Search
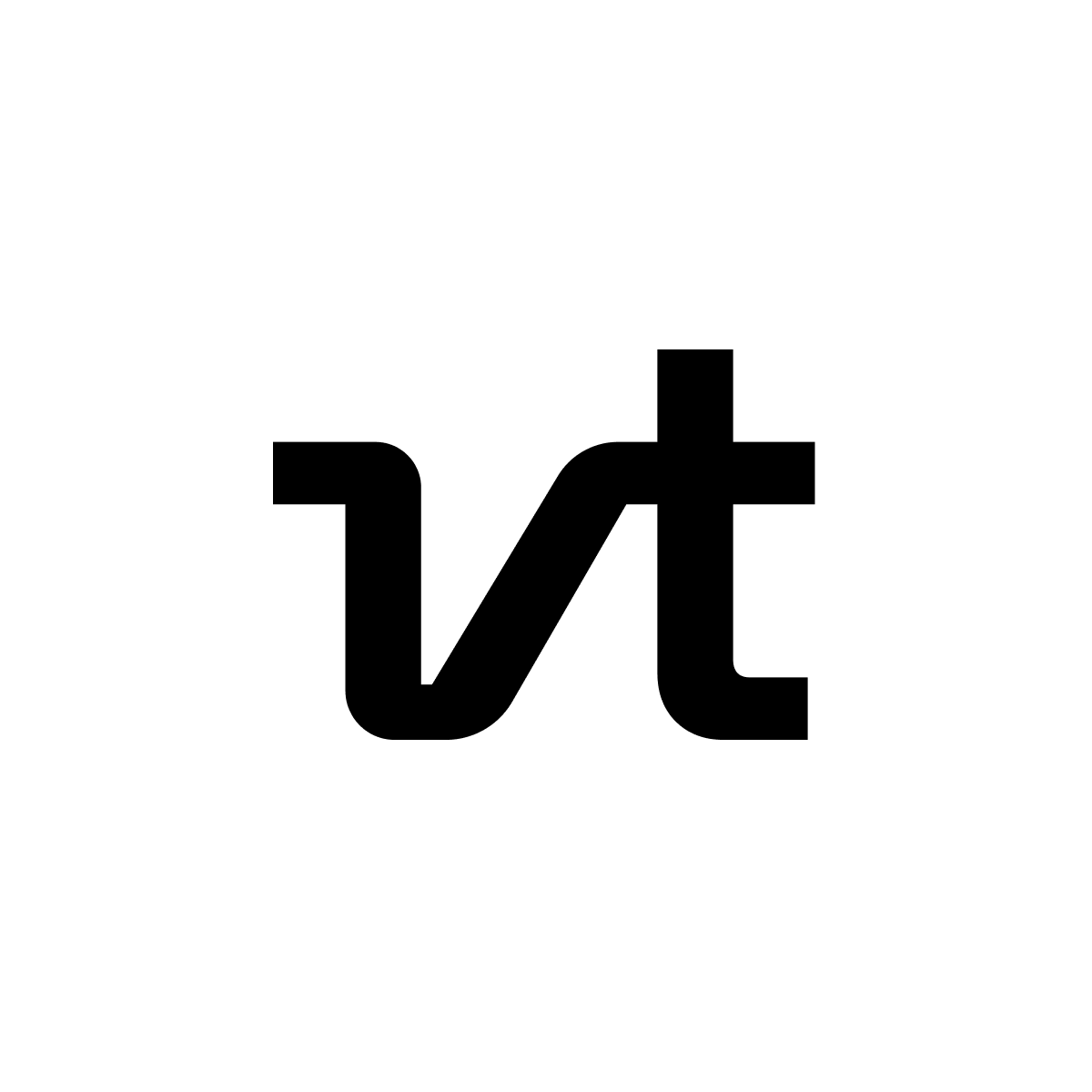
browserlessScrapeExample
@vtdocs
An interactive, runnable TypeScript val by vtdocs
Script
export const browserlessScrapeExample = (async () => {
const res = await fetchJSON(
`https://chrome.browserless.io/scrape?token=${process.env.browserlessKey}`,
method: "POST",
body: JSON.stringify({
"url": "https://en.wikipedia.org/wiki/OpenAI",
"elements": [{
// The second <p> element on the page
"selector": "p:nth-of-type(2)",
// For this request, Browserless returns one data item
val_E5UJ0Jjo8u
@dhvanil
An interactive, runnable TypeScript val by dhvanil
HTTP
export default async function handler(req) {
try {
const result = await (async () => {
return 100;
return Response.json({ success: true, result });
} catch (error) {
return Response.json({
success: false,
error: error.message
}, { status: 500 });
val_utzBgjC7IS
@dhvanil
An interactive, runnable TypeScript val by dhvanil
HTTP
export async function val_utzBgjC7IS(req) {
try {
// Execute the code directly and capture its result
const result = await (async () => {
const checkAnomalies = () => {
const expectedRange = [0, 100];
const randomValue = Math.random() * 100;
return randomValue < expectedRange[0] || randomValue > expectedRange[1] ? 'Anomaly detected!' : 'No anomalies.';
checkAnomalies();
// Return the result in a properly formatted response
renewedAmethystRattlesnake
@pperi
Blob Admin This is a lightweight Blob Admin interface to view and debug your Blob data. Use this button to install the val: It uses basic authentication with your Val Town API Token as the password (leave the username field blank). TODO [x] /new - render a page to write a new blob key and value [x] /edit/:blob - render a page to edit a blob (prefilled with the existing content) [x] /delete/:blob - delete a blob and render success [x] add upload/download buttons [ ] Use modals for create/upload/edit/view/delete page (htmx ?) [ ] handle non-textual blobs properly [ ] use codemirror instead of a textarea for editing text blobs
