Search
FetchBasic
@gdm
Framer Fetch: Basic A basic example of an API endpoint to use with Framer Fetch.
HTTP
export default async function(req: Request): Promise<Response> {
// Setup CORS Headers
const headers = new Headers();
exampleTextGeneration
@iamseeley
An interactive, runnable TypeScript val by iamseeley
HTTP
import Pipeline from "https://esm.town/v/iamseeley/pipeline";
export default async function handler(req) {
if (req.method === "GET") {
return new Response(`
myApi
@cburgos0511
An interactive, runnable TypeScript val by cburgos0511
Script
export function myApi(name) {
return "hi " + name;
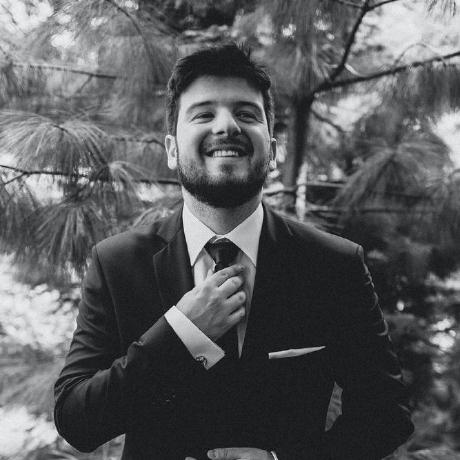
myApi
@marckraw
An interactive, runnable TypeScript val by marckraw
Script
export function myApi(name) {
return "hi " + name;
BikramNpExplorerApp
@air
@jsxImportSource https://esm.sh/react@18.2.0
HTTP
import { createRoot } from "https://esm.sh/react-dom@18.2.0/client";
function App() {
const [messages, setMessages] = useState([
</div>
function client() {
createRoot(document.getElementById("root")).render(<App />);
if (typeof document !== "undefined") { client(); }
export default async function server(request: Request): Promise<Response> {
if (request.method === 'POST' && new URL(request.url).pathname === '/chat') {
const { OpenAI } = await import("https://esm.town/v/std/openai");
const openai = new OpenAI();
const body = await request.json();
const completion = await openai.chat.completions.create({
messages: [
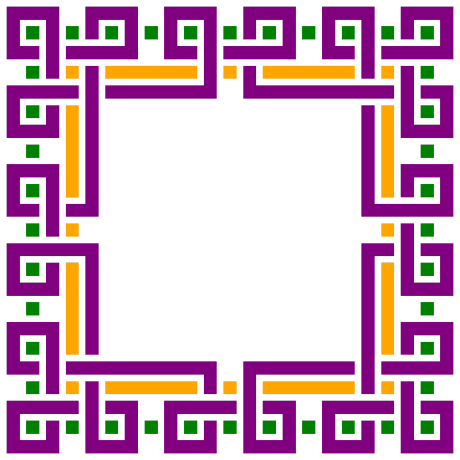
rpc
@zarutian
RPC Turn any function into an API! This lets you call a function in Val Town without manually handling a Request or Response object. This val can be used as a replacement for the Run API, which implicitly did something similar. Create an API from your function: This is how you can expose a function as an API: Create an HTTP handler val Import this val Call rpc with your function as an argument Here's an example from @std/rpc_example: import { rpc } from "https://esm.town/v/std/rpc?v=6";
export const rpc_example = rpc(async (a: number, b: number) => {
return a + b;
}); And here is how you do it for one of your already existing private val, say floobie, import { rpc } from "https://esm.town/v/std/rpc?v=6";
import { floobie } from "https://esm.town/v/stevekrouse/floobie";
export const rpced_floobie = rpc(floobie, true); Send a request to your val You can invoke that function from your client or your local machine with an HTTP request. You can either use a GET or a POST request, passing arguments in the query parameters or the body respectively. Make sure your val's privacy is set to Unlisted or Public. GET request const res = await fetch("https://std-rpc_example.web.val.run/?args=[2,3]");
console.log(await res.json()); // 5 POST request const res = await fetch("https://std-rpc_example.web.val.run/", {
method: "POST",
body: JSON.stringify({ args: [2, 3] })
});
console.log(await res.json()); // 5
Script
# RPC
Turn any function into an API! This lets you call a function in Val Town without manually handling a `Request` or `Response` object.
This val can be used as a replacement for the Run API, which implicitly did something similar.
## Create an API from your function:
This is how you can expose a function as an API:
1. Create an HTTP handler val
2. Import this val
3. Call `rpc` with your function as an argument
Here's an example from @std/rpc_example:
## Send a request to your val
You can invoke that function from your client or your local machine with an HTTP request. You can either use a GET or a POST request, passing arguments in the query parameters or the body respectively. Make sure your val's privacy is set to Unlisted or Public.
