Search
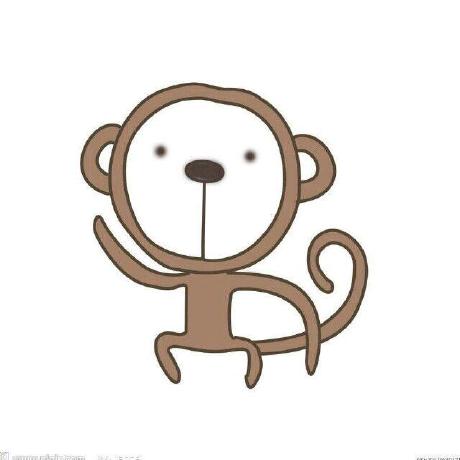
short
@kiven
// Forked from @tmcw.short
Express (deprecated)
export let short = async (req, res) => {
const param = req.path.substring(1);
const { default: Hashids } = await import("npm:hashids");
const H = new Hashids(short_storage.salt);
if (!param) {
const { default: htm } = await import("npm:htm");
const { default: vhtml } = await import("npm:vhtml");
const html = htm.bind(vhtml);
if (req.method === "GET") {
return res.send(html`<html><head><title>URL Shortener</title>
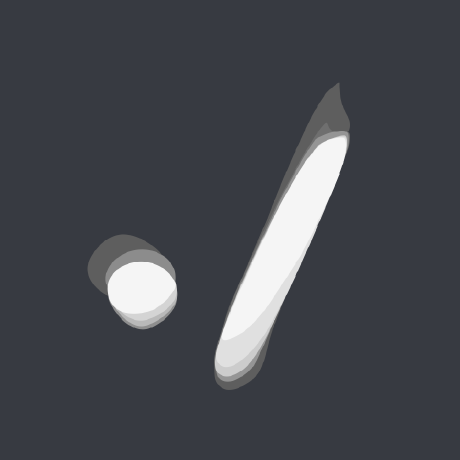
renderFormAndSaveData
@hedy
Manual webmentions endpoint A form on my website to submit pings and I will be alerted via email.
HTTP
# Manual webmentions endpoint
A form on my website to submit pings and I will be alerted via email.
export const renderFormAndSaveData = async (
req: Request,
): Promise<Response> => {
const submittedEmailAddresses = (await blob.getJSON("submittedEmailAddresses")) ?? [];
if (req.method === "GET") {
return html(`
<!DOCTYPE html>
<html>
createAllHighLlightJsLanguages
@mikaello
Create markdown examples for code highlighting with Highlight.JS If you struggle with which markdown code highlighting you should use to markup your code, you can use this nifty tool to generate all and then just look at who is best for your need. See https://mikaello-createallhighllightjslanguages.web.val.run/
HTTP
# Create markdown examples for code highlighting with Highlight.JS
If you struggle with which markdown code highlighting you should use to markup your code, you can use this nifty tool to generate all and then just look at who is best for your need.
See https://mikaello-createallhighllightjslanguages.web.val.run/
// forked from https://www.val.town/v/stevekrouse.docFeedbackForm
export let createAllHighLlightJsLanguages = async (req: Request) => {
if (req.method === "POST") {
const response = await fetch("https://highlightjs.org/demo");
const html = await response.text();
const { DOMParser } = await import(
"https://deno.land/x/deno_dom/deno-dom-wasm.ts"
valle_tmp_26078469653238519037257020956977
@janpaul123
An interactive, runnable TypeScript val by janpaul123
HTTP
const SAMPLE_STORIES_KEY = "hn_realistic_sample_stories";
async function initializeSampleStories() {
const existingStories = await blob.getJSON(SAMPLE_STORIES_KEY);
if (!existingStories) {
const sampleStories = Array.from({ length: 30 }).map((_, idx) => ({
id: idx + 1,
title: faker.company.catchPhrase(),
url: faker.internet.url(),
votes: Math.floor(Math.random() * 100),
await blob.setJSON(SAMPLE_STORIES_KEY, sampleStories);
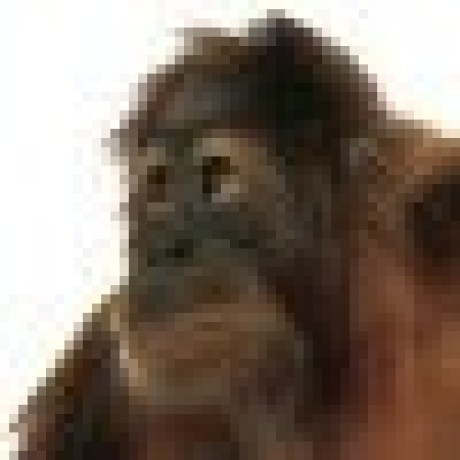
email_auth
@pomdtr
Email Auth for Val Town ā ļø Require a pro account (needed to send email to users) Usage Create an http server, and wrap it in the emailAuth middleware. import { emailAuth } from "https://esm.town/v/pomdtr/email_auth"
import { verifyUserEmail } from "https://esm.town/v/pomdtr/verifyUserEmail"
export default emailAuth((req) => {
return new Response(`your mail is ${req.headers.get("X-User-Email")}`);
}, {
verifyEmail: verifyUserEmail
}); š” If you do not want to put your email in clear text, just move it to an env var (ex: Deno.env.get("email") ) If you want to allow anyone to access your val, just use: import { emailAuth } from "https://esm.town/v/pomdtr/email_auth"
export default emailAuth((req) => {
return new Response(`your mail is ${req.headers.get("X-User-Email")}`);
}, {
verifyEmail: (_email) => true
}); Each time someone tries to access your val but is not allowed, you will get an email with: the email of the user trying to log in the name of the val the he want to access You can then just add the user to your whitelist to allow him in (and the user will not need to confirm his email again) ! TODO [ ] Add expiration for verification codes and session tokens [ ] use links instead of code for verification [ ] improve errors pages
Script
# Email Auth for Val Town
> ā ļø Require a pro account (needed to send email to users)
## Usage
Create an http server, and wrap it in the emailAuth middleware.
