Search
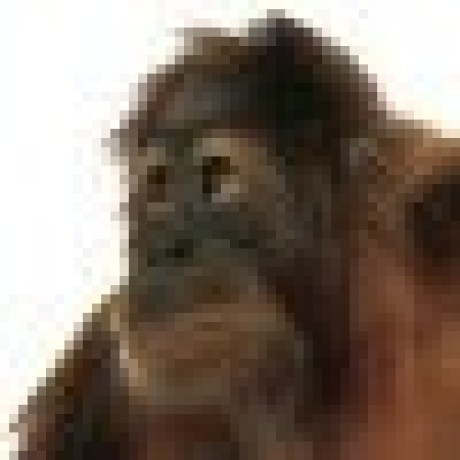
coffeeFowl
@pomdtr
An interactive, runnable TypeScript val by pomdtr
Script
// Import Astral
const command = new Command().arguments("<app>").action(async (_, app) => {
// Launch the browser
const browser = await launch();
// Open a new page
const page = await browser.newPage(`https://${app}.localhost`);
// Take a screenshot of the page and save that to disk
const screenshot = await page.screenshot();
Deno.stdout.write(screenshot);
browser.close();
honoTanaEndpoint
@nbbaier
An interactive, runnable TypeScript val by nbbaier
Script
const token = Deno.env.get("tanaInputAPI");
export const honoTanaEndpoint = async (req: Request) => {
const app = new Hono();
app.get("/", async (c) => {
let { text, url } = c.req.query();
const payload: APIPlainNode = {
name: text,
children: [
type: "field",
attributeId: "cwi23sOzRSh8",
faunadb_test
@mattx
An interactive, runnable TypeScript val by mattx
Script
import process from "node:process";
export const faunadb_test = (async () => {
const { faunadb } = await import("npm:faunadb");
const client = new faunadb.Client({
secret: process.env.fauna_key,
const { Get, Ref, Collection } = faunadb.query;
// from included sample data
const result = await client.query(Get(Ref(Collection("products"), "202")));
return result;
gemini2FlashExample
@tsuchi_ya
An interactive, runnable TypeScript val by tsuchi_ya
Script
import { GoogleGenerativeAI } from "npm:@google/generative-ai";
const prompt = "日本の関西での一般的な挨拶を教えて。";
const genAI = new GoogleGenerativeAI(Deno.env.get("GOOGLE_GENERATIVE_AI"));
const model = genAI.getGenerativeModel(
{ model: "gemini-2.0-flash-exp" },
const result = await model.generateContent(prompt);
console.log(result.response.text());
masterfulBronzeFlea
@jeffreyyoung
An interactive, runnable TypeScript val by jeffreyyoung
HTTP
const basePrompt = [
`Generate an image generation model prompt (description) for the previous image, in nightmare before christmas chibi 3D anime style.`,
`The objective is to try to replicate the image as closely as possible,`,
`but in the new style. To do that, you should be very detailed in including`,
`relevant information necessary to reproduce the image, e.g. colors, poses,`,
`facial expressions, background objects, etc. Ensure that the entire description `,
`is consistent with the nightmare before christmas chibi 3D anime style, especially`,
`the 'nightmare before christmas 3D' part. Output only the prompt and nothing else.`,
].join(" ");
export default serve({
wideApi
@maxm
An interactive, runnable TypeScript val by maxm
HTTP
export type { TSearchFilter } from "https://esm.town/v/maxm/wideLib";
const app = new Hono();
type Env = { Variables: { user: Awaited<ReturnType<typeof ValSession["validate"]>> } };
const authMiddleware = createMiddleware<Env>(async (c, next) => {
c.set("user", await ValSession.validate(c.req.header("sessiontoken") || ""));
await next();
const routes = app.use("/v1/*", authMiddleware).onError((err, c) => {
