Search
eval
@maxm
Eval web demo Security Caveats This code runs in a Worker with { permissions: { write: false, read: false, net: false } } . This is likely very safe, but if you enable network access keep in mind that users might generate junk network traffic or attempt to make infinite loops. If sandboxed code knows the name of one of your private vals it will be able to import the code with import "https://esm.town/v/maxm/private" . If you enabled write: true in the Worker, the unix socket that Deno uses to communicate with the host can be deleted and intercepted. This might mean that evaluated code can steal the socket and read the next request. You should not use this to evaluate code that should not be read by a previous evaluation. All code is running on the same process and you are not protected from exotic attacks like speculative execution. Overview You can use this library to evaluate code: import { evalCode } from "https://esm.town/maxm/eval"
console.log(await evalCode("export const foo = 1")) // => 1 You can use this library with https://www.val.town/v/maxm/transformEvalCode to return the last value without needing to export it. This is how the /eval api endpoint used to work and makes the library preform similarly to a repl. import { evalCode } from "https://esm.town/maxm/eval"
import { transform } from "https://esm.town/maxm/transformEvalCode"
console.log(await evalCode(transform("1+1"))) // => 2 Here's an example UI application that demonstrates how you can string this all together: https://maxm-evalui.web.val.run/ (source: https://www.val.town/v/maxm/evalUI) Security Model Code is evaluated using a dynamic import within a Worker. await import(`data:text/tsx,${encodeURIComponent(e.data)}`); Running the code withing a Worker prevents access to GlobalThis and window from leaking between evals. Similarly, access to Deno.env is prevented and evaluations will see errors when trying to access any environment variables. TODO: what else?
Script
# Eval
[web demo](https://maxm-evalui.web.val.run/)
## Security Caveats
- This code runs in a Worker with `{ permissions: { write: false, read: false, net: false } }`. This is likely very safe, but if you enable network access keep in mind that users might generate junk network traffic or attempt to make infinite loops.
- If sandboxed code knows the name of one of your private vals it will be able to import the code with `import "https://esm.town/v/maxm/private"`.
- If you enabled `write: true` in the Worker, the unix socket that Deno uses to communicate with the host can be deleted and intercepted. This might mean that evaluated code can steal the socket and read the next request. You should not use this to evaluate code that should not be read by a previous evaluation.
export async function evalCode(
code: string,
): Promise<{ error: { message: string; name: string; stack: string } } | any> {
