Search
simpsonifyMe
@jeffreyyoung
An interactive, runnable TypeScript val by jeffreyyoung
HTTP
const basePrompt =
`Generate an image generation model prompt (description) for the previous image, in the Simpsons cartoon style
Be extremely detailed in describing:
- Hair color
- Poses and expressions
- Gender
- Clothing details and materials
- Skin tone
- Race
- Exact color schemes
distance
@panphora
An interactive, runnable TypeScript val by panphora
HTTP
export default async function distance(req) {
const searchParams = new URL(req.url).searchParams;
const destinationA = encodeURIComponent(String(searchParams.get("a")).trim());
const destinationB = encodeURIComponent(String(searchParams.get("b")).trim());
const apiKey = Deno.env.get("GOOGLE_MAPS_API_KEY");
if (!apiKey) {
return new Response("Google Maps API key is not set", { status: 500 });
const response = await fetch(
`https://maps.googleapis.com/maps/api/distancematrix/json?destinations=${destinationA}&origins=${destinationB}&units=imperial&key=${apiKey}`,
if (!response.ok) {
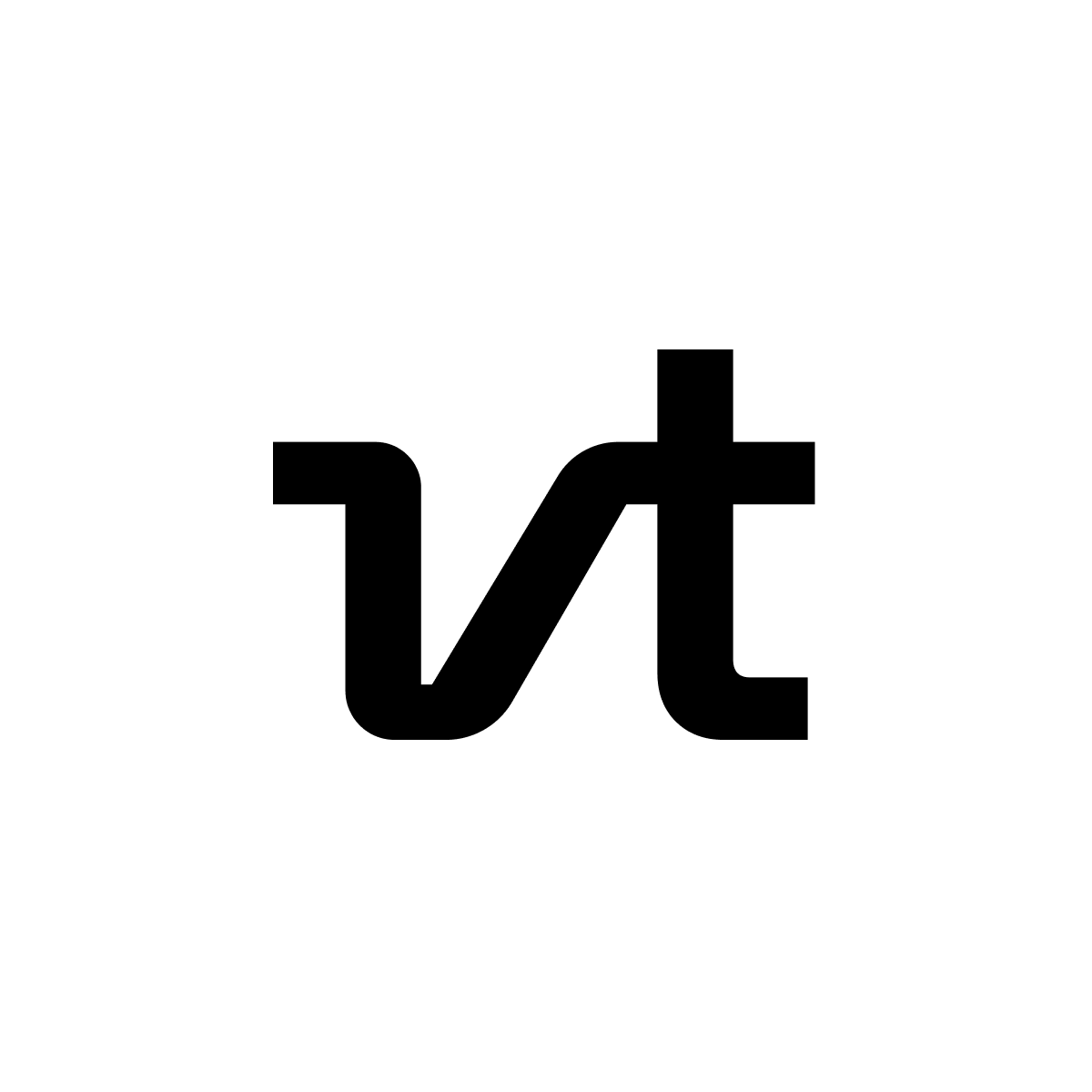
saveFormData
@vtdocs
An interactive, runnable TypeScript val by vtdocs
Script
let { submittedEmailAddresses } = await import("https://esm.town/v/vtdocs/submittedEmailAddresses");
export const saveFormData = async (req: express.Request, res: express.Response) => {
// Create somewhere to store data if it doesn't already exist
if (submittedEmailAddresses === undefined) {
submittedEmailAddresses = [];
// Pick out the form data
const emailAddress = req.body.email;
if (submittedEmailAddresses.includes(emailAddress)) {
return res.send("you're already signed up!");
await email({
defineSlackApp
@fields
An interactive, runnable TypeScript val by fields
Script
export let defineSlackApp = async (req: express.Request, res: express.Response) => {
const text = req.body.text.trim();
res.status(200);
// /undefine
if (req.body.command == "/undefine") {
if (dictionary[text.toLowerCase()]) {
delete dictionary[text.toLowerCase()];
res.send(`Removed definition for *${text}*`);
res.send(`There is no definition for *${text}*`);
return;
generateQR
@loading
Generate QR codes Generate a QR code for any link instantly! Example https://neverstew-generateQR.web.val.run?url=https://neverstew.com
HTTP
# Generate QR codes
Generate a QR code for any link instantly!
