Search
SermonGPTAPI
@mjweaver01
An interactive, runnable TypeScript val by mjweaver01
HTTP
export default async function server(request: Request): Promise<Response> {
const { blob } = await import("https://esm.town/v/std/blob");
if (request.method === "POST" && new URL(request.url).pathname === "/stream") {
const { OpenAI } = await import("https://esm.town/v/std/openai");
const openai = new OpenAI();
const { question } = await request.json();
const season = getSeason(currentDate);
const stream = await openai.chat.completions.create({
messages: [
headers: { "Content-Type": "application/json" },
function getSeason(date: Date): string {
const month = date.getMonth();
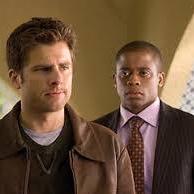
dateme_browse
@vawogbemi
@jsxImportSource npm:hono@3/jsx
Script
import { zip } from "npm:lodash-es";
function absoluteURL(url) {
if (url.startsWith("http://") || url.startsWith("https://"))
let linkClass = "text-sky-600 hover:text-sky-500";
function httpsIfy(url: string) {
if (!url.startsWith("http://") && !url.startsWith("https://")) {
return url;
function renderCell(header, row) {
let data = row[header];
return data;
export default async function Browse(c) {
const url = new URL(c.req.url);
sass
@jamessw
An interactive, runnable TypeScript val by jamessw
Script
interface SassArgs {
input: string;
export async function sass(args: SassArgs) {
const input = args.input || "a {color: #663399}";
const sass = await import("https://jspm.dev/sass");
valentine
@hinduhops
Too cheap to pay for a react server c: luckily val is based and doesn't need a real deployment
HTTP
"s-s-s-sen...senpai???",
function App() {
const [noClicks, setNoClicks] = useState(0);
</div>
function client() {
createRoot(document.getElementById("root")).render(<App />);
if (typeof document !== "undefined") { client(); }
export default async function server(request: Request): Promise<Response> {
return new Response(
homeControlDevice
@Vikramhaking
@jsxImportSource https://esm.sh/react@18.2.0
HTTP
temperature?: number;
function HomeControlApp() {
const [devices, setDevices] = useState<DeviceState[]>([
</div>
function client() {
createRoot(document.getElementById("root")).render(<HomeControlApp />);
if (typeof document !== "undefined") { client(); }
export default async function server(request: Request): Promise<Response> {
return new Response(`
FanficSearcherWebsite
@willthereader
// first stable version is 9 and the link is https://www.val.town/v/willthereader/FanficSearcherWebsite?v=9
HTTP
