Search
agileApricotPossum
@Koka
@jsxImportSource https://esm.sh/react@18.2.0
Script
// Gamification and Progress Tracking
function UserProgress() {
const [points, setPoints] = useState(0);
// Pomodoro Focus Timer
function PomodoroTimer() {
const [timeLeft, setTimeLeft] = useState(25 * 60);
// Main App Component
function StudySparkApp() {
const [courses, setCourses] = useState([]);
// Client-side rendering
function client() {
createRoot(document.getElementById("root")).render(<StudySparkApp />);
// Server-side rendering
export default async function server(request: Request): Promise<Response> {
// Future: Integrate OpenAI for AI-powered task breakdown
// const { OpenAI } = await import("https://esm.town/v/std/openai");
return new Response(
astuteAquamarineAardvark
@julesb22
An interactive, runnable TypeScript val by julesb22
HTTP
export default async function(req: Request): Promise<Response> {
return Response.json({ data: true });
exceptionalBlushCarp
@toowired
An interactive, runnable TypeScript val by toowired
Script
import { blob } from '@val.town/utils';
export async function windsurfIDE(args) {
const { type, ...params } = args;
return { error: error.message };
async function handleStartGoal(goal) {
const state = await blob.get('windsurf_state') || {
nextAction: await handleAutoNext()
async function handleCompleteTask(taskId) {
const state = await blob.get('windsurf_state');
nextAction: await handleAutoNext()
async function handleAutoNext() {
const state = await blob.get('windsurf_state');
appliedRules: rules.map(r => r.name)
async function loadMemory(memoryId) {
const state = await blob.get('windsurf_state');
context: state.memories
async function applyRule(rule, target, state) {
// Rule application logic
return { override: false };
function prioritizeTask(task, state, criteria) {
// Task prioritization logic based on rules
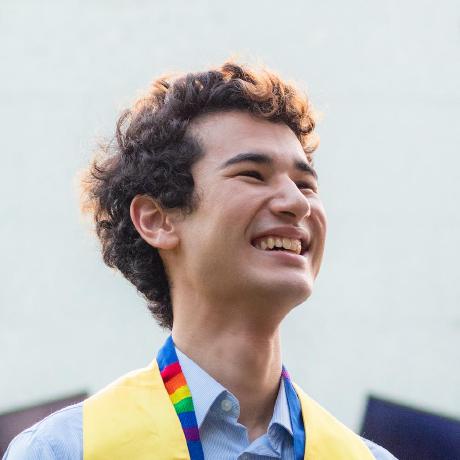
myApi
@florian
An interactive, runnable TypeScript val by florian
Script
export function myApi(name) {
return "hi " + name;
blogSqliteUniversePart2
@postpostscript
sqliteUniverse: Make SQLite Queries Against Multiple Endpoints in Deno (Val Town) (Part 2) If you haven't already, check out Part 1 for an overview of this system! Examples Example: find my vals that are related to my public tables import { Statement } from "https://esm.town/v/postpostscript/sqliteBuilder";
import { sqliteUniverse } from "https://esm.town/v/postpostscript/sqliteUniverse";
console.log(await Statement`
SELECT v.name AS val, t.name AS "table"
FROM "https://postpostscript-sqlitevals.web.val.run/vals" v
INNER JOIN "https://postpostscript-sqlitepublic.web.val.run/sqlite_schema" t
ON t.name LIKE ('https://postpostscript-sqlitepublic.web.val.run/' || v.name || '%')
AND t.type = 'table'
WHERE v.author_username = 'postpostscript'
`.execute({
sqlite: sqliteUniverse,
})) Since table names matching /^@/ will let you pass val names in the place of their HTTP endpoints, we can write the query as: SELECT v.name AS val, t.name AS "table"
FROM "@postpostscript/sqliteVals/vals" v
INNER JOIN "@postpostscript/sqlitePublic/sqlite_schema" t
ON t.name LIKE ('@postpostscript/sqlitePublic/' || v.name || '%')
AND t.type = 'table'
WHERE v.author_username = 'postpostscript' The result for the latter: [
{
val: "authId",
table: "@postpostscript/sqlitePublic/authIdExampleComments_comment"
},
{
val: "authIdExampleComments",
table: "@postpostscript/sqlitePublic/authIdExampleComments_comment"
}
] Example: query against a backup import { sqliteFromBlob } from "https://esm.town/v/postpostscript/sqliteBackup";
import { Statement } from "https://esm.town/v/postpostscript/sqliteBuilder";
import { defaultPatterns, patterns, sqliteUniverseWithOptions } from "https://esm.town/v/postpostscript/sqliteUniverse";
const sqlite = sqliteUniverseWithOptions({
interfaces: {
patterns: [
...defaultPatterns,
patterns.blob,
],
},
});
console.log(await Statement`
SELECT *
FROM "blob://backup:sqlite:1709960402936/someTable"
`.execute({ sqlite })); Example: query from @std/sqlite and public data simultaneously import { sqliteFromBlob } from "https://esm.town/v/postpostscript/sqliteBackup";
