Search
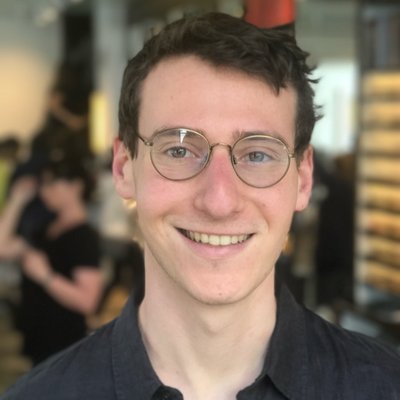
handleFormExample
@stevekrouse
An interactive, runnable TypeScript val by stevekrouse
Script
import { email } from "https://esm.town/v/std/email?v=9";
export async function handleFormExample(req, res) {
await email({
text: JSON.stringify(req.query, null, 2),
subject: "New form submission",
myTestForm.push(req.query);
return res.send(`<h1>Hi ${req.query.first} ${req.query.last}!</h1>`);
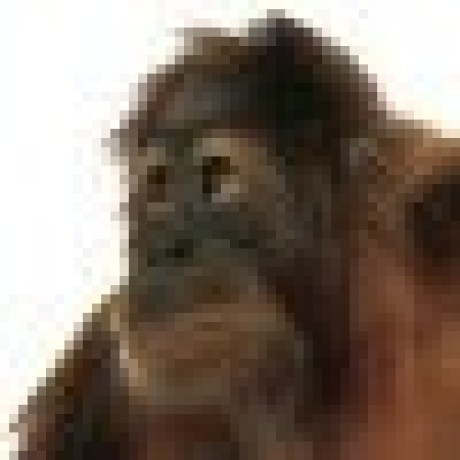
apricotPartridge
@pomdtr
// use my private proxy
HTTP
import { setEnv } from "https://esm.town/v/pomdtr/setEnv";
// use my private proxy
setEnv("VALTOWN_API_URL", "https://pomdtr-tursoproxy.web.val.run");
// need to be dynamic
const { handler } = await import("https://esm.town/v/nbbaier/sqliteExplorerApp");
export default handler;
earthquakes
@fil
Earthquake map 🌏 This val loads earthquake data from USGS, a topojson file for the land shape, and supporting libraries. It then creates a map and save it as a SVG string. The result is cached for a day. Note that we must strive to keep it under val.town’s limit of 100kB, hence the heavy simplification of the land shape. (For a simpler example, see becker barley .) | | |
|-----|-----|
| Web page | https://fil-earthquakes.web.val.run/ |
| Observable Plot | https://observablehq.com/plot/ |
| linkedom | https://github.com/WebReflection/linkedom |
| topojson | https://github.com/topojson/topojson |
| earthquakes | https://earthquake.usgs.gov |
| world | https://observablehq.com/@visionscarto/world-atlas-topojson |
| css | https://milligram.io/ |
HTTP
# Earthquake map [🌏](https://fil-earthquakes.web.val.run/)
This val loads earthquake data from USGS, a topojson file for the land shape, and supporting libraries. It then creates a map and save it as a SVG string. The result is cached for a day. Note that we must strive to keep it under val.town’s limit of 100kB, hence the heavy simplification of the land shape. (For a simpler example, see [becker barley](https://www.val.town/v/fil.beckerBarley).)

