Search
blob_admin
@pkf
Blob Admin This is a lightweight Blob Admin interface to view and debug your Blob data. Installation Use this button to install the val: It uses basic authentication with your Val Town API Token as the password (leave the username field blank). TODO [x] /new - render a page to write a new blob key and value [x] /edit/:blob - render a page to edit a blob (prefilled with the existing content) [x] /delete/:blob - delete a blob and render success [x] add upload/download buttons [ ] Use modals for create/upload/edit/view/delete page (htmx ?) [ ] handle non-textual blobs properly [ ] use codemirror instead of a textarea for editing text blobs
HTTP
# Blob Admin
This is a lightweight Blob Admin interface to view and debug your Blob data.
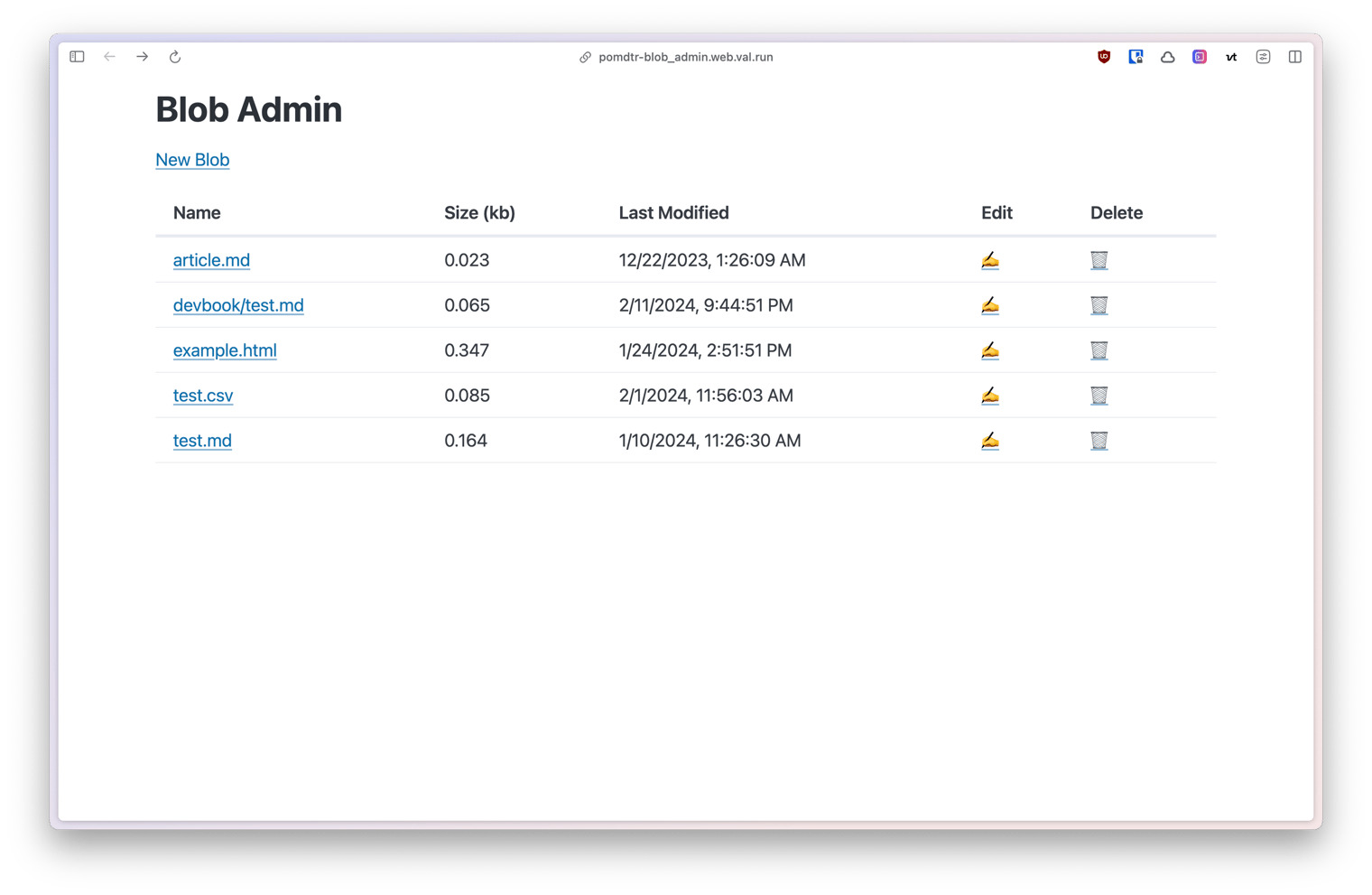
## Installation
Use this button to install the val:
[](https://www.val.town/v/stevekrouse/blob_admin_app/fork)
/** @jsxImportSource https://esm.sh/hono@4.0.8/jsx **/
* This val website is protected by a password.
