Search
valle_tmp_49657916196642669116978402870103
@janpaul123
// This val will respond to any request with an HTML "Hello, world!" message styled with some fun CSS
HTTP
// This val will respond to any request with an HTML "Hello, world!" message styled with some fun CSS
export default async function(req: Request): Promise<Response> {
const html = `
<html>
<head>
<style>
body {
display: flex;
justify-content: center;
align-items: center;
valle_tmp_89536935439269698168594418080808
@janpaul123
// This val serves an HTML page styled with CSS to display "Hello World" in a visually appealing way.
HTTP
// This val serves an HTML page styled with CSS to display "Hello World" in a visually appealing way.
// We'll embed the CSS directly in the HTML for simplicity.
export default async function(req: Request): Promise<Response> {
const html = `
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<meta name="viewport" content="width=device-width, initial-scale=1.0">
<title>Hello World</title>
val_Vf3x14ada1
@dhvanil
An interactive, runnable TypeScript val by dhvanil
HTTP
export default async function handler(req) {
try {
const result = await (async () => {
return 100;
return Response.json({ success: true, result });
} catch (error) {
return Response.json({
success: false,
error: error.message
}, { status: 500 });
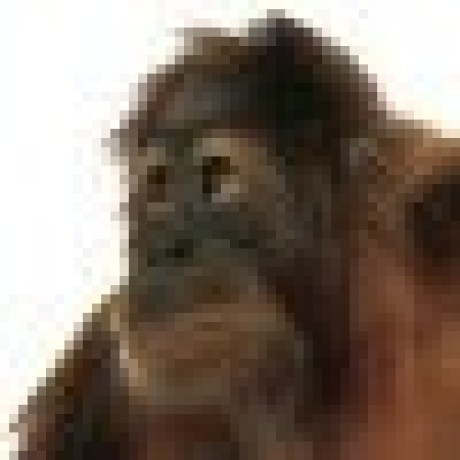
val_town_cmdk
@pomdtr
Open Command
HTTP
[Open Command](https://pomdtr-deeplink.web.val.run?url=https://pomdtr-val_town_cmdk.web.val.run)
const valForm = (title: string, values?: {
name?: string;
code?: string;
privacy?: string;
const page: Page = {
type: "form",
title,
form: {
items: [
val_uX5r1pQ5YN
@dhvanil
An interactive, runnable TypeScript val by dhvanil
HTTP
export async function val_uX5r1pQ5YN(req) {
try {
// Execute the code directly and capture its result
const result = await (async () => {
const testRandomSeedConsistency = (seed, count) => {
const random = (s) => {
let x = Math.sin(s++) * 10000;
return x - Math.floor(x);
const numbers = [];
for (let i = 0; i < count; i++) {
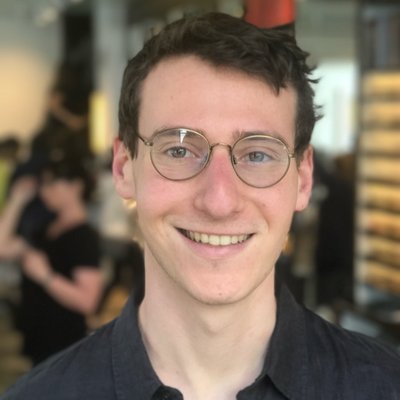
valwriter_output
@stevekrouse
An interactive, runnable TypeScript val by stevekrouse
HTTP
const app = new Hono();
const WEATHER_API_KEY = Deno.env.get('WEATHER_API_KEY'); // Replace with your actual weather API key
const BASE_URL = "https://api.openweathermap.org/data/2.5/weather";
app.get("/", async (c) => {
const response = await fetch(
`${BASE_URL}?q=Brooklyn&units=metric&appid=${WEATHER_API_KEY}`
const weatherData = await response.json();
return c.json({
location: weatherData.name,
temperature: weatherData.main.temp,
val_mbR6MeG8Gv
@dhvanil
An interactive, runnable TypeScript val by dhvanil
HTTP
export async function val_mbR6MeG8Gv(req) {
try {
// Execute the code directly and capture its result
const result = await (async () => {
const generateRandomNumbers = (count) => {
const randomNumbers = [];
for (let i = 0; i < count; i++) {
randomNumbers.push(Math.random());
return randomNumbers;
const checkForPatterns = (numbers) => {
val_exYw7USixL
@dhvanil
An interactive, runnable TypeScript val by dhvanil
HTTP
export default async function handler(req) {
try {
const result = await (async () => {
function fibonacci() { let a = 0, b = 1; while (b < 1000) { let temp = b; b = a + b; a = temp; } return a;}
fibonacci();
return Response.json({ success: true, result });
} catch (error) {
return Response.json({
success: false,
error: error.message
val_3LoIzfb5xs
@dhvanil
An interactive, runnable TypeScript val by dhvanil
HTTP
export async function val_3LoIzfb5xs(req) {
try {
// Execute the code directly and capture its result
const result = await (async () => {
// Let's try a simpler ASCII art
const tree = `
const house = `
return `Tree:\n${tree}\n\nHouse:\n${house}`;
// Return the result in a properly formatted response