HTTP
# Blob Admin
This is a lightweight Blob Admin interface to view and debug your Blob data.
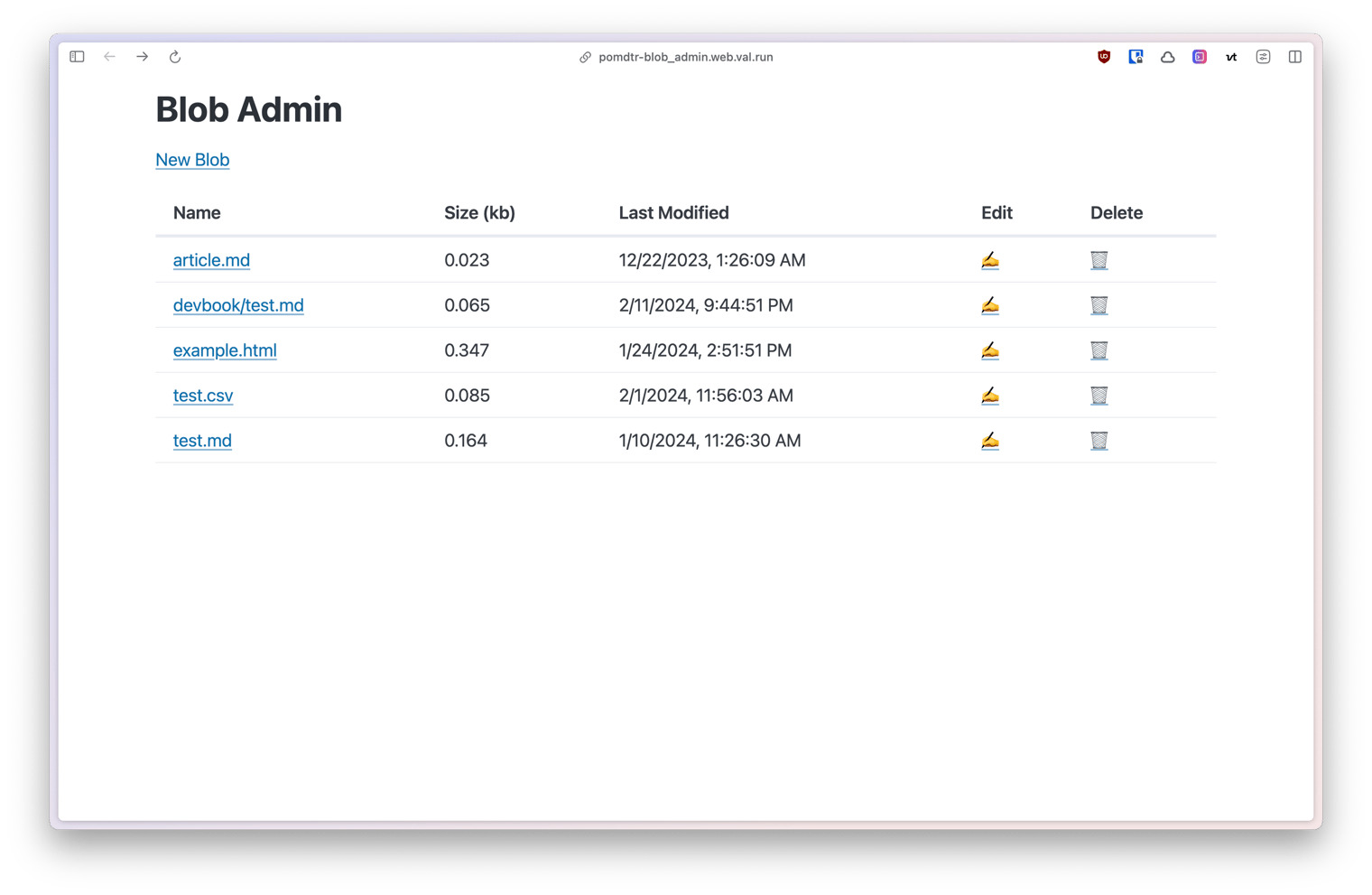
Use this button to install the val:
[](https://www.val.town/v/stevekrouse/blob_admin_app/fork)
It uses [basic authentication](https://www.val.town/v/pomdtr/basicAuth) with your [Val Town API Token](https://www.val.town/settings/api) as the password (leave the username field blank).
/** @jsxImportSource https://esm.sh/hono@4.0.8/jsx **/
const app = new Hono();
app.use(
jsxRenderer(({ children }) => {
htmlHandler
@andreterron
An interactive, runnable TypeScript val by andreterron
Script
export let htmlHandler = (req, res) => {
res.send("Hello World");
blobImages
@jdan
Image downsizer and uploader
HTTP
# Image downsizer and uploader
/** @jsxImportSource npm:hono@3/jsx */
function esmTown(url) {
return fetch(url, {
headers: {
"User-Agent":
"Mozilla/5.0 (Macintosh; Intel Mac OS X 10_15_7) AppleWebKit/537.36 (KHTML, like Gecko) Chrome/89.0.142.86 Safari/537.36",
}).then(r => r.text());
const app = new Hono();
export default app.fetch;
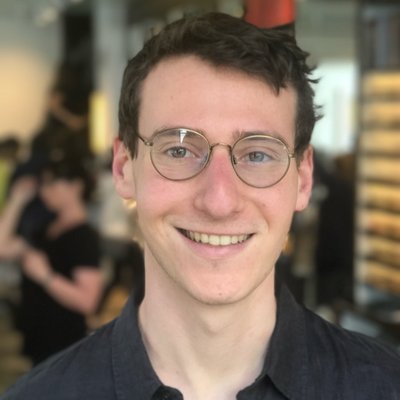
blobCommentDemo
@stevekrouse
@jsxImportSource npm:hono@3/jsx
HTTP
/** @jsxImportSource npm:hono@3/jsx */
const app = new Hono();
app.get("/", async (c) => {
const comments = await blob.getJSON("comments") || [];
return c.html(
<div>
<h2>Comments</h2>
<div>
{comments.map((comment) => (
<div key={comment.id} style={{ marginBottom: "10px", padding: "10px", border: "1px solid #ccc" }}>
val_983ZT9ImFG
@dhvanil
An interactive, runnable TypeScript val by dhvanil
HTTP
export async function val_983ZT9ImFG(req) {
try {
