Search
php
@maxm
An interactive, runnable TypeScript val by maxm
HTTP (deprecated)
const { PhpWeb } = await import("https://cdn.jsdelivr.net/npm/php-wasm/PhpWeb.mjs");
const php = new PhpWeb();
export default async function(req: Request): Promise<Response> {
let logs = [];
php.addEventListener("output", (event: any) => logs.push(...event.detail));
const script = "<?php echo \"Hello, world!\";";
const exitCode = await php.run(script);
return Response.json({ script, exitCode, logs });
linkInBioTemplate
@hbcoop
@jsxImportSource https://esm.sh/react@17.0.2
HTTP (deprecated)
/** @jsxImportSource https://esm.sh/react@17.0.2 */
const gradientBackground: React.CSSProperties = {
background: "linear-gradient(135deg, #00a8e8 0%, #007ea7 100%)",
minHeight: "100vh",
display: "flex",
alignItems: "center",
justifyContent: "center",
const containerStyle: React.CSSProperties = {
padding: "30px",
width: "300px",
momentExample
@tmcw
moment moment is a module that helps you do math and formatting with dates and times in JavaScript. It's a pretty old and large module, though: if you're writing new code, you probably want to use luxon or date-fns instead!
Script
# moment
[moment](https://momentjs.com/) is a module that helps you do math and formatting with dates and times in JavaScript. It's a pretty old and large module, though: if you're writing new code, you probably want to use luxon or date-fns instead!
export let momentExample = (async () => {
const { default: moment } = await import("npm:moment");
return moment().add(7, "days").calendar();
chat_openai
@cosmo
An interactive, runnable TypeScript val by cosmo
Script
const { default: OpenAI } = await import("npm:openai");
export async function chat(apiKey, messages) {
const openai = new OpenAI({ apiKey });
return openai.chat.completions.create({
messages,
model: "gpt-3.5-turbo",
stream: false,
naclValidateRequest
@demo
An interactive, runnable TypeScript val by demo
Script
export let naclValidateRequest = async (req: express.Request, publicKey) => {
const { default: nacl } = await import("npm:tweetnacl@1.0.3");
const signature = req.get("X-Signature-Ed25519");
const timestamp = req.get("X-Signature-Timestamp");
const body = JSON.stringify(req.body); // rawBody is expected to be a string, not raw bytes
const isVerified = nacl.sign.detached.verify(
Buffer.from(timestamp + body),