* Access requires an API token named "blob".
const app = new Hono();
valle_tmp_137659959713258178037932973670666
@janpaul123
// This script will respond with a basic HTML page, including styled content, using the built-in Response class in TypeScript.
HTTP
// This script will respond with a basic HTML page, including styled content, using the built-in Response class in TypeScript.
* HTTP Val: Hello World Example (HTML Response with CSS)
* This script will respond with a basic HTML page that says "Hello world" to any incoming HTTP request, and includes some cool CSS styles.
export default async function(req: Request): Promise<Response> {
const htmlContent = `
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<meta name="viewport" content="width=device-width, initial-scale=1.0">
GithubCommits
@iamseeley
An interactive, runnable TypeScript val by iamseeley
Script
/** @jsx jsx */
/** @jsxFrag Fragment */
import { jsx, Fragment } from 'https://deno.land/x/hono/middleware.ts'
export default function GithubCommits({}) {
return (
val_0LDoys9VFk
@dhvanil
An interactive, runnable TypeScript val by dhvanil
HTTP
export async function val_0LDoys9VFk(req) {
try {
// Execute the code directly and capture its result
const result = await (async () => {
// Create a mermaid diagram analyzing escape possibilities
const diagram = `
sequenceDiagram
participant S as Simulation Layer
participant W as Weak Points
participant E as Escape Methods
password_manager_demo
@vgrichina
An interactive, runnable TypeScript val by vgrichina
HTTP
export default async function auth(req: Request) {
const html = (content: string) =>
`<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<meta name="viewport" content="width=device-width, initial-scale=1.0">
<title>Password Demo</title>
<style>
body {
glifbux
@jamiedubs
this is the fake, zero-security economy for glif chatbots https://glif.app it is the poorest man's blockchain see also our imaginary NFT marketplace: https://www.val.town/v/jamiedubs/glifinventory
HTTP
this is the fake, zero-security economy for glif chatbots <https://glif.app>
it is the poorest man's blockchain
see also our imaginary NFT marketplace: https://www.val.town/v/jamiedubs/glifinventory
const SCHEMA_VERSION = 9; // Increased due to transaction handling improvement
const KEY = new URL(import.meta.url).pathname.split("/").at(-1);
const TABLE_NAME = `${KEY}_wallets_${SCHEMA_VERSION}`;
// accounts start with this much money
const startingBalance = 100;
interface ApiResponse {
status: number;
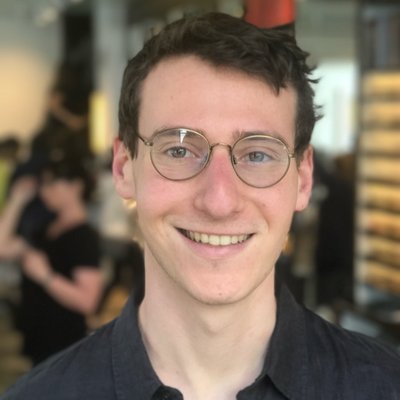
auth_middleware
@stevekrouse
Authentication middleware Guards your public http vals behind a login page. This val use a json web token stored as an http-only cookie to persist authentication. Usage Set an AUTH_SECRET_KEY env variable (used to sign/verify jwt tokens). Use an API token to authenticate. import { auth } from "https://esm.town/v/pomdtr/auth_middleware";
async function handler(req: Request): Promise<Response> {
return new Response("You are authenticated!");
}
export default auth(handler); See @pomdtr/test_auth for an example â ď¸ Make sure to only provides your api token to vals you trust (i.e. your own), as it gives access to your whole account.
