Search
val_WUemzNXhaN
@dhvanil
An interactive, runnable TypeScript val by dhvanil
HTTP
export async function val_WUemzNXhaN(req) {
try {
// Execute the code directly and capture its result
const result = await (async () => {
// Let's create a model of emergence that shows how simple patterns combine
// to create complex structures - similar to how DNA builds life
function createEmergentPatterns(basePatterns, combinations) {
// Define some basic pattern types (like DNA bases)
const patterns = {
A: [1, 0, 1],
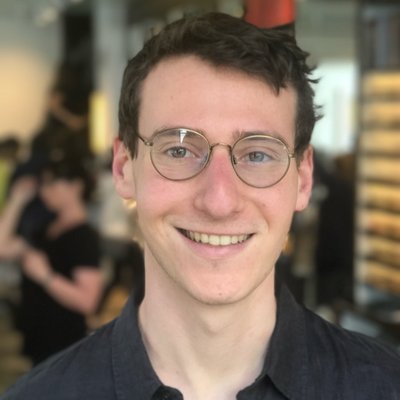
json_viewer
@stevekrouse
Inspector to browser json data in HTTP vals Example: https://val.town/v/stevekrouse/weatherDescription Thanks @mmcgrana (https://markmcgranaghan.com/) for the idea!
Script
# Inspector to browser json data in HTTP vals
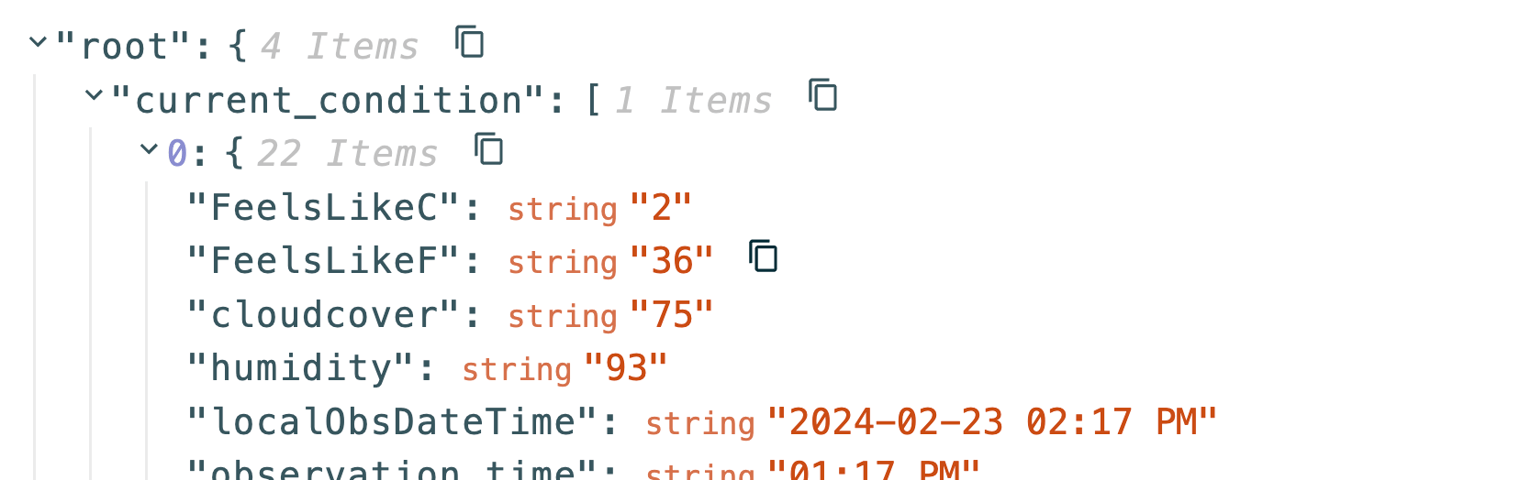
Example: https://val.town/v/stevekrouse/weatherDescription
Thanks @mmcgrana (https://markmcgranaghan.com/) for the idea!
