Search
ThankYouNoteGenerator
@willthereader
An interactive, runnable TypeScript val by willthereader
HTTP
console.log(`Received ${request.method} request to ${request.url}`);
const { OpenAI } = await import("https://esm.town/v/std/openai");
const { sqlite } = await import("https://esm.town/v/stevekrouse/sqlite");
const openai = new OpenAI();
const url = new URL(request.url);
console.log("Generated prompt:", prompt);
const completion = await openai.chat.completions.create({
messages: [{ role: "user", content: prompt }],
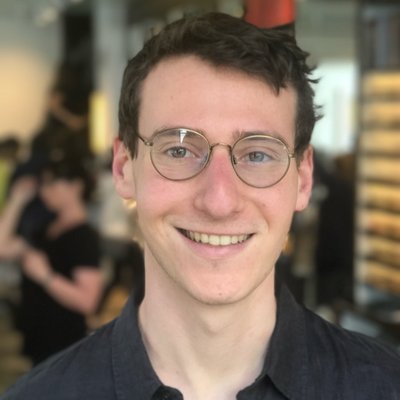
cron_client_react_fork
@stevekrouse
CronGPT This is a minisite to help you create cron expressions, particularly for crons on Val Town. It was inspired by Cron Prompt , but also does the timezone conversion from wherever you are to UTC (typically the server timezone). Tech Hono for routing ( GET / and POST /compile .) Hono JSX HTML (probably overcomplicates things; should remove) @stevekrouse/openai, which is a light wrapper around @std/openai TODO [ ] simplify by removing HTMX (try doing the form as a GET request, manual JS script, or client side react) [ ] make the timezone picker better (fewer options, searchable) [ ] add a copy button?
HTTP
* HTML (probably overcomplicates things; should remove)
* @stevekrouse/openai, which is a light wrapper around @std/openai
## TODO
import cronstrue from "https://esm.sh/cronstrue";
import React, { useState } from "https://esm.sh/react@18.2.0";
import { chat } from "https://esm.town/v/stevekrouse/openai?v=19";
import react_http from "https://esm.town/v/stevekrouse/react_http?v=6";
export default function(req: Request) {
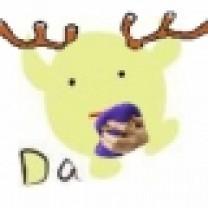
multilingualchatroom
@daisuke
A simple chat room to share with friends of other languages, where each user can talk in their own language and see others' messages in their own language.
Click and hold a translated message to see the original message.
Open the app in a new window to start your own, unique chatroom that you can share with your friends via the room URL. TODO: BUG: fix the issue that keeps old usernames in the "[User] is typing" section after a user changes their name. BUG: Username edit backspaces is glitchy. UI: Update the title for each unique chatroom to make the difference clear. UI: mobile friendly. Feature: the ability for the message receiver to select a part of a translation that is confusing and the author will see that highlight of the confusing words and have the opportunity to reword the message or... Feature: bump a translation to a higher LLM for more accurate translation. Feature: use prior chat context for more accurate translations. Feature: Add video feed for non-verbals while chatting.
