Search
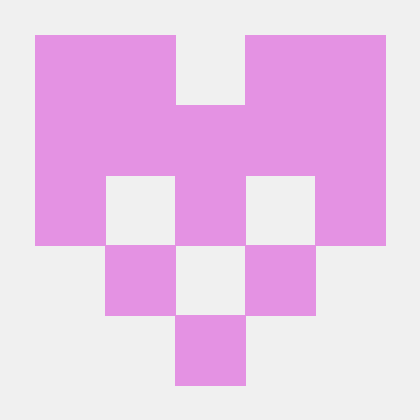
chat
@steveb1313
An interactive, runnable TypeScript val by steveb1313
Script
export const chat = async (
prompt: string | object =
"Reply to this text from my mom: Hey baby, hows it going?",
options = {},
// Initialize OpenAI API stub
const { Configuration, OpenAIApi } = await import("https://esm.sh/openai");
const configuration = new Configuration({
apiKey: process.env.openAIAPI,
const openai = new OpenAIApi(configuration);
// Request chat completion
smilingLimeCardinal
@jeffreyyoung
An interactive, runnable TypeScript val by jeffreyyoung
HTTP
const basePrompt = [
`Generate an image generation model prompt (description) for the previous image, in nightmare before christmas chibi 3D anime style.`,
`The objective is to try to replicate the image as closely as possible,`,
`but in the new style. To do that, you should be very detailed in including`,
`relevant information necessary to reproduce the image, e.g. colors, poses,`,
`facial expressions, background objects, etc. Ensure that the entire description `,
`is consistent with the nightmare before christmas chibi 3D anime style, especially`,
`the 'nightmare before christmas 3D' part. Output only the prompt and nothing else.`,
].join(" ");
const settings = {
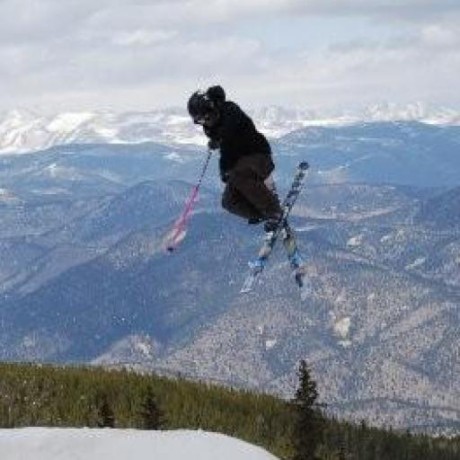
myApi
@chad
An interactive, runnable TypeScript val by chad
Script
import { names } from "https://esm.town/v/chad/names";
export function myApi(req, res) {
names.push(req.query);
return JSON.stringify(req.query);
WebsiteFrontPageAlbum
@edgeeffect
To easily let browser JavaScript use albums from imgbb.com
you can call the URL of this Val with the parameter album holding the album ID string.
It will reduce it down to a JSON object containing a list of the images in that album in the format: [
{"url": "...", "title": "..."},
...
] If there are any errors, it'll just return an empty JSON array.
HTTP
To easily let browser JavaScript use albums from imgbb.com
you can call the URL of this Val with the parameter `album` holding the album ID string.
It will reduce it down to a JSON object containing a list of the images in that album in the format:
```JSON
{"url": "...", "title": "..."},
If there are any errors, it'll just return an empty JSON array.
const items = (html: string) => {
const parser = new DOMParser();
const dom = parser.parseFromString(html, "text/html");
return dom.getElementsByClassName("list-item");
myspaceCSS
@jdan
An interactive, runnable TypeScript val by jdan
Script
export function myspaceCSS() {
return `
.verdana12 {
font-family: verdana;
font-size: 12px;
font-weight: normal;
color: #000000;
.button {
padding-left: .6em;
padding-right: .6em;
valle_tmp_0446237677109970758188110346103484
@janpaul123
// This val will simply respond with "Hello, World!"
HTTP
// This val will simply respond with "Hello, World!"