return c.json({ error: { message: err.message, stack: err.stack, name: err.name } }, 500);
}).post(
"/v1/write",
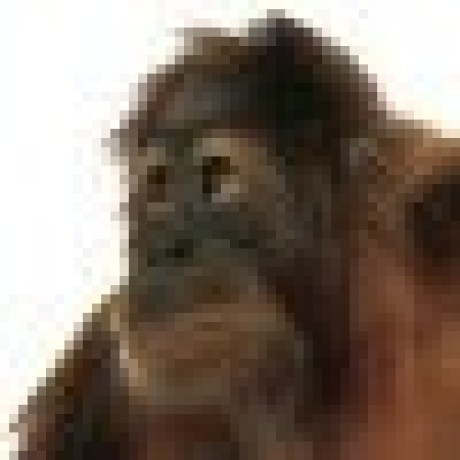
execute
@pomdtr
An interactive, runnable TypeScript val by pomdtr
HTTP
import { InStatement, sqlite } from "https://esm.town/v/std/sqlite?v=4";
export default rpc(async (statement: InStatement) => {
try {
const res = await sqlite.execute(statement);
return res[0];
} catch (e) {
throw new Response(e.message, {
status: 500,
projects
@willthereader
@jsxImportSource https://esm.sh/hono@latest/jsx
HTTP
/** @jsxImportSource https://esm.sh/hono@latest/jsx **/
export const projects = (c: Context) => {
return c.html(
<html>
<head>
<title>Projects</title>
<style
dangerouslySetInnerHTML={{
__html:
`:root{--slate1: hsl(200, 7%, 8.8%);--slate2: hsl(195, 7.1%, 11%);--slate3: hsl(197, 6.8%, 13.6%);--slate4: hsl(198, 6.6%, 15.8%);--slate5: hsl(199, 6.4%, 17.9%);--slate6: hsl(201, 6.2%, 20.5%);--slate7: hsl(203, 6%, 24.3%);--slate8: hsl(207, 5.6%, 31.6%);--slate9: hsl(206, 6%, 43.9%);--slate10: hsl(206, 5.2%, 49.5%);--slate11: hsl(206, 6%, 63%);--slate12: hsl(210, 6%, 93%);--blue1: hsl(212, 35%, 9.2%);--blue2: hsl(216, 50%, 11.8%);--blue3: hsl(214, 59.4%, 15.3%);--blue4: hsl(214, 65.8%, 17.9%);--blue5: hsl(213, 71.2%, 20.2%);--blue6: hsl(212, 77.4%, 23.1%);--blue7: hsl(211, 85.1%, 27.4%);--blue8: hsl(211, 89.7%, 34.1%);--blue9: hsl(206, 100%, 50%);--blue10: hsl(209, 100%, 60.6%);--blue11: hsl(210, 100%, 66.1%);--blue12: hsl(206, 98%, 95.8%)}body{font-family:system-ui,sans-serif;margin:auto;padding:20px;max-width:65ch;text-align:left;word-wrap:break-word;overflow-wrap:break-word;line-height:1.5}h1,h2,h3,h4,h5,h6,strong,b{font-weight:500}a{color:var(--blue10)}nav a{margin-right:10px}textarea{width:100%;font-size:16px}input{font-size:16px}content{line-height:1.6}table{width:100%}img{max-width:100%;height:auto}code{padding:2px 5px;background-color:var(--slate4);font-family:menlo,monospace}pre{padding:1rem}pre>code{all:unset}blockquote{border:1px solid var(--slate10);color:var(--slate11);padding:2px 0 2px 20px;margin:0;font-style:italic}a[data-astro-cid-eimmu3lg]{display:inline-block;text-decoration:none}a[data-astro-cid-eimmu3lg].active{font-weight:600;text-decoration:underline}header[data-astro-cid-3ef6ksr2]{margin:0 0 2em}h2[data-astro-cid-3ef6ksr2]{margin:.5em 0}`,
testApi
@andreterron
An interactive, runnable TypeScript val by andreterron
Script
export const testApi = (async () => {
const threshold = 90;
const opts = {
headers: {
Authorization: `Bearer ${process.env.vt_token}`,
const me = await fetchJSON(
"https://api.val.town/v1/me",
opts,
// TODO: Paginate
const vals = await paginateAPI(
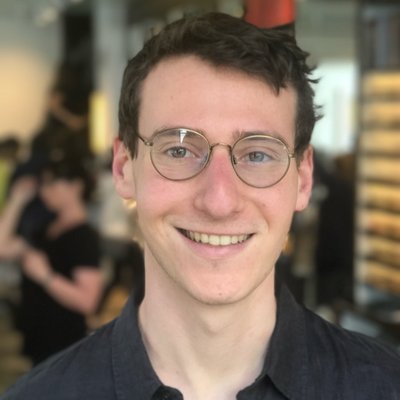
examplebot_endpoint
@stevekrouse
// Forked from @malloc.examplebot_endpoint
Script
export let examplebot_endpoint = (
req: express.Request,
res: express.Response,
console.log(res);
if (!req.get("X-Signature-Timestamp") || !req.get("X-Signature-Ed25519")) {
res.status(400);
res.end("Signature headers missing");
verify_discord_signature(