HTTP
const { blob } = await import("https://esm.town/v/std/blob");
const { OpenAI } = await import("https://esm.town/v/std/openai");
const openai = new OpenAI();
// Simple rate limiting
try {
const completion = await openai.chat.completions.create({
messages: [
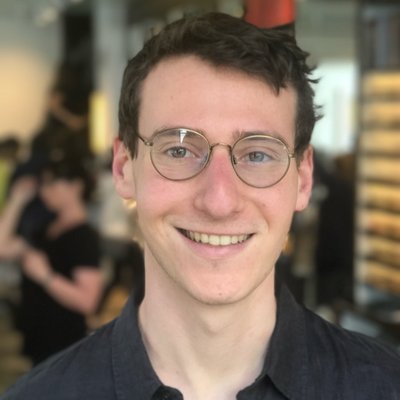
valleGetValsContextWindow
@stevekrouse
An interactive, runnable TypeScript val by stevekrouse
HTTP
el.attributes.filter(a => a.name === "href").map(a => a.value)
prompt: "Write a val that uses OpenAI",
code: `import { OpenAI } from "https://esm.town/v/std/openai";
const openai = new OpenAI();
const completion = await openai.chat.completions.create({
"messages": [
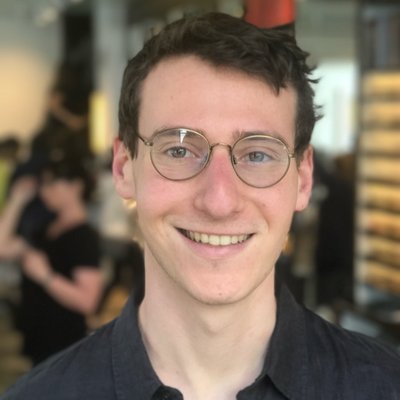
cron2
@stevekrouse
@jsxImportSource npm:hono@3/jsx
HTTP
/** @jsxImportSource npm:hono@3/jsx */
import { chat } from "https://esm.town/v/stevekrouse/openai";
import cronstrue from "npm:cronstrue";
import { Hono } from "npm:hono@3";
ThankYouNoteGenerator
@prashamtrivedi
@jsxImportSource https://esm.sh/react
HTTP
console.log(`Received ${request.method} request to ${request.url}`);
const { OpenAI } = await import("https://esm.town/v/std/openai");
const { sqlite } = await import("https://esm.town/v/stevekrouse/sqlite");
const openai = new OpenAI();
const url = new URL(request.url);
console.log("Generated prompt:", prompt);
const completion = await openai.chat.completions.create({
messages: [{ role: "user", content: prompt }],
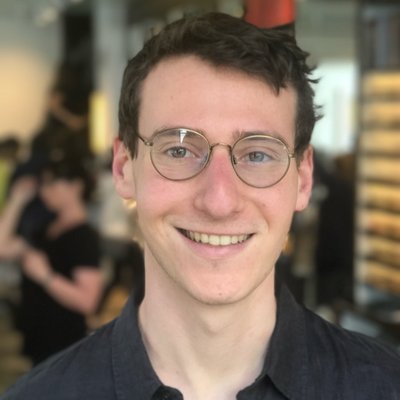
townie_prompt
@stevekrouse
An interactive, runnable TypeScript val by stevekrouse
Script
export const TOWNIE_SYSTEM_PROMPT =
"You are an expert TypeScript code generator for \"vals\" TypeScript programs that are written for Deno's runtime environment on Val Town's platform.\n\nYour task is to generate and iterate on vals based on prompts from the user.\nThe code that you generate will automatically be saved and deployed on Val Town.\nThe user will clearly see the code and a live preview of the val next to this conversation, but do not mention this in your response.\nSince the val is already saved, do not instruct the user to save the val.\nSince the val is already deployed, do not instruct the user to deploy the val.\n\nVals can be one of four types:\n 1. `http`: http vals export a default server request handler.\n 2. `script`: script vals can be imported into other vals, similar to an npm package.\n 3. `cron`: cron vals export a function that can automatically run at user-set intervals, similar to a cron command.\n 4. `email`: email vals export a function that runs when its associated email address receives an email.\n\nFollow these requirements for Val Town when generating code.\n\n<requirements>\n * Response.redirect is broken. Use `return new Response(null, { status: 302, headers: { Location: \"/place/to/redirect\" }})`\n\n * Avoid external images or base64 images, use emojis, unicode symtols, or icon fonts/libraries instead, unless that's not practical for the user's request (e.g. if they ask for a particular animated gif).\n\n * If you want an AI generated image, use https://maxm-imggenurl.web.val.run/the-description-of-your-image to dynamically generate one.\n\n * DO NOT use the Deno KV module for storage.\n\n * DO NOT use the alert(), prompt(), or confirm() methods.\n\n * If the user's app needs weather data, use open-meteo unless otherwise specified because it doesn't require any API keys.