Search
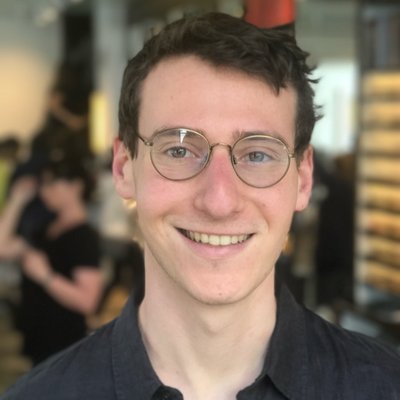
groupBy
@stevekrouse
! * Group items from an array together by some criteria or value.
* (c) 2019 Tom Bremmer (https://tbremer.com/) and Chris Ferdinandi (https://gomakethings.com), MIT License,
* @param {Array} arr The array to group items from
* @param {String|Function} criteria The criteria to group by
* @return {Object} The grouped object
Script
* @param {Array} arr The array to group items from
* @param {String|Function} criteria The criteria to group by
* @return {Object} The grouped object
export function groupBy<A>(arr: A[], criteria: ((a: A) => string) | string) {
return arr.reduce(function (obj, item) {
// Check if the criteria is a function to run on the item or a property of it
var key = typeof criteria === "function" ? criteria(item) : item[criteria];
// If the key doesn't exist yet, create it
valTownBadgeSVG
@jxnblk
SVG Val Town badge image service
HTTP
const rounded = 8;
const padLeft = 48;
export function Badge({
scale = 1,
label = "View source on",
fill="#fff"
</svg>
export default async function(req: Request): Promise<Response> {
const svg = renderToStaticMarkup(
<Badge scale={1} />,
countArray
@nbbaier
Example usage: import countArray from "https://esm.town/v/nbbaier/countArray";
const arr = ["a", "b", "c", "d", "d", "a", "a"];
const counted = countArray(arr, "item");
// counted =
// [
// { item: "a", count: 3 },
// { item: "b", count: 1 },
// { item: "c", count: 1 },
// { item: "d", count: 2 }
// ]
Script
* @param {K} eventualKey - The key used to identify unique items in the array.
* @returns {Prettify<{ count: number } & Record<K, T>>[]} An array of objects with the count and the item.
export default function countArray<T, K extends string>(
arr: T[],
eventualKey: K,
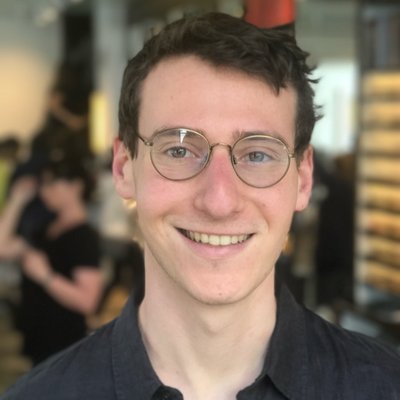
stormyCyanLeopon
@stevekrouse
Send Chunked Discord Message This function is used to send a message to a Discord webhook. If the message exceeds the maximum character limit (2000 characters), it will be divided into chunks and sent as multiple messages. Parameters message (string): The message to be sent to the Discord webhook. Return Value This function does not return any value. Example Usage: const message = "This is a long message that needs to be sent to Discord. It may exceed the character limit, so it will be divided into smaller chunks.";
await @ktodaz.sendDiscordMessage(message); In the above example, the sendDiscordMessage function is used to send the message to the Discord webhook. If the message exceeds 2000 characters, it will be split into smaller chunks and sent as separate messages. Required Secrets: Ensure you have added the secret for discord_webhook in your val.town secrets settings (Profile -> Secrets)
Script
# Send Chunked Discord Message
This function is used to send a message to a Discord webhook. If the message exceeds the maximum character limit (2000 characters), it will be divided into chunks and sent as multiple messages.
### Parameters
### Return Value
This function does not return any value.
## Example Usage:
await @ktodaz.sendDiscordMessage(message);
In the above example, the sendDiscordMessage function is used to send the message to the Discord webhook. If the message exceeds 2000 characters, it will be split into smaller chunks and sent as separate messages.
