Search
emailRandomJoke
@tylerbinns
// Fetches a random joke.
Cron
// Fetches a random joke.
async function fetchRandomJoke() {
const response = await fetch(
"https://official-joke-api.appspot.com/random_joke",
return response.json();
const randomJoke = await fetchRandomJoke();
const setup = randomJoke.setup;
const punchline = randomJoke.punchline;
// Sends an email with the joke.
export const emailRandomJoke = email({
crazyPeachCrayfish
@MichaelNollox
Code on Val Town Adds a "Code on Val Town" ribbon to your page. This lets your website visitors navigate to the code behind it. This uses github-fork-ribbon-css under the hood. Usage Here are 2 different ways to add the "Code on Val Town" ribbon: 1. Wrap your fetch handler (recommended) import { modifyFetchHandler } from "https://esm.town/v/andreterron/codeOnValTown?v=50";
import { html } from "https://esm.town/v/stevekrouse/html?v=5";
export default modifyFetchHandler(async (req: Request): Promise<Response> => {
return html(`<h2>Hello world!</h2>`);
}); Example: @andreterron/openable_handler 2. Wrap your HTML string import { modifyHtmlString } from "https://esm.town/v/andreterron/codeOnValTown?v=50";
import { html } from "https://esm.town/v/stevekrouse/html?v=5";
export default async (req: Request): Promise<Response> => {
return html(modifyHtmlString(`<h2>Hello world!</h2>`));
}; Example: @andreterron/openable_html Other ways We made sure this was very modular, so you can also add the ribbon using these methods: Get the element string directly: @andreterron/codeOnVT_ribbonElement Modify an HTTP Response: @andreterron/codeOnVT_modifyResponse Use .pipeThrough to append to a stream: @andreterron/InjectCodeOnValTownStream Customization Linking to the val These functions infer the val using the call stack or the request URL. If the inference isn't working, or if you want to ensure it links to a specific val, pass the val argument: modifyFetchHandler(handler, {val: { handle: "andre", name: "foo" }}) modifyHtmlString("<html>...", {val: { handle: "andre", name: "foo" }}) Styling You can set the style parameter to a css string to customize the ribbon. Check out github-fork-ribbon-css to learn more about how to style the element. modifyFetchHandler(handler, {style: ".github-fork-ribbon:before { background-color: #333; }"}) modifyHtmlString("<html>...", {style: ".github-fork-ribbon:before { background-color: #333; }"}) Here's how you can hide the ribbon on small screens: modifyFetchHandler(handler, {style: `@media (max-width: 768px) {
.github-fork-ribbon {
display: none !important;
}
}`}) To-dos [ ] Let users customize the ribbon. Some ideas are the text, color or placement.
Script
# Code on Val Town
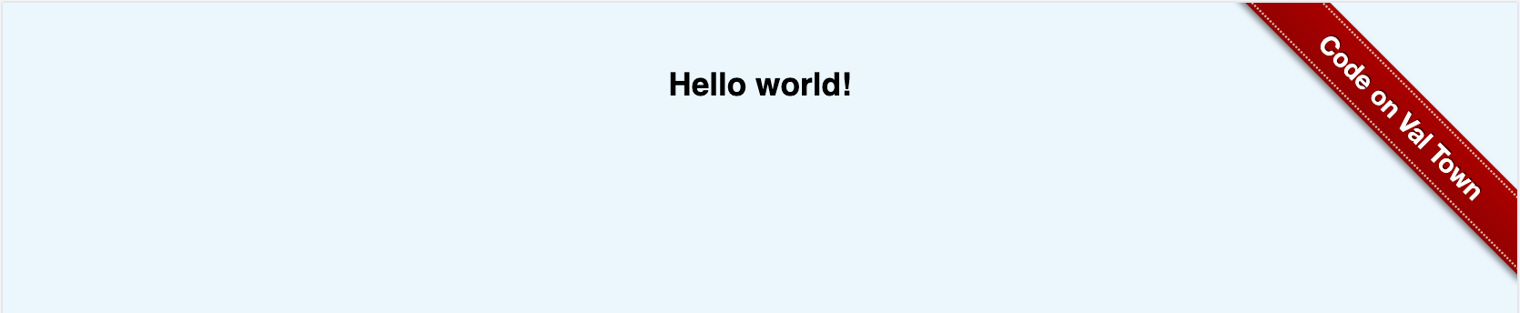
Adds a "Code on Val Town" ribbon to your page. This lets your website visitors navigate to the code behind it.