import { Statement } from "https://esm.town/v/postpostscript/sqliteBuilder";
import { defaultPatterns, sqliteUniverseWithOptions } from "https://esm.town/v/postpostscript/sqliteUniverse";
import { sqlite as sqlitePrivate } from "https://esm.town/v/std/sqlite?v=4";
const sqlite = sqliteUniverseWithOptions({
interfaces: {
patterns: defaultPatterns,
fallback({ endpoint, tables }) {
return sqlitePrivate
},
},
});
console.log(await Statement`
SELECT t.*, p.*
FROM privateTable t
JOIN "@example/sqlitePublic/publicTable" p
`.execute({ sqlite })); You could also do it like this to make it more explicit: const sqlite = sqliteUniverseWithOptions({
interfaces: {
exact: {
"@std/sqlite": sqlitePrivate,
},
patterns: defaultPatterns,
},
});
console.log(await Statement`
SELECT t.*, p.*
FROM "@std/sqlite/privateTable" t
JOIN "@example/sqlitePublic/publicTable" p
`.execute({ sqlite })); Next Steps I'd like to make patterns to allow queries against JSON and Val/ESM exports e.g. SELECT * FROM "json://example.com/example.json" or SELECT * FROM "export://@postpostscript/someVal/someExport" but those will have to come later! Another necessary feature for querying against larger databases will be to use the WHERE or JOIN conditions when dumping from them, but this will be more complicated P.S. This has been a super fun project to work on and I hope you find it interesting or inspiring too! Let me know if you find any errors in this post or if you'd like me to expand more on a specific feature, and let me know if you make anything cool with this and I'll make a note of it! P.P.S (😏) this interface is not 100% stable yet, so I would recommend pinning to specific versions if it not breaking is important to you P.P.P.S. Want to serve your own public SQLite endpoints? This is currently the simplest example I have of how to do that: @postpostscript/sqlitePublic
HTTP
import { extractValInfo } from "https://esm.town/v/pomdtr/extractValInfo?v=26";
import { getValEndpointFromName } from "https://esm.town/v/postpostscript/meta";
export default function(req: Request) {
const { author, name } = extractValInfo(import.meta.url);
const blogEndpoint = getValEndpointFromName(`@${author}/blog`);
resumeHandler
@siygle
📄 hello, resume Creating, customizing, and hosting resumes can get complicated and time-consuming. This project aims to simplify that process and maybe make it a little more enjoyable. Follow the steps in your resumeConfig to get started . Happy job hunting! 💼✨ Thanks to @nbbaier for the great feedback and resumeValidator ! Also, big thanks to Thomas Davis for JSON Resume Standard!
HTTP
import { resumeSetup } from "https://esm.town/v/iamseeley/resumeSetup";
import { resumeConfig } from "https://esm.town/v/siygle/resumeConfig";
export default async function resumeHandler(req: Request): Promise<Response> {
if (req.method === "GET") {
try {
cors_proxy
@adrienj
curl 'https://taras-free_open_router.web.val.run/api/v1/chat/completions' \
-H 'accept: application/json' \
-H 'authorization: Bearer THIS_IS_OVERRIDEN_ON_SERVER' \
-H 'content-type: application/json' \
--data-raw '{
"model": "auto",
"temperature": 0,
"messages": [
{
"role": "system",
"content": "stuff"
},
{
"role": "user",
"content": "hello"
}
],
"stream": true
}'
HTTP
"Access-Control-Allow-Methods": "GET, POST, PUT, DELETE, OPTIONS",
"Access-Control-Allow-Headers": "*",
export default async function(req: Request): Promise<Response> {
// Check if the request is an OPTIONS request
if (req.method === "OPTIONS") {
slackScout
@fahadakhan2
Slack scout sends a slack notification every time your keywords are mentioned on Twitter, Hacker News, or Reddit. Get notified whenever you, your company, or topics of interest are mentioned online. Built with Browserbase . Inspired by f5bot.com . Full code tutorial . Getting Started To run Slack Scout, you’ll need a Browserbase API key Slack Webhook URL: setup here Twitter Developer API key Browserbase Browserbase is a developer platform to run, manage, and monitor headless browsers at scale. We’ll use Browserbase to navigate to, and scrape our different news sources. We’ll also use Browserbase’s Proxies to ensure we simulate authentic user interactions across multiple browser sessions . Get started with Browserbase for free here . Twitter We’ve decided to use the Twitter API to include Twitter post results. It costs $100 / month to have a Basic Twitter Developer account. If you decide to use Browserbase, we can lend our token. Comment below for access. Once you have the SLACK_WEBHOOK_URL , BROWSERBASE_API_KEY , and TWITTER_BEARER_TOKEN , input all of these as Val Town Environment Variables . Project created by Sarah Chieng and Alex Phan 💌
