Search
EnkakuStatelessHTTPExample
@paul_lecam
An interactive, runnable TypeScript val by paul_lecam
HTTP
const transport = new ServerTransport();
serve({
public: true,
transport,
handlers: {
"example:event": (ctx) => {
console.log("received event:", ctx.data);
"example:request": () => {
console.log("received request");
return { test: true };
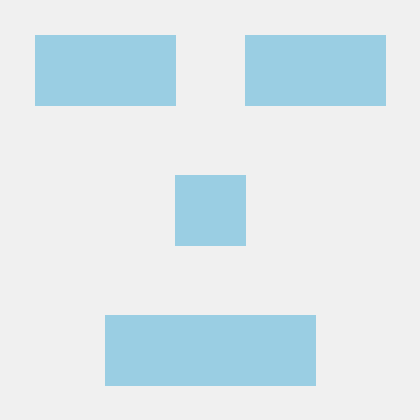
myTestForm
@sethdijkstra
// set by sethdijkstra.handleFormExample2 at 2023-04-25T08:38:19.257Z
Script
// set by sethdijkstra.handleFormExample2 at 2023-04-25T08:38:19.257Z
export let myTestForm = [{
"name": "Howdy",
"email": "Yoooo@icloud.com",
"message": "asdfassdafas"
"name": "Seth",
"email": "seth@dijkjdfs.com",
"message": "Thankyou"
"name": "set",
"email": "seth@dijkjdfs.com",
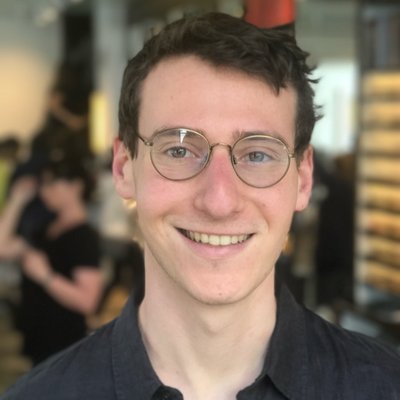
FetchBasic
@stevekrouse
Framer Fetch: Basic A basic example of an API endpoint to use with Framer Fetch. CORS headers are permissive by default on Val Town, so no need to set them.
HTTP
# Framer Fetch: Basic
A basic example of an API endpoint to use with Framer Fetch. CORS headers are permissive by default on Val Town, so no need to set them.
export default async function(req: Request): Promise<Response> {
// Pick a random greeting
const greetings = ["Hello!", "Welcome!", "Hi!", "Heya!", "Hoi!"];
const index = Math.floor(Math.random() * greetings.length);
return Response.json({ data: greetings[index] });
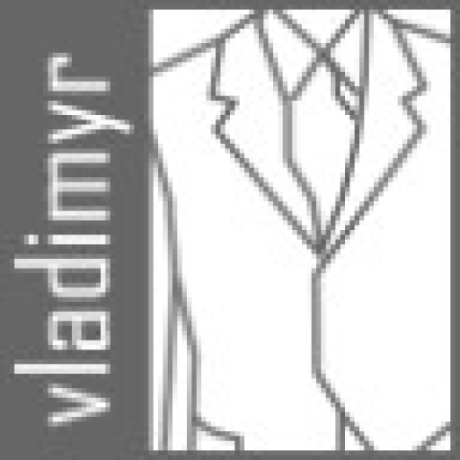
pipeResponse
@vladimyr
// SPDX-License-Identifier: 0BSD
Script
// SPDX-License-Identifier: 0BSD
export type RequestHandler = (req?: Request) => Response | Promise<Response>;
export function createWrappedHandler(
handler: RequestHandler,
...transforms: ReadableWritablePair[]
): RequestHandler {
return async (req?: Request) => {
const res = await handler(req);
return pipeResponse(res, ...transforms);
export function pipeResponse(
resumeHandler
@siygle
📄 hello, resume Creating, customizing, and hosting resumes can get complicated and time-consuming. This project aims to simplify that process and maybe make it a little more enjoyable. Follow the steps in your resumeConfig to get started . Happy job hunting! 💼✨ Thanks to @nbbaier for the great feedback and resumeValidator ! Also, big thanks to Thomas Davis for JSON Resume Standard!
HTTP
## 📄 hello, resume
Creating, customizing, and hosting resumes can get complicated and time-consuming. This project aims to simplify that process and maybe make it a little more enjoyable.
*Follow the steps in your **resumeConfig** to get started*.
Happy job hunting! 💼✨
Thanks to [@nbbaier](https://www.val.town/u/nbbaier) for the great feedback and [resumeValidator](https://www.val.town/v/nbbaier/resumeValidator)!