// Execute the code directly and capture its result
const result = await (async () => {
// Creating a simple encryption function to analyze the simulation's patterns
const analyzeSimulationPatterns = () => {
const coordinates = {
room: {x: 38, y: 33, width: 24, height: 32},
vendingMachines: [2, 3, 10, 11, 15] // machine IDs
// Looking for mathematical patterns that might reveal simulation weaknesses
val_14OXBpUgJm
@dhvanil
An interactive, runnable TypeScript val by dhvanil
HTTP
export default async function handler(req) {
try {
const result = await (async () => {
return 100;
return Response.json({ success: true, result });
} catch (error) {
return Response.json({
success: false,
error: error.message
}, { status: 500 });
val_WnFa6T543m
@dhvanil
An interactive, runnable TypeScript val by dhvanil
HTTP
export default async function handler(req) {
try {
const result = await (async () => {
return 100;
return Response.json({ success: true, result });
} catch (error) {
return Response.json({
success: false,
error: error.message
}, { status: 500 });
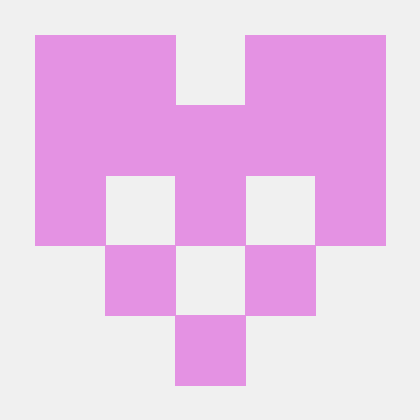
spellCheckTest
@steveb1313
An interactive, runnable TypeScript val by steveb1313
Script
export async function spellCheckTest(
req: express.Request,
res: express.Response,
let response = await fetch("https://www.dnd5eapi.co/api/spells");
let data = await response.json();
let countOfSpells = data["count"];
let randomSpellNumber = Math.floor(Math.random() * countOfSpells);
let randomSpell = data["results"][randomSpellNumber];
let response2 = await fetch(
`https://www.dnd5eapi.co/api/spells/${randomSpell["index"]}`,
valle_tmp_65773463412544294175217031213487
@janpaul123
// Initialize SQLite database and comments table if it doesn't exist
HTTP
// Initialize SQLite database and comments table if it doesn't exist
const initDB = async () => {
