Public
Script
v2
February 26, 2024
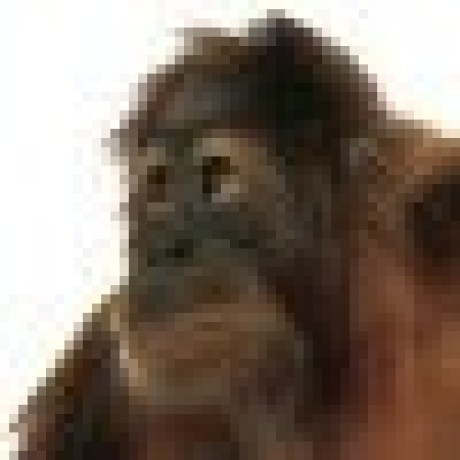
password_auth
@pomdtr
Script
Password Auth Middleware Protect your vals behind a password. Use session cookies to persist authentication. Demo See @pomdtr/password_auth_test Usage import { passwordAuth } from "https://esm.town/v/pomdtr/password_auth?v=84";
export default passwordAuth(() => {
return new Response("OK");
}, { verifyPassword: (password) => password == Deno.env.get("VAL_PASSWORD") }); If you want to use an api token to authenticate: import { passwordAuth } from "https://esm.town/v/pomdtr/password_auth?v=84";
import { verifyToken } from "https://esm.town/v/pomdtr/verifyToken";
export default passwordAuth(() => {
return new Response("OK");
}, { verifyPassword: verifyToken }); TODO [x] allow to authenticate using a val town token [ ] add a way to send an email to ask a password from the val owner [ ] automatically extend the session [ ] automatically remove expired sessions FAQ How to sign out ? Navigate to <your-site>/signout .
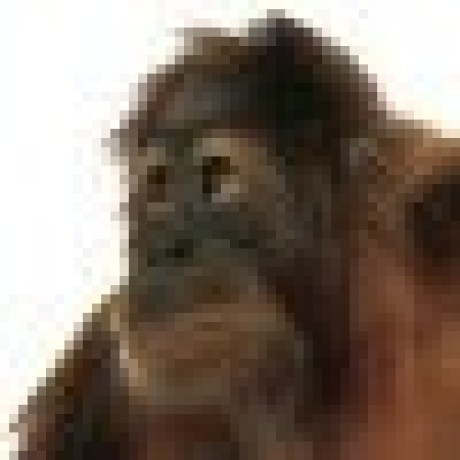
email_auth
@pomdtr
Script
Email Auth for Val Town ⚠️ Require a pro account (needed to send email to users) Usage Create an http server, and wrap it in the emailAuth middleware. import { emailAuth } from "https://esm.town/v/pomdtr/email_auth"
export default emailAuth((req) => {
return new Response(`your mail is ${req.headers.get("X-User-Email")}`);
}, {
verifyEmail: (email) => { return email == "steve@val.town" }
}); 💡 If you do not want to put your email in clear text, just move it to an env var (ex: Deno.env.get("email") ) If you want to allow anyone to access your val, just use: import { emailAuth } from "https://esm.town/v/pomdtr/email_auth"
export default emailAuth((req) => {
return new Response(`your mail is ${req.headers.get("X-User-Email")}`);
}, {
verifyEmail: (_email) => true
}); Each time someone tries to access your val but is not allowed, you will get an email with: the email of the user trying to log in the name of the val the he want to access You can then just add the user to your whitelist to allow him in (and the user will not need to confirm his email again) ! TODO [ ] Add expiration for verification codes and session tokens [ ] use links instead of code for verification [ ] improve errors pages
codeOnValTown
@andreterron
Script
Code on Val Town Adds a "Code on Val Town" ribbon to your page. This lets your website visitors navigate to the code behind it. This uses github-fork-ribbon-css under the hood. Usage Here are 2 different ways to add the "Code on Val Town" ribbon: 1. Wrap your fetch handler (recommended) import { modifyFetchHandler } from "https://esm.town/v/andreterron/codeOnValTown?v=45";
import { html } from "https://esm.town/v/stevekrouse/html?v=5";
export default modifyFetchHandler(async (req: Request): Promise<Response> => {
return html(`<h2>Hello world!</h2>`);
}); Example: @andreterron/openable_handler 2. Wrap your HTML string import { modifyHtmlString } from "https://esm.town/v/andreterron/codeOnValTown?v=45";
import { html } from "https://esm.town/v/stevekrouse/html?v=5";
export default async (req: Request): Promise<Response> => {
return html(modifyHtmlString(`<h2>Hello world!</h2>`));
}; Example: @andreterron/openable_html Other ways We made sure this was very modular, so you can also add the ribbon using these methods: Get the element string directly: @andreterron/codeOnVT_ribbonElement Modify an HTTP Response: @andreterron/codeOnVT_modifyResponse Use .pipeThrough to append to a stream: @andreterron/InjectCodeOnValTownStream Customization Linking to the val These functions infer the val using the call stack or the request URL. If the inference isn't working, or if you want to ensure it links to a specific val, pass the val argument: modifyFetchHandler(handler, {val: { handle: "andre", name: "foo" }}) modifyHtmlString("<html>...", {val: { handle: "andre", name: "foo" }}) Styling You can set the style parameter to a css string to customize the ribbon. Check out github-fork-ribbon-css to learn more about how to style the element. modifyFetchHandler(handler, {style: ".github-fork-ribbon:before { background-color: #333; }"}) modifyHtmlString("<html>...", {style: ".github-fork-ribbon:before { background-color: #333; }"}) Here's how you can hide the ribbon on small screens: modifyFetchHandler(handler, {style: `@media (max-width: 768px) {
.github-fork-ribbon {
display: none !important;
}
}`}) To-dos [ ] Let users customize the ribbon. Some ideas are the text, color or placement.
