Search
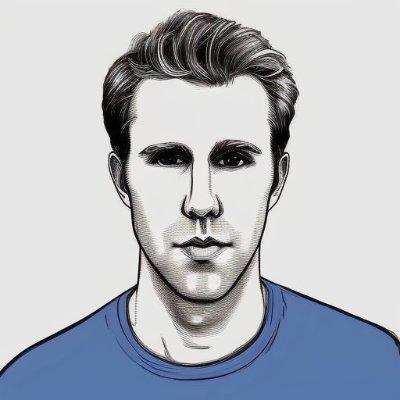
llmTS
@mrick
An interactive, runnable TypeScript val by mrick
Script
export const llmTS = (async () => {
const { LLM, MODEL } = await import("npm:llm.ts");
return new LLM({
apiKeys: {
huggingface: process.env.HF_API_TOKEN,
}).completion({
prompt: [
'Repeat the following sentence: "I am a robot."',
'Repeat the following sentence: "I am a human."',
model: ["gpt2"],
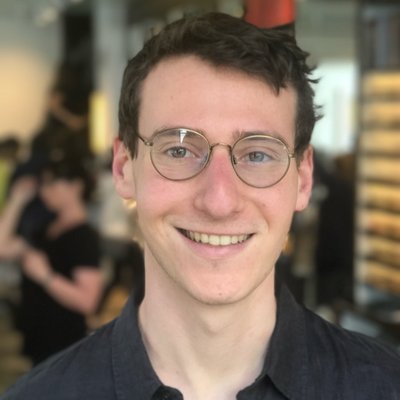
testNasa
@stevekrouse
An interactive, runnable TypeScript val by stevekrouse
Script
import process from "node:process";
import { fetchJSON } from "https://esm.town/v/stevekrouse/fetchJSON";
export const testNasa = fetchJSON(
`https://api.nasa.gov/planetary/apod?api_key=${process.env.nasa}&date=1995-06-16`,
possibleAquamarineLocust
@jeffreyyoung
An interactive, runnable TypeScript val by jeffreyyoung
HTTP
events,
forward,
PoeBotServerSentEvent,
QueryRequest,
sleep,
} from "https://esm.town/v/jeffreyyoung/poe_bot";
export default bot({
async *handleMessage(query, { req }) {
for await (const event of forward(query, "GPT-4o", "FrwBHBROBdXVDbCpSsNozHUinTVzpxaY")) {
console.log("events", event);
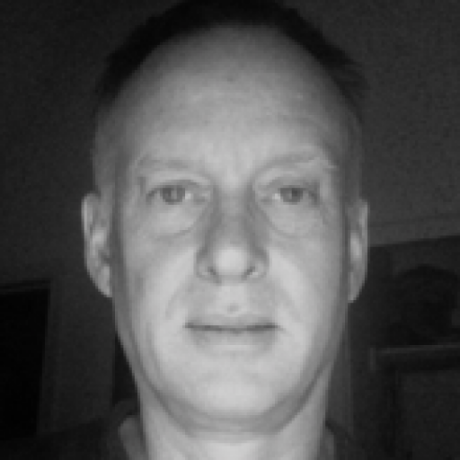
tanaSave
@impedivity
// console.log(console.log(JSON.stringify(Request)));
HTTP
const token = Deno.env.get("tanaInputAPI");
console.log(token);
console.log(Request);
// console.log(console.log(JSON.stringify(Request)));
export const tanaSave = async (req: Request) => {
// console.log("Hello, World!");
const app = new Hono();
app.get("/", async c => c.text("Hello from Hono!"));
app.get("/save", async c => c.text("Endpoint: /save"));
app.get("/save2", async c => {
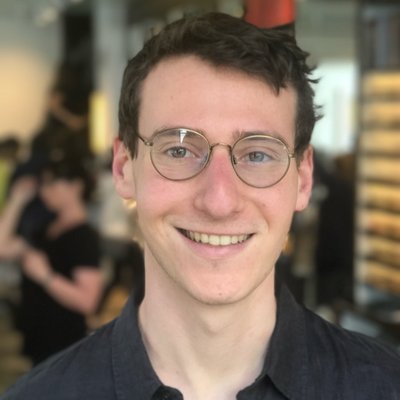
valtownApiTypesImportTest
@stevekrouse
// Forked from @nbbaier.valtownApiTypesImportTest
Script
export let valtownApiTypesImportTest = (async () => {
const openapi = await import("https://esm.sh/valtown-api-types@0.1.1");
console.log(Object.keys(openapi));
// Forked from @nbbaier.valtownApiTypesImportTest
openAQLocation
@tmcw
An interactive, runnable TypeScript val by tmcw
Script
export let openAQLocation = async ({
lat,
lon,
lat: number;
lon: number;
let q = "";
return q.charAt();
// Test
const { results } = fetchJSON(
"https://api.openaq.org/v2/locations?" +
googleGeminiAPI
@charmaine