This uses [github-fork-ribbon-css](https://github.com/simonwhitaker/github-fork-ribbon-css) under the hood.
## Usage
Here are 2 different ways to add the "Code on Val Town" ribbon:
* @param bodyText HTML string that will be used to inject the element.
* @param val Define which val should open. Defaults to the root reference
export function modifyHtmlString(
bodyText: string,
Mauritius
@sun
An interactive, runnable TypeScript val by sun
Script
export const Mauritius = "Hello from MrSunshyne";
myApi
@ubuwaits
An interactive, runnable TypeScript val by ubuwaits
Script
export function myApi(name) {
return "hi " + name;
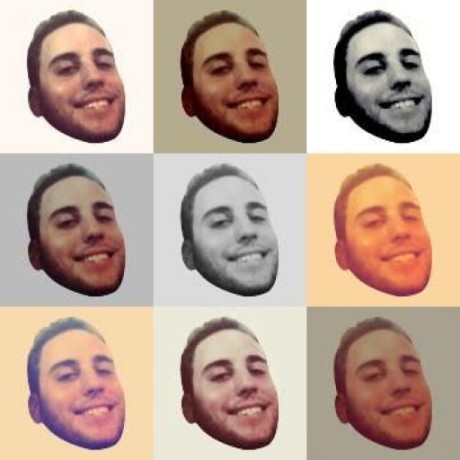
weddingAPI
@schwanksta
Takes in a few variables and writes it to a Google Sheet, sending an email each time. I'm using this to send this information
to an RSVP sheet.
RPC
/* Takes in a few variables and writes it to a Google Sheet,
sending an email each time. I'm using this to send this information
to an RSVP sheet. */
export let weddingAPI = (guest, yesno, count, additional, email) => {
const gsheet_append_example = gsheet_call(
process.env.google_service_account, // This is the file you download from the service account in Google. Just paste it in.
"1lYEDpX63DL12oGBnZ_rTafZxzRRF3jpkvv_Leu0qeqQ", // Sheet ID
"values/A2:D2:append?valueInputOption=RAW", // Append starting after the header
{ values: [[guest, yesno, count, additional, email]] }
return console.email(
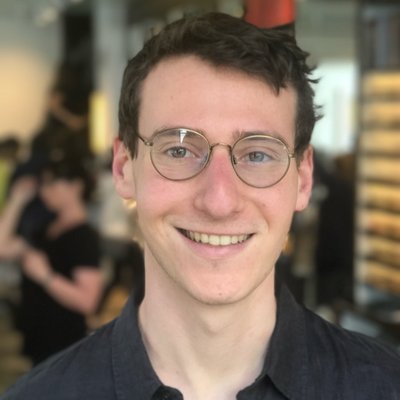
euler2
@stevekrouse
An interactive, runnable TypeScript val by stevekrouse
Script
import { fibs } from "https://esm.town/v/stevekrouse/fibs";
import { sum } from "https://esm.town/v/stevekrouse/sum";
export let euler2 = sum(fibs(30).filter(f => f < 4e6 && f % 2 === 0))
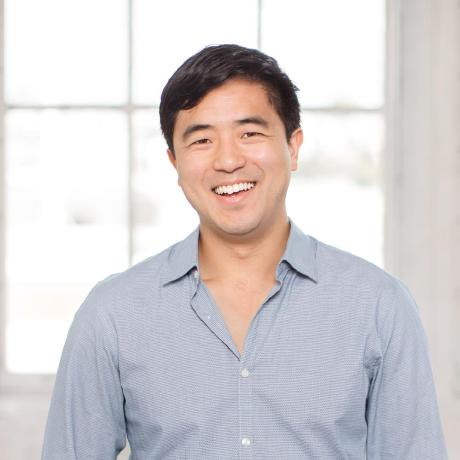
chatAgentWithCustomPrompt
@jacoblee93
An interactive, runnable TypeScript val by jacoblee93
Script
export const chatAgentWithCustomPrompt = (async () => {
const { ChatOpenAI } = await import(
"https://esm.sh/langchain/chat_models/openai"
const { initializeAgentExecutorWithOptions } = await import(
"https://esm.sh/langchain/agents"
const { Calculator } = await import(
"https://esm.sh/langchain/tools/calculator"
const model = new ChatOpenAI({
temperature: 0,
openAIApiKey: process.env.OPENAI_API_KEY,
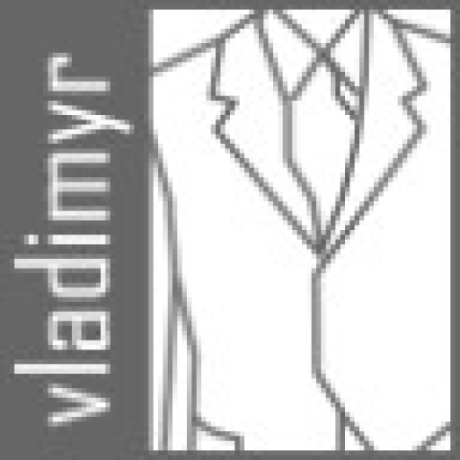
newValURL_example
@vladimyr