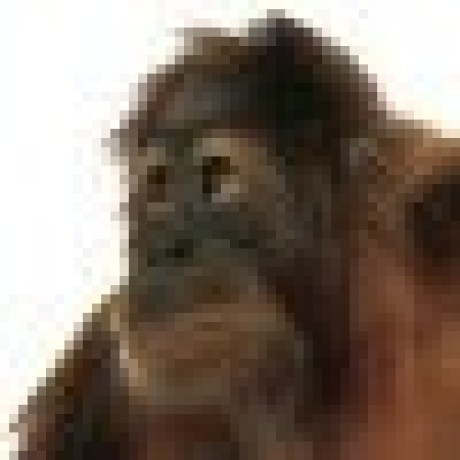
devtools
@pomdtr
Script
Devtools shortcuts Add useful shortcuts to a val website. Usage /_edit -> go to the val editor /_raw -> view val source /_logs -> view val logs Example import { devtools } from "https://esm.town/v/pomdtr/devtools";
export default devtools((_req: Request) => {
return new Response("hello world");
});
modifyIframeResponse
@nbbaier
Script
Prevents your http vals from being viewed inside iframes. If it's a valtown iframe, a helpful message is displayed. Usage: import modifyIframeResponse from "https://esm.town/v/nbbaier/modifyIframeResponse";
export default modifyIframeResponse(async (req: Rquest): Promise<Response> => {
return Response.json({ ok: true })
});
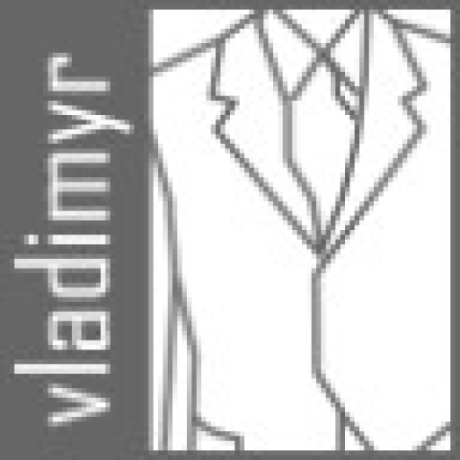
codeOnValTown
@vladimyr
Script
Code on Val Town Adds a "Code on Val Town" ribbon to your page. This lets your website visitors navigate to the code behind it. This uses github-fork-ribbon-css under the hood. Usage Here are 2 different ways to add the "Code on Val Town" ribbon: 1. Wrap your fetch handler (recommended) import { modifyFetchHandler } from "https://esm.town/v/andreterron/codeOnValTown?v=45";
import { html } from "https://esm.town/v/stevekrouse/html?v=5";
export default modifyFetchHandler(async (req: Request): Promise<Response> => {
return html(`<h2>Hello world!</h2>`);
}); Example: @andreterron/openable_handler 2. Wrap your HTML string import { modifyHtmlString } from "https://esm.town/v/andreterron/codeOnValTown?v=45";
import { html } from "https://esm.town/v/stevekrouse/html?v=5";
export default async (req: Request): Promise<Response> => {
return html(modifyHtmlString(`<h2>Hello world!</h2>`));
}; Example: @andreterron/openable_html Other ways We made sure this was very modular, so you can also add the ribbon using these methods: Get the element string directly: @andreterron/codeOnVT_ribbonElement Modify an HTTP Response: @andreterron/codeOnVT_modifyResponse Use .pipeThrough to append to a stream: @andreterron/InjectCodeOnValTownStream Customization Linking to the val These functions infer the val using the call stack or the request URL. If the inference isn't working, or if you want to ensure it links to a specific val, pass the val argument: modifyFetchHandler(handler, {val: { handle: "andre", name: "foo" }}) modifyHtmlString("<html>...", {val: { handle: "andre", name: "foo" }}) Styling You can set the style parameter to a css string to customize the ribbon. Check out github-fork-ribbon-css to learn more about how to style the element. modifyFetchHandler(handler, {style: ".github-fork-ribbon:before { background-color: #333; }"}) modifyHtmlString("<html>...", {style: ".github-fork-ribbon:before { background-color: #333; }"}) Here's how you can hide the ribbon on small screens: modifyFetchHandler(handler, {style: `@media (max-width: 768px) {
.github-fork-ribbon {
display: none !important;
}
}`}) To-dos [ ] Let users customize the ribbon. Some ideas are the text, color or placement.
