Search
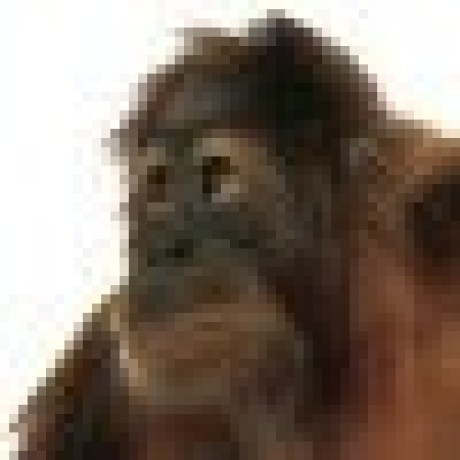
tinybase_example_client
@pomdtr
@jsxImportSource https://esm.sh/react
Script
/** @jsxImportSource https://esm.sh/react */
import ReactDom from "https://esm.sh/react-dom";
import { createStore } from "https://esm.sh/tinybase";
import { createRemotePersister } from "https://esm.sh/tinybase/persisters/persister-remote";
import { useValue } from "https://esm.sh/tinybase/ui-react";
// The store is automatically persisted on the remote server
</main>
const root = ReactDom.createRoot(document.body);
root.render(<App />);
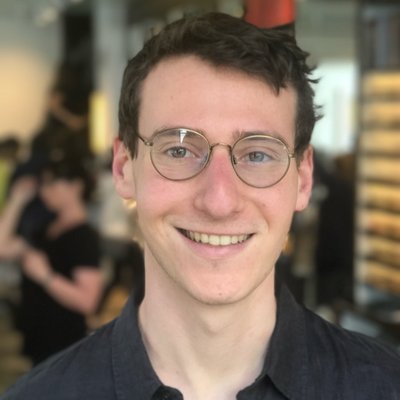
lucia_middleware_vanilla
@stevekrouse
Lucia Middleware Import users. Backed by Val Town SQLite. Demo: @stevekrouse/lucia_middleware_demo Initially built by @pomdtr.
HTTP
// It provides signup, login, and logout functionality with session management.
// The main tradeoff is the complexity of setting up Lucia, but it offers robust auth features.
/** @jsxImportSource https://esm.sh/react */
import { ValTownAdapter } from "https://esm.town/v/stevekrouse/lucia_adapter";
import { createUser, getUser, verifyPassword } from "https://esm.town/v/stevekrouse/lucia_sqlite";
import { Lucia, Session, User } from "npm:lucia@3.0.1";
import { renderToString } from "npm:react-dom/server";
import { CookieJar } from "https://deno.land/x/cookies/mod.ts";
const userTable = "user";
valreadmegenerator
@prashamtrivedi
@jsxImportSource https://esm.sh/react@18.2.0
HTTP
/** @jsxImportSource https://esm.sh/react@18.2.0 */
import { ValTown } from "https://esm.sh/@valtown/sdk";
import { createRoot } from "https://esm.sh/react-dom@18.2.0/client";
import React, { useRef, useState, useEffect } from "https://esm.sh/react@18.2.0";
function App() {
</div>
function client() {
createRoot(document.getElementById("root")).render(<App />);
if (typeof document !== "undefined") { client(); }
export default async function server(request: Request): Promise<Response> {
const openai = new OpenAI();
const valTownClient = new ValTown({
token: Deno.env.get("VALTOWN_TOKEN"),
try {
const valResponse = await valTownClient.alias.username.valName.retrieve(username, valName);
if (!valResponse.code) {
cancel() {
// Handle cancellation (e.g., client disconnection)
console.log('Stream cancelled by client');
return new Response(stream, {
rainyAquamarineDonkey
@arthrod
@jsxImportSource https://esm.sh/react
HTTP
/** @jsxImportSource https://esm.sh/react */
import React from "https://esm.sh/react";
import styled, { keyframes } from "https://esm.sh/styled-components";
</div>
function client() {
const root = document.getElementById("root");
if (root) {
import("https://esm.sh/react-dom/client").then(({ createRoot }) => {
createRoot(root).render(<App />);
if (typeof document !== "undefined") { client(); }
export default async function server(request: Request): Promise<Response> {
