Search
valle_tmp_69038696901412859657208359078762
@janpaul123
// This val responds with "Hello, World!" to all incoming HTTP requests
HTTP
// This val responds with "Hello, World!" to all incoming HTTP requests
export default async function main(req: Request): Promise<Response> {
return new Response("Hello, World!", { headers: { "Content-Type": "text/plain" } });
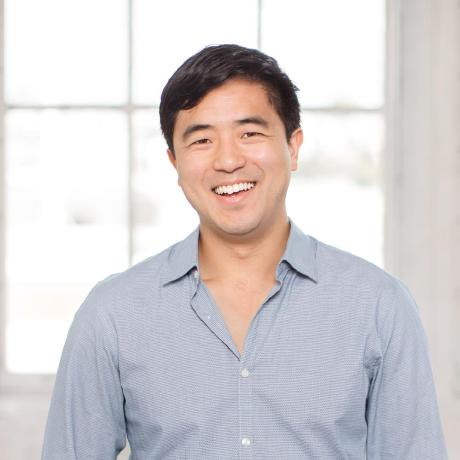
langchainEx
@jacoblee93
// Forked from @stevekrouse.langchainEx
Script
export const langchainEx = (async () => {
const { ChatOpenAI } = await import(
"https://esm.sh/langchain/chat_models/openai"
const { PromptTemplate } = await import("https://esm.sh/langchain/prompts");
const { LLMChain } = await import("https://esm.sh/langchain/chains");
const model = new ChatOpenAI({
temperature: 0.9,
openAIApiKey: process.env.OPENAI_API_KEY,
verbose: true,
const template = "What is a good name for a company that makes {product}?";
fetchPinatsPosts
@stevedylandev
An interactive, runnable TypeScript val by stevedylandev
Script
let currentDate = new Date();
let endDate = Math.floor(currentDate.getTime() / 1000);
let startDate = Math.floor(currentDate.setDate(currentDate.getDate() - 7) / 1000);
export async function fetchPinataPosts() {
try {
const soRes = await fetch(
`https://api.stackexchange.com/2.3/questions?fromdate=${startDate}&todate=${endDate}&order=desc&sort=creation&tagged=pinata&site=stackoverflow`,
method: "GET",
const soResData = await soRes.json();
const soItemsArray = soResData.items;
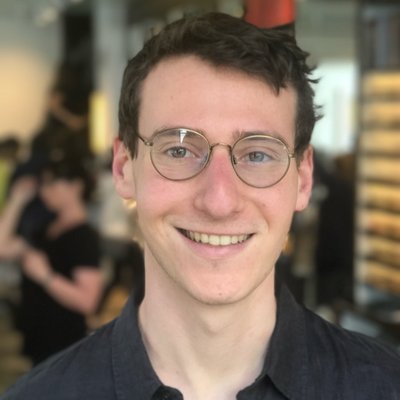
testReceive
@stevekrouse
An interactive, runnable TypeScript val by stevekrouse
Script
export function testReceive(req, res) {
res.send("ok");
val_GxgfY0QutG
@dhvanil
An interactive, runnable TypeScript val by dhvanil
HTTP
export const jsonOkExample = () => Response.json({ ok: true });
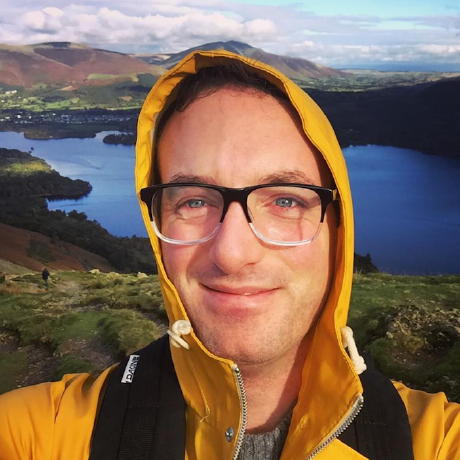
auth
@merlin
An interactive, runnable TypeScript val by merlin
Script
export const auth = (req, res) => {
// check if we've exceed the ratelimiting for the current IP
if (!rateLimit(req.ip, 50)) {
return res.status(429).send("Rate limiting exceeded");
const domainAllowList = [
"http://localhost:3000",
"https://www.merlinmason.co.uk",
const deviceId: string = req.get("Authorization").replace("Bearer ", "") ??
