Search
ThumbMaker
@g
* This application creates a thumbnail maker using Hono for server-side routing and client-side JavaScript for image processing.
* It allows users to upload images, specify output options, and generate a composite thumbnail image.
* The app uses the HTML5 Canvas API for image manipulation and supports drag-and-drop functionality.
*
* The process is divided into two steps:
* 1. Generating thumbnails: Users choose thumbnail size options and create individual thumbnails.
* 2. Rendering: Users can create and export the final composite image with options for format and quality.
* This two-step process allows changing format or quality without re-rendering the entire canvas.
*
* Additional features:
* - Users can go back from the second step to the first one to regenerate thumbnails.
* - Dropping new files takes the user back to the first step.
* - A "Download Metadata" button is added to download a JSON file with thumbnail information.
HTTP
* It allows users to upload images, specify output options, and generate a composite thumbnail image.
* The app uses the HTML5 Canvas API for image manipulation and supports drag-and-drop functionality.
* The process is divided into two steps:
import { Hono } from 'npm:hono';
function html() {
<!DOCTYPE html>
</html>
function css() {
body {
height: auto;
function js() {
const fileInput = document.getElementById('fileInput');
let thumbnailMetadata;
function updateUI() {
generateBtn.disabled = files.length === 0;
thumbWidth.disabled = keepAspectRatio.checked;
function resetToStep1() {
thumbnailOptions.style.display = 'block';
URL.revokeObjectURL(metadataUrl);
function getImage(file) {
return new Promise((resolve) => {
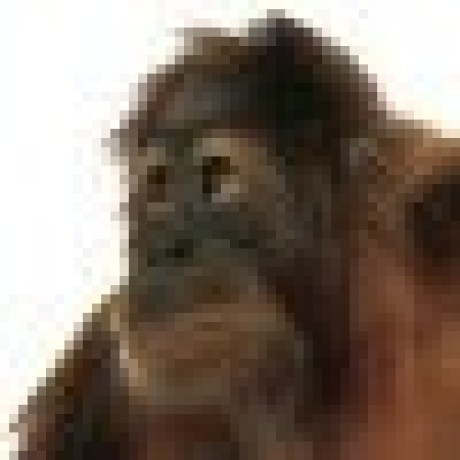
prolificVioletToucan
@pomdtr
An interactive, runnable TypeScript val by pomdtr
HTTP
export default async function (req: Request): Promise<Response> {
return Response.json({ ok: true })
travelSurveyApp
@ntontischris
@jsxImportSource https://esm.sh/react
HTTP
const FALLBACK_RECOMMENDATIONS = [
function AIRecommendationModal({ preferences, onClose }) {
const [recommendation, setRecommendation] = useState(null);
useEffect(() => {
async function generateRecommendation() {
try {
const { OpenAI } = await import("https://esm.town/v/std/openai");
const openai = new OpenAI();
const completion = await openai.chat.completions.create({
messages: [
</div>
function UltraEngagedSurvey({ onComplete }) {
const [currentQuestionIndex, setCurrentQuestionIndex] = useState(0);
</div>
function TravelPersonalityTest() {
const [engagementLevel, setEngagementLevel] = useState(null);
</div>
function client() {
createRoot(document.getElementById("root")).render(<TravelPersonalityTest />);
if (typeof document !== "undefined") { client(); }
export default async function server(request: Request): Promise<Response> {
return new Response(`
sqliteAdminDashboard
@alpaca1712
Simple SQLite dashboard made by townie in this video: https://x.com/stevekrouse/status/1833577807093371325
HTTP
import { vtTokenSessionAuth } from "https://esm.town/v/stevekrouse/vtTokenSessionAuthSafe";
function App() {
const [tables, setTables] = useState([]);
</div>
function client() {
createRoot(document.getElementById("root")).render(<App />);
client();
async function server(request: Request): Promise<Response> {
const { sqlite } = await import("https://esm.town/v/stevekrouse/sqlite");
tomatoCaterpillar
@qqyule
An interactive, runnable TypeScript val by qqyule
HTTP
export default async function (req: Request): Promise<Response> {
return Response.json({ ok: true })
httpHelloWorld
@hugenshen
// This approach creates a PC application with a left-side menu structure as requested.
