Search
maroonBadger
@masd1
// Cheerio accepts a CSS selector, here we pick the second <p>
Script
import { html, load } from "npm:cheerio";
const htmlStr = await fetchText(
"https://archive.is/pPFRB",
const $ = load(htmlStr);
// Cheerio accepts a CSS selector, here we pick the second <p>
const intro = $.html();
console.log(intro);
art_app
@char_lie
An interactive, runnable TypeScript val by char_lie
HTTP
import { art_criticism_app } from "https://esm.town/v/char_lie/art_criticism_app";
export const art_app = async (req: Request) => {
const marked = await import("npm:marked");
const art_info = await art_criticism_app();
return new Response(
marked.parse(`
${art_info.idea}

*Verdict*: ${art_info.criticism}
{ headers: { "Content-Type": "text/html" } },
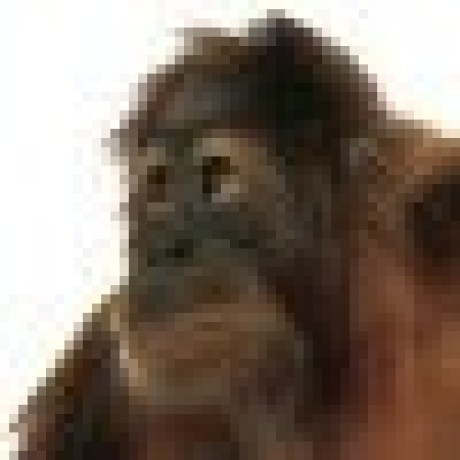
webdav
@pomdtr
An interactive, runnable TypeScript val by pomdtr
HTTP
import { serveVals } from "https://esm.town/v/pomdtr/webdavServer";
export default basicAuth(serveVals, {
verifyUser: (user) => {
return verifyToken(user);
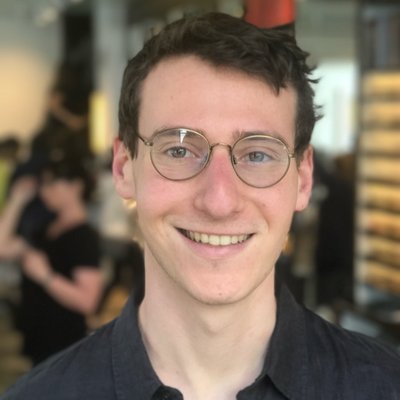
oak_demo
@stevekrouse
Oak Server Example
HTTP
# Oak Server Example
import { Application } from "https://deno.land/x/oak/mod.ts";
const app = new Application();
app.use((ctx) => {
ctx.response.body = "Hello Val Town!";
export default app.fetch;
labLogin
@todepond
An interactive, runnable TypeScript val by todepond
HTTP
export default async function(req: Request): Promise<Response> {
const body = await req.json();
let { username, password } = body;
if (!username || !password) {
return new Response(JSON.stringify({ error: "Missing username or password" }), { status: 400 });
if (password.length > 50) {
return new Response(JSON.stringify({ error: "Password too long" }), { status: 400 });
if (username.length > 50) {
return new Response(JSON.stringify({ error: "Username too long" }), { status: 400 });
const TABLE_NAME = "lab_login_users_with_times";
astuteAquamarineAardvark
@julesb22
An interactive, runnable TypeScript val by julesb22
HTTP
export default async function(req: Request): Promise<Response> {
return Response.json({ data: true });
RobotBackupCallGraph
@synapticrobotics
An interactive, runnable TypeScript val by synapticrobotics
HTTP
export default async function server(req: Request): Promise<Response> {
// Handle GET requests
if (req.method === "GET") {
return new Response(
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<meta name="viewport" content="width=device-width, initial-scale=1.0">
<title>Robot Backup Analysis</title>
spacexapi
@moe
An interactive, runnable TypeScript val by moe
HTTP
export default async function(req: Request): Promise<Response> {
const data = await getLaunches()
return Response.json(data)
export async function getLaunches() {
var y10to19 = await loadPage(
"https://en.wikipedia.org/wiki/List_of_Falcon_9_and_Falcon_Heavy_launches_(2010%E2%80%932019)",
var y20to22 = await loadPage(
"https://en.wikipedia.org/wiki/List_of_Falcon_9_and_Falcon_Heavy_launches_(2020%E2%80%932022)",
var current = await loadPage("https://en.wikipedia.org/wiki/List_of_Falcon_9_and_Falcon_Heavy_launches")
var data = {
cheerioExample
@tmcw
cheerio cheerio is a popular npm module that makes it easy to parse and manipulate HTML and XML. cheerio is modeled after a reduced version of jQuery . Note that it's pretty different than default browser DOM methods, so you can't call things like .appendChild or document.createElement when using cheerio: it's not based on a full-fledged DOM or browser implementation. But, in exchange it's a lot faster and simpler.