Also, big thanks to [Thomas Davis](https://github.com/thomasdavis) for JSON Resume Standard!
export default async function resumeHandler(req: Request): Promise<Response> {
if (req.method === "GET") {
try {
const config = await resumeSetup(resumeConfig);
createVal
@mharris717
An interactive, runnable TypeScript val by mharris717
Script
export interface ValResponse {
id: string;
author: {
id: string;
username: string;
name: string;
code: string;
public: boolean;
privacy: "public" | "unlisted" | "private"; // Added privacy type
version: number;
hnTopStory
@whatrocks
An interactive, runnable TypeScript val by whatrocks
Cron
export async function hnTopStory() {
const topStories: Number[] = await fetch(
"https://hacker-news.firebaseio.com/v0/topstories.json?print=pretty"
).then((res) => res.json());
const id = topStories[0];
const story: {
title: string;
url: string;
time: number;
type: string;
wonderfulAquaEmu
@Akshat1236
An interactive, runnable TypeScript val by Akshat1236
HTTP
export default async function(req: Request): Promise<Response> {
return Response.json({ ok: true });
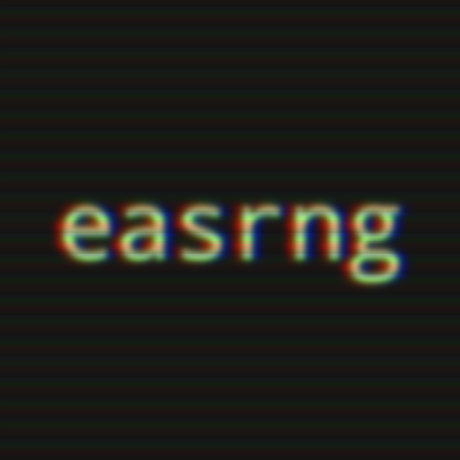
generateKeys
@easrng
An interactive, runnable TypeScript val by easrng
Script
import { Buffer } from "node:buffer";
export async function generateKeys() {
const { default: nacl } = await import("npm:tweetnacl@1.0.3");
const keyPair = nacl.sign.keyPair();
return {
publicKey: Buffer.from(keyPair.publicKey).toString("base64"),
secretKey: Buffer.from(keyPair.secretKey).toString("base64"),
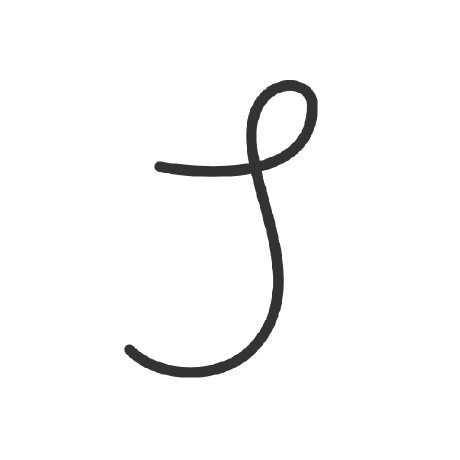
csp
@jshawl
An interactive, runnable TypeScript val by jshawl
Script
export async function csp(url) {
const response = await fetch(url);
const policies = response.headers.get("Content-Security-Policy").split(";")
.map((p) => p.trim()).filter(String);
return policies.reduce((acc, el) => {
const spaceIndex = el.indexOf(" ");
const directive = el.slice(0, spaceIndex);
const values = el.slice(spaceIndex + 1, el.length);
acc[directive] = values.split(" ");
return acc;
codeOnValTown
@AIWB
Code on Val Town Adds a "Code on Val Town" ribbon to your page. This lets your website visitors navigate to the code behind it. This uses github-fork-ribbon-css under the hood. Usage Here are 2 different ways to add the "Code on Val Town" ribbon: 1. Wrap your fetch handler (recommended) import { modifyFetchHandler } from "https://esm.town/v/andreterron/codeOnValTown?v=50";
import { html } from "https://esm.town/v/stevekrouse/html?v=5";
export default modifyFetchHandler(async (req: Request): Promise<Response> => {
return html(`<h2>Hello world!</h2>`);
}); Example: @andreterron/openable_handler 2. Wrap your HTML string import { modifyHtmlString } from "https://esm.town/v/andreterron/codeOnValTown?v=50";
import { html } from "https://esm.town/v/stevekrouse/html?v=5";
export default async (req: Request): Promise<Response> => {
return html(modifyHtmlString(`<h2>Hello world!</h2>`));
}; Example: @andreterron/openable_html Other ways We made sure this was very modular, so you can also add the ribbon using these methods: Get the element string directly: @andreterron/codeOnVT_ribbonElement Modify an HTTP Response: @andreterron/codeOnVT_modifyResponse Use .pipeThrough to append to a stream: @andreterron/InjectCodeOnValTownStream Customization Linking to the val These functions infer the val using the call stack or the request URL. If the inference isn't working, or if you want to ensure it links to a specific val, pass the val argument: modifyFetchHandler(handler, {val: { handle: "andre", name: "foo" }}) modifyHtmlString("<html>...", {val: { handle: "andre", name: "foo" }}) Styling You can set the style parameter to a css string to customize the ribbon. Check out github-fork-ribbon-css to learn more about how to style the element. modifyFetchHandler(handler, {style: ".github-fork-ribbon:before { background-color: #333; }"}) modifyHtmlString("<html>...", {style: ".github-fork-ribbon:before { background-color: #333; }"}) Here's how you can hide the ribbon on small screens: modifyFetchHandler(handler, {style: `@media (max-width: 768px) {
.github-fork-ribbon {
display: none !important;
}
}`}) To-dos [ ] Let users customize the ribbon. Some ideas are the text, color or placement.
