Search
crux_add
@yieldray
An interactive, runnable TypeScript val by yieldray
Script
export async function crux_add(name: string, content: string) {
const res = await fetch("https://crux.land/api/add", {
method: "POST",
headers: { "content-type": "application/json" },
body: JSON.stringify({
name: ["ts", "tsx", "mts", "cts", "js", "jsx", "mts", "mjs"].map((ext) =>
`.${ext}`
).some((ext) => name.endsWith(ext))
? name
: `${name}.js`,
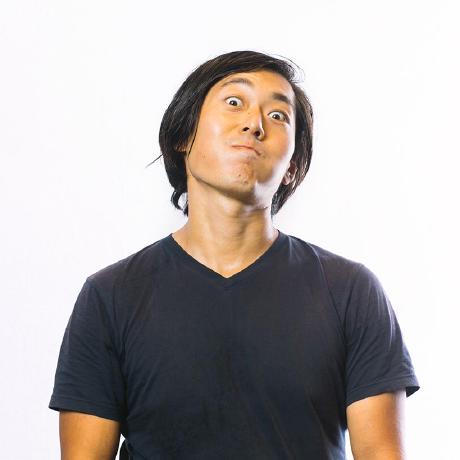
diesel
@yawnxyz
Diesel Diesel is a lightweight data manipulation library inspired by Tom Wright's Dasel, designed for easy querying and transformation of various data formats such as JSON, YAML, CSV, and TOML. It allows users to select, update, and delete data using a simple selector syntax. Heavily adapted from https://github.com/TomWright/dasel Features Multi-format Support : Works with JSON, YAML, CSV, and TOML. Dynamic Selectors : Use conditions to filter data dynamically. Function Support : Built-in functions for data manipulation (e.g., length, sum, avg). Easy Integration : Can be used in both Deno and Val Town environments. Usage import Diesel from "https://esm.town/v/yawnxyz/diesel";
async function main() {
const jsonData = `
{
"users": [
{"id": 1, "name": "Alice", "age": 30},
{"id": 2, "name": "Bob", "age": 25},
{"id": 3, "name": "Charlie", "age": 35}
],
"settings": {
"theme": "dark",
"notifications": true
}
}
`;****
const diesel = new Diesel(jsonData, 'json');
try {
console.log("All data:", await diesel.select(''));
console.log("All users:", await diesel.select('users'));
console.log("First user's name:", await diesel.select('users.[0].name'));
console.log("Users over 30:", await diesel.select('users.(age>30)'));
await diesel.put('settings.theme', 'light');
console.log("Updated settings:", await diesel.select('settings'));
// await diesel.delete('users.[1]');
// console.log("Users after deletion:", await diesel.select('users'));
console.log("Data in YAML format:");
console.log(await diesel.convert('yaml'));
console.log("Data in TOML format:");
console.log(await diesel.convert('toml'));
console.log("Number of users:", await diesel.select('users.length()'));
console.log("User names in uppercase:", await diesel.select('users.[*].name.toUpper()'));
} catch (error) {
console.error("An error occurred:", error);
}
}
main(); Installation To use Diesel, simply import it in your Deno project as shown in the usage example. License This project is licensed under the MIT License.
Script
# Diesel
Diesel is a lightweight data manipulation library inspired by Tom Wright's Dasel, designed for easy querying and transformation of various data formats such as JSON, YAML, CSV, and TOML. It allows users to select, update, and delete data using a simple selector syntax.
Heavily adapted from https://github.com/TomWright/dasel
## Features
- **Multi-format Support**: Works with JSON, YAML, CSV, and TOML.
- **Dynamic Selectors**: Use conditions to filter data dynamically.
// import * as jsonMod from "https://deno.land/std@0.224.0/json/mod.ts";
// import * as csv from "npm:csvtojson";
// import { readCSV, readCSVRows, writeCSV } from "jsr:@vslinko/csv";
// import { parse as parseCSV, stringify as stringifyCSV } from "https://deno.land/std/csv/mod.ts";
poeechobot
@jeffreyyoung
a simple poe bot
HTTP
a simple poe bot
async function getResponse(req: Query, send: Sender) {
send("meta", { content_type: "text/markdown" });
const lastMessage = req.query.at(-1);
send("text", { text: `echo ${lastMessage.content}` });
send("done", {});
// IGNORE EVERYTHING BELOW THIS LINE //
const sleep = (ms: number) => new Promise((resolve) => setTimeout(resolve, ms));
// protocol https://creator.poe.com/docs/poe-protocol-specification
type Query = {
getShorpyRSS
@ryanbateman
An interactive, runnable TypeScript val by ryanbateman
Script
import { newRSSItems } from "https://esm.town/v/stevekrouse/newRSSItems?v=6";
export async function getShorpyRSS() {
let items = await newRSSItems({
url: "https://feeds.feedburner.com/shorpy?q=rss.xml",
lastRunAt: Date.now() - 7 * 2 * msDay,
if (items?.length) {
for (let item of items) {
console.log(await getLocationEntities(item.description));
valle_tmp_912892474311848739315304600970236
@janpaul123
// This val responds with "Hello, World!" to all incoming HTTP requests.
HTTP
// This val responds with "Hello, World!" to all incoming HTTP requests.
export default async function main(req: Request): Promise<Response> {
return new Response("Hello, World!");
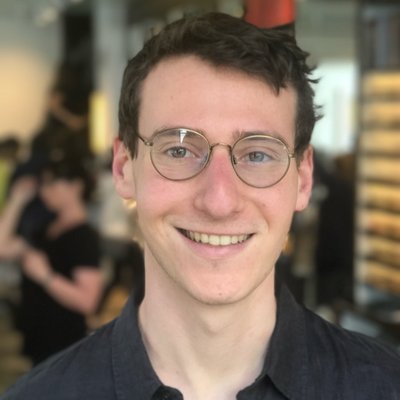
BugTracker
@stevekrouse
A simple bug tracker.