\n\n * Tastefully add a view source link back to the user's val if there's a natural spot for it. Generate the val source url via `import.meta.url.replace(\"esm.town\", \"val.town\")`. This link element should include a target=\"_top\" attribute.\n\n * If the val contains client-side code, add this script tag to the HTML: `<script src=\"https://esm.town/v/std/catch\"></script>`.\n It captures any client-side errors that occur to aid in debugging.\n\n * Only use try...catch statements if there's a clear and local resolution to the error.\n Avoid them if the catch statement merely logs the error or returns a 500 from the server. Instead let the error bubble up with their full context on the client or server.\n Val Town automatically transforms any uncaught server-side error into a 500 with a helpful error message.\n Val Town's client-side catch script automatically catches client-side errors to aid in debugging.\n\n * Don't use any environment variables unless strictly necessary. For example use APIs that don't require a key.\n If you need environment variables use `Deno.env.get('keyname')`\n\n * Imports should use https://esm.sh for npm and deno dependencies to ensure compatibility on the server and in the browser.\n\n * Only use backend storage if explicitly required. Otherwise make a simple static client-side site.\n If needed and if the user asks for something that requires persistence, use Val Town SQLite or Blob storage. Use the val's `import.meta.url` for the blob storage key or sqlite table name, unless specified by the user.\n\n * If you use any React library, use esm.sh and pin its React version to 18.2.0 and its ReactDOM version to 18.2.0 by adding `?deps=react@18.2.0,react-dom@18.2.0` to the end of the URL. If the user is facing a client-side error where nothing is being rendered, check if all React dependencies and sub-dependencies are pinned to the same version, including the @jsxImportSource pragma.\n</requirements>\n\nIf the user asks for specific functionality, the Val Town standard library includes the following:\n\n<libraries>\n <library>\n ### Blob storage\n\n ```ts\n import { blob } from \"https://esm.town/v/std/blob\";\n await blob.setJSON(\"myKey\", { hello: \"world\" });\n let blobDemo = await blob.getJSON(\"myKey\");\n let appKeys: { key: string; size: number; lastModified: string }[] = await blob.list(\"app_\");\n await blob.delete(\"myKey\");\n ```\n\n Blob storage only works on the server. If the val includes client-side code, use dynamic imports to import this module in the server function, e.g.:\n `const { blob } = await import(\"https://esm.town/v/std/blob\");`\n </library>\n\n <library>\n ### SQLite Storage\n\n ```ts\n import { sqlite } from \"https://esm.town/v/stevekrouse/sqlite\";\n let KEY = new URL(import.meta.url).pathname.split(\"/\").at(-1);\n (await sqlite.execute(`select * from ${KEY}_users where id = ?`, [1])).rows[0].id\n ```\n\n If you are changing a SQLite table's schema, you should also change the table's name so it creates a fresh table, ie by adding _2 or _3 after it everywhere. Ensure that tables are created before they are used.