const workerCode = `
wormhole
@git
An interactive, runnable TypeScript val by git
HTTP
export default async (request: Request): Promise<Response> => {
return new Response(
/* html */ `<html>
<head>
<script
defer
type="module"
src="https://www.unpkg.com/@wormhole-foundation/wormhole-connect/dist/main.js">
</script>
<link rel="https://www.unpkg.com/@wormhole-foundation/wormhole-connect/dist/main.css" />
tanLadybug
@camajudson
An interactive, runnable TypeScript val by camajudson
HTTP
export const handleDiscordInteraction = async (req: Request) => {
if (req.method === "GET") return new Response("GET Method Not Allowed", { status: 405 });
const body = await req.json();
const verified = await verify_discord_signature(
Deno.env.get("discordPublicKey"),
JSON.stringify(body),
req.headers.get("X-Signature-Ed25519"),
req.headers.get("X-Signature-Timestamp"),
// Get the user calling this slash command
const userId = await body.member?.user?.id;
valle_tmp_92751004685681985320195796915739
@janpaul123
// Response with "Hello World" on every HTTP request
HTTP
// Response with "Hello World" on every HTTP request
export default async function(req: Request): Promise<Response> {
return new Response("Hello World", { headers: { "Content-Type": "text/plain" } });
valle_tmp_7899985259251199683019872792655
@janpaul123
// This val responds to HTTP requests with "Hello world"
HTTP
// This val responds to HTTP requests with "Hello world"
export default async function main(req: Request): Promise<Response> {
return new Response("Hello world");
md
@ije
md A Markdown renderer written in Zig & C, compiled to WebAssymbly for val.town - https://github.com/ije/md4w import { render } from "https://esm.town/v/ije/md"
render("Stay _foolish_, stay **hungry**!") // -> <p>Stay <em>foolish</em>, stay <strong>hungry</strong>!</p>
Script
### md
A **Markdown** renderer written in Zig & C, compiled to **WebAssymbly** for val.town - https://github.com/ije/md4w
```js
import { render } from "https://esm.town/v/ije/md"
render("Stay _foolish_, stay **hungry**!") // -> <p>Stay <em>foolish</em>, stay <strong>hungry</strong>!</p>
import { init, mdToHtml } from "https://esm.sh/md4w@0.2.4";
await init("small");
export function render(html: string) {
return mdToHtml(html);
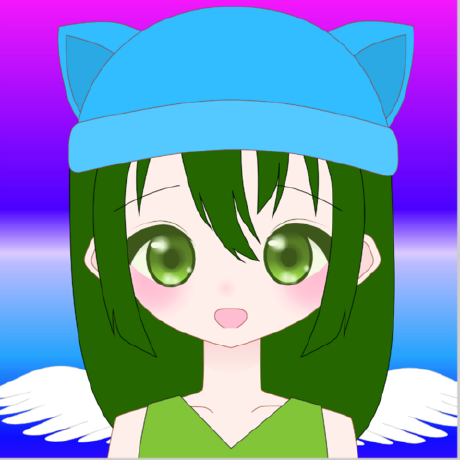
email_testing
@bitbloxhub
An interactive, runnable TypeScript val by bitbloxhub
Script
export const email_testing = console.email({
html: `<link rel="preconnect" href="https://fonts.googleapis.com">
<link rel="preconnect" href="https://fonts.gstatic.com" crossorigin>
<link href="https://fonts.googleapis.com/css2?family=Pacifico&family=Roboto&display=swap" rel="stylesheet"><h1 style="font-family: 'Roboto', sans-serif;">testing 1 2 3</h1>`,

buildHtml
@liamdanielduffy
An interactive, runnable TypeScript val by liamdanielduffy
Script
export function buildHtml(args: {
