Search
reactExample
@maxm
@jsxImportSource https://esm.sh/react
HTTP
/** @jsxImportSource https://esm.sh/react */
import { renderToString } from "npm:react-dom/server";
export const reactExample = () =>
new Response(renderToString(<div>Test {1 + 1}</div>), {
linkInBioTemplate
@tdecelle
@jsxImportSource https://esm.sh/react
HTTP
/** @jsxImportSource https://esm.sh/react */
import { renderToString } from "npm:react-dom/server";
export default async function(req: Request) {
return new Response(
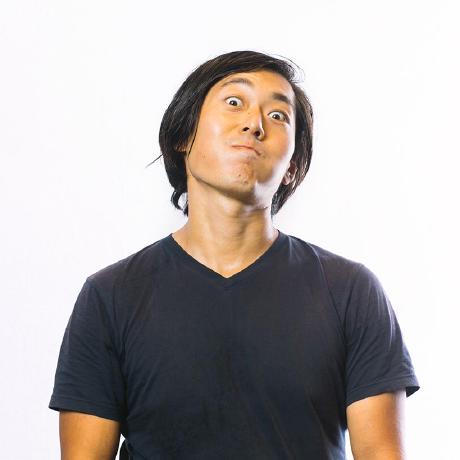
honoAlpineExample
@yawnxyz
// Endpoint to catch and log the submitted name
HTTP
<head>
<meta charset="UTF-8">
<title>Reactive Form with Alpine.js</title>
<script defer src="https://cdn.jsdelivr.net/npm/alpinejs@3.x.x/dist/cdn.min.js"></script>
<script defer src="https://unpkg.com/htmx.org"></script>
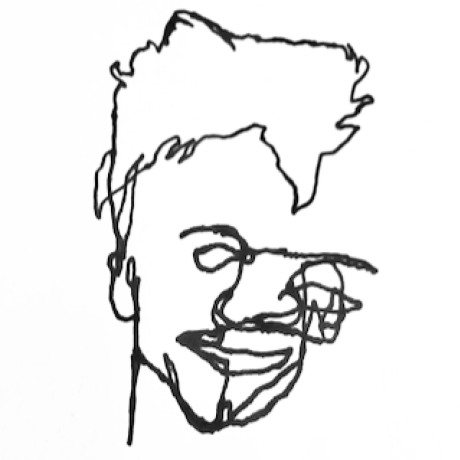
sqliteExplorerApp
@parkerdavis
SQLite Explorer View and interact with your Val Town SQLite data. It's based off Steve's excellent SQLite Admin val, adding the ability to run SQLite queries directly in the interface. This new version has a revised UI and that's heavily inspired by LibSQL Studio by invisal . This is now more an SPA, with tables, queries and results showing up on the same page. Install Install the latest stable version (v66) by forking this val: Authentication Login to your SQLite Explorer with password authentication with your Val Town API Token as the password. Todos / Plans [ ] improve error handling [ ] improve table formatting [ ] sticky table headers [ ] add codemirror [ ] add loading indication to the run button (initial version shipped) [ ] add ability to favorite queries [ ] add saving of last query run for a table (started) [ ] add visible output for non-query statements [ ] add schema viewing [ ] add refresh to table list sidebar after CREATE/DROP/ALTER statements [ ] add automatic execution of initial select query on double click [ ] add views to the sidebar [ ] add triggers to sidebar [ ] add upload from SQL, CSV and JSON [ ] add ability to connect to a non-val town Turso database [x] fix wonky sidebar separator height problem (thanks to @stevekrouse) [x] make result tables scrollable [x] add export to CSV, and JSON (CSV and JSON helper functions written in this val . Thanks to @pomdtr for merging the initial version!) [x] add listener for cmd+enter to submit query
HTTP
- [ ] add schema viewing
- [ ] add refresh to table list sidebar after `CREATE/DROP/ALTER` statements
- [ ] add automatic execution of initial select query on double click
- [ ] add views to the sidebar
- [ ] add triggers to sidebar
- [ ] add upload from SQL, CSV and JSON
- [ ] add ability to connect to a non-val town Turso database
- [x] fix wonky sidebar separator height problem (thanks to @stevekrouse)
- [x] make result tables scrollable
return c.render(