Script
# Authentication middleware
Guards your public http vals behind a login page.
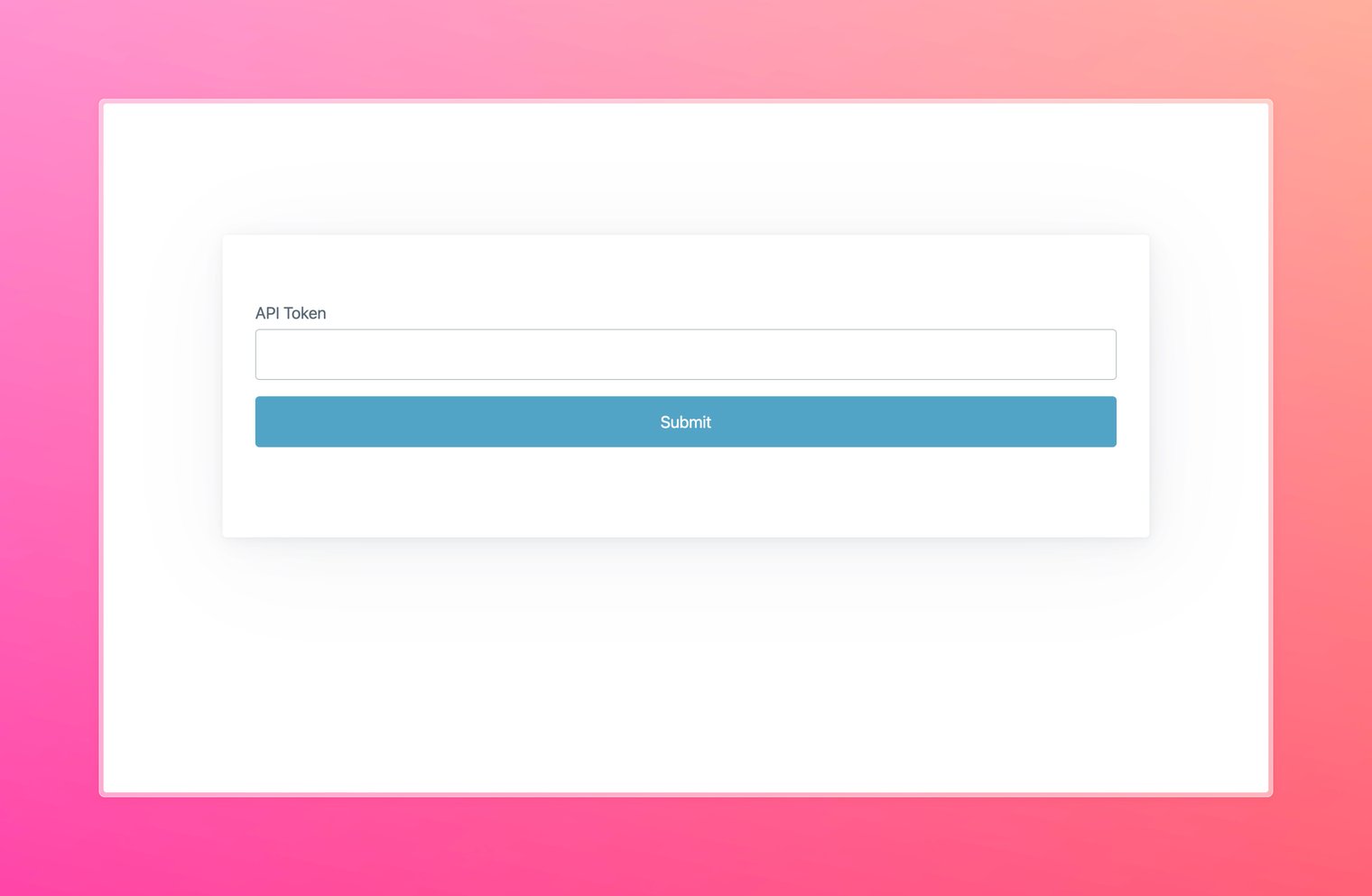
This val use a json web token stored as an http-only cookie to persist authentication.