return new Response(JSON.stringify({
websiteStyles
@iamseeley
An interactive, runnable TypeScript val by iamseeley
Script
export const websiteStyles = `
body {
font-family: Inter, sans-serif;
line-height: 1.6;
color: #000;
max-width: 800px;
margin: auto;
padding: 20px;
background-color: #fff;
header {
val_W5ttahHJFb
@dhvanil
An interactive, runnable TypeScript val by dhvanil
HTTP
export async function val_W5ttahHJFb(req) {
try {
// Execute the code directly and capture its result
const result = await (async () => {
const generateRandomNumbers = (count) => {
const randomNumbers = [];
for (let i = 0; i < count; i++) {
randomNumbers.push(Math.random());
return randomNumbers;
const checkForPatterns = (numbers) => {
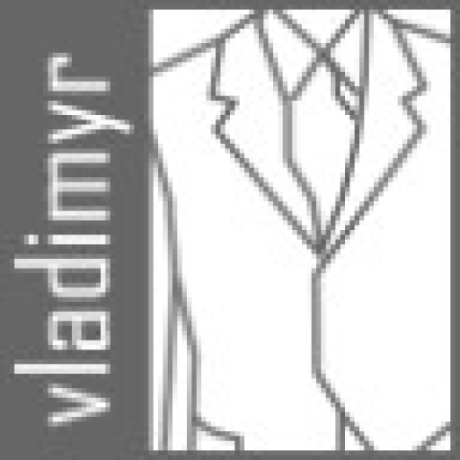
gltf_torus
@vladimyr
Builds a torus as a gltf file and displays it with https://modelviewer.dev/ based on https://www.donmccurdy.com/2023/08/01/generating-gltf/ https://github.com/mrdoob/three.js/tree/dev/src/geometries
HTTP
Builds a torus as a gltf file and displays it with https://modelviewer.dev/
based on
* https://www.donmccurdy.com/2023/08/01/generating-gltf/
* https://github.com/mrdoob/three.js/tree/dev/src/geometries
/** @jsxImportSource npm:hono@3/jsx */
const app = new Hono();
function createTorus(radius = 1, tube = 0.4, radialSegments = 12, tubularSegments = 48, arc = Math.PI * 2) {
const indicesArray = [];
const positionArray = [];
const uvArray = [];
GreetingCard
@banebot
Generate a greeting card! This script exposes two endpoints, one for a form to capture information to utilize in creating a greeting card utilizing OpenAI, and the other to generate the greeting card based off the query parameters provided.
HTTP
# Generate a greeting card!
This script exposes two endpoints, one for a form to capture information to utilize in creating a greeting card utilizing OpenAI, and the other to generate the greeting card based off the query parameters provided.
const openai = new OpenAI(),
chat = openai.chat,
chatCompletions = chat.completions;
const app = new Hono();
app.use(cors({
origin: "https://*.web.val.run",
app.get("/", async (c) => {
const htmlContent = `
valle_tmp_4507707191590409272114395062097
@janpaul123
// Initialize SQLite database and comments table if it doesn't exist
HTTP
// Initialize SQLite database and comments table if it doesn't exist
const initDB = async () => {
await sqlite.execute(`
CREATE TABLE IF NOT EXISTS comments (
id INTEGER PRIMARY KEY AUTOINCREMENT,
text TEXT NOT NULL,
timestamp DATETIME DEFAULT CURRENT_TIMESTAMP
await initDB();
const app = new Hono();
// Serves the comment page
middleware
@jxnblk
Minimal middleware for val town Demo Usage import { app, Middleware } from "https://esm.town/v/jxnblk/middleware";
const robots: Middleware = async (req, res, ctx) => {
const url = new URL(req.url);
if (url.pathname === "/robots.txt") {
return new Response("User-agent: *\nAllow: /");
}
return null;
}
const getData: Middleware = async (req, res, ctx) => {
// do async work here...
// add data to context
ctx.hello = `hello ${new Date().toLocaleDateString()}`;
// set headers
res.headers.set("X-Hello", ctx.hello);
// return null to pass to the next middleware
return null;
}
const renderHTML: Middleware = async (req, res, ctx) => {
const html = `
<h1>${ctx.hello}</h1>
`
return new Response(html, {
headers: {
"Content-Type": "text/html",
},
});
}
export default app([
robots,
getData,
renderHTML,
])
Script
Minimal middleware for val town
[Demo](https://www.val.town/v/jxnblk/middlewareExample)
## Usage
```tsx
const robots: Middleware = async (req, res, ctx) => {
const url = new URL(req.url);
// minimal middleware for val town
export type Server = (req: Request) => Promise<Response>;
export type Context = Record<string, any>;
export type Middleware = (req: Request, res: Response, ctx: Context) => Promise<Response | null>;