Likes
78
trob
multilingualchatroom
HTTP
A simple chat room to share with friends of other languages, where each user can talk in their own language and see others' messages in their own language.
Click and hold a translated message to see the original message.
Open the app in a new window to start your own, unique chatroom that you can share with your friends via the room URL. TODO: BUG: fix the issue that keeps old usernames in the "[User] is typing" section after a user changes their name. BUG: Username edit backspaces is glitchy. UI: Update the title for each unique chatroom to make the difference clear. UI: mobile friendly. Feature: the ability for the message receiver to select a part of a translation that is confusing and the author will see that highlight of the confusing words and have the opportunity to reword the message or... Feature: bump a translation to a higher LLM for more accurate translation. Feature: use prior chat context for more accurate translations. Feature: Add video feed for non-verbals while chatting.
5
flesch
altairClient
HTTP
Altair GraphQL Client import { altairClient } from "https://esm.town/v/flesch/altairClient";
import { graphql, GraphQLObjectType, GraphQLSchema, GraphQLString } from "https://esm.sh/graphql";
// Define the GraphQL schema
const schema = new GraphQLSchema({
query: new GraphQLObjectType({
name: "Query",
fields: {
hello: {
type: GraphQLString,
resolve: () => "Hello from Val Town GraphQL API!",
},
},
}),
});
// Function to handle GraphQL requests
async function handleGraphQLRequest(request: Request): Promise<Response> {
const { query, variables } = await request.json();
const result = await graphql({
schema,
source: query,
variableValues: variables,
});
return new Response(JSON.stringify(result), {
headers: {
"Content-Type": "application/json"
},
});
}
// HTTP handler function
export default altairClient(async function(req: Request): Promise<Response> {
if (req.method === "POST") {
return handleGraphQLRequest(req);
} else {
return new Response("This endpoint only accepts POST requests for GraphQL queries.", { status: 405 });
}
}); ↑ https://www.val.town/v/flesch/graphqlAPIEndpoint
1
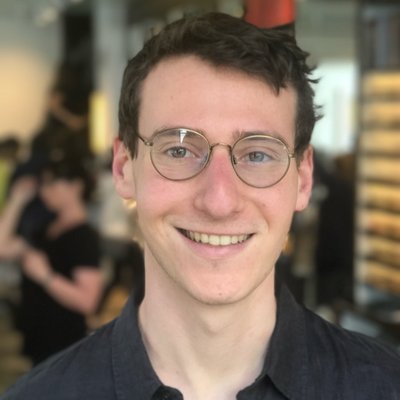
stevekrouse
cerebras_searcher
HTTP
Cerebras Searcher a Perplexity clone that uses the SerpAPI to do RAG
and summaries with Cerebras ( requires a SerpAPI key ) This val might not be working because we're out of SerpAPI credits,
but if you fork it and get your own SerpAPI key, it should work. Comment on this
val if you still have trouble, thanks! Setup Fork this val Sign up for Cerebras and get an API key Save it in a Val Town environment variable called CEREBRAS_API_KEY Get a SerpAPI key (free trial available) Set your SerpAPI API key into you Val Town Environment Variables Done!