<main class="sidebar-layout">
<div class="sidebar">
<TablesList tables={tables}></TablesList>
<Separator direction="horizontal"></Separator>
<div class="not-sidebar">
<EditorSection />
ableMoccasinGecko
@aakashsingh
@jsxImportSource https://esm.sh/react@18.2.0
HTTP
/** @jsxImportSource https://esm.sh/react@18.2.0 **/
import { renderToString } from "https://esm.sh/react-dom@18.2.0/server";
export default (req: Request) => {
return new Response(
glamorousAquaWalrus
@raaj1v
@jsxImportSource https://esm.sh/react@18.2.0
HTTP
/** @jsxImportSource https://esm.sh/react@18.2.0 **/
import { renderToString } from "https://esm.sh/react-dom@18.2.0/server";
export default (req: Request) => {
return new Response(
linkInBioTemplate
@grubdragon
@jsxImportSource https://esm.sh/react
HTTP
/** @jsxImportSource https://esm.sh/react */
import { renderToString } from "npm:react-dom/server";
export default async function(req: Request) {
return new Response(
tenderHarlequinPuffin
@aryans7054
@jsxImportSource https://esm.sh/react@18.2.0
HTTP
/** @jsxImportSource https://esm.sh/react@18.2.0 **/
import { renderToString } from "https://esm.sh/react-dom@18.2.0/server";
export default (req: Request) => {
return new Response(
rousingJadeParakeet
@kahrvba
@jsxImportSource https://esm.sh/react
HTTP
/** @jsxImportSource https://esm.sh/react **/
import { renderToString } from "https://esm.sh/react-dom@18/server";
export default (req: Request) => {
return new Response(
Rajaram
@Hiii
@jsxImportSource https://esm.sh/react@18.2.0
HTTP
/** @jsxImportSource https://esm.sh/react@18.2.0 */
import React, { useState, useEffect, useCallback } from "https://esm.sh/react@18.2.0";
import { createRoot } from "https://esm.sh/react-dom@18.2.0/client";
// Initial product data with more details
// Error Boundary Component
class ErrorBoundary extends React.Component {
constructor(props) {
</div>
function client() {
createRoot(document.getElementById("root")).render(
<React.StrictMode>
<App />
</React.StrictMode>
if (typeof document !== "undefined") { client(); }
export default async function server(request: Request): Promise<Response> {
tailwind
@moe
@jsxImportSource https://esm.sh/preact
HTTP
/** @jsxImportSource https://esm.sh/preact */
import { reactResponse } from "https://esm.town/v/moe/responses"
export default (req: Request) => {
// console.log(qp)
return reactResponse(
<html>
deftScarletKangaroo
@jjoak3
@jsxImportSource https://esm.sh/react
HTTP
/** @jsxImportSource https://esm.sh/react **/
import { renderToString } from "https://esm.sh/react-dom@18/server";
export default (req: Request) => {
return new Response(
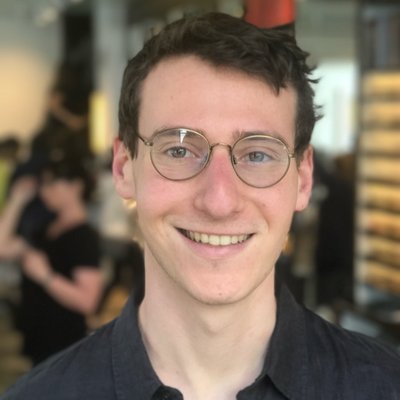
sparklineSVGReact
@stevekrouse
@jsxImportSource https://esm.sh/react
Script
/** @jsxImportSource https://esm.sh/react */
export function SparklineSVG({ strokeWidth, data, height, width, fill, stroke }) {
const padding = 2;