export const json_viewer = (data) => (req: Request) => {
const accept = accepts(req);
if (!accept.type("html")) {
return Response.json(data);
return html(`<!DOCTYPE html>
<html lang="en">
returnJsonData
@suleiman_hamza
An interactive, runnable TypeScript val by suleiman_hamza
HTTP
export default async function (request: Request): Promise<Response> {
const data = {
message: "Hello, JSON World! 🌍",
timestamp: new Date().toISOString(),
status: "active"
return new Response(JSON.stringify(data), {
headers: {
"Content-Type": "application/json"
getTeams
@brianleroux
An interactive, runnable TypeScript val by brianleroux
Script
export default async function getTeams() {
let url = "https://www.capfriendly.com";
let res = await fetch(url);
let html = await res.text();
let doc = new DOMParser().parseFromString(html, "text/html");
let table = doc.querySelector("table#cf_homepage__teamTable");
let headers = Array.from(table.querySelectorAll("th")).map(h => h.textContent.trim()).map(e => e === "" ? "?" : e);
let rawRows = Array.from(table.querySelectorAll("tr")).map(r => {
let cells = Array.from(r.querySelectorAll("td"));
let cellData = cells.map(cell => cell.textContent.trim());
valle_tmp_63664219849257147630054266136499
@janpaul123
// This val responds with an HTML form to input the user's name and greets them upon form submission
HTTP
// This val responds with an HTML form to input the user's name and greets them upon form submission
export default async function(req: Request): Promise<Response> {
if (req.method === "POST") {
const formData = new URLSearchParams(await req.text());
const name = formData.get("name") || "stranger";
const htmlResponse = `<h1>Hello, ${name}!</h1>`;
return new Response(htmlResponse, {
headers: { "Content-Type": "text/html" },
} else {
const htmlForm = `
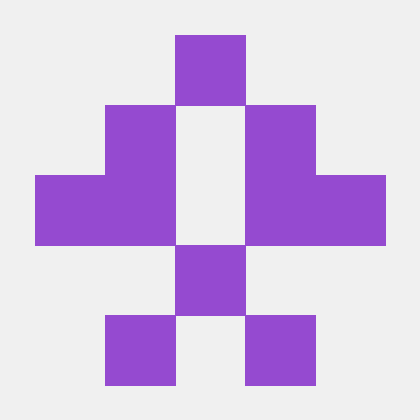
rule
@cescyang
An interactive, runnable TypeScript val by cescyang
Script
export function rule(response) {
const requiredApps = [
"55a54008ad1ba589aa210d2629c1df41",
"94b3bfd329374b9fb288f72cfcdcd4da",
"9ddc1d1392af462895f23c9aa7a64de1",
"30055ae3c14f46c38f11df1833a51893",
// "da65c5be95db4c09a68627e81a44d63c", // Scaffolding
"1876cd4464a749dbab0acfd57d797f90",
"15e54d64b8de45b1b869d012ba346c2f",
"6631176c87a24532b1a109205aec5e51",
valle_tmp_58066747016530698388629314307403
@janpaul123
// This val will respond with an HTML greeting to any HTTP request
HTTP
// This val will respond with an HTML greeting to any HTTP request
export default async function (req: Request): Promise<Response> {
return new Response(`
<html>
<head>
<title>Hello, World!</title>
</head>
<body>
<h1>Hello, world!</h1>
</body>
arenaApiExample
@deblina
Are.na API Example of using the are.na API to get the contents of an Are.na board - specifically my fun board that showcases websites with that "glossy black style." All you need is fetch to make this happen!
Script
# Are.na API
Example of using the [are.na](https://dev.are.na/documentation/channels#Block43472) API to get the contents of an Are.na board - specifically my fun board that showcases websites with that "glossy black style."
All you need is [fetch](https://developer.mozilla.org/en-US/docs/Web/API/Fetch_API) to make this happen!
import { fetch } from "https://esm.town/v/std/fetch";
export let arenaApiExample = (async () => {
const resp = await fetch(
"http://api.are.na/v2/channels/project-logs",
const json = await resp.json();
console.log(json);
return json;
handler
@maxjoygit
Hono Here's an example using the Hono server library with the Web API . It works great! Server examples Hono Peko Itty Router Nhttp
HTTP
# Hono
Here's an example using the [Hono](https://hono.dev/) server library with the [Web API](https://docs.val.town/api/web). It works great!