## Usage
Set an `AUTH_SECRET_KEY` env variable (used to sign/verify jwt tokens). Use an API token to authenticate.
const cookieName = "user-jwt";
const loginPage = `<html>
<head>
<link rel="icon" href="https://fav.farm/đ" />
stargate
@git
An interactive, runnable TypeScript val by git
HTTP
export default async (request: Request): Promise<Response> => {
return new Response(
/* html */ `<html>
<head>
<script
src="https://unpkg.com/@layerzerolabs/stargate-ui@latest/element.js"
defer
async>
</script>
</head>
tanCrane
@frmysantana
An interactive, runnable TypeScript val by frmysantana
HTTP
export default async function tacoBellNutritionScrapper(req) {
const sourceUrl = `https://www.nutritionix.com/taco-bell/menu/premium`;
const siteText = await fetch(sourceUrl);
const $ = cheerio.load(await siteText.text());
const items = $("tr.odd, tr.even").map((i, e) => {
const name = $(e).find(".nmItem").html();
const calories = $(e).find("[aria-label*='Calories']").html();
return { name: name, calories: calories };
}).toArray();
return Response.json({ items });

webHTMLExample
@neverstew
// View at https://neverstew-webHTMLExample.web.val.run?name=Steve
HTTP
// View at https://neverstew-webHTMLExample.web.val.run?name=Steve
export async function webHTMLExample(req: Request) {
const query = new URL(req.url).searchParams;
return new Response(
`<h1>Hi ${query.get("name") || (await req.json()).name}!</h1>`,
{ headers: { "Content-Type": "text/html" } },
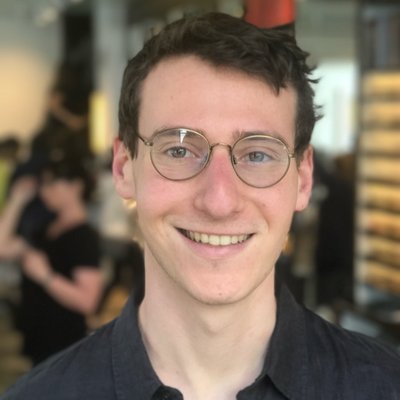
anthropicStreamDemo
@stevekrouse
An interactive, runnable TypeScript val by stevekrouse
HTTP
export default async (req: Request) => {
const anthropic = new Anthropic();
let { readable, writable } = new TransformStream();
let writer = writable.getWriter();
const textEncoder = new TextEncoder();
anthropic.messages.stream({
model: "claude-3-haiku-20240307",
max_tokens: 1024,
system: `Be funny`,
messages: [
alquranApiProxy
@rahil224
An interactive, runnable TypeScript val by rahil224
HTTP
export default async function server(request: Request): Promise<Response> {
// Extract the path after the first segment
const url = new URL(request.url);
const proxyPath = url.pathname.split("/").slice(2).join("/");
// Construct the full API URL
const apiUrl = `http://api.alquran.cloud/${proxyPath}${url.search}`;
try {
// Proxy the request to the Alquran Cloud API
const apiResponse = await fetch(apiUrl, {
method: request.method,
sunsetNYCalendar
@ejfox
// This approach uses the Sunset and Sunrise API to get sunset times for New York,
HTTP
// This approach uses the Sunset and Sunrise API to get sunset times for New York,
// and creates a simple form to generate a calendar of events occurring before sunset.
async function server(request: Request): Promise<Response> {
try {
const url = new URL(request.url);
if (url.pathname === "/generate-calendar") {
const action = url.searchParams.get("action");
const minutesBefore = parseInt(url.searchParams.get("minutesBefore"));
const endDate = new Date(url.searchParams.get("endDate"));
if (!action || isNaN(minutesBefore) || isNaN(endDate.getTime())) {

flightRadar24
@nishant
usage: async function example() {
await @nini1294.flightRadar24(
"bf721",
"01 Jan 2023",
"America/Los_Angeles"
);
}
Script
usage:
```js
async function example() {
await @nini1294.flightRadar24(
"bf721",
"01 Jan 2023",
// import { persistence } from "https://esm.town/v/raylu/persistence";
// import { get } from "https://esm.town/v/std/get";
export const flightRadar24 = async (
flightNumber,