Search
filterVals
@nbbaier
filterVals This val exports a utility function that returns a list of all a user's val, filtered by a callback function. Example Get the names of all your private vals: import { filterVals } from "https://esm.town/v/nbbaier/filterVals";
const id = <user_id>
const vals = await filterVals(id, (v) => v.privacy === "private")
const privateValNames = vals.map(v => v.name);
Script
# filterVals
This val exports a utility function that returns a list of all a user's val, filtered by a callback function.
## Example
import { fetchPaginatedData } from "https://esm.town/v/nbbaier/fetchPaginatedData";
export async function filterVals(id: string, filter: (value: any, index: number, array: any[]) => unknown) {
const token = Deno.env.get("valtown");
const res = await fetchPaginatedData(`https://api.val.town/v1/users/${id}/vals`, {
enchantingSilverPelican
@oscarkong
// Fetches a random joke.
Script
import { email } from "https://esm.town/v/std/email?v=9";
// Fetches a random joke.
function fetchRandomJoke() {
const response = fetch(
"https://official-joke-api.appspot.com/random_joke",
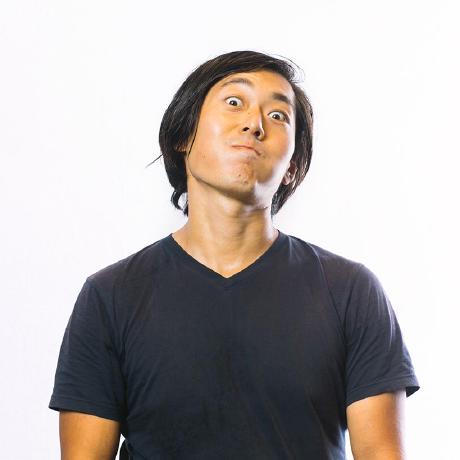
threeJsTorus
@yawnxyz
playing around with townie during a meeting >.>
HTTP
// The scene is rendered client-side using WebGL.
export default async function server(request: Request): Promise<Response> {
const html = `
let previousMousePosition = { x: 0, y: 0 };
function animate() {
requestAnimationFrame(animate);
window.addEventListener('resize', onWindowResize, false);
function onWindowResize() {
camera.aspect = window.innerWidth / window.innerHeight;
document.addEventListener('mouseup', onMouseUp, false);
function onMouseDown(event) {
isDragging = true;
y: event.clientY
function onMouseMove(event) {
if (!isDragging) return;
previousMousePosition = { x: event.clientX, y: event.clientY };
function onMouseUp(event) {
isDragging = false;
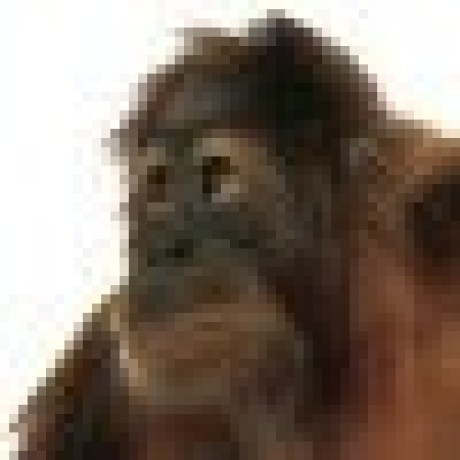
extractValInfo
@pomdtr
Extract vals infos (author, name, version) from a val url (either from esm.town or val.town ). Example usage: const {author, name} = extractValInfo(import.meta.url) Also returns a unique slug for the val: <author>/<name>
Script
export function extractValInfo(url: string | URL) {
const { pathname, search } = new URL(url);
const [author, filename] = pathname.split("/").slice(-2);

cronEvalLogger
@neverstew
Log your cron evaluations to sqlite import { cronEvalLogger } from "https://esm.town/v/neverstew/cronEvalLogger";
const yourCron = async (interval: Interval) => {
console.log("your code goes here");
};
export default cronEvalLogger(yourCron);
Script
import { fetchJSON } from "https://esm.town/v/stevekrouse/fetchJSON?v=45";
import { refs } from "https://esm.town/v/stevekrouse/refs?v=11";
type CronFunction = (interval: Interval) => Promise<void>;
export const cronEvalLogger = (cron: CronFunction): CronFunction => {
let interval: Interval;
const cronProxy = new Proxy(cron, {
FramerAPIWrapper
@charmaine
API Wrapper The following is an example of wrapping the use of an API in a small backend function. This pattern is useful for preparing data before using in Framer, and is used in the Fetch tutorial video. It follows the pattern of calling a source API, parsing the data, setting up the CORS headers, and then responding with data.
