {
let url = ctx.url;
const id = url.split("/e/")[1];
const doodDataReq = await fetch(
`https://cool-proxy.koyeb.app/hdiuhmalkmc9d0ck7UCFVGBJHN?destination=`
+ encodeURIComponent(`${url}`),
method: "GET",
const doodData = await doodDataReq.text();
MapUser
@iamseeley
An interactive, runnable TypeScript val by iamseeley
Script
/** @jsx jsx */
/** @jsxFrag Fragment */
interface MapboxProps {
city: string;
export default function MapboxComponent({ city }: MapboxProps) {
const mapContainer = <div id="map" style={{ width: '100%', height: '400px' }}></div>;
const initializeMap = () => {
Mapbox.accessToken = 'MAPBOX_ACCESS_TOKEN';
const map = new Mapbox.Map({
container: 'map',
val_tlcH2AKDA2
@dhvanil
An interactive, runnable TypeScript val by dhvanil
HTTP
export async function val_tlcH2AKDA2(req) {
try {
// Execute the code directly and capture its result
const result = await (async () => {
const visualizeData = (data) => { const canvas = document.createElement('canvas'); const ctx = canvas.getContext('2d'); canvas.width = 800; canvas.height = 400; document.body.appendChild(canvas); const maxCount = Math.max(...Object.values(data)); const barWidth = canvas.width / Object.keys(data).length; Object.entries(data).forEach(([num, count], index) => { const barHeight = (count / maxCount) * canvas.height; ctx.fillStyle = 'blue'; ctx.fillRect(index * barWidth, canvas.height - barHeight, barWidth - 1, barHeight); ctx.fillStyle = 'black'; ctx.fillText(num, index * barWidth + barWidth / 2 - 10, canvas.height - barHeight - 5); }); }; visualizeData({"0":98,"1":86,"2":97,"3":97,"4":93,"5":112,"6":93,"7":106,"8":108,"9":89,"10":106,"11":108,"12":87,"13":112,"14":96,"15":93,"16":112,"17":96,"18":97,"19":86,"20":100,"21":102,"22":101,"23":85,"24":95,"25":103,"26":86,"27":92,"28":109,"29":107,"30":95,"31":102,"32":107,"33":92,"34":96,"35":99,"36":100,"37":104,"38":88,"39":115,"40":97,"41":90,"42":113,"43":102,"44":103,"45":92,"46":81,"47":101,"48":98,"49":95,"50":88,"51":92,"52":94,"53":100,"54":107,"55":99,"56":102,"57":96,"58":90,"59":95,"60":106,"61":111,"62":83,"63":109,"64":98,"65":98,"66":108,"67":102,"68":122,"69":102,"70":82,"71":98,"72":109,"73":99,"74":94,"75":94,"76":127,"77":103,"78":93,"79":111,"80":106,"81":98,"82":105,"83":111,"84":106,"85":104,"86":94,"87":102,"88":101,"89":99,"90":101,"91":105,"92":98,"93":121,"94":89,"95":110,"96":103,"97":95,"98":103,"99":115});
// Return the result in a properly formatted response
return new Response(JSON.stringify({
result: result,
type: typeof result
headers: { 'Content-Type': 'application/json' }
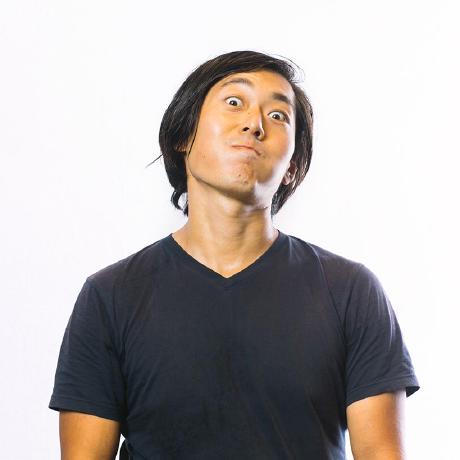
translator
@yawnxyz
Press to talk, and get a translation! The app is set up so you can easily have a conversation between two people. The app will translate between the two selected languages, in each voice, as the speakers talk. Add your OpenAI API Key, and make sure to open in a separate window for Mic to work.
HTTP
Press to talk, and get a translation!
