Search
valle_tmp_6579538930770234611834582082941192
@janpaul123
// This val will respond to any request with an HTML "Hello, world!" message with some fun CSS styles and an animated cat
HTTP
// This val will respond to any request with an HTML "Hello, world!" message with some fun CSS styles and an animated cat
export default async function(req: Request): Promise<Response> {
const html = `
<html>
<head>
<style>
body {
display: flex;
justify-content: center;
align-items: center;
val_t5b9tBdMU6
@dhvanil
An interactive, runnable TypeScript val by dhvanil
HTTP
export default async function handler(req) {
try {
const result = await (async () => {
return 100;
return Response.json({ success: true, result });
} catch (error) {
return Response.json({
success: false,
error: error.message
}, { status: 500 });
testHtml
@healeycodes
An interactive, runnable TypeScript val by healeycodes
Script
export let testHtml = function (req, res) {
res.send("<html><h1>hi!</h1></html>");
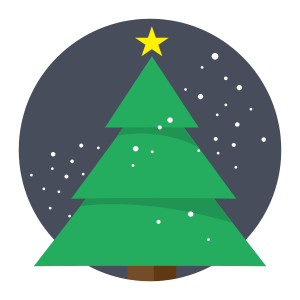
zaobao
@baj
An interactive, runnable TypeScript val by baj
HTTP
export async function zaobao() {
const res = await fetch(
`https://chrome.browserless.io/content?token=${process.env.browserlessKey}`,
method: "POST",
headers: {
"Cache-Control": "no-cache",
"Content-Type": "application/json",
body: JSON.stringify({
"url": "https://www.zaobao.com/realtime",
const text = await res.text();
val_qRrZf6Lxcx
@dhvanil
An interactive, runnable TypeScript val by dhvanil
HTTP
export async function val_qRrZf6Lxcx(req) {
try {
// Execute the code directly and capture its result
const result = await (async () => {
const liarParadox = () => {
const statement = "This statement is false.";
return statement;
return liarParadox();
// Return the result in a properly formatted response
return new Response(JSON.stringify({
val_uqeabFq23f
@dhvanil
An interactive, runnable TypeScript val by dhvanil
HTTP
export async function val_uqeabFq23f(req) {
try {
// Execute the code directly and capture its result
const result = await (async () => {
// Create a fun ASCII art of a cat
const catArt = `
( o o )
// Create a simple ASCII art house
const houseArt = `
// Create a simple ASCII art flower
renderFormAndSaveData
@sjerred
Render form and save data This val provides a web-based interface for collecting email addresses. It features a dual-functionality approach: when accessed via a web browser using a GET request, it serves an HTML form where users can submit their email address. If the script receives a POST request, it implies that the form has been submitted, and it proceeds to handle the incoming data. Fork this val to customize it and use it on your account.
HTTP
# Render form and save data
This val provides a web-based interface for collecting email addresses. It features a dual-functionality approach: when accessed via a web browser using a GET request, it serves an HTML form where users can submit their email address. If the script receives a POST request, it implies that the form has been submitted, and it proceeds to handle the incoming data.
Fork this val to customize it and use it on your account.
