Search
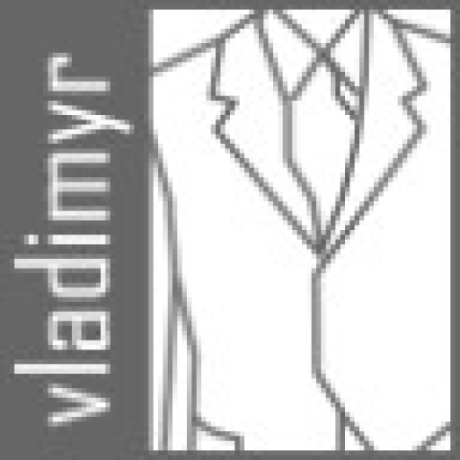
appendFragment
@vladimyr
// SPDX-License-Identifier: 0BSD
Script
// SPDX-License-Identifier: 0BSD
export function appendFragment(fragment: string, html: string) {
if (!fragment) return html;
let offset = Math.min(
sortedPosts
@andreterron
Prioritizes posts by authors with the most followers
Script
import { fetchTwitterUser } from "https://esm.town/v/andreterron/fetchTwitterUser";
export async function sortedPosts({ accessToken, posts }: {
accessToken: string;
posts: {
milligramCss
@xkonti
An interactive, runnable TypeScript val by xkonti
Script
import { Page } from "https://esm.town/v/xkonti/htmlBuilder";
export function addMilligram(page: Page): Page {
stylesheets.forEach(url => {
page.addStylesheet(url);
taxrates
@wilg
// This approach calculates taxes based on the selected filing status from the URL.
HTTP
filingStatus: string;
function parseCsv(csv: string): TaxBracket[] {
const lines = csv.trim().split("\n");
filingStatus,
function calculateTax(
income: number,
headOfHousehold: "Head of Household",
function generateHtml(taxBrackets: TaxBracket[], selectedFilingStatus: string): string {
let tableRows = "";
</html>
export default async function main(req: Request): Promise<Response> {
const taxBrackets = parseCsv(csvData);
valTownBadge
@jxnblk
Add a Val Town badge to your own HTTP vals Option 1: Middleware Import the middleware from https://www.val.town/v/jxnblk/valTownBadgeMiddleware Wrap your HTML request handler with middleware, and pass import.meta.url to link to your val import wrapper from "https://esm.town/v/jxnblk/valTownBadgeMiddleware";
async function handler(req: Request): Promise<Response> {
const html = `
<h1>Hello, world</h1>
`;
return new Response(html, {
headers: {
"Content-Type": "text/html; charset=utf-8",
},
});
}
export default wrapper(handler, import.meta.url); Option 2: HTML string generator Get the HTML string for the badge using https://www.val.town/v/jxnblk/valTownBadge Add the HTML to your response's HTML string wherever you like import valTownBadge from "https://esm.town/v/jxnblk/valTownBadge";
export default async function(req: Request): Promise<Response> {
const badge = valTownBadge(import.meta.url);
const html = `
<h1>Hello, world</h1>
${badge}
`;
return new Response(html, {
headers: {
"Content-Type": "text/html; charset=utf-8",
},
});
} Manual options You can also edit the snippet below to manually add the badge in HTML <a href="https://www.val.town/v/jxnblk/valTownBadgeExample" target="_blank" style="text-decoration:none;color:inherit">
<img src="https://jxnblk-valtownbadgesvg.web.val.run/" width="160" height="160">
</a> Or markdown: [](https://www.val.town/v/jxnblk/valTownBadgeExample) Vals used to create this https://www.val.town/v/jxnblk/valTownBadgeSVG SVG badge image service https://www.val.town/v/jxnblk/valTownLogotypeReact Val Town logo React component https://www.val.town/v/jxnblk/codeIconReact Code icon React component https://www.val.town/v/jxnblk/valTownBadge HTML generator that uses import.meta.url to create a link to your val https://www.val.town/v/jxnblk/valTownBadgeMiddleware Middleware to inject badge HTML in the lower right corner of your page
Script
import wrapper from "https://esm.town/v/jxnblk/valTownBadgeMiddleware";
async function handler(req: Request): Promise<Response> {
const html = `
import valTownBadge from "https://esm.town/v/jxnblk/valTownBadge";
export default async function(req: Request): Promise<Response> {
const badge = valTownBadge(import.meta.url);
function getHREF(mod) {
const url = new URL(mod);