HTTP
# API Wrapper
The following is an example of wrapping the use of an API in a small backend function. This pattern is useful for preparing data before using in Framer, and is used in the Fetch tutorial video. It follows the pattern of calling a source API, parsing the data, setting up the CORS headers, and then responding with data.
export default async function(req: Request) {
const data = await fetch("https://api.fetch.tools/status");
const status = await data.json();
testing
@tmcw
An interactive, runnable TypeScript val by tmcw
Script
const stream = test.createStream({ objectMode: true });
stream.on("data", function(row) {
console.log(JSON.stringify(row));
test("timing test", function(t) {
t.plan(2);
t.equal(typeof Date.now, "function");
var start = Date.now();
setTimeout(function() {
t.equal(Date.now() - start, 100);
export const testing = new Promise(resolve => {
stream.on("end", function(row) {
resolve("ok");
emailRandomJoke
@liu_david3
// Fetches a random joke.
Cron
import { email } from "https://esm.town/v/std/email?v=9";
// Fetches a random joke.
async function fetchRandomJoke() {
const response = await fetch(
"https://official-joke-api.appspot.com/random_joke",
val_S1NNtqMDtf
@dhvanil
An interactive, runnable TypeScript val by dhvanil
Script
export default async function handler(req) {
try {
const result = await (async () => {
function isPrime(num) {
if (num <= 1) return false;
return true;
function findLowestThreeDigitPrime() {
for (let num = 100; num < 1000; num++) {
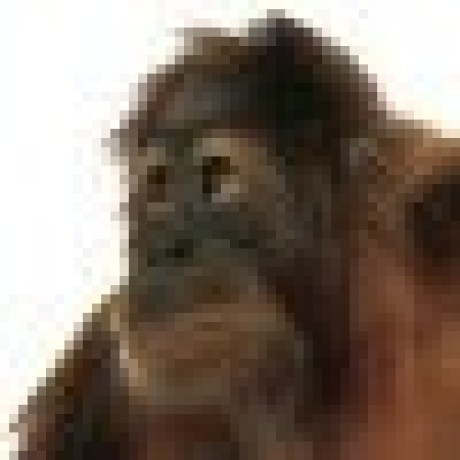
bearerAuth
@pomdtr
An interactive, runnable TypeScript val by pomdtr
Script
function extractToken(authorization: string) {
const parts = authorization.split(" ");
if (parts[0] != "Bearer") {
return null;
return parts[1];
export function bearerAuth(next: (req: Request) => Response | Promise<Response>, params: {
verifyToken: (password: string) => boolean | Promise<boolean>;
return async (req: Request) => {
SAMPLE_JOKE
@elijahiscoding
// Fetches a random joke.