const validOrigin = domainAllowList.includes(req.get("origin"));
if (deviceId.length !== 32 || !validOrigin) {

prettifyHtml
@neverstew
An interactive, runnable TypeScript val by neverstew
Script
import prettierPluginHtml from "https://unpkg.com/prettier@3.2.5/plugins/html.mjs";
import * as prettier from "npm:prettier/standalone.mjs";
export const prettifyHtml = (html: string) => prettier.format(html, { parser: "html", plugins: [prettierPluginHtml] });
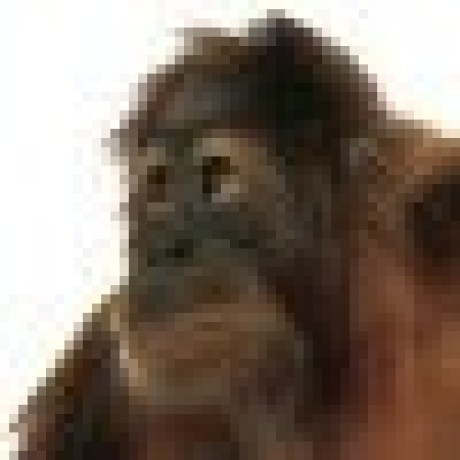
val2img
@pomdtr
This val is supposed to be used with the val.town extension. See the extension readme for installation instructions.
Script
This val is supposed to be used with the val.town extension. See [the extension readme](https://github.com/pomdtr/val-town-web-extension) for installation instructions.
export default async function(ctx: BrowserContext<{ author: string; name: string }>) {
const { author, name } = ctx.params;
const title = `@${author}/${name}`;
const resp = await fetch(`https://api.val.town/v1/alias/${author}/${name}`, {
headers: {
Authorization: `Bearer ${Deno.env.get("valtown")}`,
if (!resp.ok) {
throw new Error(await resp.text());
const { code } = await resp.json();
slackapp
@nate
// A Slack slash command app to evaluate a val.town expression.
Script
// A Slack slash command app to evaluate a val.town expression.
// Setup: Add a slash command using
// "https://api.val.town/express/@nate.slackapp" as the request URL.
// Usage: /yourcommandname {expression}
// Examples:
// - /evaltown 1+1
// - /evaltown @nate.email("Hi from Slack!")
export let slackapp = async (req, res) => {
if ("ssl_check" in req.body) return;
if (
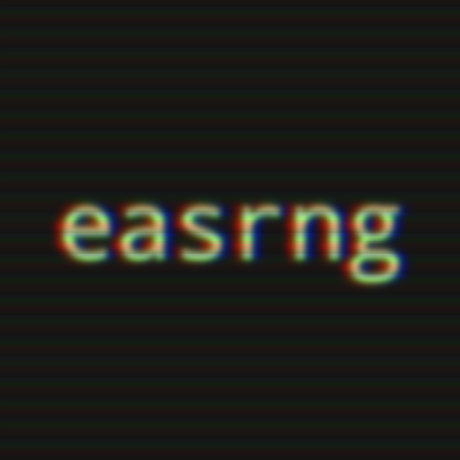
encryption
@easrng
good for session cookies or whatevs
Script
good for session cookies or whatevs
const encoder = new TextEncoder();
const decoder = new TextDecoder();
// $SECRET should be high quality random base64
const baseKey = decodeBase64(Deno.env.get("SECRET"));
function deriveKey(usage: string) {
const tag = encoder.encode(usage);
const hash = nacl.hash(concat([tag, baseKey]));
return hash;
export function encrypt(usage: string, data: Uint8Array): Uint8Array {
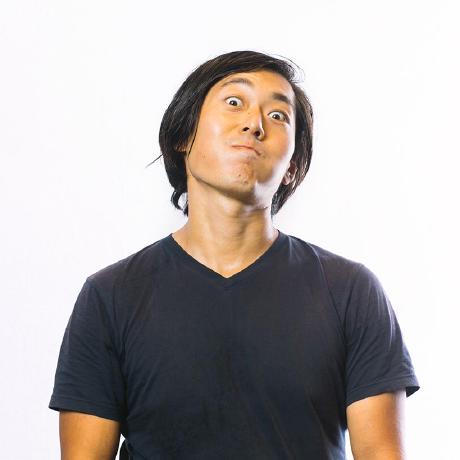
selectHtml
@yawnxyz
SelectHtml is a Deno-based HTML scraper that integrates AI with Cheerio and Pug. This tool allows you to fetch, clean, and manipulate HTML content from URLs, using the Cheerio library to select and clean the HTML elements, and html2pug to convert HTML into Pug templates. Deprecated; use htmlTo instead, as that one supports more formats: https://www.val.town/v/yawnxyz/htmlTo
Script
SelectHtml is a Deno-based HTML scraper that integrates AI with Cheerio and Pug. This tool allows you to fetch, clean, and manipulate HTML content from URLs, using the Cheerio library to select and clean the HTML elements, and html2pug to convert HTML into Pug templates.
