Search
violetFerret
@tempguy
An interactive, runnable TypeScript val by tempguy
Cron
import { blob } from "https://esm.town/v/std/blob?v=12";
export default async function(interval: Interval) {
await fetch("https://tempguy-scarletsole.web.val.run/refresh");
const date = new Date();
await blob.setJSON("turnstileCron", { ptime: date.getTime(), ntime: interval.delay + interval.lastRunAt.getTime() });
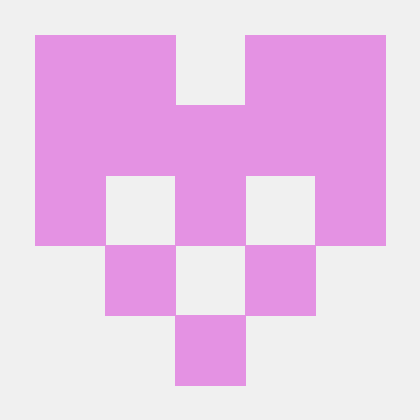
GetPelotonWorkoutsAndSaveToAirTable
@steveb1313
An interactive, runnable TypeScript val by steveb1313
Script
export async function GetPelotonWorkoutsAndSaveToAirTable(
req: express.Request,
res: express.Response,
let response = await fetch("https://api.onepeloton.com/auth/login", {
method: "POST",
mode: "no-cors",
headers: {
"Content-Type": "application/json",
// 'Content-Type': 'application/x-www-form-urlencoded',
credentials: "include",
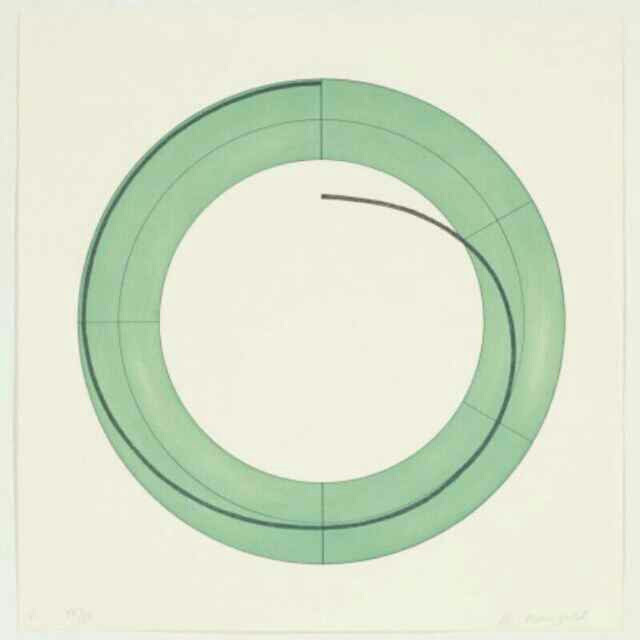
getProjects
@envl
An interactive, runnable TypeScript val by envl
HTTP
import { siteDB } from "https://esm.town/v/envl/siteDB";
export async function getProjects() {
return Response.json((await siteDB).projList);
valle_tmp_39721090126249363719208307534445
@janpaul123
// This val simply responds with "Hello, World!" to any incoming request.
HTTP
// This val simply responds with "Hello, World!" to any incoming request.
export default async function main(req: Request): Promise<Response> {
return new Response("Hello, World!");
squint
@borkdude
Run squint in val.town!
Script
Run squint in val.town!
export let dude = (async () => {
const squint = await import("npm:squint-cljs");
globalThis.squint_core = squint;
const program = `(js/console.log (+ 1 2 3))`;
let theString = squint.compileString(program, { repl: true, "elide-imports": true });
console.log(theString);
theString = theString.replace(`'squint-cljs/core.js'`, `'npm:squint-cljs/core.js'`);
const AsyncFunction = Object.getPrototypeOf(async function() {}).constructor;
await (new AsyncFunction(theString)());
xkcd
@loading
An interactive, runnable TypeScript val by loading
HTTP
export default async function(req: Request): Promise<Response> {
return new Response(
(await parseFeed(
await (await fetch(
"https://xkcd.com/atom.xml",
)).text(),
)).entries[0].description?.value!,
status: 200,
headers: {
"content-type": "text/html",
cryptoFearAndGreedIndex
@chrispie
An interactive, runnable TypeScript val by chrispie
Script
import { fetchText } from "https://esm.town/v/stevekrouse/fetchText?v=5";
export const cryptoFearAndGreedIndex = async () => {
const { default: cheerio } = await import("npm:cheerio");
const html = await fetchText(
"https://alternative.me/crypto/fear-and-greed-index/",
const $ = cheerio.load(html);
const index = $(
"#main > section > div > div.columns > div:nth-child(2) > div > div > div:nth-child(1) > div:nth-child(2) > div",
).first().text();
return parseInt(index);
camera_app
@tionis
An interactive, runnable TypeScript val by tionis
Script
import WebcamPage from "https://esm.sh/gh/fal-ai/fal-js@9cc7cf5456/apps/demo-nextjs-app-router/app/camera-turbo/page.tsx";
console.log(WebcamPage.toString());
sassy
@pinjasaur
sassy Compile Sass into CSS on the fly. Inspired by me doing the same thing on Webtask when that was still around. Read more . Use like so: curl -X POST -H "Content-Type: application/json" -d '{ "sass": "body { background: rgba(#bada55, .5) }" }' https://pinjasaur-sassy.web.val.run
HTTP
# sassy
Compile Sass into CSS on the fly.