**GET request**
const VALTOWN_TOKEN = Deno.env.get("valtown");
export function rpc(fn: Function, isPrivate: boolean = false) {
return async (req: Request): Promise<Response> => {
transcendentTanToucan
@temptemp
An interactive, runnable TypeScript val by temptemp
Cron
const key = "b540c24b3817eaad0241a2baffdd493229c279357c134cd0a1ade2388c398b7d";
const uuid = "1fd98a05-4959-42bc4-2f83-2c487c1cde6d";
export default async function(interval: Interval) {
const t = await fetch("https://workers.cloudflare.com/cdn-cgi/rum?", {
"headers": {
// console.log(await upload.text());
await relay();
async function relay() {
const reqDev = await fetch(
`https://devtools.devprod.cloudflare.dev/js_app?wss=%2Fplayground.devprod.cloudflare.dev%2Fapi%2Finspector%3Fuser%3Db540c24b3817eaad0241a2baffdd493229c279357c134cd0a1ade2388c398b7d%26h%3D87502d4ecedde10987fee740e6c9ae116ea74e13a6cb62c6efc24237ec337d2b&theme=systemPreferred&domain=workers+playground`,
apricotSloth
@paulkinlan
Usage curl https://karfau.web.val.run
Script
const newBoard = await board(() => {
const { result } = core.runJavascript({
code: "function name() { return { name: \"Hello\" };}",
"name": "name",
const output = base.output({});
codeOnValTown
@andreterron
Code on Val Town Adds a "Code on Val Town" ribbon to your page. This lets your website visitors navigate to the code behind it. This uses github-fork-ribbon-css under the hood. Usage Here are 2 different ways to add the "Code on Val Town" ribbon: 1. Wrap your fetch handler (recommended) import { modifyFetchHandler } from "https://esm.town/v/andreterron/codeOnValTown?v=50";
import { html } from "https://esm.town/v/stevekrouse/html?v=5";
export default modifyFetchHandler(async (req: Request): Promise<Response> => {
return html(`<h2>Hello world!</h2>`);
}); Example: @andreterron/openable_handler 2. Wrap your HTML string import { modifyHtmlString } from "https://esm.town/v/andreterron/codeOnValTown?v=50";
import { html } from "https://esm.town/v/stevekrouse/html?v=5";
export default async (req: Request): Promise<Response> => {
return html(modifyHtmlString(`<h2>Hello world!</h2>`));
}; Example: @andreterron/openable_html Other ways We made sure this was very modular, so you can also add the ribbon using these methods: Get the element string directly: @andreterron/codeOnVT_ribbonElement Modify an HTTP Response: @andreterron/codeOnVT_modifyResponse Use .pipeThrough to append to a stream: @andreterron/InjectCodeOnValTownStream Customization Linking to the val These functions infer the val using the call stack or the request URL. If the inference isn't working, or if you want to ensure it links to a specific val, pass the val argument: modifyFetchHandler(handler, {val: { handle: "andre", name: "foo" }}) modifyHtmlString("<html>...", {val: { handle: "andre", name: "foo" }}) Styling You can set the style parameter to a css string to customize the ribbon. Check out github-fork-ribbon-css to learn more about how to style the element. modifyFetchHandler(handler, {style: ".github-fork-ribbon:before { background-color: #333; }"}) modifyHtmlString("<html>...", {style: ".github-fork-ribbon:before { background-color: #333; }"}) Here's how you can hide the ribbon on small screens: modifyFetchHandler(handler, {style: `@media (max-width: 768px) {
.github-fork-ribbon {
display: none !important;
}
}`}) To-dos [ ] Let users customize the ribbon. Some ideas are the text, color or placement.
Script
### Linking to the val
These functions infer the val using the call stack or the request URL. If the inference isn't working, or if you want to ensure it links to a specific val, pass the `val` argument:
- `modifyFetchHandler(handler, {val: { handle: "andre", name: "foo" }})`
* @param val Define which val should open. Defaults to the root reference
export function modifyHtmlString(
bodyText: string,
* @param val Define which val should open
export function modifyFetchHandler(
handler: (req: Request) => Response | Promise<Response>,
myApi
@jacob_kettle
An interactive, runnable TypeScript val by jacob_kettle
Script
export function myApi(name) {
return "hi " + name;
myApi
@gjuggler
An interactive, runnable TypeScript val by gjuggler
Script
export function myApi(name) {
return "hi " + name;
val_3kuijJRJiV
@dhvanil
An interactive, runnable TypeScript val by dhvanil
HTTP
export async function val_3kuijJRJiV(req) {
try {
// Execute the code directly and capture its result
sendLarkMessage
@tzq
An interactive, runnable TypeScript val by tzq
Script
import { fetch } from "https://esm.town/v/std/fetch";
import process from "node:process";
export async function sendLarkMessage(message) {
return fetch(process.env.larkRobotUrl, {
method: "POST",