Gemini API A simple and easy to use client for the Gemini API This template shows you how to use the Gemini. This starter template was ported from this one on Docs . To run it: Click Fork on this val Get your Gemini API key Add it to your Environment Variables as GEMINI_API_KEY Click Run on this script val
Script
# Gemini API
A simple and easy to use client for the Gemini API
*_This template shows you how to use the Gemini. This starter template was ported from [this one on Docs](https://ai.google.dev/gemini-api/docs/quickstart?lang=node#install-gemini-library)._*
## To run it:
- Click `Fork` on this val
- Get your [Gemini API key](https://aistudio.google.com/app/apikey)
const genAI = new GoogleGenerativeAI(Deno.env.get("GEMINI_API_KEY"));
const model = genAI.getGenerativeModel({ model: "gemini-1.5-flash" });
const prompt = "Explain how AI works";
const result = await model.generateContent(prompt);
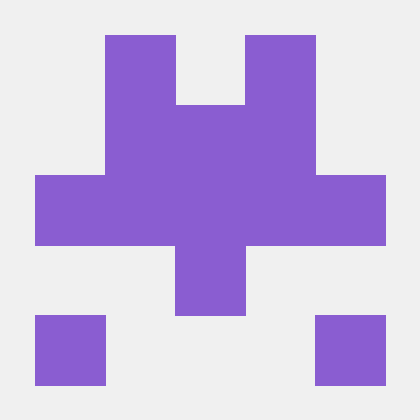
tanHedgehog
@greatcode
An interactive, runnable TypeScript val by greatcode
HTTP
const app = new Hono();
type X = "asdf" | "qwer";
app.get("/api/page", (c) => {
const y: X = "zxcv";
return c.json({ message: "You are authorized" });
app.post('/gmail', async (c) => {
try {
const body = await c.req.json();
console.log(body)
} catch (e) {
emailAPI
@agmm
// https://username-valname.web.val.run?k=myKey&s=mySubject&m=myMessage
HTTP
// Usage exmaple:
// https://username-valname.web.val.run?k=myKey&s=mySubject&m=myMessage
const app = new Hono();
app.get("/", async (c) => {
const now = new Date();
let { to, s, m, k } = c.req.query();
s = s || "message from api";
m = m || "<empty>";
const checks = [
c.req.header("cf-ipcountry") === "US",
foo
@runningj
An interactive, runnable TypeScript val by runningj
Script
import { fetch } from "https://esm.town/v/std/fetch";
export const foo = () => {
fetch("ipinfo.io")
.then((res) => {
console.log("ok");
console.log(res);
console.email("runningj@duck.com");
.catch((e) => {
console.log("error");
console.error(e);
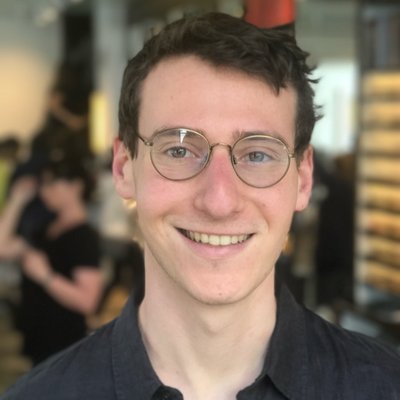
hiddenAPI
@stevekrouse
An interactive, runnable TypeScript val by stevekrouse
RPC (deprecated)
import { hiddenAPIInternal } from "https://esm.town/v/stevekrouse/hiddenAPIInternal";
export let hiddenAPI = (...args) => hiddenAPIInternal(...args);
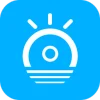
pipeSampleLLMRetrieverConversation
@webup
An interactive, runnable TypeScript val by webup
Script
export const pipeSampleLLMRetrieverConversation = (async () => {
