Search
mergician_demo
@jhildenbiddle
// ES module shown. CommonJS module also available.
Script
// ES module shown. CommonJS module also available.
import { mergician } from "https://cdn.jsdelivr.net/npm/mergician@2";
const obj1 = { a: [1, 1], b: { c: 1, d: 1 } };
const clonedObj = mergician({}, obj1);
// Results
console.log(clonedObj); // { a: [1, 1], b: { c: 1, d: 1 } }
console.log(clonedObj === obj1); // false
console.log(clonedObj.a === obj1.a); // false
console.log(clonedObj.b === obj1.b); // false
bilibili_methods
@rebelpotato
Methods for accessing the bilibili api with a cookie.
Script
Methods for accessing the bilibili api with a cookie.
function getBilibiliHeaders(cookie) {
return new Headers({
"connection": "keep-alive",
"User-Agent":
"Mozilla/5.0 (Windows NT 10.0; Win64; x64) AppleWebKit/537.36 (KHTML, like Gecko) Chrome/85.0.4183.121 Safari/537.36",
"referer": "https://www.bilibili.com/",
"Cookie": "SESSDATA=" + cookie.sessdata + "; bili_jct=" + cookie.bilijct + "; DedeUserID=" + cookie.dedeuserid,
function postBilibiliHeaders(cookie) {
return new Headers({
grotesqueAquamarineShrew
@slowchaz
An interactive, runnable TypeScript val by slowchaz
HTTP
const KEY = "views";
interface StoredData {
pageViews: number;
export default async function handler(req: Request): Promise<Response> {
const headers = new Headers({
"Access-Control-Allow-Origin": "*",
"Access-Control-Allow-Methods": "GET, OPTIONS",
"Access-Control-Allow-Headers": "Content-Type, Authorization",
if (req.method === "OPTIONS") {
return new Response(null, { headers });
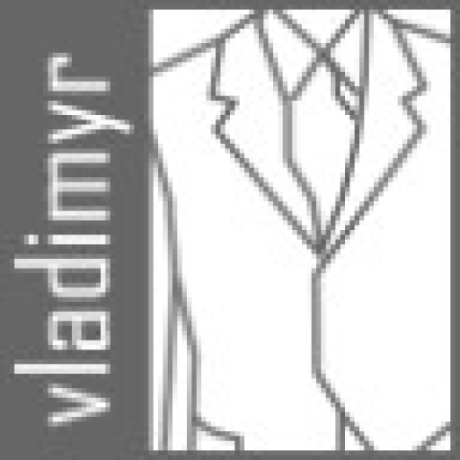
appendFragment_example
@vladimyr
An interactive, runnable TypeScript val by vladimyr
HTTP
const { author, name } = extractValInfo(import.meta.url);
const html = `<html>
<head>
<title>test</title>
</head>
<body>
<p><code>@vladimyr/appendFragment</code> test</p>
</body>
</html>
`.trim();
googletrends
@dglazkov
An interactive, runnable TypeScript val by dglazkov
HTTP
NodeDescriberResult,
service,
} from "https://esm.town/v/dglazkov/servicefactory";
parseXml,
XmlCdata,
XmlComment,
XmlDocument,
XmlElement,
XmlText,
} from "npm:@rgrove/parse-xml";
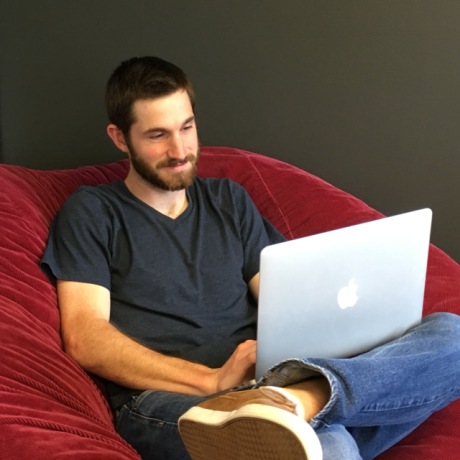
publicTimePlayedLeaderboard
@tr3ntg
An interactive, runnable TypeScript val by tr3ntg
Express (deprecated)
import { timePlayedLeaderboardHTML } from "https://esm.town/v/tr3ntg/timePlayedLeaderboardHTML";
export async function publicTimePlayedLeaderboard(
req: express.Request,
res: express.Response,
let html = await timePlayedLeaderboardHTML();
res.send(html);
tidbytCircle
@andreterron
An interactive, runnable TypeScript val by andreterron
Script
export async function tidbytCircle({ size = 7, fill, border }: {
size?: number;
fill?: number;
border?: number;
const { default: Jimp } = await import("npm:jimp@0");
const img = await new Jimp(size, size);
for (const { x, y, idx, image } of img.scanIterator(0, 0, size, size)) {
const dx = x - size / 2 + 0.5;
const dy = y - size / 2 + 0.5;
const d = Math.sqrt(dx * dx + dy * dy);
labLoginGetUsers
@svenlaa
// export default async function(req: Request): Promise<Response> {
HTTP
export default async function(req: Request): Promise<Response> {
const res = await fetch("https://api.svenlaa.com/logiverse/logs");
const data = await res.json();
const ts = new Date().getTime();
const d = new Date(ts - 3600000);
const date = d.toISOString().split("T")[0];
const time = d.toTimeString().split(" ")[0];
var d_string = `${date} ${time}`;
data.push([