| Web page | https://fil-earthquakes.web.val.run/ |
| Observable Plot | https://observablehq.com/plot/ |
| linkedom | https://github.com/WebReflection/linkedom |
let { earthquakes_storage } = await import("https://esm.town/v/fil/earthquakes_storage");
export async function earthquakes(req?) {
const yesterday = new Date(-24 * 3600 * 1000 + +new Date()).toISOString();
if (!(earthquakes_storage?.date > yesterday)) {
cssReset
@nbbaier
An interactive, runnable TypeScript val by nbbaier
Script
export const reset = `
Josh's Custom CSS Reset
https://www.joshwcomeau.com/css/custom-css-reset/
*, *::before, *::after {
box-sizing: border-box;
margin: 0;
body {
line-height: 1.5;
-webkit-font-smoothing: antialiased;
img, picture, video, canvas, svg {
streamingResponseExample
@maxm
An interactive, runnable TypeScript val by maxm
HTTP
export default async function(req: Request): Promise<Response> {
let timer: number | undefined = undefined;
const body = new ReadableStream({
start(controller) {
timer = setInterval(() => {
const message = `It is ${new Date().toISOString()} ${getRandomEmoji()}\n`;
controller.enqueue(new TextEncoder().encode(message));
}, 200);
cancel() {
if (timer !== undefined) {

saveFormData
@neverstew
An interactive, runnable TypeScript val by neverstew
HTTP
let { submittedEmailAddresses } = await import("https://esm.town/v/neverstew/submittedEmailAddresses");
export const saveFormData = async (req: Request) => {
// Create somewhere to store data if it doesn't already exist
if (submittedEmailAddresses === undefined) {
submittedEmailAddresses = [];
// Pick out the form data
const formData = await req.formData();
const emailAddress = formData.get("email");
if (submittedEmailAddresses.includes(emailAddress)) {
return new Response("you're already signed up!");
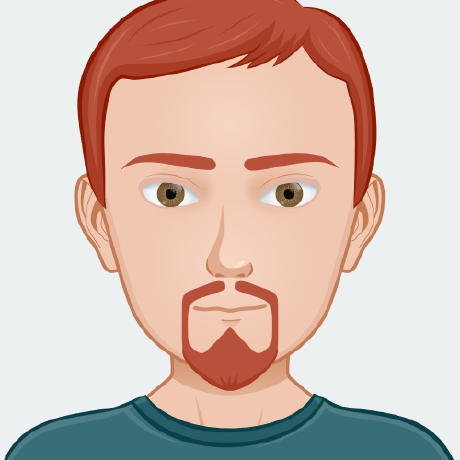
xstateEx
@frankysnow
// Forked from @stevekrouse.xstateEx
Script
let { xstateStateEx } = await import("https://esm.town/v/frankysnow/xstateStateEx");
export const xstateEx = async (event: string) => {
const { createMachine } = await import("npm:xstate");
const toggleMachine = createMachine({
id: "toggle",
initial: "inactive",
states: {
inactive: { on: { TOGGLE: "active" } },
active: { on: { TOGGLE: "inactive" } },
let state = xstateStateEx || toggleMachine.initialState;
form_handler
@andreterron
Receive form responses Live demo: https://andreterron-form_demo.web.val.run/ Create a form that posts to your val: <form action="https://andreterron-form_handler.web.val.run" method="post">
<label for="username">Val Town username:</label>
<input name="username" type="text">
<button type="submit">Submit</button>
</form> And get your results stored as an array: // set by andreterron.form_handler at 2023-08-10T21:04:43.364Z
let formResponses = [{
"username": "andreterron",
},
{
"username": "stevekrouse",
}]; Usage Fork this val and click the 🔒 to set it as "Unlisted" Open the val menu → Endpoints → "Copy web endpoint" Use that url as the action attribute of your form Check out the example val: https://www.val.town/v/andreterron.form_demo Storage of form submissions This val saves to another val ( @me.formResponse ), which has a 100kb limitation (250kb for pro users), if you want to store them in a more scalable solution, check out our guides
HTTP
# Receive form responses
Live demo: https://andreterron-form_demo.web.val.run/
Create a form that posts to your val:
```html
<form action="https://andreterron-form_handler.web.val.run" method="post">
<label for="username">Val Town username:</label>
let { formResponses } = await import("https://esm.town/v/andreterron/formResponses");
export async function form_handler(req: Request) {
const data: any = Object.fromEntries((await req.formData()).entries());
formResponses = (formResponses ?? []).concat(
aquamarineTurtle
@iamseeley
📄 hello, resume Creating, customizing, and hosting resumes can get complicated and time-consuming. This project aims to simplify that process and maybe make it a little more enjoyable. Follow the steps in your resumeConfig to get started . Happy job hunting! 💼✨ Thanks to @nbbaier for the great feedback and resumeValidator !
HTTP
## 📄 hello, resume
Creating, customizing, and hosting resumes can get complicated and time-consuming. This project aims to simplify that process and maybe make it a little more enjoyable.
*Follow the steps in your **resumeConfig** to get started*.
Happy job hunting! 💼✨
Thanks to [@nbbaier](https://www.val.town/u/nbbaier) for the great feedback and [resumeValidator](https://www.val.town/v/nbbaier/resumeValidator)!