const { PromptTemplate } = await import("npm:langchain/prompts");
const { RunnableSequence, RunnablePassthrough } = await import(
"npm:langchain/schema/runnable"
const { StringOutputParser } = await import(
"npm:langchain/schema/output_parser"
const { Document } = await import("npm:langchain/document");
const modelBuilder = await getModelBuilder();
const model = await modelBuilder();
const docs = await getSampleDocuments();
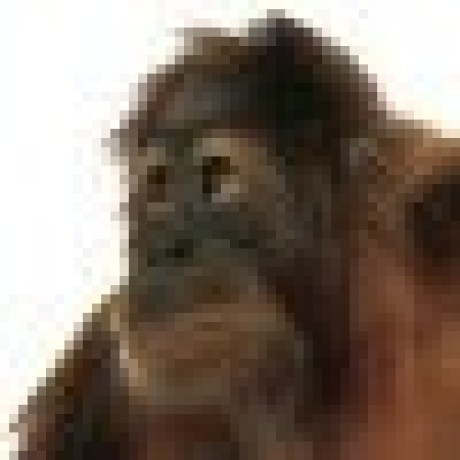
oauthConcept
@pomdtr
// oauth middleware protect the val behind github oauth
HTTP
// oauth middleware protect the val behind github oauth
export default oauth({
scopes: ["read:user"],
client_id: Deno.env.get("GITHUB_CLIENT_ID"),
client_secret: Deno.env.get("GITHUB_CLIENT_SECRET"),
auth_url: "https://github.com/login/oauth/authorize",
token_url: "https://github.com/login/oauth/access_token",
}, async (req: Request, accessToken: string) => {
const octokit = new Octokit({ auth: accessToken });
const { data: { login } } = await octokit.rest.users.getAuthenticated();
chatbot
@odicho
An example of using Breadboard Board as a Service helper script. The board is a single turn of a chat bot: takes the conversation context and returns updated conversation context. To use: fork this val set the URL of the board to be served add "GEMINI_KEY" to your environment variables query directly or use as Service URL in the Core Kit service node.
HTTP
An example of using [Breadboard Board as a Service](https://www.val.town/v/dglazkov/bbaas) helper script. The board is a single turn of a chat bot: takes the conversation context and returns updated conversation context.
To use:
- fork this val
- set the URL of the board to be served
- add "GEMINI_KEY" to your environment variables
- query directly or use as Service URL in the Core Kit `service` node.
import { service } from "https://esm.town/v/odicho/entireGoldLark";
export default service(
"https://breadboard-community.wl.r.appspot.com/boards/@AdorableGorilla/catchup-local-copy.bgl.json",
nasaAPOD
@henrii
// Returns NASA's Astronomy Picture of the Day (APOD)
Script
import { fetchJSON } from "https://esm.town/v/stevekrouse/fetchJSON?v=41";
// Returns NASA's Astronomy Picture of the Day (APOD)
export const nasaAPOD = await fetchJSON("https://api.nasa.gov/planetary/apod?api_key=DEMO_KEY");
console.log(nasaAPOD.url);
// Forked from @iBrokeit.nasaAPOD