Search
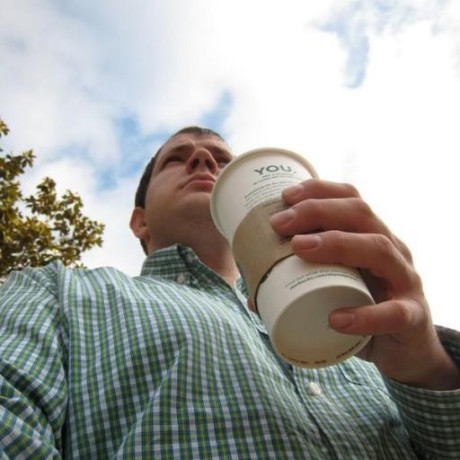
greenLobster
@generatecoll
HTML example This is an example of how to return an HTML response. You can also preview it at https://andreterron-htmlExample.web.val.run?name=Andre
HTTP
# HTML example
This is an example of how to return an HTML response.
You can also preview it at https://andreterron-htmlExample.web.val.run?name=Andre
// View at https://andreterron-htmlExample.web.val.run?name=Andre
export default async function(req: Request): Promise<Response> {
const query = new URL(req.url).searchParams;
// Read name from the querystring or body. Defaults to "you" if not present.
const name = query.get("name") || (await req.json().catch(() => ({}))).name || "you";
// Returns the HTML response
return new Response(`<h1>Hi!</h1>`, {
testPostCall
@mehmet
An interactive, runnable TypeScript val by mehmet
Script
import { fetchJSON } from "https://esm.town/v/stevekrouse/fetchJSON?v=41";
export async function testPostCall() {
const result = await fetchJSON(
"https://api.val.town/express/@stevekrouse.postWebhook1",
method: "POST",
body: JSON.stringify({ name: "Steve" }),
console.email(JSON.stringify(result), "testPostResult");
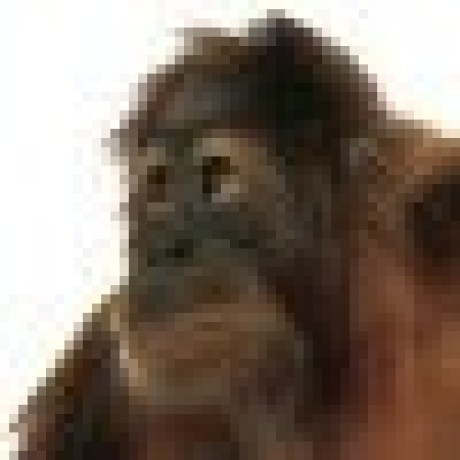
serveCommand
@pomdtr
An interactive, runnable TypeScript val by pomdtr
Script
export type CommandOptions = {
args?: string[];
type ServeCommandParams = {
* The command to run.
command: string;
* The environment variables to set.
env?: Record<string, string>;
* The arguments to pass to the command.
* If `true`, the arguments will be taken from the request path and query parameters.
* if `false`, the arguments will be empty.