Inspired by me doing the [same thing on Webtask](https://paul.af/compiling-scss-with-webtask) when that was still around. [Read more](https://paul.af/val-town).
Use like so:
```sh
curl -X POST -H "Content-Type: application/json" -d '{ "sass": "body { background: rgba(#bada55, .5) }" }' https://pinjasaur-sassy.web.val.run
export default async function(req: Request): Promise<Response> {
if (req.method !== "POST") {
return Response.json({ error: "This val responds to POST requests." }, {
status: 400,
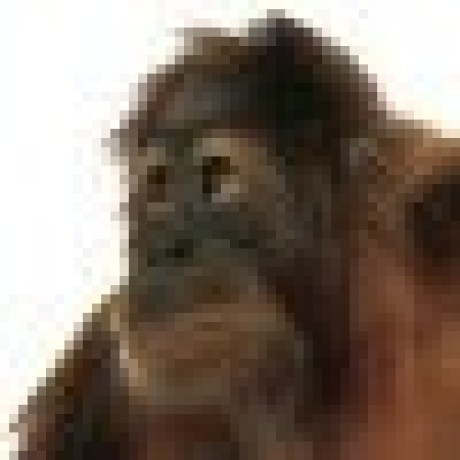
heynote
@pomdtr
An interactive, runnable TypeScript val by pomdtr
HTTP
import { handler } from "jsr:@pomdtr/val-town-heynote@0.1.1";
export default handler;
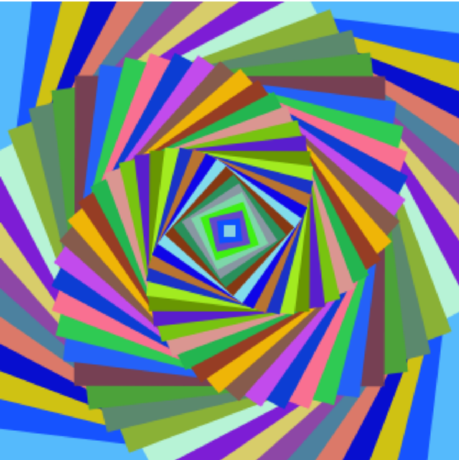
consoleEmailEx
@rpf
An interactive, runnable TypeScript val by rpf
Script
export let consoleEmailEx = (() => {
console.email("message"); // any JSON object can be the message
console.email({ html: "<h1>hello html emails!</h1>" }); // you can send HTML emails
console.email({ hi: "there" }, "Subject Line"); // optional second arg is the subject line
console.email({ html: "<b>hi!</b>", subject: "Subject accepted here too" });
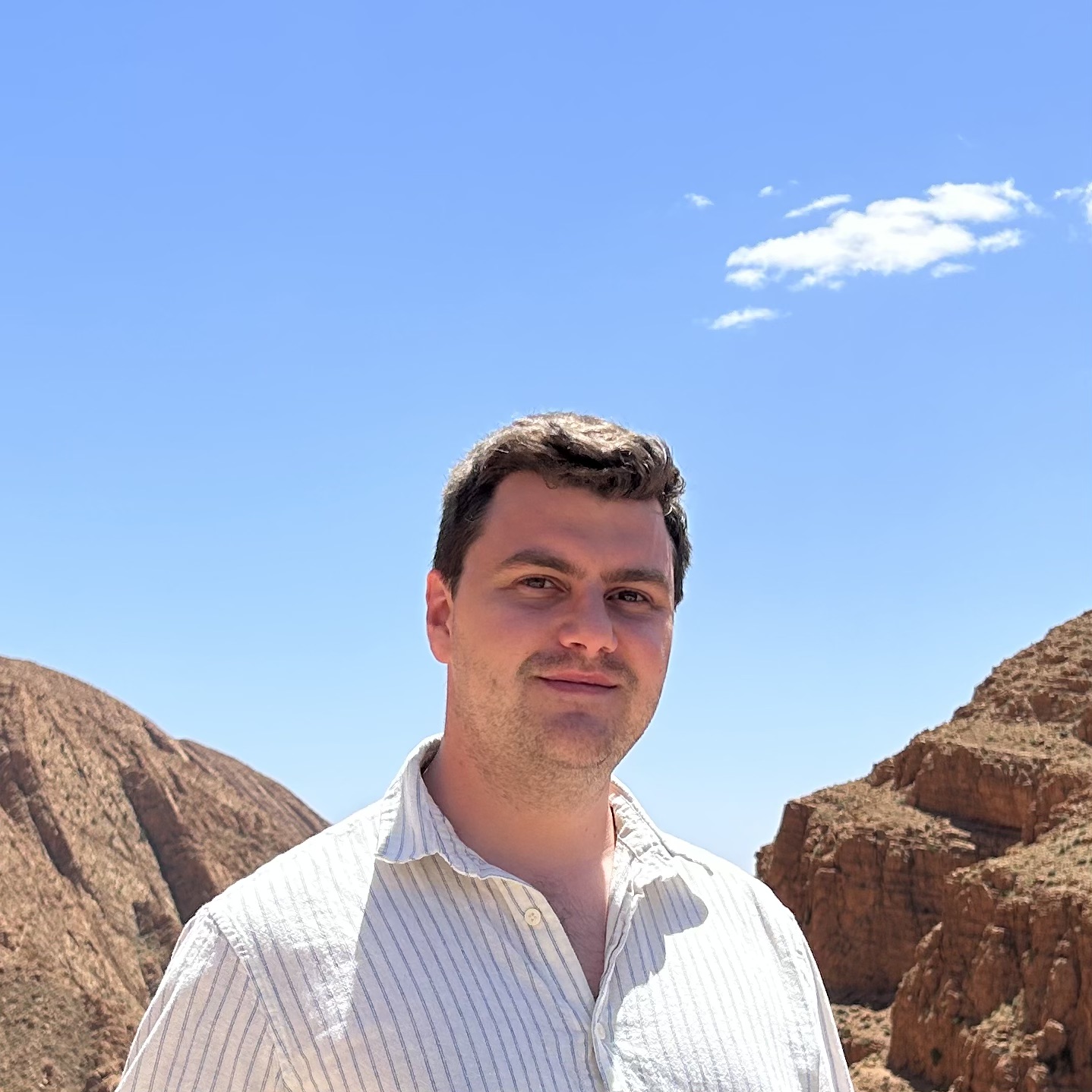
pushover
@rlesser
// Send a pushover message.
Script
// Send a pushover message.
// token, user, and other opts are as specified at https://pushover.net/api
export async function pushover({ token, user, message, title, url, ...opts }) {
return await fetch("https://api.pushover.net/1/messages.json", {
method: "POST",
headers: {