// ==> https://www.val.town/new?code64=aW1wb3J0IHsgYmFzZTY0IGFzIGI2NCB9IGZyb20gIm5wbTptdWx0aWZvcm1hdHMvYmFzZXMvYmFzZTY0IjsKCmV4cG9ydCBmdW5jdGlvbiBuZXdWYWxVUkwoY29kZTogc3RyaW5nLCBiYXNlNjQgPSB0cnVlKSB7CiAgY29uc3QgdXJsID0gbmV3IFVSTCgiaHR0cHM6Ly93d3cudmFsLnRvd24vbmV3Iik7CiAgaWYgKCFiYXNlNjQpIHsKICAgIHVybC5zZWFyY2hQYXJhbXMuc2V0KCJjb2RlIiwgY29kZSk7CiAgICByZXR1cm4gdXJsOwogIH0KICAvLyBAc2VlOiBodHRwczovL3d3dy52YWwudG93bi92L2Vhc3JuZy9wbGF5Z3JvdW5kP3Y9MzQzI0w3MDMtNzA0CiAgY29uc3QgYnl0ZXMgPSBuZXcgVGV4dEVuY29kZXIoKS5lbmNvZGUoY29kZSk7CiAgdXJsLnNlYXJjaFBhcmFtcy5zZXQoImNvZGU2NCIsIGI2NC5iYXNlRW5jb2RlKGJ5dGVzKSk7CiAgcmV0dXJuIHVybDsKfQ
Script
import { newValURL } from "https://esm.town/v/vladimyr/newValURL";
const { code } = await fetchVal("vladimyr", "newValURL");
const valURL = newValURL(code);
console.log(valURL.href);
// ==> https://www.val.town/new?code64=aW1wb3J0IHsgYmFzZTY0IGFzIGI2NCB9IGZyb20gIm5wbTptdWx0aWZvcm1hdHMvYmFzZXMvYmFzZTY0IjsKCmV4cG9ydCBmdW5jdGlvbiBuZXdWYWxVUkwoY29kZTogc3RyaW5nLCBiYXNlNjQgPSB0cnVlKSB7CiAgY29uc3QgdXJsID0gbmV3IFVSTCgiaHR0cHM6Ly93d3cudmFsLnRvd24vbmV3Iik7CiAgaWYgKCFiYXNlNjQpIHsKICAgIHVybC5zZWFyY2hQYXJhbXMuc2V0KCJjb2RlIiwgY29kZSk7CiAgICByZXR1cm4gdXJsOwogIH0KICAvLyBAc2VlOiBodHRwczovL3d3dy52YWwudG93bi92L2Vhc3JuZy9wbGF5Z3JvdW5kP3Y9MzQzI0w3MDMtNzA0CiAgY29uc3QgYnl0ZXMgPSBuZXcgVGV4dEVuY29kZXIoKS5lbmNvZGUoY29kZSk7CiAgdXJsLnNlYXJjaFBhcmFtcy5zZXQoImNvZGU2NCIsIGI2NC5iYXNlRW5jb2RlKGJ5dGVzKSk7CiAgcmV0dXJuIHVybDsKfQ
const expiringShortURL = await shortenURL(valURL);
console.log(expiringShortURL.href);
// ==> https://dub.sh/<key>
load_new_subreddit_posts
@bnorick
An interactive, runnable TypeScript val by bnorick
Script
export let load_new_subreddit_posts = async ({
subreddit,
filters,
after_utc,
fetch_limit,
let patterns = [];
for (const pattern_def of filters) {
let pattern_str, mode;
if (typeof pattern_def === "string") {
pattern_str = pattern_def;
stripFrontmatter
@nbbaier
utility to strip yaml frontmatter from a md file
Script
utility to strip yaml frontmatter from a md file
import { matter } from "npm:vfile-matter";
export async function stripMetadata(markdown: string) {
const file = await unified()
.use(remarkParse)
.use(remarkStringify)
.use(remarkFrontmatter)
.process(markdown);
matter(file, { strip: true });
return String(file);
substrateBadge
@substrate
Render this badge: Use the middleware: https://www.val.town/v/substrate/substrateBadgeMiddleware Or: import substrateBadge from "https://esm.town/v/substrate/substrateBadge";
export default async function(req: Request): Promise<Response> {