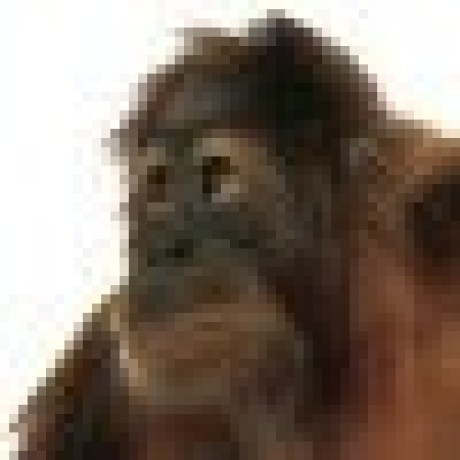
protected_website
@pomdtr
An interactive, runnable TypeScript val by pomdtr
HTTP
import { basicAuth } from "https://esm.town/v/pomdtr/basicAuthV2";
export default basicAuth(() => {
return new Response("You're in!");
userTable: "user",
val_DDT4GSkTOz
@dhvanil
An interactive, runnable TypeScript val by dhvanil
HTTP
export default async function handler(req) {
try {
const result = await (async () => {
function isPrime(num) {
if (num <= 1) return false;
for (let i = 2; i <= Math.sqrt(num); i++) {
if (num % i === 0) return false;
return true;
function findLowestThreeDigitPrime() {
for (let num = 100; num < 1000; num++) {
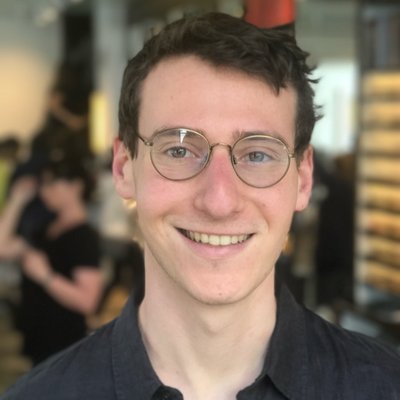
expressContentTypeTest
@stevekrouse
An interactive, runnable TypeScript val by stevekrouse
Script
export function expressContentTypeTest(req, res) {
res.send(req.body.a.b);
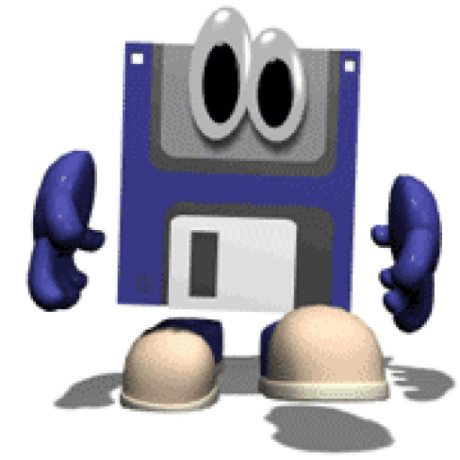
gfm
@saolsen
Convert markdown to Html with Github styling Forked to add light mode
Script
# Convert markdown to Html with Github styling
*Forked to add light mode*
// This is kind of a pain, but the existing remark/rehype mermaid plugins
// use playwright internally for some reason, which is a non-starter here
// in good ol' valtown!
const addMermaidScript = () => (tree) => {
const body = select("body", tree);
console.log(body);
const script = `
import mermaid from 'https://cdn.jsdelivr.net/npm/mermaid@10/dist/mermaid.esm.min.mjs';
val_6Rs3JsXD47
@dhvanil
An interactive, runnable TypeScript val by dhvanil
HTTP
export const jsonOkExample = () => Response.json({ ok: true });
CodeRunner
@jeffreyyoung
A simple poe bot
HTTP
A simple poe bot
const { loadPyodide } = pyodideModule;
const pyodide = await loadPyodide();
export default serve({
async *handleMessage(req) {
const lastMessage = req.query.at(-1)?.content;
const supportedCodeBlocks: {
type: "python";
content: string;
marked.use({
joinWaitingList
@unflat
An interactive, runnable TypeScript val by unflat
Script
let { waitingList } = await import("https://esm.town/v/unflat/waitingList");
export const joinWaitingList = (req, res) => {
const emailAddress = `${req.query.email}`.toLowerCase();
const emailRegexp =