Cron
export default async function(interval: Interval): Promise<void> {
async function createTable(): Promise<void> {
async function fetchHackerNewsResults(topic: string): Promise<Website[]> {
// async function fetchTwitterResults(topic: string): Promise<Website[]> {
async function fetchRedditResults(topic: string): Promise<Website[]> {
function formatSlackMessage(website: Website): string {
async function sendSlackMessage(message: string): Promise<Response> {
async function isURLInTable(url: string): Promise<boolean> {
async function addWebsiteToTable(website: Website): Promise<void> {
async function processResults(results: Website[]): Promise<void> {
randomTextGenerator
@aleaf
@jsxImportSource https://esm.sh/react
HTTP
import { createRoot } from "https://esm.sh/react-dom/client";
function App() {
const [paragraphs, setParagraphs] = useState(1);
</div>
function client() {
createRoot(document.getElementById("root")).render(<App />);
if (typeof document !== "undefined") { client(); }
export default async function server(request: Request): Promise<Response> {
const url = new URL(request.url);
if (url.searchParams.has("paragraphs")) {
const { OpenAI } = await import("https://esm.town/v/std/openai");
const openai = new OpenAI();
const completion = await openai.chat.completions.create({
messages: [
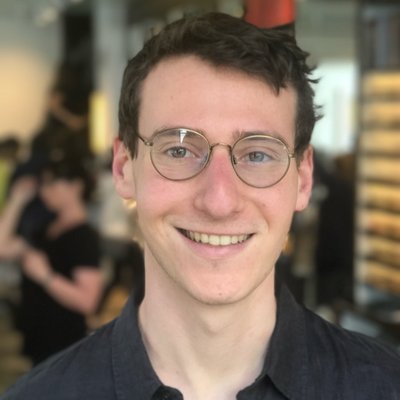
shySapphireLeopard
@stevekrouse
@jsxImportSource https://esm.sh/react
HTTP
import { createRoot } from "https://esm.sh/react-dom/client";
function App() {
const links = [
</div>
function client() {
createRoot(document.getElementById("root")).render(<App />);
if (typeof document !== "undefined") { client(); }
async function server(request: Request): Promise<Response> {
return new Response(
codeOnVT_modifyResponse
@andreterron
"Code on Val Town" Response modifier Appends the "Code on Val Town" ribbon to an HTTP Response. Usage new modifyResponse(res, { handle: "andre", name: "foo" }) - define which val to link to; new modifyResponse(res) - infer the val from the call stack. Example: @andreterron/openable_res import { modifyResponse } from "https://esm.town/v/andreterron/codeOnVT_modifyResponse";
import { html } from "https://esm.town/v/stevekrouse/html?v=5";
export default async (req: Request): Promise<Response> => {
return modifyResponse(html(`
<h2>Hello world!</h2>
<style>* { font-family: sans-serif }</style>
`));
};
Script
* @param res Response
* @param val Define which val should open. Defaults to the root reference.
export function modifyResponse(
res: Response,
{ val, style }: { val?: ValRef; style?: string } = {},
val_X6JYYp11Hg
@dhvanil
An interactive, runnable TypeScript val by dhvanil
HTTP
export default async function handler(req) {
try {
const result = await (async () => {
function isPrime(num) {
if (num <= 1) return false;
return true;
function findLowestThreeDigitPrime() {
for (let num = 100; num < 1000; num++) {
valle_tmp_26782413535145323813564331992605
@janpaul123
// This val will serve an HTML page emulating a Hacker News clone.
HTTP
// we can make it interactive with additional scripts.
export default async function(req: Request): Promise<Response> {
const url = new URL(req.url);
return new Response("Page not found", { status: 404 });
function serveHomePage(): Response {
const htmlContent = `
"Content-Type": "text/html",
function serveStoryPage(title: string, content: string): Response {
const htmlContent = `
mermaidHtml
@jdan
An interactive, runnable TypeScript val by jdan
Script
import { markov } from "https://esm.town/v/jdan/markov";
import { mermaidStateDiagramOfMarkov } from "https://esm.town/v/jdan/mermaidStateDiagramOfMarkov";
export async function mermaidHtml() {
const numElements = 30;
const ngram = 2;