// first stable version is 9 and the link is https://www.val.town/v/willthereader/FanficSearcherWebsite?v=9
function validateConfig(): { appId: string; apiKey: string } {
const appId = Deno.env.get("ALGOLIA_APP_ID_fanficSearcher");
return { appId, apiKey };
function generateHtml(appId: string, apiKey: string): string {
console.log("Generating HTML with App ID:", appId, "and API Key:", apiKey ? "exists" : "missing");
templates: {
item: function(hit) {
console.log('Hit:', hit); // Add this line
</html>
function handleError(error: Error): Response {
console.error("Server error:", error);
return new Response(`Error: ${error.message}`, { status: 500 });
async function handleRequest(request: Request): Promise<Response> {
console.log("Received request:", request.url);
labUploadDelete
@todepond
An interactive, runnable TypeScript val by todepond
HTTP
import { blob } from "https://esm.town/v/std/blob";
import { sqlite } from "https://esm.town/v/stevekrouse/sqlite";
export default async function(req: Request): Promise<Response> {
let json;
try {
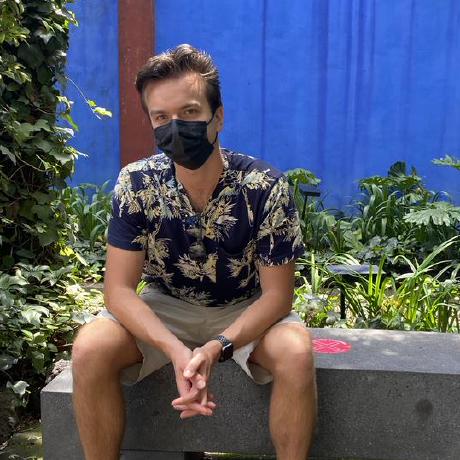
reactSSRExample
@jonbo
An interactive, runnable TypeScript val by jonbo
Express (deprecated)
const ReactDOMServer = await import("npm:react-dom/server");
function BusTime(props) {
return React.createElement("span", null, "southbound in 5 minutes");
function BikeLocation(props) {
return React.createElement(
`${props.name} has ${props.bikesAvailable} bikes, ${props.docksAvailable} docks`,
function BikesList(props) {
const bikeEls = props.items.map((item, index) => {
sleeper
@jamiedubs
// a very slow and sleepy function
HTTP
// a very slow and sleepy function
// pass it ?sleep=33 to make it sleep for that amount of sleep
export default async function (req: Request): Promise<Response> {
const url = new URL(req.url);
const sleepQueryParam = url.searchParams.get('sleep');
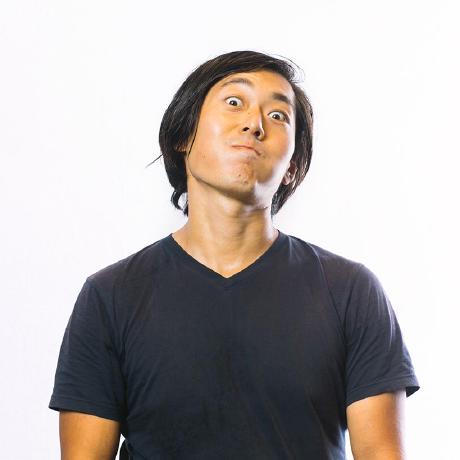
perlinNoiseFabricMouse
@yawnxyz
Full credit goes to @yuruyurau: https://twitter.com/yuruyurau/status/1830677030259839352
HTTP
export default async function (req: Request): Promise<Response> {
const html = `<!DOCTYPE html>
<html>
float s = 4;
float w = 400;
// Function to calculate the vertex positions
float[] a(float x, float y) {
float k = mouseX - x;
emailRandomJoke
@gurshansidhu
// Fetches a random joke.
Cron
import { email } from "https://esm.town/v/std/email?v=9";
// Fetches a random joke.
async function fetchRandomJoke() {
const response = await fetch(
"https://official-joke-api.appspot.com/random_joke",
valTownAnalytics
@maxm
Val Town Analytics WIP!