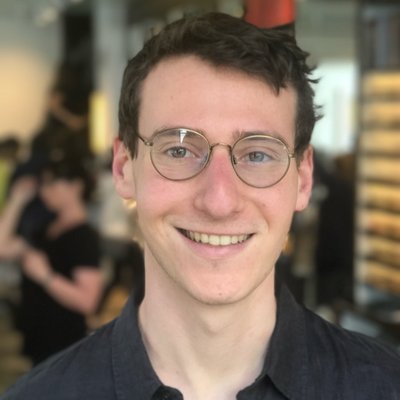
password_auth
@stevekrouse
Script
Password Auth Middleware Protect your vals behind a password. Use session cookies to persist authentication. Demo See @pomdtr/password_auth_test Usage If you want to use an api token to authenticate: import { passwordAuth } from "https://esm.town/v/pomdtr/password_auth";
export default passwordAuth(() => {
return new Response("OK");
}); Or if you prefer to use a string: import { passwordAuth } from "https://esm.town/v/pomdtr/password_auth";
export default passwordAuth(() => {
return new Response("OK");
}, { password: Deno.env.get("MY_PASSWORD") }); Or if you want to share your val with someone without sharing your main password, you can set multiple ones import { passwordAuth } from "https://esm.town/v/pomdtr/password_auth";
export default passwordAuth(() => {
return new Response("OK");
}, { password: [Deno.env.get("MY_PASSWORD"), Deno.env.get("STEVE_PASSWORD")] }); Note that authenticating using your api token is always an option. TODO [x] allow to authenticate using a val town token [ ] add a way to send an email to ask a password from the val owner [ ] automatically extend the session [ ] automatically remove expired sessions FAQ How to sign out ? Navigate to <your-site>/signout .
devtools
@iamseeley
Script
Devtools shortcuts Add useful shortcuts to a val website. Usage /_edit -> go to the val editor /_raw -> view val source /_logs -> view val logs Example import { devtools } from "https://esm.town/v/pomdtr/devtools";
export default devtools((_req: Request) => {
return new Response("hello world");
});
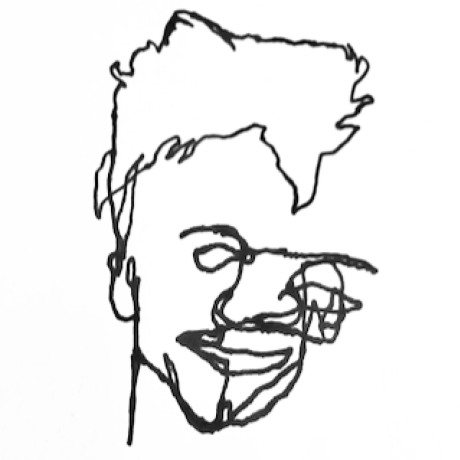
password_auth
@parkerdavis
Script
Password Auth Middleware Protect your vals behind a password. Use session cookies to persist authentication. Demo See @pomdtr/password_auth_test Usage If you want to use an api token to authenticate: import { passwordAuth } from "https://esm.town/v/pomdtr/password_auth";
export default passwordAuth(() => {
return new Response("OK");
}); Or if you prefer to use a string: import { passwordAuth } from "https://esm.town/v/pomdtr/password_auth";
export default passwordAuth(() => {
return new Response("OK");
}, { password: Deno.env.get("VAL_PASSWORD") }); You can also set multiple ones import { passwordAuth } from "https://esm.town/v/pomdtr/password_auth";
export default passwordAuth(() => {
return new Response("OK");
}, { password: [Deno.env.get("VAL_PASSWORD"), Deno.env.get("STEVE_PASSWORD")] }); Note that authenticating using your api token remain an option even after setting a password. TODO [x] allow to authenticate using a val town token [ ] add a way to send an email to ask a password from the val owner [ ] automatically extend the session [ ] automatically remove expired sessions FAQ How to sign out ? Navigate to <your-site>/signout .