export default async function(req: Request): Promise<Response> {
return new Response("Hello, World!");
val_6ob0t5Fmjm
@dhvanil
An interactive, runnable TypeScript val by dhvanil
Script
export default async function handler(req) {
try {
const result = await (async () => {
function isPrime(num) {
if (num <= 1) return false;
for (let i = 2; i <= Math.sqrt(num); i++) {
if (num % i === 0) return false;
return true;
function findLowestThreeDigitPrime() {
for (let num = 100; num < 1000; num++) {
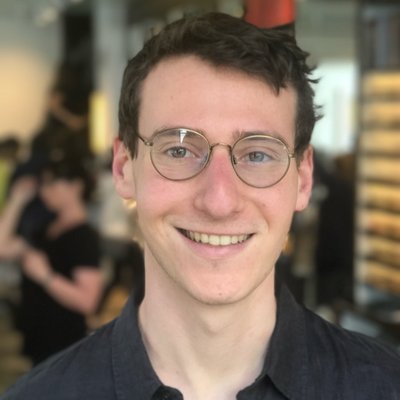
badArgs1
@stevekrouse
An interactive, runnable TypeScript val by stevekrouse
Script
export let badArgs1 = (async () => {
// this should error helpfully
console.log(
await fetchText(
"https://api.val.town/v1/run/stevekrouse.id",
method: "POST",
body: JSON.stringify("hi"),
// this should error helpfully
console.log(
await fetchText(
reqFresh
@yieldray
An interactive, runnable TypeScript val by yieldray
Script
export async function reqFresh(
info: RequestInfo,
init?: RequestInit & {
query?: Record<string, string>;
const res = await request(info, init);
const key = res.url;
const text = await res.clone().text();
try {
if ((await kv(key)) === text)
return { isFresh: false, response: res };
syllabus
@rayyan
@jsxImportSource npm:hono@3/jsx
HTTP
/** @jsxImportSource npm:hono@3/jsx */
function omit<T>(key: keyof T, obj: T) {
const { [key]: omitted, ...rest } = obj;
return rest;
function omitKeys<T>(keys: Array<keyof T>, obj: T) {
const newObj: Record<keyof T, T[keyof T]> = {};
for (const key in obj) {
if (!keys.includes(key)) {
newObj[key] = obj[key];
return newObj;
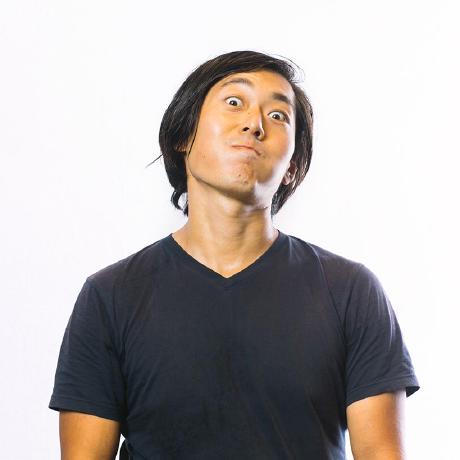
magentaCanidae
@yawnxyz
An interactive, runnable TypeScript val by yawnxyz
HTTP
export default async function (req: Request): Promise<Response> {
return new Response('HELLO HELLO', { status: 200 });
tldraw_computer_example
@ir1d
tldraw computer custom endpoint example This val is an example custom endpoint for tldraw computer 's data component. Usage To use this val with tldraw.computer, follow these steps: Fork this Val. Click the Copy endpoint button on your new Val. Open a project on tldraw.computer Create a Data component in your tldraw computer project In the Source dropdown, select Custom and the POST method Paste the endpoint into Data component's the HTTP Endpoint input. Run the component. To see the output, connect the Data component to a Text component. How it works In tldraw computer, you can configure a Data component to use a custom HTTP endpoint as its data source. You can also configure the request method, either GET or POST. If a Data component's request method is POST, then when the component next updates, it will send a POST request to the endpoint. The request's body will contain an array of the Data objects that the component had received as inputs. If the request method if GET, then the component will only make the request—its body will be empty. In both cases, the component will expect back a response that includes an array of Data objects these objects will be passed along as the data component's outputs. The endpoint (your forked version of this Val) can do whatever it likes between the request and response, but the response must include data in the correct format. If the format is wrong, the computer app will create a text data object instead that includes the response as plain text. Support If you're running into any difficulties, check out the #tldraw-computer channel on the tldraw discord.
HTTP
# tldraw computer custom endpoint example
This val is an example custom endpoint for [tldraw computer](tldraw.computer)'s data component.
### Usage
To use this val with tldraw.computer, follow these steps:
1. Fork this Val.
2. Click the **Copy endpoint** button on your new Val.
// This endpoint accepts and returns data.
type BooleanData = {
type: "boolean";
text: "true" | "false" | "maybe";

zodStringToJSON_example
@freecrayon
// usually this will be passed to you by your Server (i.e. SvelteKit, Remix)
Script
import { zfd } from "npm:zod-form-data";
const $CommentFormData = zfd.formData({
commentId: zfd.text(),
content: zfd.text(zodStringToJSON()),
// usually this will be passed to you by your Server (i.e. SvelteKit, Remix)
const formData = new FormData();
formData.set("commentId", "abc123");
formData.set("content", `{"hello":"world","value":12}`);
const { commentId, content } = $CommentFormData.parse(formData);
console.log({ commentId, content });
val_Ox1arYWfoX
@dhvanil
An interactive, runnable TypeScript val by dhvanil
HTTP
export const jsonOkExample = () => Response.json({ ok: true });

peelSessionArtists
@aeaton
An interactive, runnable TypeScript val by aeaton
Script
import { fetchHtmlDom } from "https://esm.town/v/aeaton/fetchHtmlDom";
export async function peelSessionArtists(url) {
const dom = await fetchHtmlDom(url);
const items = dom.querySelectorAll(".link-list > li > a");
return [...items].map((link) =>
new URL(link.getAttribute("href"), url).toString()