Search
turquoisePiranha
@hmy
An interactive, runnable TypeScript val by hmy
HTTP
export default async function (req: Request): Promise<Response> {
return Response.json({ ok: true })
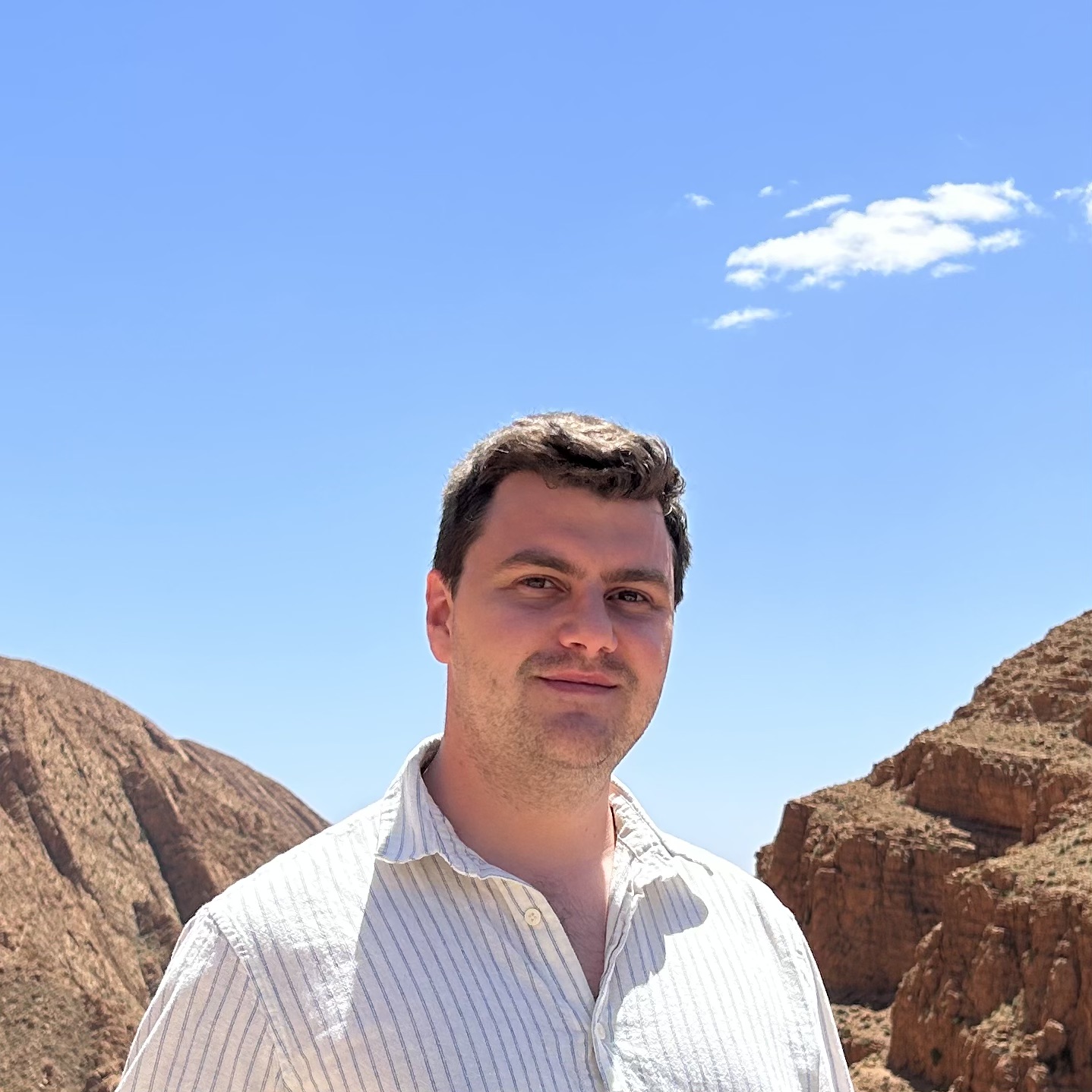
Resy_setNotify
@rlesser
An interactive, runnable TypeScript val by rlesser
Script
export const Resy_setNotify = async (params: {
authToken: string;
notifies: {
venue_id: number;
day: string;
time_preferred_start: string;
time_preferred_end: string;
num_seats: number;
service_type_id: number;
const url = "https://api.resy.com";
duckdbExample
@taras
DuckDB DuckDB works on Val Town, with only one small tweak! We're basically using DuckDB in the same way you'd use it with a browser - using the WASM package with its dependencies fetched from jsdelivr . The only trick is to create the worker ourselves rather than using duckdb.createWorker . DuckDB's built-in createWorker method doesn't specify a worker type, which causes type to default to classic , and Deno (our runtime) doesn't support classic workers. Note: A small check was added to make this compatible with local Deno. if (typeof Deno !== "undefined") {
return new Worker(url, { type: "module", deno: true });
}
HTTP
# DuckDB
[DuckDB](https://duckdb.org/) works on Val Town, with only one small tweak! We're basically using DuckDB in the same way you'd use it with a browser - using the WASM package with its dependencies fetched from [jsdelivr](https://www.jsdelivr.com/).