"⚠️ notice: If you see me, you are using the val.town endpoints. please use the new cloudflare-based endpoints. You can find them on https://svenlaa.com/playground/loggo/logiverse.js",
viewMailbox
@nimalu
// https://api.val.town/express/@nimalu.viewMailbox
Script
// https://api.val.town/express/@nimalu.viewMailbox
export const viewMailbox = (req, res) => {
const messages = mailbox
.map(
({ message, sender }) =>
`<li><span class="font-semibold text-gray-900">${sender}: </span>${message}</li>`
.join("");
res.set("Content-Type", "text/html");
res.send(`
<!doctype html>
redditMatches
@bnorick
An interactive, runnable TypeScript val by bnorick
Script
export let redditMatches = async ({ username, filters, current_state, options }) => {
let matches = {};
let new_state = current_state ? current_state : {};
current_state = current_state || {};
for (const subreddit in filters) {
console.log(`processing subreddit ${subreddit}`);
let subreddit_filters = filters[subreddit];
let state = current_state[subreddit] ? current_state[subreddit] : {};
if (state.disabled) {
if (!options.force) {
TextTransformer
@chet
An interactive, runnable TypeScript val by chet
HTTP
const title = "Text Transformer";
const html = (url: string) => `
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<meta name="viewport" content="width=device-width, initial-scale=1.0">
<title>${title}</title>
<style>
body {
val_HhH0AK0vuP
@dhvanil
An interactive, runnable TypeScript val by dhvanil
HTTP
export default async function handler(req) {
try {
const result = await (async () => {
function isPrime(num) {
if (num <= 1) return false;
for (let i = 2; i <= Math.sqrt(num); i++) {
if (num % i === 0) return false;
return true;
let lowestPrime = 101;
while (!isPrime(lowestPrime)) {

duckdbExample
@neverstew
An interactive, runnable TypeScript val by neverstew
Script
export let duckdbExample = (async () => {
async function createWorker(url: string) {
const workerScript = await fetch(url);
const workerURL = URL.createObjectURL(await workerScript.blob());
return new Worker(workerURL, { type: "module" });
const duckdb = await import(
"https://cdn.jsdelivr.net/npm/@duckdb/duckdb-wasm@1.17.0/+esm"
const bundles = duckdb.getJsDelivrBundles();
const bundle = await duckdb.selectBundle(bundles);
const logger = new duckdb.ConsoleLogger();
kyselyVtTypes
@nbbaier
Kysely type generator for @std/sqlite Usage Fork to your account. Update allowedTables to expose any tables you'd like to import the schema of. This will make their schemas public! Add import type { DB } from "https://yourusername-kyselyVtTypes.web.val.run/?tables=tables,you,need" to your program.
See that QueryParams` type at the top? Add those to your URL to set more options. Demo See @easrng/kyselyVtDemo.
HTTP
# Kysely type generator for @std/sqlite
## Usage
- Fork to your account.
- Update allowedTables to expose any tables you'd like to import the schema of.
**This will make their schemas public!**
- Add `import type { DB } from "https://yourusername-kyselyVtTypes.web.val.run/?tables=tables,you,need" to your program.
type QueryParams = {
camelCase?: "true" | "false";
excludePattern?: string;
includePattern?: string;
valle_tmp_06883828762455286767916178260256
@janpaul123
// This val will simply respond with "Hello world" to all incoming requests
HTTP
// This val will simply respond with "Hello world" to all incoming requests
export default async function(req: Request): Promise<Response> {
return new Response("Hello world");