Search
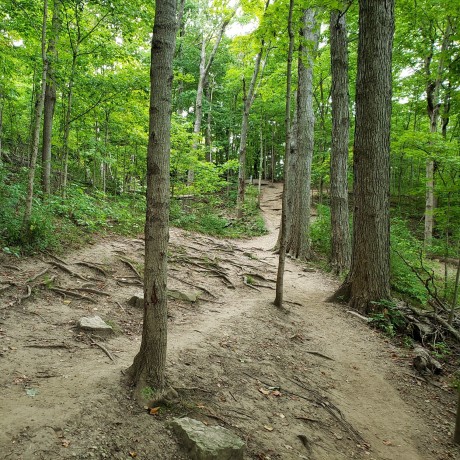
valBotEndpoint
@rayman
// Forked from @mattx.examplebot_endpoint
Script
export let valBotEndpoint = async (
req: express.Request,
res: express.Response,
if (!req.get("X-Signature-Timestamp") || !req.get("X-Signature-Ed25519")) {
res.status(400);
res.end("Signature headers missing");
const verified = await verify_discord_signature(
process.env.valbot_pubkey,
JSON.stringify(req.body),
req.get("X-Signature-Ed25519"),
codepen_debug
@g
Codepen Debug Direct URLs to your Codepen projects. Not in an iframe!
HTTP
# Codepen Debug
Direct URLs to your Codepen projects. Not in an iframe!
export default async function(req: Request): Promise<Response> {
const url = new URL(req.url);
const id = url.pathname.slice(1);
if (!id) return new Response("go to /{id}");
if (!/^[A-Za-z0-9]+$/.test(id)) return new Response("invalid id");
try {
const pen = await parseCodepen(id);
return new Response(
vueExample
@maxm
@jsxImportSource https://esm.sh/vue
HTTP
/** @jsxImportSource https://esm.sh/vue */
import { renderToString } from "npm:vue/server-renderer";
export const vueExample = async () =>
new Response(await renderToString(<div>Test {1 + 1}</div>), {
headers: {
"Content-Type": "text/html",
upload_and_fetch_by_keyvalue_metadata
@stevedylandev
An interactive, runnable TypeScript val by stevedylandev
Script
const JWT = Deno.env.get("PINATA_JWT");
async function main() {
try {
console.log("uploading file");
const file = new File(["This is a metadata test"], "test-metadata.txt", { type: "text/plain" });
const formData = new FormData();
formData.append("file", file);
formData.append(
"pinataMetadata",
JSON.stringify({
githubRepoViewer
@ejfox
An interactive, runnable TypeScript val by ejfox
HTTP
export default async function server(request: Request): Promise<Response> {
const url = new URL(request.url);
if (url.pathname === "/api/files") {
const user = url.searchParams.get("user");
const repo = url.searchParams.get("repo");
if (!user || !repo) {
return new Response("Missing user or repo parameter", { status: 400 });
const apiUrl = `https://api.github.com/repos/${user}/${repo}/contents`;
const response = await fetch(apiUrl);
const contents = await response.json();
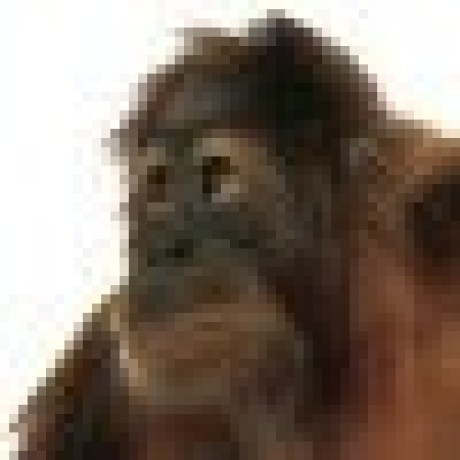
honoJSX
@pomdtr
@jsxImportSource https://esm.sh/hono/jsx
HTTP
/** @jsxImportSource https://esm.sh/hono/jsx **/
const Layout = (props) => {
return (
<html>
<body>{props.children}</body>
</html>
const Top = (props: { messages: string[] }) => {
return (
<Layout>
<h1>Hello Hono!</h1>
codeOnVT_ribbonElement
@andreterron
"Code on Val Town" Ribbon HTML Element Ribbon element used by @andreterron/codeOnValTown Usage ribbonElement({val: { handle: "andre", name: "foo" }}) - define which val to link to; ribbonElement() - infer the val from the call stack. Example: @andreterron/openable_element import { ribbonElement } from "https://esm.town/v/andreterron/codeOnVT_ribbonElement?v=3";
import { html } from "https://esm.town/v/stevekrouse/html?v=5";
export default async (req: Request): Promise<Response> => {
return html(`
<h2>Hello world!</h2>
<style>* { font-family: sans-serif }</style>
${ribbonElement()}
`);
};
Script
# "Code on Val Town" Ribbon HTML Element
Ribbon element used by @andreterron/codeOnValTown
## Usage
- `ribbonElement({val: { handle: "andre", name: "foo" }})` - define which val to link to;
- `ribbonElement()` - infer the val from the call stack.