Script
# cheerio
[cheerio](https://cheerio.js.org/) is a popular npm module that makes it easy to parse and manipulate HTML and XML.
cheerio is modeled after a reduced version of [jQuery](https://jquery.com/). Note that it's pretty different than default browser DOM methods, so you can't call things like `.appendChild` or `document.createElement` when using cheerio: it's not based on a full-fledged DOM or browser implementation. But, in exchange it's a lot faster and simpler.
export let cheerioExample = (async () => {
const { default: $ } = await import("npm:cheerio");
const document = $(`<article>
<div>Hello</div>
<span>World</span>
</article>`);
return document.find("span").text();
sharedMagentaChameleon
@jeffreyyoung
A simple poe bot
HTTP
A simple poe bot
export default serve({
async *handleMessage(req) {
const lastMessage = req.query.at(-1)?.content;
for await (
const event of forward({
accessKey: Deno.env.get("botToken1"),
query: req,
toBotName: "StableDiffusionXL",
if (event.event !== "done") {
refreshAnimalWidget
@crsven
An interactive, runnable TypeScript val by crsven
Cron
export let refreshAnimalWidget = async () => {
const bird = await fetchABird();
const photoUrl = await getBirdPhotoUrl(
`${bird.gen} ${bird.sp}`,
const message = `${bird.en} (${bird.cnt})`;
const colorCombo = await getNextColorCombo();
const newState = { ...animalWidgetJson };
const birdName = bird.en.replace(" ", "+");
newState.data.content_url =
`https://api.val.town/express/@crsven.embedBirdSound?args=${birdName},${bird.file},${photoUrl}`;
blob_admin_edit
@Daniel
@jsxImportSource https://esm.sh/hono@4.0.8/jsx
Script
/** @jsxImportSource https://esm.sh/hono@4.0.8/jsx **/
const route = new Hono();
route.get("/:name", async (c) => {
const name = c.req.param("name");
const resp = await blob.get(name);
const content = await resp.text();
return c.render(
<form method="POST">
<h1>
<input name="new_name" value={name} />
grandBlackReindeer
@Azazil
An interactive, runnable TypeScript val by Azazil
HTTP
import cheerio from "npm:cheerio";
const response = await fetch("https://example.com");
const body = await response.text();
const $ = cheerio.load(body);
console.log($("a").toArray().flatMap(el =>
el.attributes.filter(a => a.name === "href").map(a => a.value)
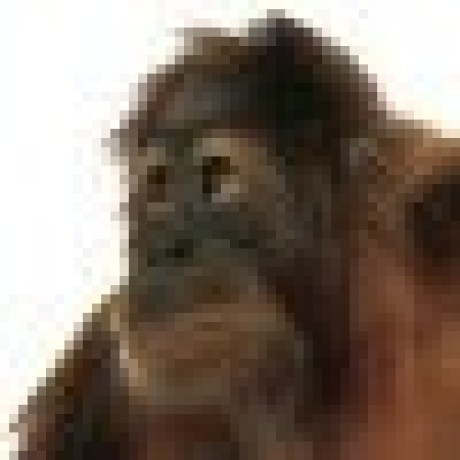
astro
@pomdtr
Astro served from Val Town (with SSR) ! The ultimate goal would be to serve it if from jsr, but the deno astro adapter still relies on deno.land.
HTTP
# Astro served from Val Town (with SSR) !
The ultimate goal would be to serve it if from jsr, but the deno astro adapter still relies on deno.land.
import { handle } from "https://raw.githubusercontent.com/pomdtr/astro-val-town-template/c57a0694e0c9e3927083580b38d8e282c2/mod.ts";
export default handle;
hello_cli
@jxnblk
Example CLI hosted on Val Town Install with Deno on your computer (name it whatever you want): deno install -n hello https://esm.town/v/jxnblk/hello_cli Run: hello Uninstall: deno uninstall hello Read the deno install docs
Script
Example CLI hosted on Val Town
Install with Deno on your computer (name it whatever you want):
```sh
deno install -n hello https://esm.town/v/jxnblk/hello_cli
Run:
```sh
// deno install -n hello https://esm.town/v/jxnblk/hello_cli
export default function main() {
const name = Deno.args[0] || "";
console.log(`hello ${name}`);