Search
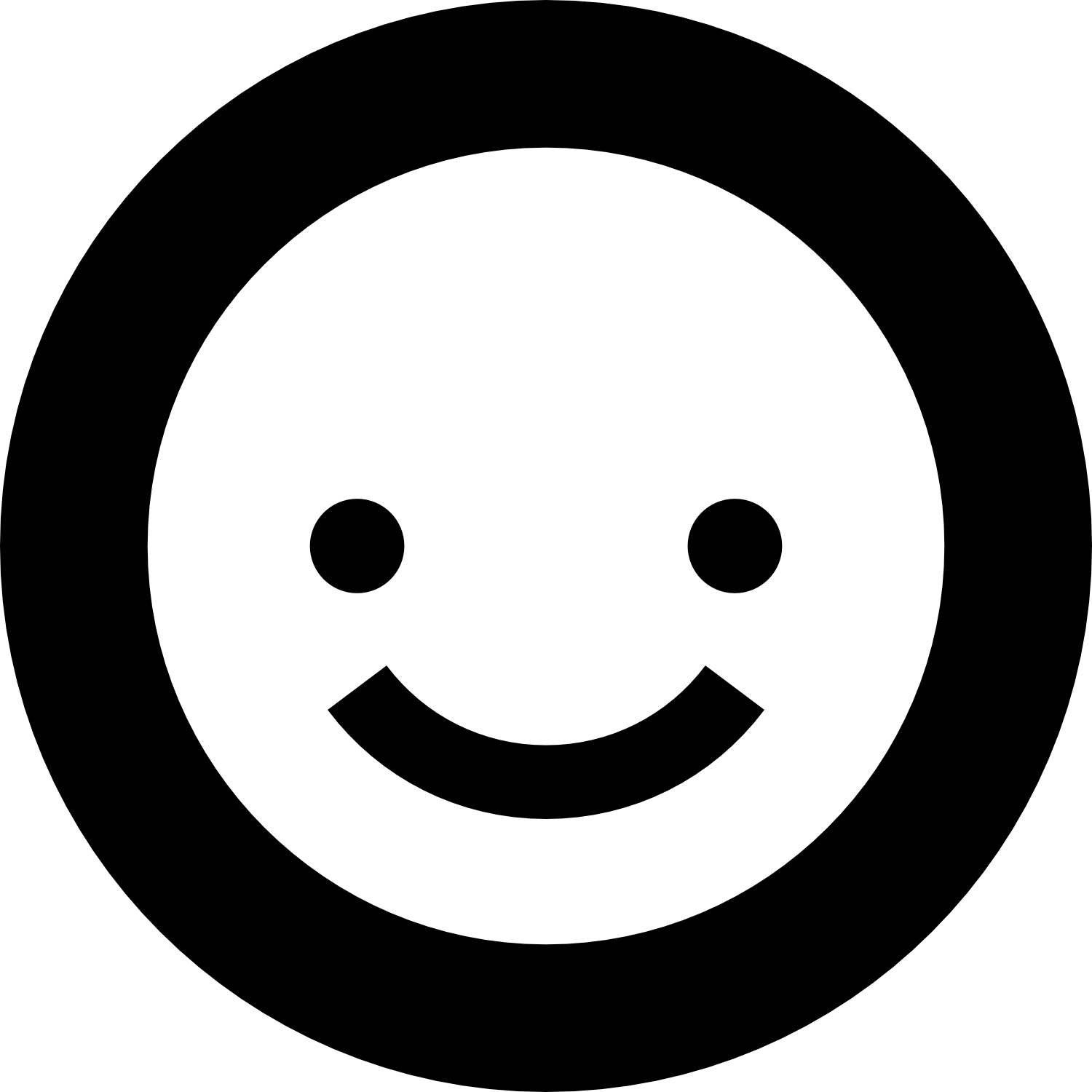
valForm
@rodrigotello
An interactive, runnable TypeScript val by rodrigotello
Script
export function valForm(functionIWantIntoAForm) {
let inputFields = "";
for (let i = 0; i < functionIWantIntoAForm.length; i++) {
inputFields += `<input type="text" id="input${i}" name="input${i}"><br>`;
const formHTML = `
<form id="myForm">
${inputFields}
<input type="submit" value="Submit">
</form>
<p id="result"></p>
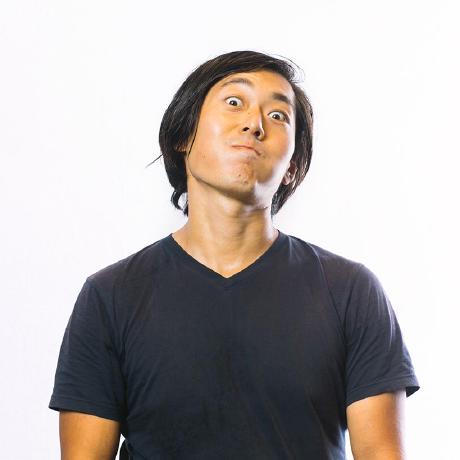
perlinNoiseFabricColor
@yawnxyz
Full credit goes to @yuruyurau: https://twitter.com/yuruyurau/status/1830677030259839352
HTTP
Full credit goes to @yuruyurau: https://twitter.com/yuruyurau/status/1830677030259839352
export default async function (req: Request): Promise<Response> {
const html = `<!DOCTYPE html>
<html>
<head>
<title>Colorful Triangle Pattern</title>
<!-- Load the Processing.js library -->
<script src="https://cdnjs.cloudflare.com/ajax/libs/processing.js/1.6.0/processing.min.js"></script>
</head>
<body>
mockdevices
@juliendorra
An interactive, runnable TypeScript val by juliendorra
Script
export let mockdevices = (user) => {
// use user name to select devices
let devices = [];
let id = 0;
for (let letter of user) {
// add a device if charcode is even
if (letter.charCodeAt() % 2 === 0) {
// pick type
let type;
if (letter.charCodeAt() % 3 === 0) {
testing
@temptemp
An interactive, runnable TypeScript val by temptemp
HTTP
export default async function (req: Request): Promise<Response> {
return Response.json({ ok: true })
weatherBot
@jdan
An interactive, runnable TypeScript val by jdan
Script
const openai = new OpenAI();
const toolbox = {
"latLngOfCity": {
openAiTool: {
type: "function",
function: {
name: "latLngOfCity",
description: "Get the latitude and longitude of a city",
parameters: {
type: "object",
FigmaFrameToHTML
@vez
// Forked from @rodrigotello.FigmaFrameToHTML
Script
import { FigmaFrameObjectToHTML } from "https://esm.town/v/rodrigotello/FigmaFrameObjectToHTML?v=6";
export async function FigmaFrameToHTML(
req: express.Request,
res: express.Response,
return res.send(
FigmaFrameObjectToHTML(
(await figmaFramesFromURL(
"https://www.figma.com/file/kljuHD3J7yYMJxXUX9zY4S/valtowntest",
))[0],
// Forked from @rodrigotello.FigmaFrameToHTML
AddLink
@iamseeley
@jsxImportSource https://esm.sh/hono@latest/jsx
Script
/** @jsxImportSource https://esm.sh/hono@latest/jsx **/
export default function AddLink({ username }) {
return (
<form action="/links" method="post" id="new-link" hx-push-url={`/edit-profile/${username}`} className="space-y-4">
<input
type="text"
name="label"
placeholder="Link Label"
className="w-full px-4 py-2 border rounded-md focus:outline-none focus:ring-2 focus:ring-blue-500"
<input
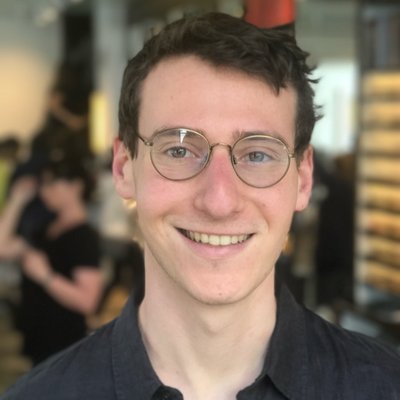
tell2
@stevekrouse
// Store messages via SQLite
Script
let { messages2 } = await import("https://esm.town/v/stevekrouse/messages2");
// Store messages via SQLite