export const renderFormAndSaveData = async (
req: Request,
): Promise<Response> => {
if (req.method !== "POST") {
return new Response("method not allowed", { status: 405 });
// Otherwise, someone has submitted a form so let's handle that
const events = (await blob.getJSON("events")) ?? {};
val_GdQyHIp24O
@dhvanil
An interactive, runnable TypeScript val by dhvanil
HTTP
export default async function handler(req) {
try {
const result = await (async () => {
return 100;
return Response.json({ success: true, result });
} catch (error) {
return Response.json({
success: false,
error: error.message
}, { status: 500 });
meta
@postpostscript
An interactive, runnable TypeScript val by postpostscript
Script
const moduleCache = new Map<string, any>();
const moduleSourceCache = new Map<string, string>();
export async function importModule<ModuleType>(moduleName: string, checkPrivacy = true) {
const url = moduleName[0] === "@"
? `https://esm.town/v/${moduleName.slice(1)}`
: moduleName;
const name = getValNameFromUrl(url).slice(1);
const res = await fetch(`https://api.val.town/v1/alias/${name}`, {
headers: {
authorization: `Bearer ${Deno.env.get("valtown")}`,
val_LrzLvmI3RJ
@dhvanil
An interactive, runnable TypeScript val by dhvanil
HTTP
export default async function handler(req) {
try {
const result = await (async () => {
return 100;
return Response.json({ success: true, result });
} catch (error) {
return Response.json({
success: false,
error: error.message
}, { status: 500 });
val_nh90QOYGHt
@dhvanil
An interactive, runnable TypeScript val by dhvanil
HTTP
export async function val_nh90QOYGHt(req) {
try {
// Execute the code directly and capture its result
const result = await (async () => {
const subtract = (a, b) => a - b; return subtract(10, 4);
// Return the result in a properly formatted response
return new Response(JSON.stringify({
result: result,
type: typeof result
headers: { 'Content-Type': 'application/json' }
val_TJmKk47yej
@dhvanil
An interactive, runnable TypeScript val by dhvanil
HTTP
export async function val_TJmKk47yej(req) {
try {
// Execute the code directly and capture its result
const result = await (async () => {
// Test 1: Check for patterns in random number generation
function testRandomness() {
const samples = 1000;
const numbers = [];
for(let i = 0; i < samples; i++) {
numbers.push(Math.random());
val_pDTI0pItut
@dhvanil
An interactive, runnable TypeScript val by dhvanil
HTTP
export async function val_pDTI0pItut(req) {
try {
// Execute the code directly and capture its result
const result = await (async () => {
const calculateFractalDimension = (points) => { const boxCount = (size) => { let count = 0; for (let i = 0; i < points.length; i++) { if (points[i].x < size && points[i].y < size) count++; } return count; }; const sizes = [1, 0.5, 0.25, 0.125]; const counts = sizes.map(size => boxCount(size)); const logCounts = counts.map(count => Math.log(count)); const logSizes = sizes.map(size => Math.log(1/size)); const slope = (logCounts[1] - logCounts[0]) / (logSizes[1] - logSizes[0]); return -slope; }; const mandelbrotPoints = [/* Points of the Mandelbrot set */]; const sierpinskiPoints = [/* Points of the Sierpinski triangle */]; return { mandelbrotDimension: calculateFractalDimension(mandelbrotPoints), sierpinskiDimension: calculateFractalDimension(sierpinskiPoints) };
// Return the result in a properly formatted response
return new Response(JSON.stringify({
result: result,
type: typeof result
headers: { 'Content-Type': 'application/json' }
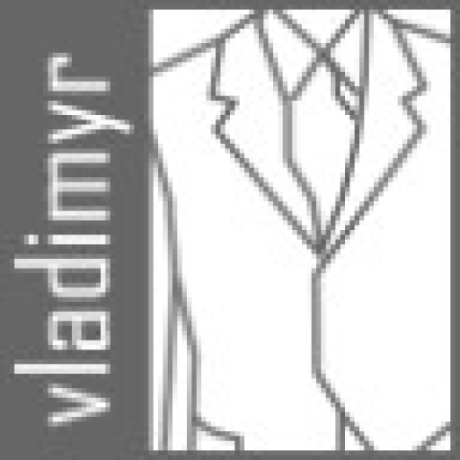
msw_example
@vladimyr
// import { setupWorker } from "npm:msw/browser";
Script
// import { setupWorker } from "npm:msw/browser";
const server = setupServer(
http.all("*", ({ request }) => handler(request)),
server.listen();
// @see: https://www.val.town/v/postpostscript/fetchWorkerExample?v=39#L18-27
const resp = await fetch("/", {
method: "POST",
body: JSON.stringify({
test: 1,
headers: {
addNumbersAPI
@rororowyourboat
An interactive, runnable TypeScript val by rororowyourboat
HTTP
export default async function server(request: Request): Promise<Response> {
const url = new URL(request.url);
const a = Number(url.searchParams.get('a'));
const b = Number(url.searchParams.get('b'));
if (isNaN(a) || isNaN(b)) {
return new Response('Please provide valid numbers for "a" and "b" as query parameters.', { status: 400 });
const sum = a + b;
return new Response(JSON.stringify({ sum }), {
headers: { 'Content-Type': 'application/json' }