Buffer.from(signature, "hex"),
Buffer.from(publicKey, "hex"),
return isVerified;
valle_tmp_46943868107891815759175483516619
@janpaul123
An interactive, runnable TypeScript val by janpaul123
HTTP (deprecated)
const SAMPLE_STORIES_KEY = "hn_realistic_sample_stories";
async function initializeSampleStories() {
const existingStories = await blob.getJSON(SAMPLE_STORIES_KEY);
if (!existingStories) {
const sampleStories = Array.from({ length: 30 }).map((_, idx) => ({
id: idx + 1,
title: faker.company.catchPhrase(),
url: faker.internet.url(),
votes: Math.floor(Math.random() * 100),
await blob.setJSON(SAMPLE_STORIES_KEY, sampleStories);
xkcd
@loading
An interactive, runnable TypeScript val by loading
HTTP (deprecated)
export default async function(req: Request): Promise<Response> {
return new Response(
(await parseFeed(
await (await fetch(
"https://xkcd.com/atom.xml",
)).text(),
)).entries[0].description?.value!,
status: 200,
headers: {
"content-type": "text/html",
blobmanager
@agmm
An interactive, runnable TypeScript val by agmm
Script
const app = new Hono();
function getValUrl() {
const val = rootRef();
return `https://${val.userHandle}-${val.valName}.web.val.run`;
const valUrl = getValUrl();
app.use(
basicAuth({
username: "admin",
password: Deno.env.get("MANAGER_KEY"),
app.get("/", async (c) => {
valle_tmp_14787389514247828561625352472568
@janpaul123
An interactive, runnable TypeScript val by janpaul123
HTTP (deprecated)
const SAMPLE_STORIES_KEY = "hn_realistic_sample_stories";
async function initializeSampleStories() {
const existingStories = await blob.getJSON(SAMPLE_STORIES_KEY);
if (!existingStories) {
const sampleStories = Array.from({ length: 30 }).map((_, idx) => ({
id: idx + 1,
title: faker.company.catchPhrase(),
url: faker.internet.url(),
votes: Math.floor(Math.random() * 100),
await blob.setJSON(SAMPLE_STORIES_KEY, sampleStories);
testplaywright
@alexdphan
// Import Playwright using the Deno-compatible URL
Script
// Import Playwright using the Deno-compatible URL
// import { chromium } from "https://esm.sh/playwright-core";
console.info("Launching browser...");
// Use Deno.env to get the API key
const apiKey = Deno.env.get("BROWSERBASE_API_KEY");
if (!apiKey) {
console.error("BROWSERBASE_API_KEY environment variable is not set");
Deno.exit(1);
try {
const browser = await chromium.connectOverCDP(