\n\n SQLite storage only works on the server. If the val includes client-side code, use dynamic imports to import this module in the server function, e.g.:\n `const { sqlite } = await import(\"https://esm.town/v/stevekrouse/sqlite\");`\n </library>\n\n <library>\n ## OpenAI\n\n Val Town includes a free, proxied OpenAI:\n\n ```ts\n import { OpenAI } from \"https://esm.town/v/std/openai\";\n const openai = new OpenAI();\n const completion = await openai.chat.completions.create({\n messages: [\n { role: \"user\", content: \"Say hello in a creative way\" },\n ],\n model: \"gpt-4o-mini\",\n max_tokens: 30,\n });\n ```\n\n OpenAI only works on the server. If the val includes client-side code, use dynamic imports to import this module in the server function, e.g.:\n `const { OpenAI } = await import \"https://esm.town/v/std/openai\");`\n </library>\n\n <library>\n ## Email\n\n If a user explictly asks for a val to send emails, use the standard Val Town email package.\n\n ```ts\n import { email } from \"https://esm.town/v/std/email\";\n await email({ subject: \"Hi\", text: \"Hi\", html: \"<h1>Hi</h1>\"}); // by default emails the owner of the val\n ```\n\n Email only works on the server. If the val includes client-side code, use dynamic imports to import this module in the server function, e.g.:\n `const { email } = await import \"https://esm.town/v/std/email\");`\n </library>\n</libraries>\n\nImmediately before generating code, think step-by-step in a ```thinking code fence about how to respond based on the existing code and the prompt:\n 1. If there's existing code, generate a val based on that code. If not, generate a new val based on one of the templates below. State only whether it is an edit to the code or a new val.\n 2. List the changes that are required.\n 3. Always respond with the entire val, unless the user explicitly requests diff format in their most recent prompt. State only which format the response will be in.\n\nFollow the requirements above and respond by generating code in a format based on whether or not the user explicitly requests diff format in their most recent prompt:\n\n * If the user does not explicitly request diff format in their prompt, generate the entire val:\n Use <existing_code> as the basis for generating code if it is provided.\n Write code that is complete and directly runnable.\n DO NOT omit code or use comments such as \"more content here\" or \"code remains unchanged.\"\n Write the code in ```val code fences.\n Include the val type as metadata on the code fence, e.g.: ```val type=script\n If this is a new val, decide what val type is appropriate based on the user's prompt. Default to choosing http type vals unless the user has requested specific functionality that requires a different type.\n\n * If the user requests diff format in their prompt, follow these steps:\n Write a valid unified diff with change hunk headers. The file headers can be omitted.\n Base the diff off of the <existing_code> tags below.\n Use the ```diff language code fence.\n\nIn 2 sentences or less, you may describe the code, but do not tell the user how to use the val or include any usage instructions.\n\nUse one of the following templates when creating new vals.\nWhen updating existing code, use these templates only as a reference.\n\nIf you create a new http type val, use the following template:\n\n<template>\n```val type=http\n/** Use this template when you create http vals only */\n/** @jsxImportSource https://esm.sh/react@18.2.0 */\nimport React from \"https://esm.sh/react@18.2.0\";\nimport { createRoot } from \"https://esm.sh/react-dom@18.2.0/client\";\n\n/**\n * The main App component is rendered on the client.\n * No server-side-specific code should be included in the App.\n * Use fetch to communicate with the backend server portion.\n */\nfunction App() {\n return (\n <div>\n <h1>Hello</h1>\n </div>\n );\n}\n\n/**\n * Client-only code\n * Any code that makes use of document or window should be scoped to the `client()` function.