Example: @andreterron/openable_element
export function ribbonElement({ val, style }: { val?: ValRef; style?: string } = {}) {
const valRef = val?.handle && val?.name ? val : rootValRef();
if (!valRef) {
console.error("Failed to infer val. Please set the val parameter to the desired `{ handle, name }`");
epg
@taoji
// Simple in-memory cache
HTTP
const Config = {
repository: 'e.erw.cc',
FETCH_TIMEOUT: 3000, // 3 seconds timeout
CACHE_DURATION: 1000 * 60 * 60 // 1 hour cache
// Simple in-memory cache
const EPG_CACHE = new Map();
async function timeoutFetch(request, timeout = Config.FETCH_TIMEOUT) {
const controller = new AbortController();
const timeoutId = setTimeout(() => controller.abort(), timeout);
try {
val_FTRpMzznMJ
@dhvanil
An interactive, runnable TypeScript val by dhvanil
HTTP
export default async function handler(req) {
try {
const result = await (async () => {
function fibonacci() {
let a = 0, b = 1;
let lowestThreeDigit;
while (b < 1000) {
if (b >= 100 && b < 1000) {
lowestThreeDigit = b;
let temp = b;
Fetch
@niek
Fetch Template Example template to quickly get started with a backend for Fetch in Framer.
HTTP
# Fetch Template
Example template to quickly get started with a backend for Fetch in Framer.
import { cors } from "npm:hono/cors";
const app = new Hono();
app.use(cors());
app.get("/", (c) => {
const speed = Math.floor(Math.random() * (230 - 170 + 1)) + 170;
return {
speed,
export default app.fetch;
messageBroker
@mauforonda
An interactive, runnable TypeScript val by mauforonda
Script
import { messages } from "https://esm.town/v/mauforonda/messages";
export let messageBroker = (req, res) => {
messages.push({ time: Date.now(), message: req.body.message });
markdown_download
@taras
markdown.download Handy microservice/library to convert various data sources into markdown. Intended to make it easier to consume the web in ereaders Introductory blog post: https://taras.glek.net/post/markdown.download/ Package: https://jsr.io/@tarasglek/markdown-download Features Apply readability Further convert article into markdown to simplify it Allow webpages to be viewable as markdown via curl Serve markdown converted to html to browsers Extract youtube subtitles Can paste htmlContent in curl 'https://val.markdown.download/' -H 'content-type: application/x-www-form-urlencoded' --data-urlencode "htmlContent@$HOME/Downloads/oai-reference.html" Source https://github.com/tarasglek/markdown-download https://www.val.town/v/taras/markdown_download License: MIT Usage:
https://markdown.download/ + URL Dev:
https://val.markdown.download/ + URL
HTTP
# markdown.download
Handy microservice/library to convert various data sources into markdown. Intended to make it easier to consume the web in ereaders
Introductory blog post: https://taras.glek.net/post/markdown.download/
Package: https://jsr.io/@tarasglek/markdown-download
### Features
- Apply readability
const isCloudflareWorker = typeof Request !== "undefined" && typeof Response !== "undefined";
// import { convertHtmlToMarkdown } from ;
// init async loading of modules
const AgentMarkdownImport = isCloudflareWorker ? import("npm:agentmarkdown@6.0.0") : null;
objectExperiment
@eric
An interactive, runnable TypeScript val by eric
Script
export let objectExperiment = () => new Proxy({a: 1, b: 2}, {get(target, prop, reciever) { if (prop === "b") { return 3} return target[prop]}})
valle_tmp_228422435641258538528486008331893
@janpaul123
// Initialize sample stories and store them in blob storage
HTTP
// Initialize sample stories and store them in blob storage
const SAMPLE_STORIES_KEY = "hn_realistic_stories";
async function initializeSampleStories() {
const existingStories = await blob.getJSON(SAMPLE_STORIES_KEY);
if (!existingStories) {
const sampleStories = Array.from({ length: 30 }).map((_, idx) => ({
id: idx + 1,
title: faker.lorem.sentence(),
url: faker.internet.url(),
votes: Math.floor(Math.random() * 100),
rockSevenEndpoint
@erlenstar
An interactive, runnable TypeScript val by erlenstar
Script
export let rockSevenEndpoint = (req, res) => {
// $erlenstar.rockSevenMessage = req.body;
messages2.push({
time: Date.now(),
msg: req.body,
res.json({
status: "ok",
status_code: 200,
time: Date.now(),
messages: messages2,