The only trick is to create the worker ourselves rather than using `duckdb.createWorker`. DuckDB's built-in createWorker method doesn't specify a worker type, which causes `type` to default to `classic`, and Deno (our runtime) doesn't support classic workers.
Note: A small check was added to make this compatible with local Deno.
if (typeof Deno !== "undefined") {
return new Worker(url, { type: "module", deno: true });
export let duckdbExample = await (async () => {
async function createWorker(url) {
// For Val Town / Deno environments
if (typeof Deno !== "undefined") {
val_6mHf5OkPYV
@dhvanil
An interactive, runnable TypeScript val by dhvanil
HTTP
export default async function handler(req) {
try {
const result = await (async () => {
function findLowestThreeDigitFibonacci() { let a = 0, b = 1; let lowestThreeDigit; while (b < 1000) { let temp = b; b = a + b; a = temp; } lowestThreeDigit = b; return lowestThreeDigit;}
findLowestThreeDigitFibonacci();
return Response.json({ success: true, result });
} catch (error) {
return Response.json({
success: false,
error: error.message
handler
@temptemp
An interactive, runnable TypeScript val by temptemp
Script
// HTML renderer
primewireApiKey,
primewireBase,
} from "https://raw.githubusercontent.com/Ciarands/mw-providers/dev/src/providers/sources/primewire/common.ts";
interface Meta {
current_quality: string;
id: string;
imdb_id: string;
title: string;
tvdb_id?: null | string;
cleanHtml
@chet
An interactive, runnable TypeScript val by chet
Script
export function cleanHtml(html: string) {
// Remove script, link tags
html = html.replace(
/<\s*(script|link|time|form)\b[^<]*(?:(?!<\/\s*\1\s*>)<[^<]*)*<\/\s*\1\s*>/gi,
// Remove inputs because date initial values can change
html = html.replace(/<\s*(input)\b[^<]*\s*>/gi, "");
// Remove style attributes
html = html.replace(/ style="[^"]*"/gi, "");
// Separate all tags by newlines
html = html.replace(/><+/g, ">\n<");

manchesterBerylStations
@neverstew
An interactive, runnable TypeScript val by neverstew
Script
export let manchesterBerylStations = fetchJSON(
"https://gbfs.beryl.cc/v2_2/Greater_Manchester/station_information.json",
).then((data) =>
data.data.stations.map((station) => {
const { station_id, name, lat, lon, capacity } = station;
const stationStatus =
manchesterBerylStationStatus[station.station_id];
const { num_bikes_available, num_docks_available } = stationStatus;
return ({
station_id,
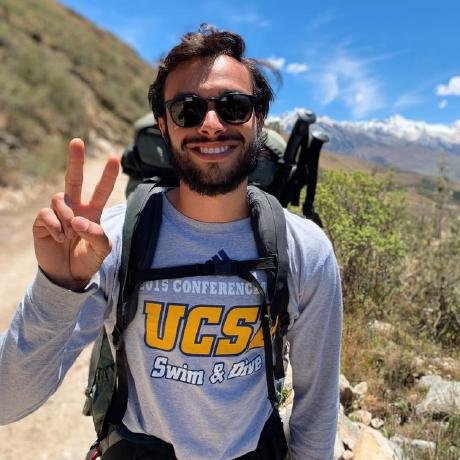
weather
@cjpais
// Forked from @marcel.weather
Script
export const weather = async (city: string) => {
weather2(city)
.then(({ weather }) => ({
w: weather,
.then(({ w }) =>
w.map((d) => ({
maxTempF: `${d.maxtempF}`,
minTempF: `${d.mintempF}`,
description: d.hourly.map((h) => h.weatherDesc[0].value),
// Forked from @marcel.weather

remember
@mxderouet
An interactive, runnable TypeScript val by mxderouet
Script
export let remember = function () {
console.email("Remember to try val.town for Weather Bot");
primewire
@tempdev
