Search
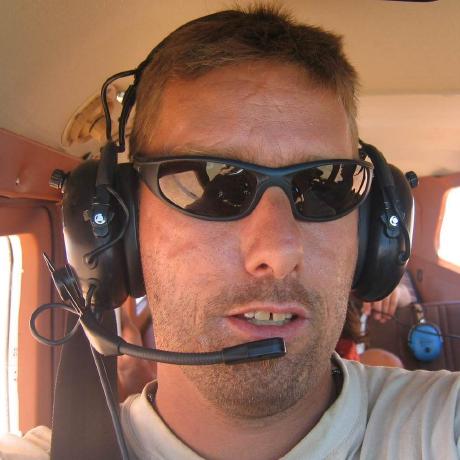
myApi
@danny_engelman
An interactive, runnable TypeScript val by danny_engelman
Script
export function myApi(name) {
return "hi " + name;
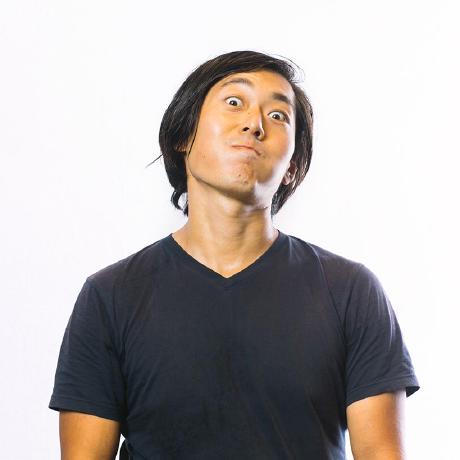
nighthawksChat
@yawnxyz
It's Late. Get a drink. Have a chat.
Welcome to Nighthawks. Nighthawks is an experiment in building interesting NPC characters with AI. The system combines large language models, simulates conversations using character sheets, and attempts to build memories based on the conversation. Improvise, have fun. Approach them like you would at a late night diner. Inspired by the Nighthawks painting. Built on a stripped down agent-based memory system.
HTTP
const kv = new KV();
async function generateText(prompt, charId) {
if (charId) {
return "No character available.";
async function createCharacter(obj) {
console.log('Creating character with:', obj)
return char;
async function saveCharacter(char) {
console.log('Saving character:', char)
return char;
async function getStoredCharacter(charId) {
await nighthawks.loadCharacter(charId);
FindTrendsUsingGPT
@weaverwhale
An interactive, runnable TypeScript val by weaverwhale
HTTP
import { Hono } from "npm:hono";
import { OpenAI } from "npm:openai";
const trendGPT = async (data, onData) => {
const openai = new OpenAI();
// Start the OpenAI stream
const chatStream = await openai.chat.completions.create({
messages: [
val_yG9zG92FbQ
@dhvanil
An interactive, runnable TypeScript val by dhvanil
HTTP
export async function val_yG9zG92FbQ(req) {
try {
// Execute the code directly and capture its result
val_FASG6Vrgm2
@dhvanil
An interactive, runnable TypeScript val by dhvanil
HTTP
export async function val_FASG6Vrgm2(req) {
try {
// Execute the code directly and capture its result
workerifyExample
@postpostscript
Example for @postpostscript/workerify
Script
import { workerify } from "https://esm.town/v/postpostscript/workerify";
export function createWorker() {
return workerify(() => import("https://esm.town/v/postpostscript/workerifyExampleUnsafeLibrary"));
using mod = await createWorker();
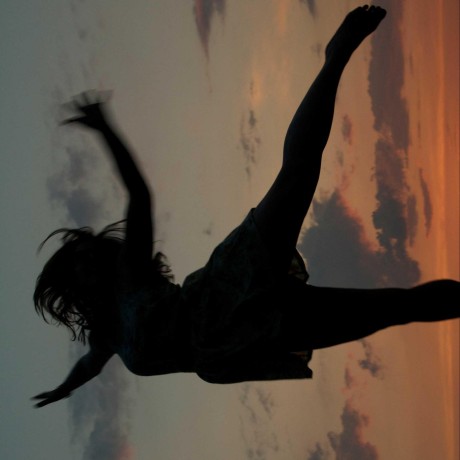
myApi
@simply_say_m
An interactive, runnable TypeScript val by simply_say_m
Script
export function myApi(name) {
return "hi " + name;
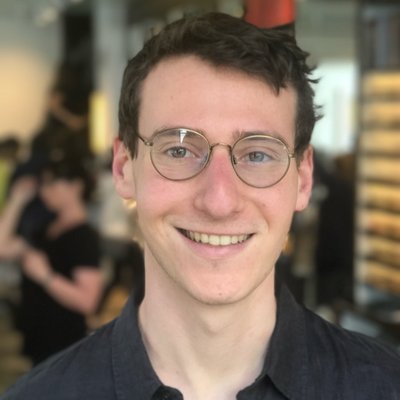
currency
@stevekrouse
An interactive, runnable TypeScript val by stevekrouse
Script
import { fetch } from "https://esm.town/v/std/fetch";
function fetchJSON(url) {
return fetch(url).then(res => res.json());
export let currency = async (desired, base = "usd", amount = 1) => {
nasa
@ejfox
* This program creates an HTTP server that fetches various data from NASA APIs
* and returns a JSON response with a collection of interesting information about today.