export default async function resumeHandler(req: Request): Promise<Response> {
if (req.method === 'GET') {
try {
const config = await resumeSetup(resumeConfig);
if (!config.resumeDetails || Object.keys(config.resumeDetails).length === 0) {
blink_create_tree
@nickfrosty
* Solana Actions Example
Script
* Solana Actions Example
ActionPostResponse,
ACTIONS_CORS_HEADERS,
createPostResponse,
ActionGetResponse,
ActionPostRequest,
} from "@solana/actions";
createNoopSigner,
createSignerFromKeypair,
generateSigner,
naclValidate
@demo
An interactive, runnable TypeScript val by demo
Script
import { Buffer } from "node:buffer";
export let naclValidate = async (publicKey, signature, timestamp, body) => {
const { default: nacl } = await import("npm:tweetnacl@1.0.3");
const isVerified = nacl.sign.detached.verify(
Buffer.from(timestamp + body),
Buffer.from(signature, "hex"),
Buffer.from(publicKey, "hex"),
return isVerified;
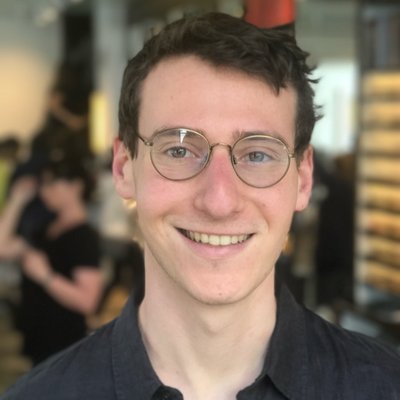
createVal
@stevekrouse
An interactive, runnable TypeScript val by stevekrouse
Script
export interface ValResponse {
id: string;
author: {
id: string;
username: string;
name: string;
code: string;
public: boolean;
privacy: "public" | "unlisted" | "private";
version: number;
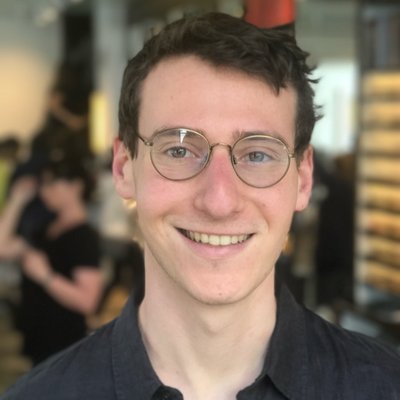
harlequinCobra
@stevekrouse
An interactive, runnable TypeScript val by stevekrouse
Script
export async function getWeather(city: string): Promise<WeatherResponse> {
return fetchJSON(`https://wttr.in/${city}?format=j1`);
export interface WeatherResponse {
current_condition: CurrentCondition[];
nearest_area: NearestArea[];
request: Request[];
weather: Weather[];
export interface CurrentCondition {
FeelsLikeC: string;
FeelsLikeF: string;
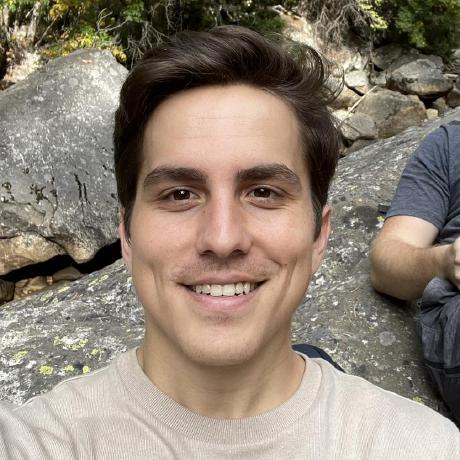
TodayForAnnalisa
@mttlws
An interactive, runnable TypeScript val by mttlws
Express (deprecated)
export async function TodayForAnnalisa(req: express.Request, res: express.Response) {
const { activity } = await fetchJSON(
"https://www.boredapi.com/api/activity",
return res.send(`
<html>
<head>
<title>Today for Annalisa 🥹</title>
<link rel="icon" type="image/svg+xml" href="data:image/svg+xml;charset=UTF-8,%3Csvg%20xmlns%3D%22http%3A%2F%2Fwww.w3.org%2F2000%2Fsvg%22%20viewBox%3D%220%200%2024%2024%22%20fill%3D%22none%22%20stroke%3D%22currentColor%22%20stroke-width%3D%222%22%20stroke-linecap%3D%22round%22%20stroke-linejoin%3D%22round%22%3E%3Ccircle%20cx%3D%2212%22%20cy%3D%2212%22%20r%3D%2210%22%3E%3C%2Fcircle%3E%3Cline%20x1%3D%2212%22%20y1%3D%2216%22%20x2%3D%2212%22%20y2%3D%2212%22%3E%3C%2Fline%3E%3Cline%20x1%3D%2212%22%20y1%3D%2212%22%20x2%3D%2216%22%20y2%3D%2212%22%3E%3C%2Fline%3E%3Cline%20x1%3D%2212%22%20y1%3D%2212%22%20x2%3D%2210%22%20y2%3D%2212%22%3E%3C%2Fline%3E%3C%2Fsvg%3E" />
<meta name="description" content="Today for Annalisa 🥹" />
<meta name="description" content="Today for Annalisa 🥹" />