An interactive, runnable TypeScript val by tempdev
Script
const NotFoundError = Error;
primewireApiKey,
primewireBase,
} from "https://raw.githubusercontent.com/Ciarands/mw-providers/dev/src/providers/sources/primewire/common.ts";
async function search(imdbId: string) {
const searchResult = await fetch(`${primewireBase}/api/v1/show?key=${primewireApiKey}&imdb_id=${imdbId}`);
return await searchResult.json().then((searchResult) => {
return searchResult.id;
async function getStreams(title: string) {
const titlePage = load(title);
val_YvPY6DIAQD
@dhvanil
An interactive, runnable TypeScript val by dhvanil
HTTP
export const jsonOkExample = () => Response.json({ ok: true });
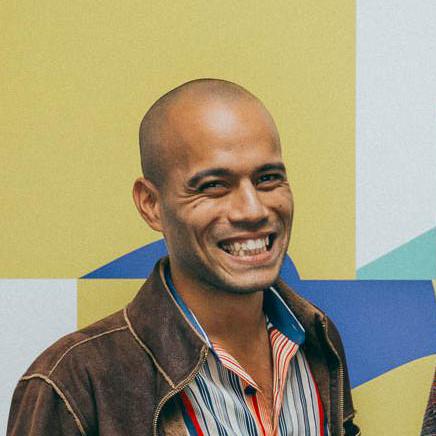
annoy
@ajax
An interactive, runnable TypeScript val by ajax
Script
export async function annoy() {
const resume = resume2;
// const boo = await import("https://esm.sh/@atproto/api");
const { default: BskyAgent } = await import("npm:@atproto/api");
console.log(BskyAgent);
const agent = new BskyAgent.BskyAgent({ service: "https://bsky.social" });
await agent.login({
identifier: process.env.BLUESKY_USERNAME!,
password: process.env.BLUESKY_PASSWORD!,
// const actor = await agent.getProfile();
valle_tmp_53901278199117542092711693057927
@janpaul123
// This val fetches weather data from an open API
HTTP
// This val fetches weather data from an open API
// The approach involves using the fetch API provided by Deno
// to retrieve weather information for a specific location
export default async function main(req: Request): Promise<Response> {
const apiUrl = "https://api.open-meteo.com/v1/forecast?latitude=40.6782&longitude=-73.9442&hourly=temperature_2m¤t_weather=true";
const response = await fetch(apiUrl);
const weatherData = await response.json();
return new Response(JSON.stringify(weatherData), { headers: { "Content-Type": "application/json" } });
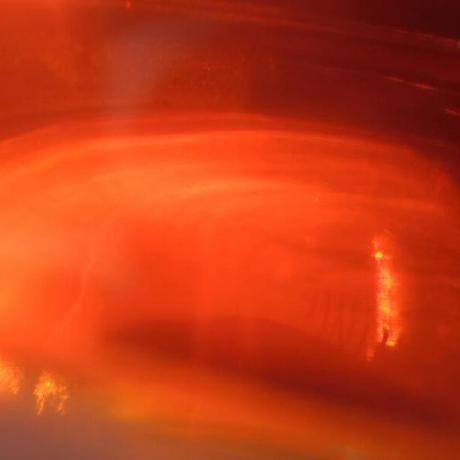
proxyFetch
@dpetrouk
// Forked from @alp.proxyFetch
Script
export const proxyFetch = async (req, res) => {
const { url, options } = req.body;
try {
const response = await fetch(url, options);
return res.status(response.status).send(await response.text());
catch (e) {
const errorMessage = e instanceof Error ? e.message : "Unknown error";
console.error("Failed to initiate fetch", e);
return res.status(500).send(`Failed to initiate fetch: ${errorMessage}`);
// Forked from @alp.proxyFetch
dataTableScript
@skyehersh
// Changes to the inputs will trigger a redraw to update the table
Script
const minEl = document.getElementById("min");
const maxEl = document.getElementById("max");
const searchEl = document.getElementById("search");
const desiredGenderEl = document.getElementById("desired-gender");
const yourGenderEl = document.getElementById("your-gender");
const styleEl = document.getElementById("style");
DataTable.ext.search.push(function(settings, data, dataIndex) {
let min = parseInt(minEl.value, 10);
let max = parseInt(maxEl.value, 10);
let age = parseFloat(data[1]) || 0;