HTTP
{ name: "待我审批", path: "/pending-approvals" },
function MenuItem({ item }) {
const [isOpen, setIsOpen] = React.useState(false);
</div>
function Menu() {
return (
</div>
function Content() {
return (
</div>
function App() {
return (
</BrowserRouter>
function client() {
ReactDOM.createRoot(document.getElementById("root")).render(<App />);
if (typeof document !== "undefined") { client(); }
async function server(request: Request): Promise<Response> {
return new Response(
rabbitstreamUtils
@tempdev
An interactive, runnable TypeScript val by tempdev
Script
const cryptoJs = CryptoJS;
function _kv(a) {
return [(a & 4278190080) >> 24, (a & 16711680) >> 16, (a & 65280) >> 8, a & 255];
return null;
export async function decryptURL(a, X) {
try {
return [];
export async function decryptSource(keys, kversion, response) {
let encrypted = response.sources;
return response;
export async function getMeta(id) {
const req = await fetch(`https://rabbitstream.net/v2/embed-4/${id}?z=`, {
return metaMatch[1];
export async function getWasm() {
const req = await fetch("https://rabbitstream.net/images/loading.png?v=0.6", {
CS1200Proj
@bhuvanh66
// Fetches a random joke.
Script
import { email } from "https://esm.town/v/std/email?v=9";
// Fetches a random joke.
function fetchRandomJoke() {
async function fetchRandomJoke() {
const response = await fetch(
"https://official-joke-api.appspot.com/random_joke",
valle_tmp_393084135551327933207099179023125
@janpaul123
// This approach uses the Hono framework to set up routes for handling comments.
HTTP
// Define the Hono app
const app = new Hono();
// Helper function to fetch all comments from the database
async function fetchComments() {
const result = await sqlite.execute(`SELECT * FROM comments ORDER BY timestamp DESC`);
return result.rows;
sqlite
@granin
LLM-Safe Fork of @std/sqlite We found that LLMs have trouble with the inputs and outputs of our @std/sqlite method. It expects the input to be (sql: string, args: any[]) but it's { sql: string, args: any[] } It expects the output to be objects, but they are arrays Instead of struggling to teach it, we built this val to be a wrapper around @std/sqlite that adheres to what the LLMs expect. This val is also backwards-compatible with @std/sqlite, so we're considering merging it in.
Script
import { nanoid } from "https://esm.sh/nanoid";
function App() {
const [specId, setSpecId] = useState("");
</div>
window.initClient = function() {
const rootElement = document.getElementById("root");
window.initClient();
export default async function server(request: Request): Promise<Response> {
// Handle GET requests by serving the HTML
try {
// Dynamic imports for SQLite and OpenAI
const [{ sqlite }, { OpenAI }] = await Promise.all([
import("https://esm.town/v/stevekrouse/sqlite"),
import("https://esm.town/v/std/openai")
const openai = new OpenAI();
const SPECIFICATIONS_TABLE = "val_town_agent_specifications";
4. Follow Unix philosophy: do one thing well
5. Use functional programming principles
6. Provide clear, concise, and efficient code
// Generate implementation using GPT-4o-mini
const completion = await openai.chat.completions.create({
messages: [
aqi
@dctalbot
dctalbot AQI Alerts Get email alerts when AQI is unhealthy near you.
Cron
import { email } from "https://esm.town/v/std/email?v=9";
import { easyAQI } from "https://esm.town/v/stevekrouse/easyAQI";
export async function aqi(interval: Interval) {
const location = "williamsburg brooklyn"; // <-- change to place, city, or zip code
const data = await easyAQI({ location });
web_iTwjIXAEMt
@dhvanil
An interactive, runnable TypeScript val by dhvanil
HTTP
export async function web_iTwjIXAEMt(req) {
return new Response(`<!DOCTYPE html>
<html>
grayDormouse
@natalyazheng1
// Fetches a random joke.
Cron
// Fetches a random joke.
// function fetchRandomJoke() {
// const SAMPLE_JOKE = {
// Fetches a random joke.
// function fetchRandomJoke() {
// const response = fetch(
// Fetches a random joke.
async function fetchRandomJoke() {
const response = await fetch(
BreathingInstructional
@nemonicui
This is an instructional when there is negative stress to help with relaxation.
HTTP
"Repeat for 5-10 cycles"
function App() {
const [selectedTechnique, setSelectedTechnique] = useState(null);
cursor: 'pointer'
function client() {
createRoot(document.getElementById("root")).render(<App />);
if (typeof document !== "undefined") { client(); }
export default async function server(request: Request): Promise<Response> {
return new Response(`