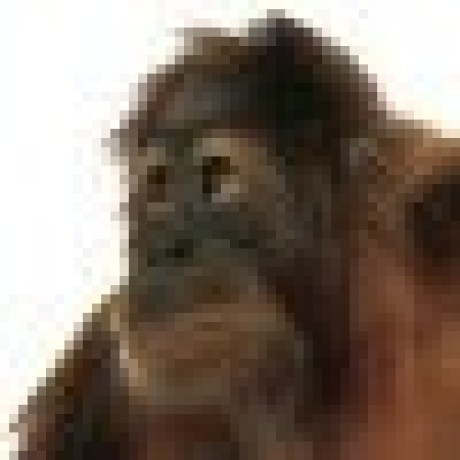
codeOnValTown
@pomdtr
Script
Code on Val Town Adds a "Code on Val Town" ribbon to your page. This lets your website visitors navigate to the code behind it. This uses github-fork-ribbon-css under the hood. Usage Here are 2 different ways to add the "Code on Val Town" ribbon: 1. Wrap your fetch handler (recommended) import { modifyFetchHandler } from "https://esm.town/v/andreterron/codeOnValTown?v=45";
import { html } from "https://esm.town/v/stevekrouse/html?v=5";
export default modifyFetchHandler(async (req: Request): Promise<Response> => {
return html(`<h2>Hello world!</h2>`);
}); Example: @andreterron/openable_handler 2. Wrap your HTML string import { modifyHtmlString } from "https://esm.town/v/andreterron/codeOnValTown?v=45";
import { html } from "https://esm.town/v/stevekrouse/html?v=5";
export default async (req: Request): Promise<Response> => {
return html(modifyHtmlString(`<h2>Hello world!</h2>`));
}; Example: @andreterron/openable_html Other ways We made sure this was very modular, so you can also add the ribbon using these methods: Get the element string directly: @andreterron/codeOnVT_ribbonElement Modify an HTTP Response: @andreterron/codeOnVT_modifyResponse Use .pipeThrough to append to a stream: @andreterron/InjectCodeOnValTownStream Customization Linking to the val These functions infer the val using the call stack or the request URL. If the inference isn't working, or if you want to ensure it links to a specific val, pass the val argument: modifyFetchHandler(handler, {val: { handle: "andre", name: "foo" }}) modifyHtmlString("<html>...", {val: { handle: "andre", name: "foo" }}) Styling You can set the style parameter to a css string to customize the ribbon. Check out github-fork-ribbon-css to learn more about how to style the element. modifyFetchHandler(handler, {style: ".github-fork-ribbon:before { background-color: #333; }"}) modifyHtmlString("<html>...", {style: ".github-fork-ribbon:before { background-color: #333; }"}) Here's how you can hide the ribbon on small screens: modifyFetchHandler(handler, {style: `@media (max-width: 768px) {
.github-fork-ribbon {
display: none !important;
}
}`}) To-dos [ ] Let users customize the ribbon. Some ideas are the text, color or placement.
password_auth
@kamek
Script
Password Auth Middleware Protect your vals behind a password. Use session cookies to persist authentication. Demo See @pomdtr/password_auth_test Usage If you want to use an api token to authenticate: import { passwordAuth } from "https://esm.town/v/pomdtr/password_auth";
export default passwordAuth(() => {
return new Response("OK");
}); Or if you prefer to use a string: import { passwordAuth } from "https://esm.town/v/pomdtr/password_auth";
export default passwordAuth(() => {
return new Response("OK");
}, { password: Deno.env.get("VAL_PASSWORD") }); You can also set multiple ones import { passwordAuth } from "https://esm.town/v/pomdtr/password_auth";
export default passwordAuth(() => {
return new Response("OK");
}, { password: [Deno.env.get("VAL_PASSWORD"), Deno.env.get("STEVE_PASSWORD")] }); Note that authenticating using your api token remain an option even after setting a password. TODO [x] allow to authenticate using a val town token [ ] add a way to send an email to ask a password from the val owner [ ] automatically extend the session [ ] automatically remove expired sessions FAQ How to sign out ? Navigate to <your-site>/signout .
v2
February 26, 2024