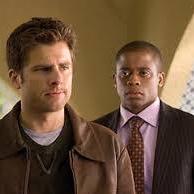
instantdbReactionsExample
@vawogbemi
@jsxImportSource https://esm.sh/react
HTTP
/** @jsxImportSource https://esm.sh/react */
import { init } from "https://esm.sh/@instantdb/react";
import React, { createRef, useRef } from "https://esm.sh/react";
import { createRoot } from "https://esm.sh/react-dom/client";
type EmojiName = keyof typeof emoji;
const elRefsRef = useRef<{
[k: string]: React.RefObject<HTMLDivElement>;
}>(refsInit);
return <InstantTopics />;
function client() {
createRoot(document.getElementById("root")!).render(<App />);
if (typeof document !== "undefined") {
client();
export default async function server(request: Request): Promise<Response> {
react_example_server
@nbbaier
// The server response includes a script referencing the client val
HTTP
// The server response includes a script referencing the client val
export const server = (req) =>
<head>
<title>React Example</title>
</head>
<main id="root"></main>
<script type="module" src="https://esm.town/v/nbbaier/react_client"></script/>
</body>
birthdayAlertsApp
@abidiqrar
@jsxImportSource https://esm.sh/react@18.2.0
HTTP
/** @jsxImportSource https://esm.sh/react@18.2.0 */
import React, { useState, useEffect } from "https://esm.sh/react@18.2.0";
import { createRoot } from "https://esm.sh/react-dom@18.2.0/client";
function App() {
</div>
function client() {
createRoot(document.getElementById("root")).render(<App />);
if (typeof document !== "undefined") { client(); }
export default async function server(request: Request): Promise<Response> {
responsiveMagentaBear
@Pushpam
@jsxImportSource https://esm.sh/react@18.2.0
Script
/** @jsxImportSource https://esm.sh/react@18.2.0 */
import React, { useState, useEffect } from "https://esm.sh/react@18.2.0";
import { createRoot } from "https://esm.sh/react-dom@18.2.0/client";
function RunningGame() {
</div>
function client() {
createRoot(document.getElementById("root")).render(<RunningGame />);
if (typeof document !== "undefined") { client(); }
export default async function server(request: Request): Promise<Response> {
routineTrackerApp
@ashryanio
Routine Tracker This is a little React component to make our 7yo's after school routine self-serve so she can be a bit more independent after school. To change the items in the list, modify the routineTasks array of objects: [
{name: "item", timed: false},
{name: "item 2", timed: true, duration: 2 * 60 }
{name: "item 3", timed: false, requiresParent: true}
] You can set the parent password on this line: const PARENT_PASSWORD_HASH = simpleHash("1234"); Todos Make the parent password modal touch friendly Track dates/times of list completions Show a tracker of how many completions this week
HTTP
# Routine Tracker
This is a little React component to make our 7yo's after school routine self-serve so she can be a bit more independent after school.