### Server examples
- [Hono](https://www.val.town/v/tmcw.honoExample)
- [Peko](https://www.val.town/v/tmcw.pekoExample)
- [Itty Router](https://www.val.town/v/tmcw.ittyRouterExample)
// src/index.ts
const app = new Hono();
app.get("/", (c) => c.text("Hello Lagon!"));
app.get("/test", (c) => c.text("Hello Lagon test!"));
posts
@jorrdang
An interactive, runnable TypeScript val by jorrdang
Cron
import { fetch } from "https://esm.town/v/std/fetch";
export const posts = async () => {
const data = await fetch("https://jsonplaceholder.typicode.com/posts");
return data;
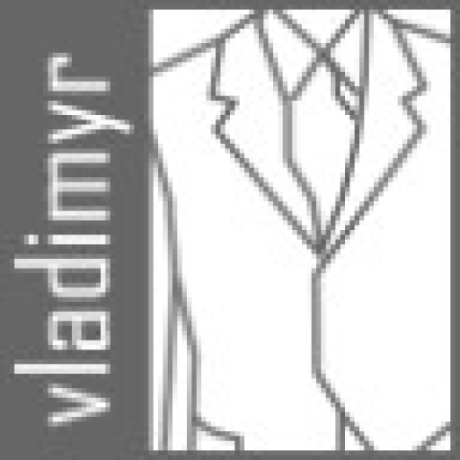
unpkg
@vladimyr
An interactive, runnable TypeScript val by vladimyr
HTTP
const { author, name } = extractValInfo(import.meta.url);
const BASE_URL = `https://${author}-${name}.web.val.run`;
const app = new Hono();
app.get("/-/:params{x;[^/]+}/:filename", (c) => {
const params = Object.fromEntries(new URLSearchParams(c.req.param("params").slice(2).replace(/;/g, "&")));
try {
const redirectUrl = new TextDecoder().decode(decodeBase64Url(params.url));
return c.redirect(new URL(redirectUrl).href);
} catch {
return c.text("400 Bad Request", 400);
tanaSave
@nbbaier
An interactive, runnable TypeScript val by nbbaier
Script
const token = Deno.env.get("tanaInputAPI");
export const tanaSave = async (req: Request) => {
const app = new Hono();
app.get("/save", async c => {
let { text, url } = c.req.query();
const payload: APIPlainNode = {
name: text,
children: [
{ name: "child 1" },
{ type: "field", attributeId: "cwi23sOzRSh8" },

competentBlushPuffin
@wilt
An interactive, runnable TypeScript val by wilt
HTTP
import app from "https://wilt-npmproxy.web.val.run/cachebust4/raw.githubusercontent.com/wilt00/bskye/refs/heads/type-imports/src/index.ts";
export default app.fetch;
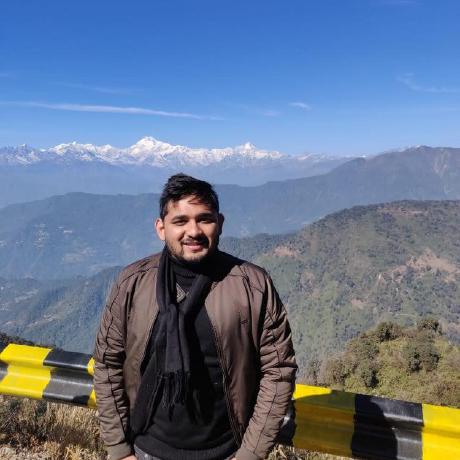
scraper
@pranjaldotdev
An interactive, runnable TypeScript val by pranjaldotdev
Script
const NEWSLETTER_URL = "https://bytes.dev/archives";
function normalizeURL(url: string) {
return url.startsWith("http://") || url.startsWith("https://")
? url
: "http://" + url;
async function fetchText(url: string, options?: any) {
const response = await fetch(normalizeURL(url), {
redirect: "follow",
...(options || {}),
return response.text();
provideBlobToHtml
@postpostscript
An interactive, runnable TypeScript val by postpostscript
Script
export { provideBlob } from "https://esm.town/v/postpostscript/provideBlob";
export function provideBlobToHtml(jsPromise: RawHTML, placeholder?: unknown) {
const id = "provideBlobHtml-" + Math.random().toString().slice(3);
return html`
<div id="${id}">
${placeholder}
<script>
const id = "${id}";
${jsPromise}
.then(blob => blob.text())