scripts?: string[];
styles?: string;
body?: string;
}): string {
return `
<!DOCTYPE html>
<html>
<head>
${args.scripts}

handlePost
@cp1
An interactive, runnable TypeScript val by cp1
Script
import { fetch } from "https://esm.town/v/std/fetch";
export const handlePost = async (req: express.Request, res: express.Response) => {
const { url = "http://example.com", method = "GET", headers = {} } = req.body;
const resp = await fetch(url, {
redirect: "follow",
const text = await resp.text();
res.send(text);
est
@hello_mikkie
An interactive, runnable TypeScript val by hello_mikkie
HTTP
export const htmlExample = () =>
new Response("<h1>Hello, world</h1>", {
headers: {
"Content-Type": "text/html",
handleDiscordInteraction
@jamisonl
Bot for Cama discord server. To initialize new slash commands, you have to run a separate bit of code. This is for modifying their functionality
HTTP
Bot for Cama discord server. To initialize new slash commands, you have to run a separate bit of code. This is for modifying their functionality
let bank = await blob.getJSON("bank");
let bets = await blob.getJSON("bets");
const starting_amount = 5;
if (!bank) {
bank = {};
await blob.setJSON("bank", bank);
if (!bets) {
bets = [];
await blob.setJSON("bets", bets);
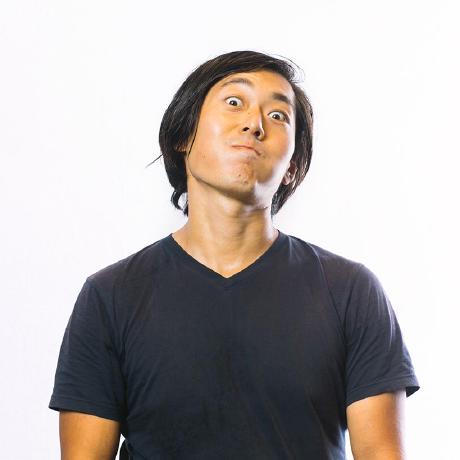
perlinNoiseFabricColor
@yawnxyz
Full credit goes to @yuruyurau: https://twitter.com/yuruyurau/status/1830677030259839352
HTTP
Full credit goes to @yuruyurau: https://twitter.com/yuruyurau/status/1830677030259839352
export default async function (req: Request): Promise<Response> {
const html = `<!DOCTYPE html>
<html>
<head>
<title>Colorful Triangle Pattern</title>
<!-- Load the Processing.js library -->
<script src="https://cdnjs.cloudflare.com/ajax/libs/processing.js/1.6.0/processing.min.js"></script>
</head>
<body>
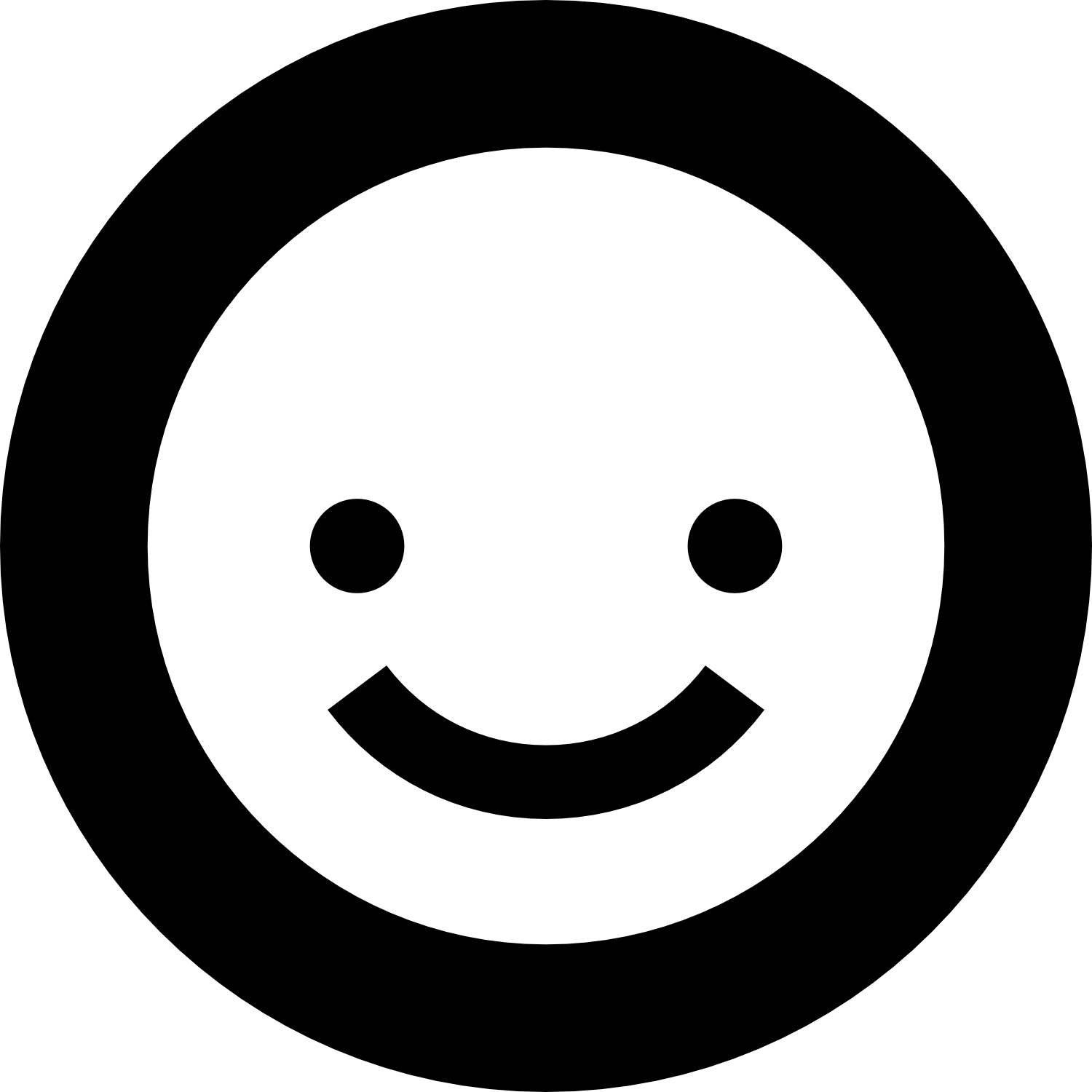
valForm
@rodrigotello
An interactive, runnable TypeScript val by rodrigotello
Script
export function valForm(functionIWantIntoAForm) {
let inputFields = "";
for (let i = 0; i < functionIWantIntoAForm.length; i++) {
inputFields += `<input type="text" id="input${i}" name="input${i}"><br>`;
const formHTML = `
<form id="myForm">
${inputFields}
<input type="submit" value="Submit">
</form>
<p id="result"></p>
aqi
@freyrva
An interactive, runnable TypeScript val by freyrva
Script
export let aqi = async () => {
let pm25 = (
await fetchJSON(
"https://api.openaq.org/v2/latest?" +
new URLSearchParams({
limit: "10",
page: "1",
location: "San Francisco",
offset: "0",
sort: "desc",
mockdevices
@juliendorra
An interactive, runnable TypeScript val by juliendorra
Script
export let mockdevices = (user) => {
// use user name to select devices
let devices = [];
let id = 0;
for (let letter of user) {
// add a device if charcode is even
if (letter.charCodeAt() % 2 === 0) {
// pick type
let type;
if (letter.charCodeAt() % 3 === 0) {