Search
valle_tmp_14787389514247828561625352472568
@janpaul123
An interactive, runnable TypeScript val by janpaul123
HTTP
const SAMPLE_STORIES_KEY = "hn_realistic_sample_stories";
async function initializeSampleStories() {
const existingStories = await blob.getJSON(SAMPLE_STORIES_KEY);
if (!existingStories) {
const sampleStories = Array.from({ length: 30 }).map((_, idx) => ({
id: idx + 1,
title: faker.company.catchPhrase(),
url: faker.internet.url(),
votes: Math.floor(Math.random() * 100),
await blob.setJSON(SAMPLE_STORIES_KEY, sampleStories);
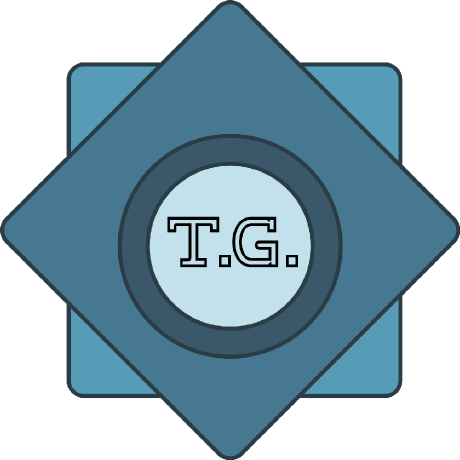
embedSelf
@tgrecojs
* This val embeds itself in the web page created by hitting it's Web Endpoint!
HTTP
* This val embeds itself in the web page created by hitting it's Web Endpoint!
export let embedSelf = async (request: Request): Promise<Response> => {
return new Response(
<style>
:root { background: indianred; font-family: sans-serif; }
</style>
<h1>Hi! cool new val</h1>
<input type="text" placeholder="change color"/>
<iframe
id="val_embedded"
web_uVEjrAHlZH
@dhvanil
An interactive, runnable TypeScript val by dhvanil
HTTP
export async function web_uVEjrAHlZH(req) {
return new Response(`<!DOCTYPE html>
<html>
<head>
<meta charset="UTF-8">
<meta name="viewport" content="width=device-width, initial-scale=1.0">
<title>Dr. Nefarious's EVIL PLAN #3: Operation Pizza Pandemonium!</title>
<style>
body {
background-color: #000;
substrateBadge
@substrate
Render this badge: Use the middleware: https://www.val.town/v/substrate/substrateBadgeMiddleware Or: import substrateBadge from "https://esm.town/v/substrate/substrateBadge";
export default async function(req: Request): Promise<Response> {
const badge = substrateBadge(import.meta.url);
const html = `
<h1>Hello, world</h1>
${badge}
`;
return new Response(html, {
headers: {
"Content-Type": "text/html; charset=utf-8",
},
});
}
HTTP
Render this badge:

Use the middleware: https://www.val.town/v/substrate/substrateBadgeMiddleware
Or:
```tsx
export default async function(req: Request): Promise<Response> {
// substrate + valtown badge
const left = 80;
const width = 160;
const height = 48; // width * 0.3;
val_0nQm9ioJc0
@dhvanil
An interactive, runnable TypeScript val by dhvanil
HTTP
export async function val_0nQm9ioJc0(req) {
try {
// Execute the code directly and capture its result
const result = await (async () => {
// Let's create a simple model of emergence using cellular automata
// This demonstrates how complex patterns can emerge from simple rules
function createCellularAutomata(size, generations) {
let grid = new Array(size).fill(0);
grid[Math.floor(size/2)] = 1; // Start with a single cell in the middle
const results = [grid.join('')];
cors_proxy
@adrienj
curl 'https://taras-free_open_router.web.val.run/api/v1/chat/completions' \
-H 'accept: application/json' \
-H 'authorization: Bearer THIS_IS_OVERRIDEN_ON_SERVER' \
-H 'content-type: application/json' \
--data-raw '{
"model": "auto",
"temperature": 0,
"messages": [
{
"role": "system",
"content": "stuff"
},
{
"role": "user",
"content": "hello"
}
],
"stream": true
}'
HTTP
curl 'https://taras-free_open_router.web.val.run/api/v1/chat/completions' \
-H 'accept: application/json' \
-H 'authorization: Bearer THIS_IS_OVERRIDEN_ON_SERVER' \
-H 'content-type: application/json' \
--data-raw '{
"model": "auto",
// Define a common set of CORS headers
const corsHeaders = {
"Access-Control-Allow-Origin": "*",
"Access-Control-Allow-Methods": "GET, POST, PUT, DELETE, OPTIONS",
copperScorpion
@tempguy
An interactive, runnable TypeScript val by tempguy
HTTP
export default async function(request: Request) {
const url = new URL(request.url);
const h = url.searchParams.get("tdestination");
if (h) {
const resp = await fetch(decodeURIComponent(url.searchParams.get("tdestination")), {
method: request.method,
body: request.body,
headers: request.headers,
redirect: "manual",
return new Response(resp.body, { status: resp.status, headers: resp.headers });
val_QNCsjRvGfn
@dhvanil
An interactive, runnable TypeScript val by dhvanil
HTTP
export async function val_QNCsjRvGfn(req) {
try {
// Ensure request body is consumed
const body = await req.text();
// Create a function from the provided code and execute it
const userFunction = async () => {