* It uses multiple NASA APIs to gather diverse space-related data, including real-time imagery
* and additional interesting data points.
HTTP
import { DOMParser } from "https://esm.sh/linkedom";
const NASA_API_KEY = 'vYg1cCNLVbcgNemMWuLEjoJsGOGbBXZjZjmwVwuV';
async function fetchNASAData() {
const today = new Date().toISOString().split('T')[0];
// Fetch Astronomy Picture of the Day
noradCatId: sat.NORAD_CAT_ID,
objectType: sat.OBJECT_TYPE
export default async function server(request: Request): Promise<Response> {
try {
const nasaData = await fetchNASAData();
myApi
@manurana
An interactive, runnable TypeScript val by manurana
Script
export function myApi(name) {
return "hi " + name;
valle_tmp_610457425865436710444833460520275
@janpaul123
@jsxImportSource https://esm.sh/react
HTTP
import OpenAI from "npm:openai";
unless strictly necessary, for example use APIs that don't require a key, prefer internal function
functions where possible. Unless specified, don't add error handling,
The val should create a "export default async function main() {" which
is the main function that gets executed on every HTTP request.
function write(text) {
function updateValName(valName) {
function saveVal() {
function openTab(tab) {
function toggleTab() {
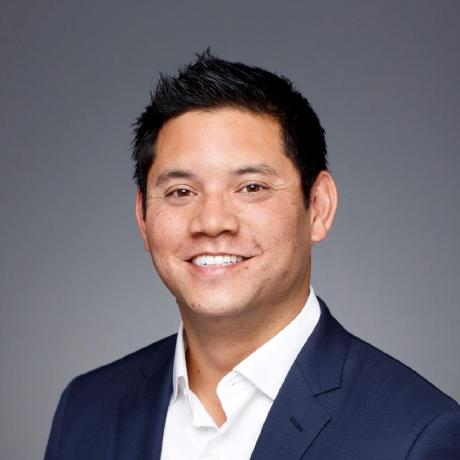
myApi
@plecong
An interactive, runnable TypeScript val by plecong
Script
export function myApi(name) {
return "hi " + name;
exampleHTTP
@maxm
An interactive, runnable TypeScript val by maxm
HTTP
export function handler(request: Request) {
return Response.json({ ok: true });
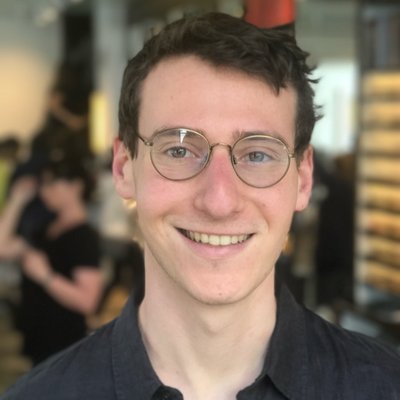
yellowSpider
@stevekrouse
An interactive, runnable TypeScript val by stevekrouse
Script
import { jsPython } from "npm:jspython-interpreter";
const interpreter = jsPython()
.addFunction("fetch", fetch);
const script = `
print(fetch("https://datasette.simonwillison.net/simonwillisonblog/blog_quotation.json?_labels=on&_shape=objects").text())
htmlExample
@andreterron
HTML example This is an example of how to return an HTML response. You can also preview it at https://andreterron-htmlExample.web.val.run?name=Andre
HTTP
// View at https://andreterron-htmlExample.web.val.run?name=Andre
export default async function(req: Request): Promise<Response> {
const query = new URL(req.url).searchParams;
// Read name from the querystring or body. Defaults to "you" if not present.