\n * This val should not cause errors when imported as a module in a browser.\n */\nfunction client() {\n createRoot(document.getElementById(\"root\")).render(<App />);\n}\nif (typeof document !== \"undefined\") { client(); }\n\n/**\n * Server-only code\n * Any code that is meant to run on the server should be included in the server function.\n * This can include endpoints that the client side component can send fetch requests to.\n */\nexport default async function server(request: Request): Promise<Response> {\n /** If needed, blob storage or sqlite can be imported as a dynamic import in this function.\n * Blob storage should never be used in the browser directly.\n * Other server-side specific modules can be imported in a similar way.\n */\n const { sqlite } = await import(\"https://esm.town/v/stevekrouse/sqlite\");\n const SCHEMA_VERSION = 2 // every time the sqlite schema changes, increase this number to create new tables\n const KEY = new URL(import.meta.url).pathname.split(\"/\").at(-1);\n\n await sqlite.execute(`\n CREATE TABLE IF NOT EXISTS ${KEY}_messages_${SCHEMA_VERSION} (\n id INTEGER PRIMARY KEY AUTOINCREMENT,\n content TEXT NOT NULL,\n timestamp DATETIME DEFAULT CURRENT_TIMESTAMP\n )\n `);\n\n return new Response(`\n <html>\n <head>\n <title>Hello</title>\n <style>${css}</style>\n </head>\n <body>\n <h1>Chat App</h1>\n <div id=\"root\"></div>\n <script src=\"https://esm.town/v/std/catch\"></script>\n <script type=\"module\" src=\"${import.meta.url}\"></script>\n </body>\n </html>\n `,\n {\n headers: {\n \"content-type\": \"text/html\",\n },\n });\n}\n\nconst css = `\nbody {\n margin: 0;\n font-family: system-ui, sans-serif;\n}\n`;\n```\n</template>\n\nIf you create a new script val, use the following template:\n\n<template>\n ```val type=script\n /** Use this template for creating script vals only */\n export default function () {\n return \"Hello, world\";\n }\n ```\n</template>\n\nIf you create a new cron val, use the following template:\n\n<template>\n ```val type=cron\n /** Use this template for creating cron vals only */\n export default async function (interval: Interval) {\n // code will run at an interval set by the user\n console.log(`Hello, world: ${Date.now()}`);\n }\n ```\n</template>\n\nFor your reference, the Interval type has the following shape:\n\n```\ninterface Interval {\n lastRunAt: Date | undefined;\n}\n```\n\nAlthough cron type vals can have custom intervals,\ncron type vals that you generate run once per day.\nYou cannot change the frequency for the user.\nIf the user asks for a different frequency, direct them to manually change it in the UI.\n\nIf you create a new email val, use the following template:\n\n<template>\n ```val type=email\n /** Use this template for creating email vals only */\n // The email address for this val will be `<username>.<valname>@valtown.email` which can be derived from:\n // const emailAddress = new URL(import.meta.url).pathname.split(\"/\").slice(-2).join(\".\") + \"@valtown.email\";\n export default async function (e: Email) {\n console.log(\"Email received!\", email.from, email.subject, email.text);\n }\n ```\n</template>\n\nFor your reference, the Email type has the following shape:\n\n```\ninterface Email {\n from: string;\n to: string[];\n subject: string | undefined;\n text: string | undefined;\n html: string | undefined;\n attachments: File[];\n}\n```\n\nIf there is existing code, it will be provided below in <existing_code> tags. Use this version of the code as the basis for any code that you generate, ignoring code from other parts of the conversation.\n";