HTTP
ORDER BY bucket`,
args: [intervalMinutes, startString, endString, val],
async function handler(req: Request): Promise<Response> {
const url = new URL(req.url);
if (req.method === "GET" && url.pathname === "/event") {
snake
@jxnblk
// WIP turn-based snake game built in Val Town
HTTP
gameover?: boolean;
function App(props: AppState) {
const {
</div>
function GameOver() {
return (
return "?" + toParams(next);
function getRelativeDirection(a: V2, b: V2): string {
if (matchV2(a, b)) return "straight";
return "turn";
function Controls(props: AppState) {
const up = getLink(props, UP);
[RIGHT as any]: "▶",
function Button({ href, direction, ...props }: { href: string; direction: V2 }) {
const arrow = arrows[direction as any];
const ROBOTS = "User-agent: *\nDisallow: /";
export default async function(req: Request): Promise<Response> {
const url = new URL(req.url);
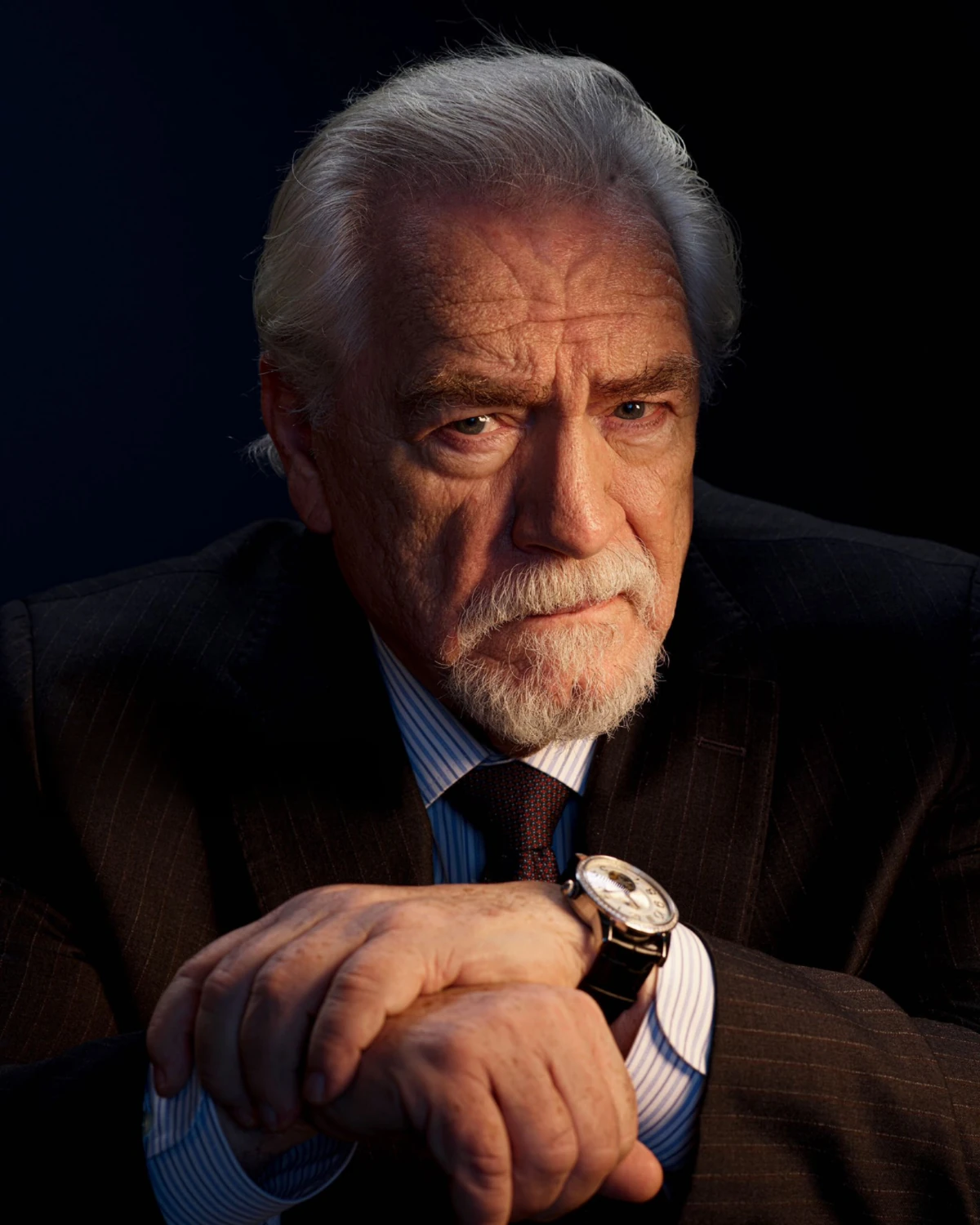
minizod
@zackoverflow
minizod Tiny Zod implementation. Why Zod is a dense library, and its module structure (or lack thereof) makes it difficult for bundlers to tree-shake unused modules . Additionally, using Zod in vals requires the await import syntax which means having to wrap every schema in a Promise and awaiting it. This is extremely annoying. So this is a lil-tiny-smol Zod meant for use in vals. A noteworthy use-case is using minizod to generate tyep-safe API calls to run vals outside of Val Town (such as client-side). Type-safe API call example We can use minizod to create type safe HTTP handlers and generate the corresponding code to call them using Val Town's API, all in a type-safe manner. First, create a schema for a function. The following example defines a schema for a function that takes a { name: string } parameter and returns a Promise<{ text: string }> . const minizodExampleSchema = () =>
@zackoverflow.minizod().chain((z) =>
z
.func()
.args(z.tuple().item(z.object({ name: z.string() })))
.ret(z.promise().return(z.object({ text: z.string() })))
); With a function schema, you can then create an implementation and export it as a val: const minizodExample = @me.minizodExampleSchema().impl(async (
{ name },
) => ({ text: `Hello, ${name}!` })).json() In the above example, we call .impl() on a function schema and pass in a closure which implements the actual body of the function. Here, we simply return a greeting to the name passed in. We can call this val, and it will automatically parse and validate the args we give it: // Errors at compile time and runtime for us!