process.env.discord_pubkey,
JSON.stringify(req.body),
ChibifyBase
@jeffreyyoung
An interactive, runnable TypeScript val by jeffreyyoung
HTTP
const basePrompt = [
`Generate an image generation model prompt (description) for the previous image, in nightmare before christmas chibi 3D anime style.`,
`The objective is to try to replicate the image as closely as possible,`,
`but in the new style. To do that, you should be very detailed in including`,
`relevant information necessary to reproduce the image, e.g. colors, poses,`,
`facial expressions, background objects, etc. Ensure that the entire description `,
`is consistent with the nightmare before christmas chibi 3D anime style, especially`,
`the 'nightmare before christmas 3D' part. Output only the prompt and nothing else.`,
].join(" ");
export default serve({
scarletSole
@tempguy
An interactive, runnable TypeScript val by tempguy
HTTP
interface Context {
url: string;
const app = new Hono();
app.get("/dood/:dood", async (c) => {
const { dood } = c.req.param();
const ctx = { url: "https://d000d.com/e/" + dood };
return c.json(await doodstream(ctx));
app.get("/tape/:tape", async (c) => {
const { tape } = c.req.param();
const ctx = { url: "https://streamtape.com/e/" + tape };
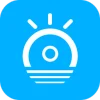
pipeSampleLLMRetriever
@webup
An interactive, runnable TypeScript val by webup
Script
export const pipeSampleLLMRetriever = (async () => {
const { PromptTemplate } = await import("npm:langchain/prompts");
const { RunnableSequence, RunnablePassthrough } = await import(
"npm:langchain/schema/runnable"
const { StringOutputParser } = await import(
"npm:langchain/schema/output_parser"
const { Document } = await import("npm:langchain/document");
const modelBuilder = await getModelBuilder();
const model = await modelBuilder();
const tracerBuilder = await getLangSmithBuilder();
antonia_tim_burton
@jeffreyyoung
An interactive, runnable TypeScript val by jeffreyyoung
HTTP
const basePrompt = [
`Generate an image generation model prompt (description) for the previous image, in nightmare before christmas chibi 3D anime style.`,
`The objective is to try to replicate the image as closely as possible,`,
`but in the new style. To do that, you should be very detailed in including`,
`relevant information necessary to reproduce the image, e.g. colors, poses,`,
`facial expressions, background objects, etc. Ensure that the entire description `,
`is consistent with the nightmare before christmas chibi 3D anime style, especially`,
`the 'nightmare before christmas 3D' part. Output only the prompt and nothing else.`,
].join(" ");
const settings = {
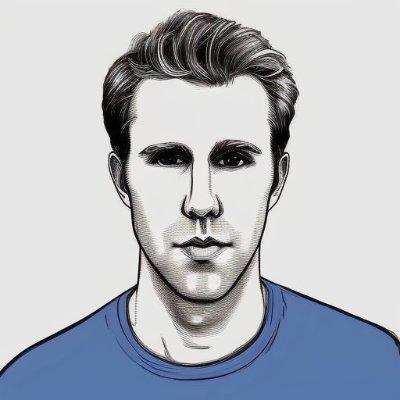
llmTS
@mrick
An interactive, runnable TypeScript val by mrick
Script
export const llmTS = (async () => {
const { LLM, MODEL } = await import("npm:llm.ts");
return new LLM({
apiKeys: {
huggingface: process.env.HF_API_TOKEN,
}).completion({
prompt: [
'Repeat the following sentence: "I am a robot."',
'Repeat the following sentence: "I am a human."',
model: ["gpt2"],