const badge = substrateBadge(import.meta.url);
const html = `
<h1>Hello, world</h1>
${badge}
`;
return new Response(html, {
headers: {
"Content-Type": "text/html; charset=utf-8",
},
});
}
HTTP (deprecated)
Render this badge:

Use the middleware: https://www.val.town/v/substrate/substrateBadgeMiddleware
Or:
```tsx
export default async function(req: Request): Promise<Response> {
// substrate + valtown badge
const left = 80;
const width = 160;
const height = 48; // width * 0.3;
aocDay1
@nbbaier
Advent of Code 2023 - Day 1 solutions
Script
## Advent of Code 2023 - Day 1 solutions
// #folder:aoc2023
// @aoc2023
// @title Day 1 solutions
const data = await getAocData(1);
const input = (await data.text()).split("\n").slice(0, -1);
const numberMap: Map<string, number> = new Map<string, number>([
["one", 1],
["two", 2],
["three", 3],
aqi
@antoniojtorres
AQI Alerts Get email alerts when AQI is unhealthy near you. Set up Click Fork Change location (Line 4) to describe your location. It accepts fairly flexible English descriptions which it turns into locations via nominatim's geocoder API . Click Run Background This val uses nominatim's geocoder to get your lat, lon, and air quality data from OpenAQ. It uses EPA's NowCast
AQI Index calculation and severity levels. Learn more: https://www.val.town/v/stevekrouse.easyAQI
Cron
# AQI Alerts
Get email alerts when AQI is unhealthy near you.

## Set up
1. Click `Fork`
2. Change `location` (Line 4) to describe your location. It accepts fairly flexible English descriptions which it turns into locations via [nominatim's geocoder API](https://www.val.town/v/stevekrouse/nominatimSearch).
export async function aqi(interval: Interval) {
const location = "los angeles california"; // <-- change to place, city, or zip code
const data = await easyAQI({ location });
console.log("Quality:", data);
handleDiscordInteraction
@jamisonl
Bot for Cama discord server. To initialize new slash commands, you have to run a separate bit of code. This is for modifying their functionality
HTTP (deprecated)
Bot for Cama discord server. To initialize new slash commands, you have to run a separate bit of code. This is for modifying their functionality
let bank = await blob.getJSON("bank");
let bets = await blob.getJSON("bets");
const starting_amount = 5;
if (!bank) {
bank = {};
await blob.setJSON("bank", bank);
if (!bets) {
bets = [];
await blob.setJSON("bets", bets);