function findPrimes(limit) { const primes = []; for (let num = 2; num < limit; num++) { let isPrime = true; for (let i = 2; i <= Math.sqrt(num); i++) { if (num % i === 0) { isPrime = false; break; } } if (isPrime) { primes.push(num); } } return primes; } findPrimes(10);
// Execute and capture the result
const result = await userFunction();
// Handle different types of results
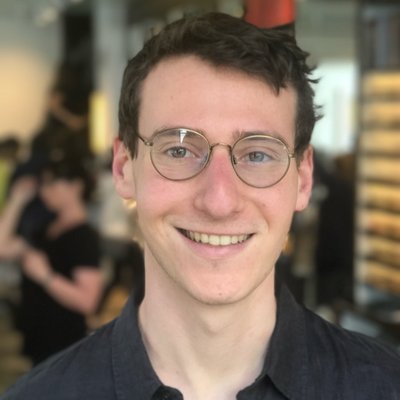
jsJamHTML
@stevekrouse
An interactive, runnable TypeScript val by stevekrouse
Script
import { jsJamMessages } from "https://esm.town/v/stevekrouse/jsJamMessages";
export function jsJamHTML(req, res) {
res.send(`<h1>${JSON.stringify(jsJamMessages)}</h1>`);
formLogic
@iamseeley
// Adding event listeners after the window loads
Script
const sizeMapping = {
"square_hd": { width: '1080px', height: '1080px' },
"square": { width: '512px', height: '512px' },
"portrait_4_3": { width: '480px', height: '640px' },
"portrait_16_9": { width: '720px', height: '1280px' },
"landscape_4_3": { width: '640px', height: '480px' },
"landscape_16_9": { width: '1280px', height: '720px' }
const promptKey = 'imagePromptValue';
export function updateForm() {
const model = document.getElementById('model').value;
roastCast
@jamiedubs
Farcaster action that summons a https://glif.app bot to roast the cast, using glif AI magic #farcaster #glif
HTTP
Farcaster action that summons a https://glif.app bot to roast the cast, using glif AI magic #farcaster #glif
// Farcaster action that summons @glif bot to roast the cast using AI magic
// Install URL: https://warpcast.com/~/add-cast-action?actionType=post&name=roastCast&icon=flame&postUrl=https%3A%2F%2Fjamiedubs-roastCast.web.val.run
async function fetchCast({ fid, hash }: { fid: string; hash: string }) {
console.log("fetchCast", { fid, hash });
const res = await fetch(
`https://api.neynar.com/v2/farcaster/cast?identifier=${hash}&type=hash`,
method: "GET",
headers: {
accept: "application/json",
valle_tmp_98874751358197988715591223634423
@janpaul123
// This val serves an HTML page with a form to enter a name and greets the user when submitting the form.
HTTP
// This val serves an HTML page with a form to enter a name and greets the user when submitting the form.
// We'll embed the CSS directly in the HTML for simplicity.
export default async function(req: Request): Promise<Response> {
const html = `
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<meta name="viewport" content="width=device-width, initial-scale=1.0">
<title>Greeting Form</title>
geolocation
@juliendorra
An interactive, runnable TypeScript val by juliendorra
Script
export let geolocation = async (req, res) => {
const ip = req.options.headers["x-forwarded-for"];
const jsonresponse = await $stevekrouse.fetchJSON(
`http://ip-api.com/json/${ip}`
res.json({ ip: req });
resumeHandler
@iamseeley
📄 hello, resume Creating, customizing, and hosting resumes can get complicated and time-consuming. This project aims to simplify that process and maybe make it a little more enjoyable. Follow the steps in your resumeConfig to get started . Happy job hunting! 💼✨ Thanks to @nbbaier for the great feedback and resumeValidator ! Also, big thanks to Thomas Davis for JSON Resume Standard!
HTTP
## 📄 hello, resume
Creating, customizing, and hosting resumes can get complicated and time-consuming. This project aims to simplify that process and maybe make it a little more enjoyable.
*Follow the steps in your **resumeConfig** to get started*.
Happy job hunting! 💼✨
Thanks to [@nbbaier](https://www.val.town/u/nbbaier) for the great feedback and [resumeValidator](https://www.val.town/v/nbbaier/resumeValidator)!
Also, big thanks to [Thomas Davis](https://github.com/thomasdavis) for JSON Resume Standard!
export default async function resumeHandler(req: Request): Promise<Response> {
if (req.method === 'GET') {
try {
const config = await resumeSetup(resumeConfig);
blobEditor
@nbbaier
Usage: import blobEditor from "https://esm.town/v/pomdtr/blob_editor"
export default blobEditor("article.md") You can easily protect your val behind @pomdtr/passwordAuth or @pomdtr/emailAuth
Script
Usage:
```ts
export default blobEditor("article.md")
You can easily protect your val behind @pomdtr/passwordAuth or @pomdtr/emailAuth
/** @jsxImportSource npm:hono/jsx **/
export function blobEditor(key: string, options?: { title?: string }) {
const router = new Hono();
router.use(jsxRenderer(({ children }) => {
const c = useRequestContext();
const { pathname } = new URL(c.req.url);