## Example
[https://neverstew-generateQR.web.val.run?url=https://neverstew.com](https://neverstew-generateQR.web.val.run?url=https://neverstew.com)
export default async function server(req: Request): Promise<Response> {
let url: URL;
const base64Image = await qrcode(
`https://loading-file.web.val.run/?peer=${new URL(req.url).searchParams.get("peer")}`,
type: "text/html",
return Response.json({ base64Image });
ViewCounter
@youhavetrouble
View counter with unique visitor count
HTTP
/** View counter with unique visitor count */
export default async function(req: Request): Promise<Response> {
if (req.method !== "GET") {
return new Response("Method not allowed", {
status: 405,
headers: {
"Content-Type": "application/json",
let shouldIncrement = true;
if (!req.headers.get("User-Agent")) {
shouldIncrement = false;
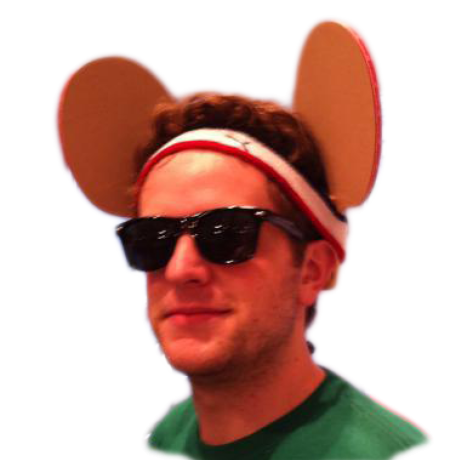
fetchAndStore
@tal
An interactive, runnable TypeScript val by tal
Script
export async function fetchAndStore(opts: {
schoolYear: `${number}-${number}`;
month: string;
menuType: string;
const menuText = await fetchDOEMenu(opts);
const lines: ReturnType<typeof parseMenuLine>[] = await Promise
.all(menuText.split("\r\n").map(parseMenuLine));
const key = dateCalendarKey(opts);
doeMenuResponseStore[key] = {
lastFetchedAt: new Date(),
exampleTranslation
@iamseeley
An interactive, runnable TypeScript val by iamseeley
HTTP
export default async function handler(req) {
if (req.method === "GET") {
return new Response(`
<!DOCTYPE html>
<html>
<body>
<h2>Translate English to German</h2>
<form id="translationForm">
<label for="text">English Text:</label><br>
<input type="text" id="text" name="text"><br><br>
valle_tmp_318720770269803174245544142195994
@janpaul123
// This val will respond with "Hello, world!" to any HTTP request
HTTP
// This val will respond with "Hello, world!" to any HTTP request
export default async function (req: Request): Promise<Response> {
return new Response("Hello, world!");
extraordinaryApricotGuineafowl
@nicosql
An interactive, runnable TypeScript val by nicosql
HTTP
export default async function(req: Request): Promise<Response> {
const url = "https://get.conduitbi.com/conduitbi--5933c";
// Get the current domain
const domain = new URL(req.url).hostname;
// Generate QR code as SVG
const qr = await QRCode.toString(url, {
type: "svg",
width: 512, // High resolution
margin: 4,
color: {
val_wdRvdd0aOO
@dhvanil
An interactive, runnable TypeScript val by dhvanil
Script
export default async function handler(req) {
try {
const result = await (async () => {
function isPrime(num) {
if (num <= 1) return false;
for (let i = 2; i <= Math.sqrt(num); i++) {
if (num % i === 0) return false;
return true;
function findLowestThreeDigitPrime() {
for (let num = 100; num < 1000; num++) {
MyFooter
@leomp12
Fork this if you want to share random vals from your likes in your projects!
HTTP
Fork this if you want to share random vals from your likes in your projects!
const USERNAME = extractValInfo(import.meta.url).author;
const hello = Deno.env.get("HELLO_WORLD");
export async function MyFooter(logo = valTownLogoAuto) {
const recommendation = rootValRef().handle === USERNAME
? html`<span class="recommends">${await recommends()}</span>`
return html`
<footer>
Made by
<a href="https://val.town/u/${USERNAME}" target="_blank">@${USERNAME}</a>
radiodb
@temptemp
// await sqlite.execute(`DROP TABLE IF EXISTS SCHEDULE;`);
Script
// await sqlite.execute(`DROP TABLE IF EXISTS SCHEDULE;`);
await sqlite.execute(`
CREATE TABLE IF NOT EXISTS SCHEDULE (
id DATETIME PRIMARY KEY,
file TEXT,
p_index INTEGER
export async function schedulePush(id: Date, file: string, index: number) {
// console.log(id.toISOString(), file);
await sqlite.execute(
`INSERT INTO SCHEDULE (id,file,p_index) VALUES ('${id.toISOString()}','${file}',${index})`,