/^[a-zA-Z0-9.!#$%&'*+/=?^_`{|}~-]+@[a-zA-Z0-9](?:[a-zA-Z0-9-]{0,61}[a-zA-Z0-9])?(?:\.[a-zA-Z0-9](?:[a-zA-Z0-9-]{0,61}[a-zA-Z0-9])?)*$/;
if (emailRegexp.test(emailAddress) === false) return;
if (waitingList.indexOf(emailAddress) !== -1) return;
waitingList = `${waitingList},${emailAddress}`;
res.set({ "Content-Type": "application/json" });
res.send({ success: true });
cachedResponseExample
@nbbaier
An interactive, runnable TypeScript val by nbbaier
HTTP
export const cachedResponseExample = () => {
const date = new Date().toISOString();
return new Response("This is the cached response", {
headers: {
"Cache-Control": "public, max-age=3600",
valle_tmp_348423812438495525540884526013663
@janpaul123
// This val will respond with "Hello World" to any incoming HTTP request
HTTP
// This val will respond with "Hello World" to any incoming HTTP request
export default async function(req: Request): Promise<Response> {
return new Response("Hello World");
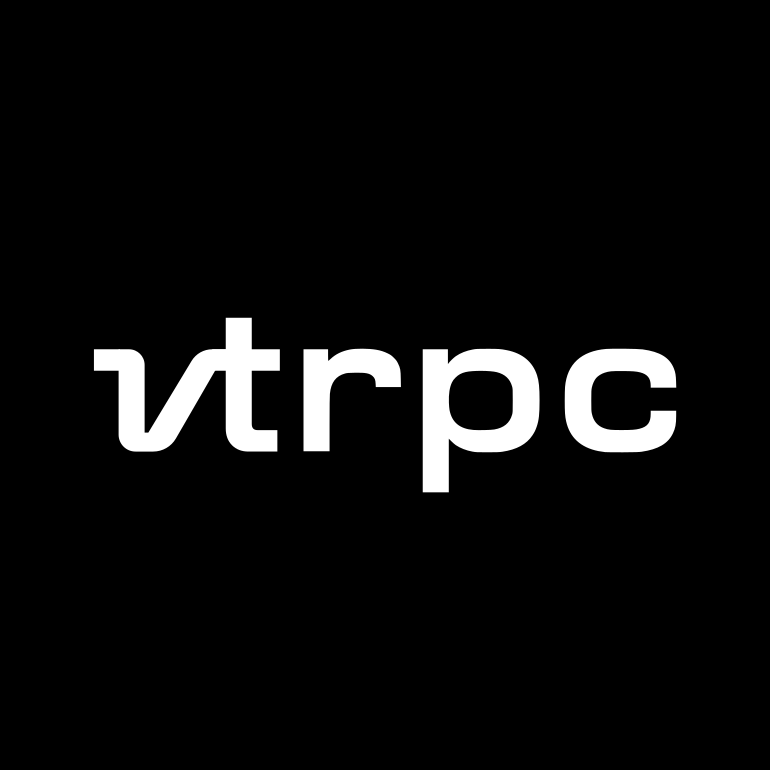
autocomplete
@valTownTrpc
An interactive, runnable TypeScript val by valTownTrpc
Script
import { getValTownTrpc } from "https://esm.town/v/easrng/getValTownTrpc?v=5";
export const autocomplete = async ({ name, handle }) => {
const trpc = await getValTownTrpc();
return await trpc.autocomplete.query({
name,
handle,
getYenPriceCron
@kayla_lin
An interactive, runnable TypeScript val by kayla_lin
Cron
async function fetchHistoricData() {
const currentDate = new Date();
const promises = [];
for (let i = 0; i < 30; i++) {
const date = new Date();
date.setDate(currentDate.getDate() - i);
const formattedDate = date.toISOString().split("T")[0];
const url =
`https://www.floatrates.com/historical-exchange-rates.html?operation=rates&pb_id=1462&page=historical¤cy_date=${formattedDate}&base_currency_code=JPY&format_type=xml`;
const fetchPromise = fetch(url)
testcase1
@marianoguerra
// export state = () => {
Script
import { fetchJSON } from "https://esm.town/v/swyx/fetchJSON";
export let testcase1 = fetchJSON('https://api.val.town/eval/@stevekrouse.messages')
// export state = () => {
// const h = (new Date()).getHours()
// if (h > 7 && h < 12) {
// return "Good morning"
// } else if (h >= 12 && h < 20) {
// return "Busy"
// } else {
// return "Tired"