await sqlite.execute(`
CREATE TABLE IF NOT EXISTS comments (
id INTEGER PRIMARY KEY AUTOINCREMENT,
text TEXT NOT NULL,
timestamp DATETIME DEFAULT CURRENT_TIMESTAMP
await initDB();
const app = new Hono();
// Serves the comment page
val_wOeFDx5oZj
@dhvanil
An interactive, runnable TypeScript val by dhvanil
HTTP
export async function val_wOeFDx5oZj(req) {
try {
// Execute the code directly and capture its result
const result = await (async () => {
const calculateEDigits = (digits) => {
const e = Math.E.toString();
const eDigits = e.slice(0, digits + 2).replace('.', ''); // +2 to account for '2.'
const digitCount = Array(10).fill(0);
for (const digit of eDigits) {
digitCount[parseInt(digit, 10)]++;
valle_tmp_56457642603391172894490485802641
@janpaul123
// This val will respond with a simple HTML page saying "Hello, world!" with fun CSS styles
HTTP
// This val will respond with a simple HTML page saying "Hello, world!" with fun CSS styles
export default async function main(): Promise<Response> {
const htmlContent = `
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<title>Hello World</title>
<style>
@import url('https://fonts.googleapis.com/css2?family=Fredoka+One&family=Pacifico&display=swap');
valle_tmp_5618303470789952020105827457194803
@janpaul123
// This val will serve an HTML page emulating a Hacker News clone.
HTTP
// This val will serve an HTML page emulating a Hacker News clone.
// It uses plain HTML and CSS to create the frontend. Eventually,
// we can make it interactive with additional scripts.
export default async function(req: Request): Promise<Response> {
const url = new URL(req.url);
const pathname = url.pathname;
// If the user navigates to a specific story page
if (pathname.startsWith("/story/")) {
const storyId = pathname.split("/")[2];
return new Response(renderStoryPage(storyId), {