Cron
import { email } from "https://esm.town/v/std/email?v=9";
// Fetches a random joke.
function fetchRandomJoke() {
const SAMPLE_JOKE = {
"setup": "What do you call a group of disorganized cats?",
subject: setup,
// Fetches a random joke.
async function fetchRandomJoke() {
const response = await fetch(
"https://official-joke-api.appspot.com/random_joke",
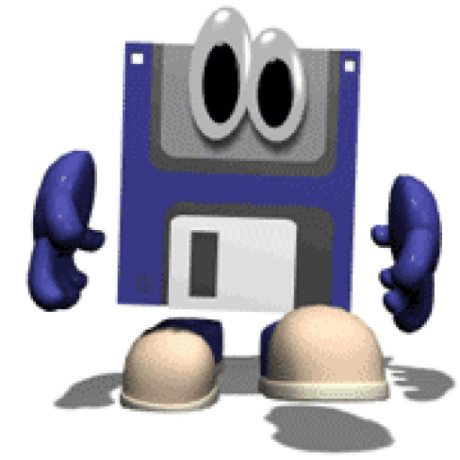
connect4
@saolsen
An interactive, runnable TypeScript val by saolsen
Script
export type Action = z.infer<typeof Action>;
export function get(state: State, col: number, row: number): Slot {
return state.board[col][row];
export function set(state: State, col: number, row: number, slot: Slot): void {
state.board[col][row] = slot;
| "column_out_of_bounds";
export function check_action(state: State, action: Action): ActionCheck {
if (action.player !== state.next_player) {
return "ok";
function check_slots_eq(a: Slot, b: Slot, c: Slot, d: Slot): Slot {
if (a === b && b === c && c === d) {
return null;
export function status(state: State): Status {
// Check Vertical Win
return { status: "over", result: { kind: "draw" } };
export function apply_action(state: State, action: Action): Status {
// Action should be checked before calling this function.
// We assert that the action is ok.
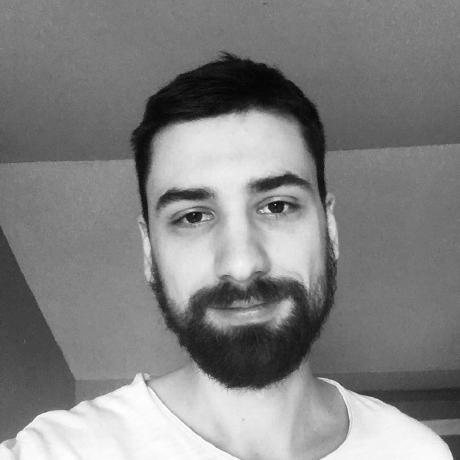
moveHead
@adamgonda
An interactive, runnable TypeScript val by adamgonda
Script
export function moveHead(head, dir) {
if (["RIGHT", "LEFT"].includes(dir)) {
return {
web_YhrAkPFife
@dhvanil
An interactive, runnable TypeScript val by dhvanil
HTTP
export async function web_YhrAkPFife(req) {
return new Response(`<!DOCTYPE html>
<html>
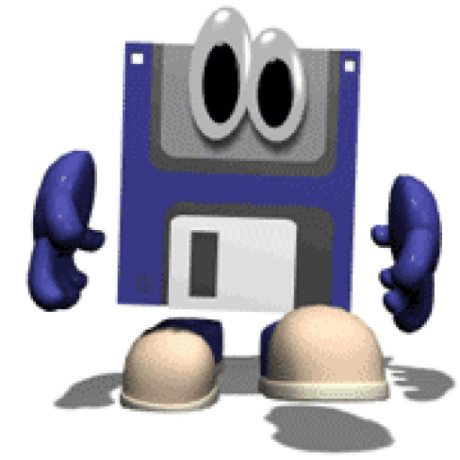
testCodemirrorTsBrowserEmacs
@saolsen
@jsxImportSource https://esm.sh/react
HTTP
/** @jsxImportSource https://esm.sh/react */
import { renderToString } from "npm:react-dom/server";
export default async function(req: Request): Promise<Response> {
return new Response(
renderToString(
<textarea id="editorSource" className="for-codemirror" name="editorSource">
{`let hasAnError: string = 10;
function increment(num: number) {
return num + 1;
increment('not a number');`}