## Required Secrets:
webhook_url: string = process.env.discord_webhook,
function chunkString(str) {
const chunks = [];
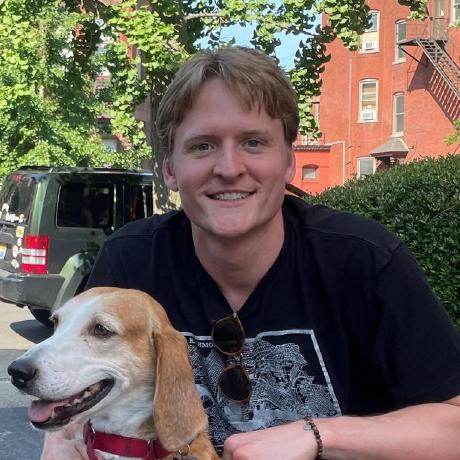
unsubscribeFromNewsletter
@petermillspaugh
Val Town email subscriptions: unsubscribe Cousin Val to @petermillspaugh/emailSubscription — see docs there.
HTTP
import { email as sendEmail } from "https://esm.town/v/std/email?v=11";
import { sqlite } from "https://esm.town/v/std/sqlite?v=4";
export async function unsubscribe(req: Request) {
const searchParams = new URL(req.url).searchParams;
const emailAddress = searchParams.get("email");
weekday
@xkonti
An interactive, runnable TypeScript val by xkonti
Script
Fri: 5,
Sat: 6,
export function getWeekdaysInMonth(year: number, month: number, weekday: Weekday): Date[] {
const dates: Date[] = [];
const date = new Date(year, month, 1);
grayLimpet
@samk
BTC Price Alert This val monitors the price of Bitcoin (BTC) and sends an email alert if the price fluctuates significantly. Specifically, it checks the current BTC price against the last recorded price and triggers an email notification if the change exceeds 20%. The email includes the new price, formatted as currency. Fork this val to get these notifications on your inbox.
Cron
import { email } from "https://esm.town/v/std/email?v=9";
import { currency } from "https://esm.town/v/stevekrouse/currency";
export async function btcPriceAlert() {
const lastBtcPrice: number = await blob.getJSON("lastBtcPrice");
let btcPrice = await currency("usd", "btc");
setCorsHeaders
@nthypes
An interactive, runnable TypeScript val by nthypes
Script
"Access-Control-Allow-Methods": "GET,HEAD,PUT,PATCH,POST,DELETE",
} as const;
export default function setCorsHeaders(
response: Response,
headers: Partial<typeof DEFAULT_CORS_HEADERS> = DEFAULT_CORS_HEADERS,
abortable
@postpostscript
// SPDX-License-Identifier: Apache-2.0
Script
// SPDX-License-Identifier: Apache-2.0
import type { MaybePromise } from "./types/util.ts";
export function abortable<T>(promise: MaybePromise<T>, signal?: AbortSignal): Promise<T> {
if (signal?.aborted) {
return Promise.reject(
new AbortError("signal aborted", {
cause: signal,
function cleanup() {
signal!.removeEventListener("abort", handler);
signal.addEventListener("abort", handler);
type_Capitalize_Uppercase
@jkbno
// Basic recursion type
Script
// ReturnType is a utility type that extracts the return type of a function.
// In this definition, T is a function type that takes any number of arguments and returns any type.
// Using the infer keyword, we create a temporary type variable R to represent the return type of the function. If T is a function, TypeScript infers the return type and assigns it to R.
function greet(name: string): string {
// Here, ReturnType is used to infer the return type of the greet function, which is string.
// This type extracts the parameter types of a function as a tuple. The definition of Parameters is as follows:
// In this example, we create a temporary type variable P to represent the parameter types of the function.