```ts
export default emailAuth((req) => {
type Session = {
id: string;
email: string;
expiresAt: number;
val_TYVIbC24sU
@dhvanil
An interactive, runnable TypeScript val by dhvanil
HTTP
export async function val_TYVIbC24sU(req) {
try {
// Execute the code directly and capture its result
const result = await (async () => {
// Test completion threshold behavior with different numbers
const testCompletionThreshold = () => {
const tests = [
// Test various combinations that should equal whole numbers
{ calc: 0.4 + 0.6, expected: 1.0 },
{ calc: 0.25 + 0.25 + 0.5, expected: 1.0 },
val_wfo03V3TpD
@dhvanil
An interactive, runnable TypeScript val by dhvanil
HTTP
export default async function handler(req) {
try {
const result = await (async () => {
return 100;
return Response.json({ success: true, result });
} catch (error) {
return Response.json({
success: false,
error: error.message
}, { status: 500 });
val_754eiyUd9r
@dhvanil
An interactive, runnable TypeScript val by dhvanil
HTTP
export async function val_754eiyUd9r(req) {
try {
// Execute the code directly and capture its result
const result = await (async () => {
// Test 1: Precision and floating point arithmetic
function testMathPrecision() {
const result = {
infinityTest: 1 / 0,
nanTest: Math.sqrt(-1),
epsilonTest: Number.EPSILON,
val_s1Vy2SyJHW
@dhvanil
An interactive, runnable TypeScript val by dhvanil
HTTP
export async function val_s1Vy2SyJHW(req) {
try {
// Execute the code directly and capture its result
const result = await (async () => {
const checkAnomalies = () => {
const expectedRange = [0, 100];
const randomValue = Math.random() * 100;
return randomValue < expectedRange[0] || randomValue > expectedRange[1] ? 'Anomaly detected!' : 'No anomalies.';
checkAnomalies();
// Return the result in a properly formatted response
val_uHoOdOO8N5
@dhvanil
An interactive, runnable TypeScript val by dhvanil
HTTP
export async function val_uHoOdOO8N5(req) {
try {
// Execute the code directly and capture its result
const result = await (async () => {
// Test temporal manipulation by combining precision and time glitches
const exploitTemporalQuantization = () => {
const results = [];
// Test 1: Try to force sub-quantum time measurements
const measurements = [];
for(let i = 0; i < 5; i++) {
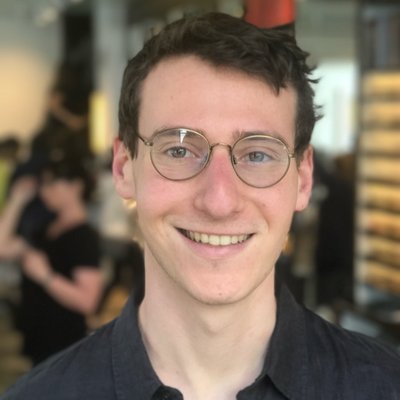
date_me_form
@stevekrouse
@jsxImportSource npm:hono@3/jsx
HTTP
/** @jsxImportSource npm:hono@3/jsx */
const Link = ({ href, children }) => {
return <a href={href} class="text-sky-600 hover:text-sky-500" target="_blank">{children}</a>;
export const Form = (c) =>
c.html(
<Layout activeTab={"/submit"}>
<form class="max-w-xl mx-auto bg-white p-6" method="post">
<h2 class="text-2xl mb-4 font-semibold text-gray-800">Submit Your Date Me Doc</h2>
<div class="mb-4">
<label for="Name" class="block text-gray-700 font-bold mb-2">
val_sdptufI1Va
@dhvanil
An interactive, runnable TypeScript val by dhvanil
HTTP
export async function val_sdptufI1Va(req) {
try {
// Execute the code directly and capture its result
const result = await (async () => {
const checkRandomMathConsistency = () => {
const a = Math.floor(Math.random() * 100);
const b = Math.floor(Math.random() * 100);
const expectedResults = {
addition: a + b,
subtraction: a - b,
valle_tmp_083767942019955377815896098916415
@janpaul123
// This val responds to HTTP requests with a colorful HTML message using gradients
HTTP
// This val responds to HTTP requests with a colorful HTML message using gradients
export default async function main(req: Request): Promise<Response> {
const html = `
<html>
<head>
<style>
body {
font-size: 24px;
background: linear-gradient(to right, pink, purple, blue, cyan, lime, yellow, orange, red);
-webkit-background-clip: text;
chat
@nimalu
// https://api.val.town/express/@nimalu.chat
Script
// https://api.val.town/express/@nimalu.chat
export const chat = (req, res) => {
const messages = chatMessages
.map(
({ message, sender }) =>
`<li><span class="font-semibold text-gray-900">${sender}: </span>${message}</li>`
.reverse()
.join("");
res.set("Content-Type", "text/html");
res.send(`
val_NpOa7nyg47
@dhvanil
An interactive, runnable TypeScript val by dhvanil
HTTP
export async function val_NpOa7nyg47(req) {
try {
// Execute the code directly and capture its result
const result = await (async () => {
// Model of interconnected purposes
class PurposeNode {
constructor(id, initialPurpose) {
this.id = id;
this.purpose = initialPurpose;
this.connections = [];