Script
# Code on Val Town
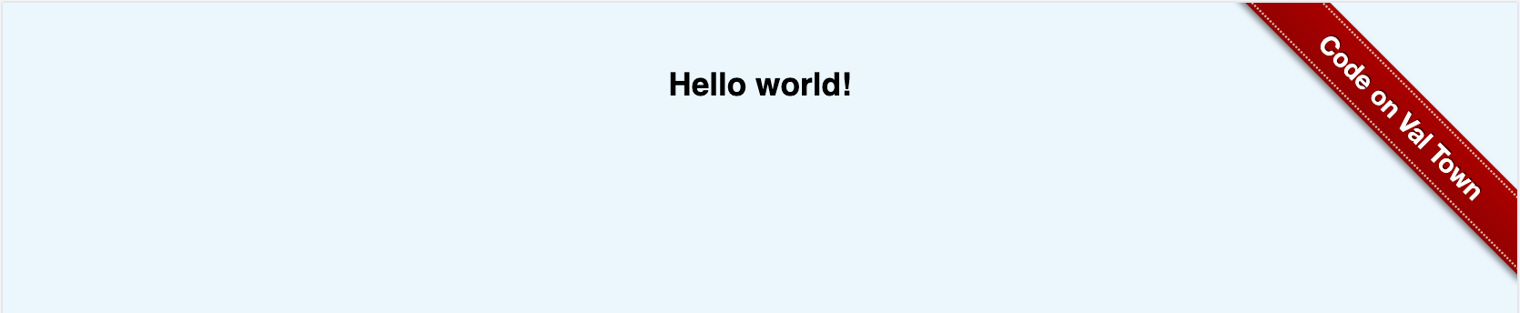
Adds a "Code on Val Town" ribbon to your page. This lets your website visitors navigate to the code behind it.
This uses [github-fork-ribbon-css](https://github.com/simonwhitaker/github-fork-ribbon-css) under the hood.
## Usage
Here are 2 different ways to add the "Code on Val Town" ribbon:
* @param bodyText HTML string that will be used to inject the element.
* @param val Define which val should open. Defaults to the root reference
export function modifyHtmlString(
bodyText: string,
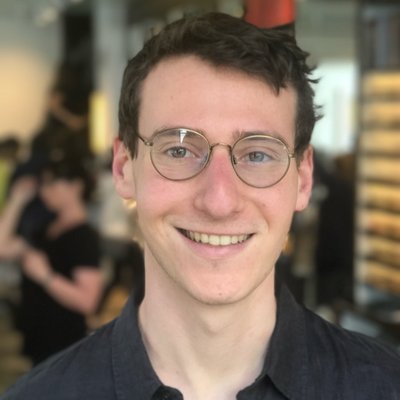
politelyinvinciblepointerHandleFormEx
@stevekrouse
An interactive, runnable TypeScript val by stevekrouse
Script
import { fetch } from "https://esm.town/v/std/fetch";
export const politelyinvinciblepointerHandleFormEx = (async () => {
let body = { args: [{ email: "example@example.com" }] };
const response =
(await fetch(
"https://api.val.town/v1/run/politelyinvinciblepointer.handleForm",
method: "POST",
body: JSON.stringify(body),
)).json();
console.log(response);
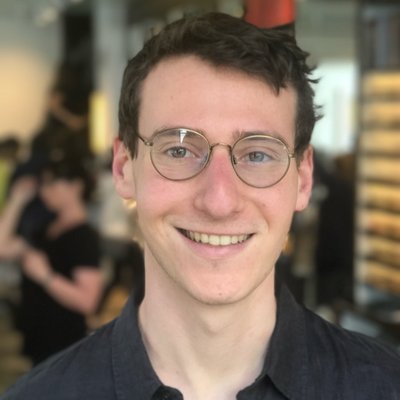
hello_world
@stevekrouse
An interactive, runnable TypeScript val by stevekrouse
HTTP
export default async function(req: Request): Promise<Response> {
return Response.json("hello world!");