To change the items in the list, modify the `routineTasks` array of objects:
/** @jsxImportSource https://esm.sh/react@18.2.0 */
import confetti from "https://esm.sh/canvas-confetti@1.6.0";
import { createRoot } from "https://esm.sh/react-dom@18.2.0/client";
import React, { useCallback, useEffect, useRef, useState } from "https://esm.sh/react@18.2.0";
const routineTasks = {
</div>
function client() {
// Detect which user from query param in the script URL
if (typeof document !== "undefined") {
client();
async function server(request: Request): Promise<Response> {
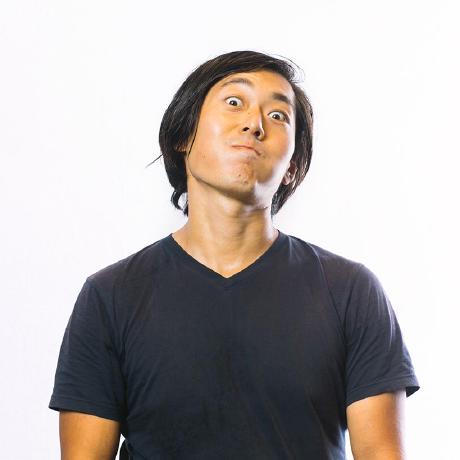
WobbleShapes
@yawnxyz
@jsxImportSource https://esm.sh/react
HTTP
/** @jsxImportSource https://esm.sh/react */
import React, { useState, useRef, useEffect } from "https://esm.sh/react";
import { createRoot } from "https://esm.sh/react-dom/client";
function PotteryPlate({ radius, color, wobbliness, wobbleFrequency, wobbleIrregularity, rotation }) {
</div>
function client() {
createRoot(document.getElementById("root")).render(<App />);
if (typeof document !== "undefined") { client(); }
async function server(request: Request): Promise<Response> {
substantialAquamarineFowl
@winderrobot
not
HTTP
/** @jsxImportSource https://esm.sh/react */
import React, { useState } from "https://esm.sh/react";
import { createRoot } from "https://esm.sh/react-dom/client";
function App() {
</div>
function client() {
createRoot(document.getElementById("root")).render(<App />);
if (typeof document !== "undefined") {
client();
export default async function server(request: Request): Promise<Response> {
routineTrackerApp
@jmt_es
Routine Tracker This is a little React component to make our 7yo's after school routine self-serve so she can be a bit more independent after school. To change the items in the list, modify the routineTasks array of objects: [
{name: "item", timed: false},
{name: "item 2", timed: true, duration: 2 * 60 }
{name: "item 3", timed: false, requiresParent: true}
] You can set the parent password on this line: const PARENT_PASSWORD_HASH = simpleHash("1234"); Todos Make the parent password modal touch friendly Track dates/times of list completions Show a tracker of how many completions this week
HTTP
# Routine Tracker
This is a little React component to make our 7yo's after school routine self-serve so she can be a bit more independent after school.
To change the items in the list, modify the `routineTasks` array of objects:
/** @jsxImportSource https://esm.sh/react */
import confetti from "https://esm.sh/canvas-confetti@1.6.0";
import React, { useEffect, useRef, useState } from "https://esm.sh/react";
import { createRoot } from "https://esm.sh/react-dom/client";
const taskIcons = {
</div>
function client() {
createRoot(document.getElementById("root")).render(<App />);
if (typeof document !== "undefined") { client(); }
async function server(request: Request): Promise<Response> {
falDemoApp
@d0ccc
@jsxImportSource https://esm.sh/react
HTTP
/** @jsxImportSource https://esm.sh/react */
import { createFalClient } from "https://esm.sh/@fal-ai/client";
import React, { useState } from "https://esm.sh/react";
import { createRoot } from "https://esm.sh/react-dom/client";
import { falProxyRequest } from "https://esm.town/v/stevekrouse/falProxyRequest";
const [numImages, setNumImages] = useState(1);
const generateImage = async (e?: React.FormEvent) => {
e?.preventDefault();
setImageUrls([]);
const fal = createFalClient({
proxyUrl: "/api/fal/proxy",
</div>
function client() {
createRoot(document.getElementById("root")).render(<App />);
if (typeof document !== "undefined") { client(); }
export default async function server(req: Request): Promise<Response> {
createMicrosoftOfficeOverview
@smail1
@jsxImportSource https://esm.sh/react@18.2.0
HTTP
/** @jsxImportSource https://esm.sh/react@18.2.0 */
import React from "https://esm.sh/react@18.2.0";
import { createRoot } from "https://esm.sh/react-dom@18.2.0/client";
function App() {
</div>
function client() {
createRoot(document.getElementById("root")).render(<App />);
if (typeof document !== "undefined") { client(); }
export default async function server(request: Request): Promise<Response> {
v3FanFicScraper
@willthereader
@jsxImportSource https://esm.sh/react
HTTP
/** @jsxImportSource https://esm.sh/react */
import React, { useState } from "https://esm.sh/react";
import { createRoot } from "https://esm.sh/react-dom/client";
console.log("Script started");
</div>
function client() {
console.log("Client function called");
const rootElement = document.getElementById("root");
if (typeof document !== "undefined") {
console.log("Document is defined, calling client function");
client();
} else {
console.log("Document is undefined, skipping client function");
async function server(request: Request): Promise<Response> {