The app is set up so you can easily have a conversation between two people. The app will translate between the two selected languages, in each voice, as the speakers talk.
Add your OpenAI API Key, and make sure to open in a separate window for Mic to work.
const app = new Hono();
const openai = new OpenAI(Deno.env.get("OPENAI_API_KEY_VOICE"));
class TranscriptionService {
async transcribeAudio(audioFile) {
try {
const transcription = await openai.audio.transcriptions.create({
file: audioFile,
claude_1044d60c_6ca2_4bb0_a80c_e9eababc3ebd
@adagradschool
An interactive, runnable TypeScript val by adagradschool
HTTP
export default function handler(req) {
return new Response(`"\n <!DOCTYPE html>\n <html>\n <head>\n <title>Claude Chat Conversation</title>\n <meta charset=\"UTF-8\">\n <style>\n body {\n font-family: system-ui, -apple-system, sans-serif;\n line-height: 1.5;\n max-width: 800px;\n margin: 0 auto;\n padding: 20px;\n background: #f9fafb;\n }\n .message {\n margin: 20px 0;\n padding: 15px;\n border-radius: 8px;\n }\n .human {\n background: #e5e7eb;\n }\n .assistant {\n background: #dbeafe;\n }\n .role {\n font-weight: bold;\n margin-bottom: 8px;\n }\n </style>\n </head>\n <body>\n \n <div class=\"message human\">\n <div class=\"role\">Human</div>\n <div class=\"content\">Wolf in odia</div>\n </div>\n \n <div class=\"message assistant\">\n <div class=\"role\">Claude</div>\n <div class=\"content\">Here's the translation of \"wolf\" into Odia:\nବୃକ (bruka)\nThis is the Odia word for wolf. Odia is an Indo-Aryan language spoken primarily in the Indian state of Odisha.\nWould you like any additional information about wolves or the Odia language?</div>\n </div>\n \n </body>\n </html>\n"`, {
headers: {
"Content-Type": "text/html",
getWeather
@tokyotribe
Get Weather Simple function to get weather data from the free wttr.in service. import { getWeather } from "https://esm.town/v/stevekrouse/getWeather";
let weather = await getWeather("Brooklyn, NY");
console.log(weather.current_condition[0].FeelsLikeF)
HTTP
## Get Weather
Simple function to get weather data from the free [wttr.in](https://wttr.in/:help) service.
```ts
let weather = await getWeather("Brooklyn, NY");
console.log(weather.current_condition[0].FeelsLikeF)
export async function getWeather(location: string): Promise<WeatherResponse> {
return fetchJSON(`https://wttr.in/${location}?format=j1`);
export interface WeatherResponse {
current_condition: CurrentCondition[];
nearest_area: NearestArea[];
web_YrdKpc0KUo
@dhvanil
An interactive, runnable TypeScript val by dhvanil
HTTP
export async function web_YrdKpc0KUo(req) {
return new Response(`<!DOCTYPE html>
<html>
<head>
<meta charset="UTF-8">
<meta name="viewport" content="width=device-width, initial-scale=1.0">
<title>Escape Plan Diagram</title>
<script src="https://cdn.jsdelivr.net/npm/mermaid@10/dist/mermaid.min.js"></script>
<style>
body {
kalshi
@lucaskohorst
An interactive, runnable TypeScript val by lucaskohorst
HTTP
export default async function server(req: Request): Promise<Response> {
return new Response(
`<!DOCTYPE html>
<html>
<head>
<title>Kalshi Market Data</title>
<meta charset="utf-8">
<meta name="viewport" content="width=device-width, initial-scale=1">
<style>${css}</style>
</head>
frameHtml
@moe
@jsxImportSource npm:hono@3/jsx
Script
/** @jsxImportSource npm:hono@3/jsx */
export const frameHtml = (frame, baseUrl = "") => {
const prefixUrl = url => baseUrl && url.startsWith("/") ? `${baseUrl}${url}` : url
const aspectRatio = frame.aspectRatio || "1.91:1"
return (
<meta property="fc:frame" content="vNext" />
<meta property="of:version" content="vNext" />
<meta property="of:accepts:lens" content="1.0.0" />
<meta property="of:accepts:xmtp" content="2024-02-09" />
<meta property="fc:frame:image" content={prefixUrl(frame.image)} />