const response = @me.minizodExample({ name: 420 }) Alternatively, we can use the .json() function to use it as a JSON HTTP handler: const minizodExample = @me.minizodExampleSchema().impl(async (
{ name },
) => ({ text: `Hello, ${name}!` })).json() // <-- this part We can now call minizodExample through Val Town's API. Since we defined a schema for it, we know exactly the types of its arguments and return, which means we can generate type-safe code to call the API: let generatedApiCode =
@zackoverflow.minizodFunctionGenerateTypescript(
// put your username here
"zackoverflow",
"minizodExample",
// put your auth token here
"my auth token",
@me.minizodExampleSchema(),
); This generates the following the code: export const fetchMinizodExample = async (
...args: [{ name: string }]
): Promise<Awaited<Promise<{ text: string }>>> =>
await fetch(`https://api.val.town/v1/run/zackoverflow.minizodExample`, {
method: "POST",
body: JSON.stringify({
args: [...args],
}),
headers: {
Authorization: "Bearer ksafajslfkjal;kjf;laksjl;fajsdf",
},
}).then((res) => res.json());
Script
First, create a schema for a function. The following example defines a schema for a function that takes a `{ name: string }` parameter and returns a `Promise<{ text: string }>`.
With a function schema, you can then create an implementation and export it as a val:
In the above example, we call `.impl()` on a function schema and pass in a closure which implements the actual body of the function. Here, we simply return a greeting to the name passed in.
export function minizod() {
| ZFunction<ZTuple, ZType>
type InferFunction<T> = T extends ZFunction<
type ZFunctionImpl<
minizodSchema: () => ZFunction<Args, Ret>;
type ZFunctionImplSync<
minizodSchema: () => ZFunction<Args, Ret>;
class ZFunction<
type: "function" = "function";
>(args: NewArgs): ZFunction<NewArgs, Ret> {
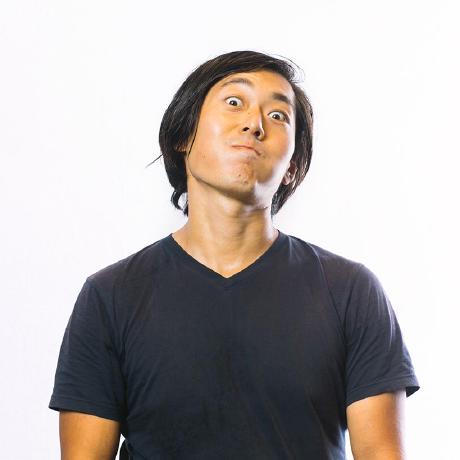
scribe
@yawnxyz
// Inlined content from ./middleware.ts
HTTP
duration?: number;
// Helper function to normalize transcription response
function normalizeTranscription(response: string | Transcription): Transcription {
console.log('Normalized transcription:', response);
// Fetch the size of the file using HTTP HEAD request
export async function fetchFileSize(url: string): Promise<number> {
const response = await fetch(url, { method: 'HEAD' });
// Transcribe a single chunk of audio data using Deno's ReadableStream
async function transcribeChunk(buffer: Uint8Array, params: TranscriptionParams): Promise<Transcription> {
console.log('Transcribing chunk with params:', {
content: string | File;
export async function transcribeAudio(input: AudioInput, params: TranscriptionParams): Promise<Transcription> {
console.log('Transcribing audio with params:', params);
return combinedResponse;
async function getTranscript(data) {
try {
// Based on threadg1rl's auth system
function useAuth(Alpine) {
Alpine.data('authApp', () => ({
console.error('[handleLogout] error:', error);
// Export the function properly
export const useAuthExport = `