width?: number;
export default function GetBadgeHTML(url: string, opts: BadgeOptions = {}): string {
const href = getHREF(url);
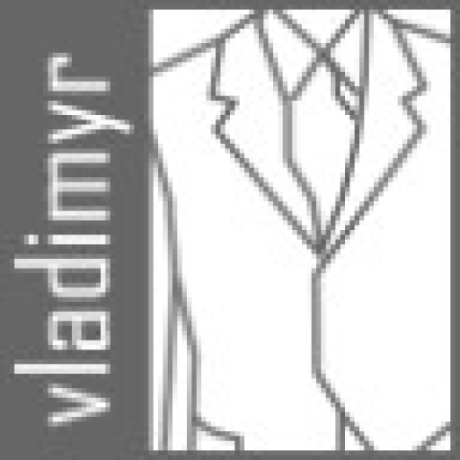
libbuffer
@vladimyr
// SPDX-License-Identifier: 0BSD
Script
// SPDX-License-Identifier: 0BSD
export function bufferEquals(buffer1: ArrayBuffer, buffer2: ArrayBuffer) {
if (buffer1.byteLength !== buffer2.byteLength) return false;
let i = 0;
i += 1;
return true;
export function splitBuffer(buffer: ArrayBuffer, chunkSize: number) {
const chunks = [];
let offset = 0;
trips
@mxdvl
Turn an array of coordinates in the format [longitude, latitude] into an array of GeoJSON features as line strings. An optional distance threshold between subsequent points decides when distinct trips are created. Defaults to 120m. Example on Observable
Script
properties: { from: string; to: string | undefined };
geometry: { type: "LineString"; coordinates: readonly Coordinates[] };
async function to_line_string(
coordinates: readonly Coordinates[],
en_route = false,
type: "LineString",
coordinates,
export async function* trips(
coordinates: readonly Coordinates[],
distance_threshold = 120,

blobDirList
@neverstew
List things in a blob directory Blobs can be organised using "directories" e.g. /animals
all-animals.txt
/dogs
sausage.txt
/cats
tom.txt is really only three files: /animals/all-animals.txt , /animals/dogs/sausage.txt and /animals/cats/tom.txt because directories don't really exist, we're just making longer filenames. When you want to list things only "in a directory" and none of the child "directories", you can use this val. import { blobDirList } from "https://esm.town/v/neverstew/blobDirList";
console.log(await blobDirList("/animals"));
// returns only "/animals/all-animals.txt"
Script
type BlobMetadata = Awaited<ReturnType<typeof blob.list>>[0];
export const inDir = (directory: string) => (blobMeta: BlobMetadata) => blobMeta => dirname(blobMeta.key) === directory;
export async function blobDirList(directory: string) {
const list = await blob.list(directory);
return list.filter(inDir(directory));

toDatesWithTz
@wilt
An interactive, runnable TypeScript val by wilt
Script
export async function toDatesWithTz(
dateStrings: string[],
timeZone: string,
formatFloat
@tmcw
An interactive, runnable TypeScript val by tmcw
Script
[87, "⅞"],
// TODO: refactor
export function formatFloat(a: number) {
const intPart = Math.floor(a);
const remainder = Math.floor((a - intPart) * 100);
timeSpanUnion
@xkonti
timeSpanUnion function Combines overlapping time spans into single time spans. Parameters: timespans - An array of TimeSpan objects to unify.
Returns: An array of unified TimeSpan objects. TimeSpan type Represents a span of time with a starting point and a duration in minutes.
Script
# `timeSpanUnion` function
Combines overlapping time spans into single time spans.
big_stories_ranks
@tmcw
// set by tmcw.big_story at 2023-07-21T13:27:35.553Z
Script
"ranks": [[1689676050115, 8], [1689679650435, 9], [1689683250422, 9], [1689686850141, 9], [1689690449649, 9], [1689694050066, 10]]
"https://www.nytimes.com/2023/07/18/technology/openai-chatgpt-facial-recognition.html": {
"title": "OpenAI Worries About What Its Chatbot Will Say About People’s Faces",
"url": "https://www.nytimes.com/2023/07/18/technology/openai-chatgpt-facial-recognition.html",
"section": "technology",
bulletJournalMonth
@eczajk
An interactive, runnable TypeScript val by eczajk
Script
export async function bulletJournalMonth(month, year) {
const d = new Date();
let month2 = typeof month === "number" ? month : d.getMonth();