"Content-Type": "application/json",
body: JSON.stringify({
token,
user,
cleanup
@flymaster
Every day, this val sends a message to NTFY.sh, causing my phone to prompt me to fill out a Google Form recording a single object that I decluttered from my house that day.
Cron
Every day, this val sends a message to NTFY.sh, causing my phone to prompt me to fill out a Google Form recording a single object that I decluttered from my house that day.
export default async function(interval: Interval) {
const ntfyChannel = Deno.env.get("CLEANUP_NTFY");
const cleanupFormUrl = Deno.env.get("CLEANUP_FORM");
if (!ntfyChannel || !cleanupFormUrl) {
console.error("Missing required environment variables");
return;
const response = await fetch(`https://ntfy.sh/${ntfyChannel}`, {
method: "POST",
body: `What did you throw out today?`,
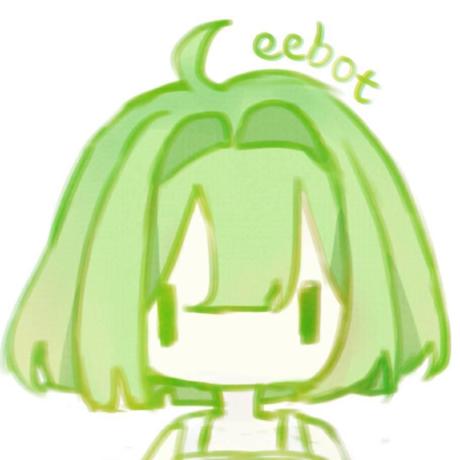
pingReplit
@paperee
An interactive, runnable TypeScript val by paperee
Cron
import { fetch } from "https://esm.town/v/std/fetch";
export async function pingReplit() {
fetch("https://bed.paperee.repl.co/");
fetch("https://school-work.paperee.repl.co/");
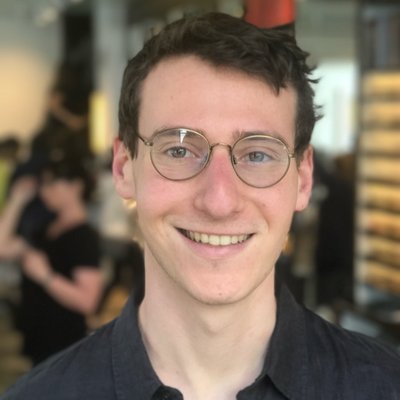
postRequestExample
@stevekrouse
// make POST fetch to https://stevekrouse-parsePostBodyExample.web.val.run
Script
// make POST fetch to https://stevekrouse-parsePostBodyExample.web.val.run
console.log(
await (await fetch("https://stevekrouse-parsePostBodyExample.web.val.run", {
method: "POST",
headers: {
"Content-Type": "application/json",
body: JSON.stringify({ your: "data" }),
})).json(),