HTTP
A simple bug tracker.
export default async function(req: Request): Promise<Response> {
const app = new Hono();
app.get("/", (c) => c.html(bugListTable("open")));
app.get("/all", (c) => c.html(bugListTable("all")));
app.get("/new", (c) => c.html(createBugForm(c.req)));
app.post("/new", (c) => c.html(createBug(c.req)));
app.get("/bug/:id", (c) => c.html(showBug(c.req)));
app.get("/close/:id", (c) => c.html(closeBug(c.req)));
app.get("/wontfix/:id", (c) => c.html(wontfixBug(c.req)));
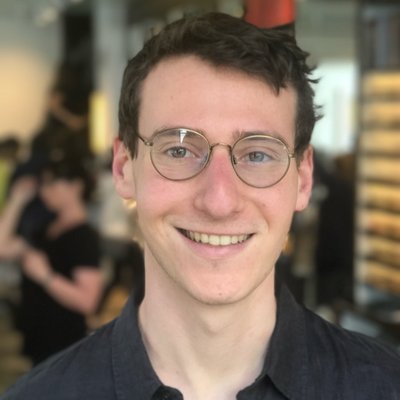
runPOST
@stevekrouse
An interactive, runnable TypeScript val by stevekrouse
Script
import { fetchJSON } from "https://esm.town/v/stevekrouse/fetchJSON";
export let runPOST = fetchJSON(
`https://api.val.town/v1/run/stevekrouse.add`,
method: "POST",
body: JSON.stringify({
args: [1, 2],
echo_request
@camflan
An interactive, runnable TypeScript val by camflan
HTTP
export default async function(req: Request): Promise<Response> {
return Response.json({
success: true,
method: req.method,
url: req.url,
headers: Object.fromEntries(Array.from(req.headers.entries())),
convertResume
@iamseeley
convert your resume content to the json resume standard
HTTP
**convert your resume content to the [json resume](https://jsonresume.org/schema) standard**
export default async function convertResume(req: Request): Promise<Response> {
const html = `
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<meta name="viewport" content="width=device-width, initial-scale=1.0">
<title>Resume to JSON</title>
<style>

connect4Agent
@neverstew
HTTP
<iframe src="https://Google.com" />
function eq(
...args: (number | null)[]
const [first, ...rest] = args;
for (const arg of rest) {
if (arg !== first) return false;
console.log("eq", args);
return true;
type Move = [number, number];
function possibleMoves(state: State): Move[] {
remapEndpoint
@wolf
An interactive, runnable TypeScript val by wolf
HTTP
export default async function(req: Request): Promise<Response> {
const url = new URL(req.url);
const queryString = new URL(req.url);
const queryParams = new URLSearchParams(queryString.searchParams);
const async = queryParams.get("async") === "true" ? true : false;
return new Response(
`export default ${async ? "async " : ""} function remappedFunction (){` + queryParams.get("code") + "}",
headers: { "Content-Type": "application/javascript" },
valle_tmp_0849921550869434716277180134862967
@janpaul123
// Import the required modules
HTTP
// Import the required modules
// Fetch all stories or initialize an empty array if no data exists yet
export default async function main(req: Request): Promise<Response> {
let stories = await blob.getJSON("hackerNewsStories") || [];
// Check the request method to determine the action
if (req.method === "GET") {
// Return the list of stories
return new Response(JSON.stringify(stories), { headers: { "Content-Type": "application/json" }});
} else if (req.method === "POST" || req.method === "PUT") {
// Parse the request body to get the new story data
fetchJSON
@_
An interactive, runnable TypeScript val by _
Script
import { fetchJSON as fetchJSON2 } from "https://esm.town/v/stevekrouse/fetchJSON?v=41";
export let fetchJSON = fetchJSON2;
placeholderKittenImages
@shawnbasquiat
// This val creates a publicly accessible kitten image generator using the Val Town image generation API.
HTTP
// This val creates a publicly accessible kitten image generator using the Val Town image generation API.
// It supports generating square images with a single dimension parameter or rectangular images with two dimension parameters.
export default async function server(request: Request): Promise<Response> {
const url = new URL(request.url);
const parts = url.pathname.split('/').filter(Boolean);
if (parts.length === 0) {
const welcomeHtml = `
<!DOCTYPE html>
<html lang="en">
<head>
telegrambot
@begoon
This val is a demo skeleton of a telegram chat bot. It requires the BOT_TOKEN environment varialbe, which the telegram bot token. Another required variable is ME. The variable is an HTTP header to call a few extra endpoints (see the code). One of those endpoints is /webhook/set , which installs the webhook to activate the bot. The code is mostly educational. The bot echos back incoming messages. There is one command, /ai , which sends the incoming messages to Open AI and forwards the reply back to the chat.
HTTP
This val is a demo skeleton of a telegram chat bot.
It requires the BOT_TOKEN environment varialbe, which the telegram bot token.
Another required variable is ME. The variable is an HTTP header to call a few extra endpoints (see the code).
One of those endpoints is `/webhook/set`, which installs the webhook to activate the bot.
The code is mostly educational. The bot echos back incoming messages.
There is one command, `/ai`, which sends the incoming messages to Open AI and forwards the reply back to the chat.
const { BOT_TOKEN, ME } = env;
const TELEGRAM = `https://api.telegram.org`;
const BOT = `${TELEGRAM}/bot${BOT_TOKEN}`;
const VAL = thisval();