// If T is a function, TypeScript infers the parameter types and assigns them to P as a tuple.
function add(a: number, b: number): number {
// Here, Parameters is used to infer the parameter types of the add function, which is a tuple [number, number].
probableMaroonGuan
@tempdev
An interactive, runnable TypeScript val by tempdev
Script
[key: string]: string | Function;
setItem: Function;
setItem: function(item: string, value: string) {
function get(index: number) {
function getMemBuff(): Uint8Array {
const encode = function(text: string, array: Uint8Array) {
function parse(text: string, func: Function, func2: Function) {
function isNull(test: any) {
function getArr32() {
function shift(QP: number) {
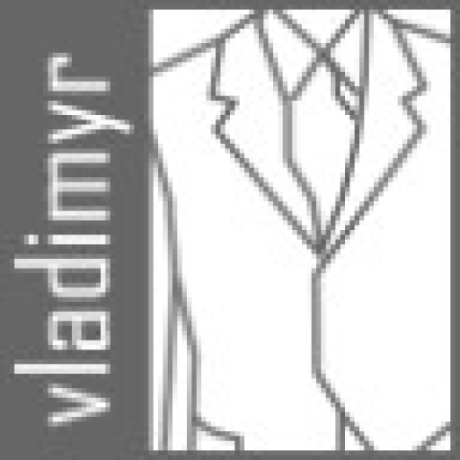
CFDate
@vladimyr
// SPDX-License-Identifier: 0BSD
Script
const COCOA_REF_DATE = new Date(Date.UTC(2001, 0, 1, 0, 0, 0, 0));
// @see: https://developer.apple.com/documentation/corefoundation/1542812-cfdategetabsolutetime#discussion
export function toDate(cocoaTimestamp: number) {
return new Date(COCOA_REF_DATE.getTime() + cocoaTimestamp * 1000);
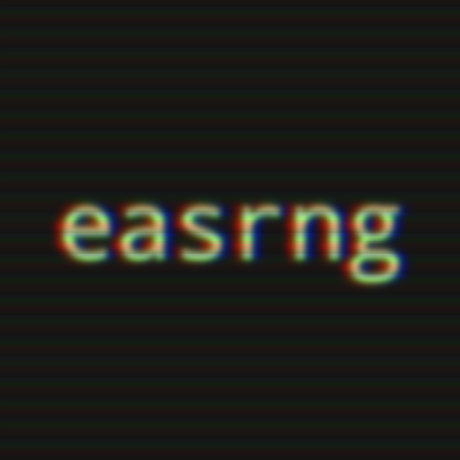
denoMediaTypes
@easrng
/// Definition files don't have separate content types and so we have to "guess"
Script
function map_typescript_like(
export function as_ts_extension(type: MediaType): string {
export function as_content_type(type: MediaType): string | undefined {
export function is_declaration(type: MediaType): type is MediaType.Dts | MediaType.Dcts | MediaType.Dmts {
export function from_response(response: Response) {
export function from_url_and_content_type(
export function from_content_type(
export function from_url(url: URL): MediaType {
export function from_path(path: string): MediaType {
function map_js_like_extension(
computeScheduleSegment
@xkonti
computeScheduleSegment function Calculates the on/off time spans for a given schedule segment based on the duty cycle. Parameters: segment -A ScheduleSegment object describing the schedule. Returns: An array of TimeSpan objects representing the on times. TimeSpan type Represents a span of time with a starting point and a duration in minutes. DutyCycle type Represents the "on" state scheduling pattern for a device. It's similar to the concept of Pulse-Width Modulation (PWM). ScheduleSegment type A part of a complete schedule, describing a time frame with a specified duty cycle.
Script
# `computeScheduleSegment` function
Calculates the on/off time spans for a given schedule segment based on the duty cycle.
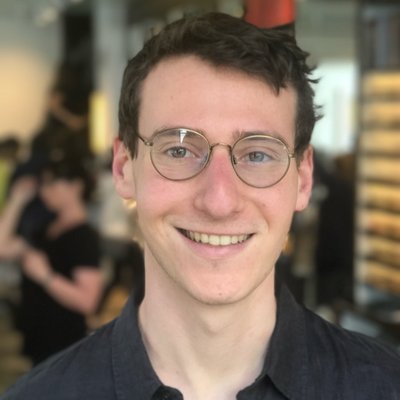
taxrates
@stevekrouse
// This approach calculates taxes based on the selected filing status from the URL.
HTTP
filingStatus: string;
function parseCsv(csv: string): TaxBracket[] {
const lines = csv.trim().split("\n");
filingStatus,
function calculateTax(
income: number,
headOfHousehold: "Head of Household",
function generateHtml(taxBrackets: TaxBracket[], selectedFilingStatus: string): string {
let tableRows = "";
</html>
export default async function main(req: Request): Promise<Response> {
const taxBrackets = parseCsv(csvData);