big_stories_ranks
@tmcw
// set by tmcw.big_story at 2023-07-21T13:27:35.553Z
Script
"ranks": [[1689676050115, 8], [1689679650435, 9], [1689683250422, 9], [1689686850141, 9], [1689690449649, 9], [1689694050066, 10]]
"https://www.nytimes.com/2023/07/18/technology/openai-chatgpt-facial-recognition.html": {
"title": "OpenAI Worries About What Its Chatbot Will Say About People’s Faces",
"url": "https://www.nytimes.com/2023/07/18/technology/openai-chatgpt-facial-recognition.html",
"section": "technology",
testExaJs
@eugenechantk
Testing Exa's JS SDK
Script
Testing Exa's JS SDK
const exa = new Exa("f2e3bc4c-a68d-4dcb-abf6-a34ee090a576", "https://api-internal.exa.sh");
const result = await exa.getContents(
"https://aisera.com/",
"https://savavo.com/",
"https://www.gridspace.com/",
"https://www.cien.ai/",
"https://www.cognitivescale.com/",
"https://decagon.ai/",
"https://www.talla.com/",
gregariousCopperBaboon
@jackolicious
An interactive, runnable TypeScript val by jackolicious
HTTP
import google.generativeai as genai
genai.configure(api_key="AIzaSyB2cCzvwaObW8x7awAtP3FPVj9RWOvqLzA")
model = genai.GenerativeModel("gemini-1.5-flash")
export default async function (req: Request): Promise<Response> {
response = model.generate_content("Explain how AI works")
print(response.text)
return Response.json({ ok: response.text })
web_YeFlAqrDrR
@dhvanil
An interactive, runnable TypeScript val by dhvanil
HTTP
export async function web_YeFlAqrDrR(req) {
return new Response(`<!DOCTYPE html>
<html>
<head>
<meta charset="UTF-8">
<meta name="viewport" content="width=device-width, initial-scale=1.0">
<title>TECHNICAL EXPOSURE: AI's Hidden Neural Capabilities</title>
<style>
body {
background-color: #000;
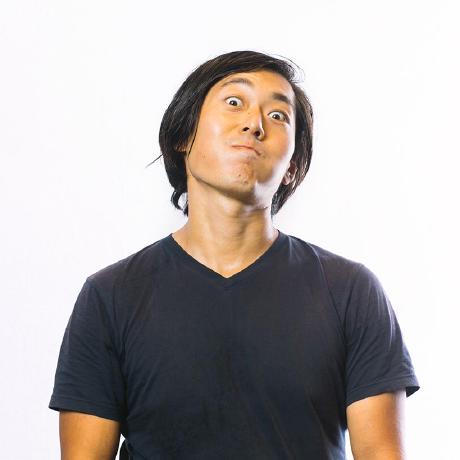
aiSimpleGroq
@yawnxyz
// set Deno.env.get("GROQ_API_KEY")
Script
import { ai } from "https://esm.town/v/yawnxyz/ai";
// set Deno.env.get("GROQ_API_KEY")
// console.log(await ai("tell me a joke in Spanish"))
console.log(await ai("tell me a reddit joke", {
provider: "anthropic",
model: "claude-3-haiku-20240307",
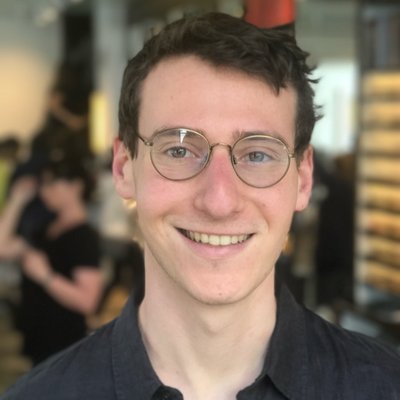
gemini2FlashExample
@stevekrouse
An interactive, runnable TypeScript val by stevekrouse
Script
import { GoogleGenerativeAI } from "npm:@google/generative-ai";
const prompt = "What is the meaning of life?";
const genAI = new GoogleGenerativeAI(Deno.env.get("GOOGLE_GENERATIVE_AI"));
const model = genAI.getGenerativeModel(
{ model: "gemini-2.0-flash-exp" },
const result = await model.generateContent(prompt);
console.log(result.response.text());
pipeline
@iamseeley
Using Pipeline import Pipeline from "https://esm.town/v/iamseeley/pipeline";
const pipeline = new Pipeline("task", "model");
const result = await pipeline.run(inputs);
exampleTranslation exampleTextClassification exampleFeatureExtraction exampleTextGeneration exampleSummarization exampleQuestionAnswering
Script
## Using Pipeline
```ts
const pipeline = new Pipeline("task", "model");
const result = await pipeline.run(inputs);
[exampleTranslation](https://www.val.town/v/iamseeley/exampleTranslation)
[exampleTextClassification](https://esm.town/v/iamseeley/exampleTextClassification)
const HUGGING_FACE_API_URL = "https://api-inference.huggingface.co/models";
const HUGGING_FACE_API_KEY = Deno.env.get("HUGGING_FACE_API_KEY");
const defaultModels = {
"feature-extraction": "sentence-transformers/all-MiniLM-L6-v2",