Deprecated; use htmlTo instead, as that one supports more formats: https://www.val.town/v/yawnxyz/htmlTo
// import { convertHtmlToMarkdown as semanticMarkdown } from 'npm:dom-to-semantic-markdown'; // uses Node so won't run here
// Function to fetch HTML from a URL and remove <style> and <script> tags
export async function fetchHtml(url, removeSelectors = "style, script, link, noscript, frame, iframe, comment") {
try {
const response = await fetch(url);
const html = await response.text();
// Load the HTML into Cheerio
const $ = cheerio.load(html);
valle_tmp_1383961774625823777944450091493644
@janpaul123
// This code will create a simple Hacker News clone with 30 fake sample stories,
HTTP
// This code will create a simple Hacker News clone with 30 fake sample stories,
// supporting submissions of new stories and upvotes. The data is stored in
// Val Town's blob storage.
// Initial sample stories
const sampleStories = Array.from({ length: 30 }).map((_, index) => ({
id: v4.generate(),
title: `Sample Story ${index + 1}`,
url: `https://example.com/story/${index + 1}`,
upvotes: 0,
const storiesKey = "hn_stories";
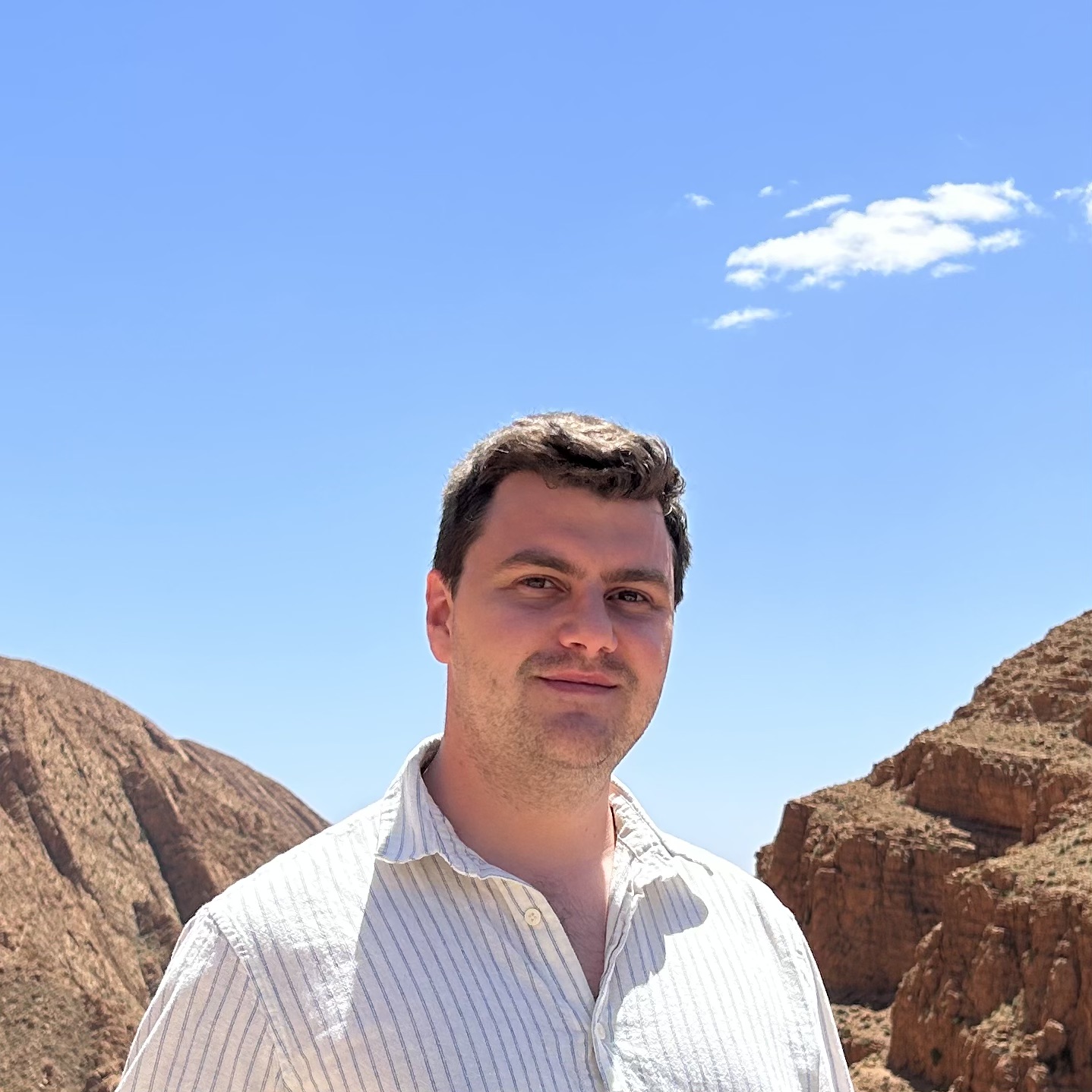
gfm
@rlesser
Convert markdown to Html with Github styling Forked to add remark-mermaid
Script
# Convert markdown to Html with Github styling
*Forked to add remark-mermaid*
// This is kind of a pain, but the existing remark/rehype mermaid plugins
// use playwright internally for some reason, which is a non-starter here
// in good ol' valtown!