// (stores at @me.messages2 in the current version)
// like `https://www.val.town/@stevekrouse.tell` but cooler!
export const tell2 = async (msg) => {
const { DB } = await import("https://deno.land/x/sqlite/mod.ts");
const db = new DB();
// Get existing messages (if any)
if (messages2 !== undefined) {
db.deserialize(messages2);

friendOfAFriend
@lukas
An interactive, runnable TypeScript val by lukas
Script
type GetFeedSkeleton = {
feed: string;
limit?: number;
cursor?: string;
type SkeletonFeedPostReason = SkeletonReasonRepost;
// format: at-uri
type SkeletonReasonRepost = {
repost: string;
type SkeletonFeedPost = {
post: string;
forbetEventsScrapper
@nmsilva
An interactive, runnable TypeScript val by nmsilva
Script
const forbetEventsScrapper = (html: string, baseUrl: string) => {
const $ = load(html);
const events = [];
$("td.contentmiddle > div > div.schema .rcnt").each((i, el) => {
const bet = $(el).text();
const id = $(el).find(".stcn .nofav.fav_icon").attr("id");
const link = $(el).find(".tnms a").attr("href");
const homeTeam = $(el).find(".tnms .homeTeam").text();
const awayTeam = $(el).find(".tnms .awayTeam").text();
const date = $(el).find(".tnms .date_bah").text();
a
@hoa
An interactive, runnable TypeScript val by hoa
Script
let getEpicTvProduct = async (url) => {
const cheerio = await import("npm:cheerio@1.0.0-rc.12");
const html = await (await fetch(url)).text();
const $ = cheerio.load(html);
console.log("how it run?");
return {
name: $(".page-title").text().trim(),
discount: $(".sale-value").text().trim(),
price: $("[data-price-type=\"finalPrice\"]").text().trim(),
oldPrice: $("[data-price-type=\"oldPrice\"]:first").text().trim(),
moccasinRabbit
@flxa
// View at https://andreterron-htmlExample.web.val.run?name=Andre
HTTP
// View at https://andreterron-htmlExample.web.val.run?name=Andre
export default async function(req: Request): Promise<Response> {
const query = new URL(req.url).searchParams;
// Read name from the querystring or body. Defaults to "you" if not present.
const name = query.get("name") || (await req.json().catch(() => ({}))).name || "you";
// Returns the HTML response
return new Response(
<style>
h1 {
text-decoration-line: line-through;
val_ha4AaveNX7
@dhvanil
An interactive, runnable TypeScript val by dhvanil
HTTP
export default async function handler(req) {
try {
const result = await (async () => {
function isPrime(num) {
if (num <= 1) return false;
for (let i = 2; i <= Math.sqrt(num); i++) {
if (num % i === 0) return false;
return true;
let lowestPrime = 100;
while (!isPrime(lowestPrime)) {
val_7kR931x6hU
@dhvanil
An interactive, runnable TypeScript val by dhvanil
HTTP
export async function val_7kR931x6hU(req) {
try {
// Execute the code directly and capture its result
const result = await (async () => {
const isValidBalancedParentheses = (str) => {
const stack = [];
for (const char of str) {
if (char === '(') {
stack.push(char);
} else if (char === ')') {