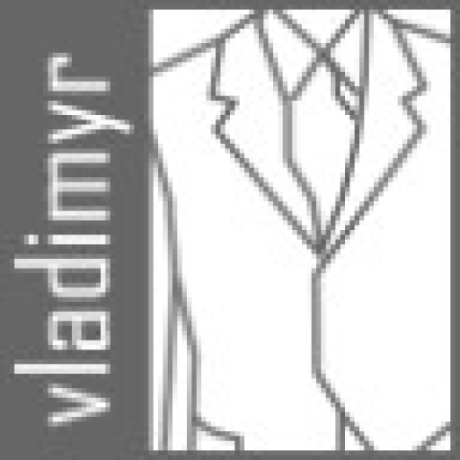
jsrApi
@vladimyr
// SPDX-License-Identifier: 0BSD
Script
// SPDX-License-Identifier: 0BSD
const client = ky.create({ prefixUrl: "https://jsr.io" });
const configFiles = [
"jsr.json",
"jsr.jsonc",
"deno.json",
"deno.jsonc",
// @see: https://jsr.io/docs/api#package-metadata
const PkgMetaSchema = v.object({
scope: v.string(),
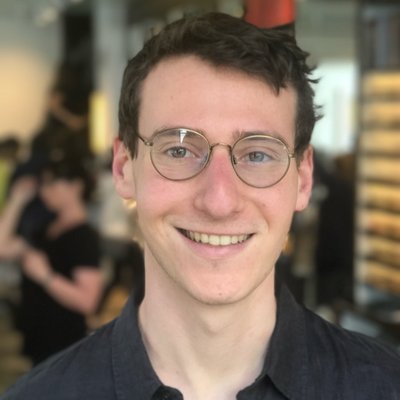
nvc
@stevekrouse
Non-Violent Communication (NVC) Mobile Worksheet Inspired by the lovely iGrok mobile app .
HTTP
# Non-Violent Communication (NVC) Mobile Worksheet
Inspired by the lovely [iGrok mobile app](https://apps.apple.com/ca/app/igrok/id352477754).
/** @jsxImportSource https://esm.sh/react */
export const reactExample = (request: Request) =>
new Response(
modifyHtmlString(
renderToString(
<html
style={{
height: "100%",
ittyExample
@maxm
An interactive, runnable TypeScript val by maxm
HTTP (deprecated)
import { Router, json } from "npm:itty-router@4";
export const ittyRouterExample = async (request: Request) => {
const router = Router();
router.get("/", () => "Hi from itty-router!");
return router.handle(request).then(json);
valle_tmp_94440105214223307666476397039723
@janpaul123
// Initialize sample stories and store them in blob storage
HTTP (deprecated)
// Initialize sample stories and store them in blob storage
const SAMPLE_STORIES_KEY = "hn_sample_stories";
async function initializeSampleStories() {
const existingStories = await blob.getJSON(SAMPLE_STORIES_KEY);
if (!existingStories) {
const sampleStories = Array.from({ length: 30 }).map((_, idx) => ({
id: idx + 1,
title: `Sample Story ${idx + 1}`,
url: `https://example.com/story${idx + 1}`,
votes: Math.floor(Math.random() * 100),
myspace
@triptych
Create your own Myspace profile, deployed to Val town. https://jdan-myspace.web.val.run Click "..." and select Fork to create your own. From there you can: Customize your own profile Or post on my wall by appending to messages and sending me a pull request
HTTP (deprecated)
Create your own Myspace profile, deployed to Val town. https://jdan-myspace.web.val.run
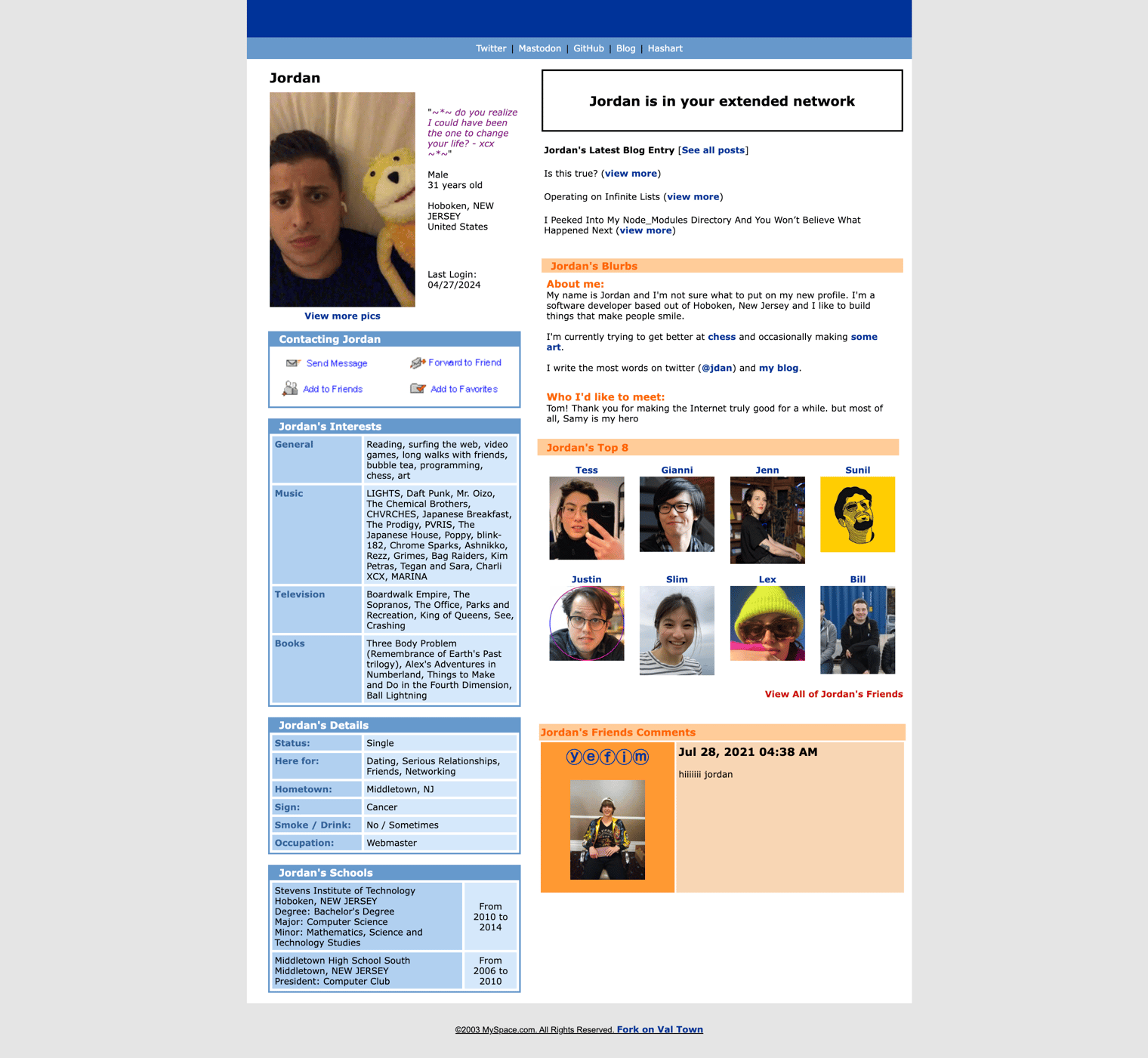
Click "..." and select Fork to create your own.
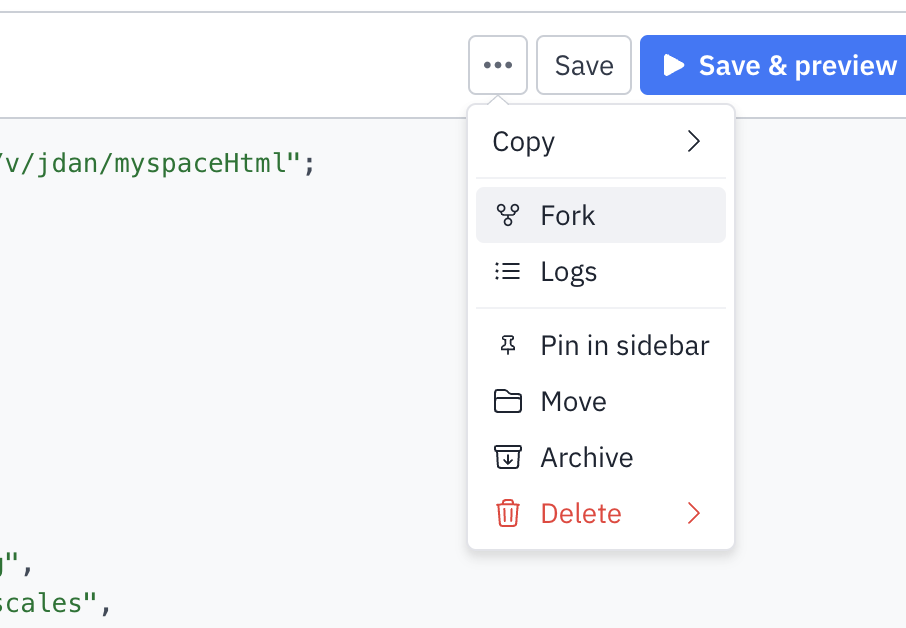
From there you can:
* Customize your own profile
// TODO: Fetch from key-value
const profile = {
displayName: "Jordan",
seo: {