const addMermaidScript = () => (tree) => {
const body = select("body", tree);
const script = `
import mermaid from 'https://cdn.jsdelivr.net/npm/mermaid@10/dist/mermaid.esm.min.mjs';
mermaid.initialize({ startOnLoad: false });
diesel
@maxm
Diesel Diesel is a lightweight data manipulation library inspired by Tom Wright's Dasel, designed for easy querying and transformation of various data formats such as JSON, YAML, CSV, and TOML. It allows users to select, update, and delete data using a simple selector syntax. Heavily adapted from https://github.com/TomWright/dasel Features Multi-format Support : Works with JSON, YAML, CSV, and TOML. Dynamic Selectors : Use conditions to filter data dynamically. Function Support : Built-in functions for data manipulation (e.g., length, sum, avg). Easy Integration : Can be used in both Deno and Val Town environments. Usage import Diesel from "https://esm.town/v/yawnxyz/diesel";
async function main() {
const jsonData = `
{
"users": [
{"id": 1, "name": "Alice", "age": 30},
{"id": 2, "name": "Bob", "age": 25},
{"id": 3, "name": "Charlie", "age": 35}
],
"settings": {
"theme": "dark",
"notifications": true
}
}
`;****
const diesel = new Diesel(jsonData, 'json');
try {
console.log("All data:", await diesel.select(''));
console.log("All users:", await diesel.select('users'));
console.log("First user's name:", await diesel.select('users.[0].name'));
console.log("Users over 30:", await diesel.select('users.(age>30)'));
await diesel.put('settings.theme', 'light');
console.log("Updated settings:", await diesel.select('settings'));
// await diesel.delete('users.[1]');
// console.log("Users after deletion:", await diesel.select('users'));
console.log("Data in YAML format:");
console.log(await diesel.convert('yaml'));
console.log("Data in TOML format:");
console.log(await diesel.convert('toml'));
console.log("Number of users:", await diesel.select('users.length()'));
console.log("User names in uppercase:", await diesel.select('users.[*].name.toUpper()'));
} catch (error) {
console.error("An error occurred:", error);
}
}
main(); Installation To use Diesel, simply import it in your Deno project as shown in the usage example. License This project is licensed under the MIT License.
Script
# Diesel
Diesel is a lightweight data manipulation library inspired by Tom Wright's Dasel, designed for easy querying and transformation of various data formats such as JSON, YAML, CSV, and TOML. It allows users to select, update, and delete data using a simple selector syntax.
Heavily adapted from https://github.com/TomWright/dasel
## Features
- **Multi-format Support**: Works with JSON, YAML, CSV, and TOML.
- **Dynamic Selectors**: Use conditions to filter data dynamically.
// import * as jsonMod from "https://deno.land/std@0.224.0/json/mod.ts";
// import * as csv from "npm:csvtojson";
// import { readCSV, readCSVRows, writeCSV } from "jsr:@vslinko/csv";
// import { parse as parseCSV, stringify as stringifyCSV } from "https://deno.land/std/csv/mod.ts";
weatherOfLatLon
@jdan
An interactive, runnable TypeScript val by jdan
Script
import { weatherGovGrid } from "https://esm.town/v/stevekrouse/weatherGovGrid?v=4";
export async function weatherOfLatLon(args: { lat: number; lon: number }) {
const { lat, lon } = args;
const grid = await weatherGovGrid({ lat, lon });
return grid;