3
iamseeley
valTownUser
Script
👤 valTownUser The valTownUser object provides a convenient interface to interact with Val Town's API and access user-specific information and functionalities. User Information getUserInfo() Fetches and caches the user's information. Use case: Retrieving all user details at once. import { valTownUser } from "https://esm.town/v/iamseeley/valTownUser";
export const getUserInfo = () => valTownUser.getUserInfo();
// Example usage:
const userInfo = await getUserInfo();
console.log('User Information:', userInfo);
// You can also destructure specific properties you need:
const { username, id, email, tier } = await getUserInfo();
console.log(`Username: ${username}`);
console.log(`User ID: ${id}`);
console.log(`Email: ${email}`);
console.log(`Account Tier: ${tier}`); getUsername() Retrieves the user's username. Use case: Displaying the current user's name in a val. import { valTownUser } from "https://esm.town/v/iamseeley/valTownUser";
export const getUsername = () => valTownUser.getUsername();
// Example usage:
const username = await getUsername();
console.log(`Current user: ${username}`); getId() Retrieves the user's ID. Use case: Using the user ID for database queries or API calls. import { valTownUser } from "https://esm.town/v/iamseeley/valTownUser";
export const getId = () => valTownUser.getId();
// Example usage:
const userId = await getId();
console.log(`User ID: ${userId}`); getBio() Retrieves the user's biography. Use case: Displaying the user's bio on a profile page. import { valTownUser } from "https://esm.town/v/iamseeley/valTownUser";
export const getBio = () => valTownUser.getBio();
// Example usage:
const bio = await getBio();
console.log(`User bio: ${bio || 'Not set'}`); getProfileImageUrl() Retrieves the URL of the user's profile image. Use case: Showing the user's avatar in a val. import { valTownUser } from "https://esm.town/v/iamseeley/valTownUser";
export const getProfileImageUrl = () => valTownUser.getProfileImageUrl();
// Example usage:
const profileImageUrl = await getProfileImageUrl();
console.log(`Profile image URL: ${profileImageUrl || 'Not set'}`); getEmail() Retrieves the user's email address. Use case: Sending notifications or verifications. import { valTownUser } from "https://esm.town/v/iamseeley/valTownUser";
export const getEmail = () => valTownUser.getEmail();
// Example usage:
const email = await getEmail();
console.log(`User email: ${email}`); getTier() Retrieves the user's account tier. Use case: Implementing tier-specific features. import { valTownUser } from "https://esm.town/v/iamseeley/valTownUser";
export const getTier = () => valTownUser.getTier();
// Example usage:
const tier = await getTier();
console.log(`User tier: ${tier}`); URL Generation getEndpointUrl(valName) Generates the endpoint URL for a specified val. Use case: Creating links to val endpoints in your application. import { valTownUser } from "https://esm.town/v/iamseeley/valTownUser";
export const getEndpointUrl = (valName: string) => valTownUser.getEndpointUrl(valName);
// Example usage:
const endpointUrl = await getEndpointUrl('mySitesServer');
console.log(`Endpoint URL: ${endpointUrl}`); getValUrl(valName) Generates the Val Town URL for a specified val. Use case: Creating links to val pages in Val Town. import { valTownUser } from "https://esm.town/v/iamseeley/valTownUser";
export const getValUrl = (valName: string) => valTownUser.getValUrl(valName);
// Example usage:
const valUrl = await getValUrl('myVal');
console.log(`Val URL: ${valUrl}`); User Activity getLikedVals(options) Retrieves the vals liked by the user. Use case: Displaying a list of the user's favorite vals. import { valTownUser } from "https://esm.town/v/iamseeley/valTownUser";
export const getLikedVals = (options = {}) => valTownUser.getLikedVals(options);
// Example usage:
const likedVals = await getLikedVals({ limit: 5 });
console.log('Liked vals:', likedVals.data); getComments(options) Retrieves comments related to the user. Use case: Showing a user's comment history. import { valTownUser } from "https://esm.town/v/iamseeley/valTownUser";
export const getComments = (options = {}) => valTownUser.getComments(options);
// Example usage:
const comments = await getComments({ limit: 10, relationship: 'given' });
console.log('User comments:', comments.data); getReferences(options) Retrieves references to the user's vals. Use case: Tracking how often a user's vals are used by others. import { valTownUser } from "https://esm.town/v/iamseeley/valTownUser";
export const getReferences = (options = {}) => valTownUser.getReferences(options);
// Example usage:
const references = await getReferences({ limit: 5 });
console.log('Val references:', references.data); Blob Management listBlobs(prefix) Lists the user's blobs. Use case: Displaying a file manager for the user's stored data. import { valTownUser } from "https://esm.town/v/iamseeley/valTownUser";
export const listBlobs = (prefix?: string) => valTownUser.listBlobs(prefix);
// Example usage:
const blobs = await listBlobs();
console.log('User blobs:', blobs); storeBlob(key, data) Stores a new blob or updates an existing one. Use case: Uploading user files or storing large datasets. import { valTownUser } from "https://esm.town/v/iamseeley/valTownUser";
export const storeBlob = (key: string, data: any) => valTownUser.storeBlob(key, data);
// Example usage:
await storeBlob('myFile.txt', 'Hello, World!');
console.log('Blob stored successfully'); getBlob(key) Retrieves a specific blob. Use case: Downloading a user's stored file. import { valTownUser } from "https://esm.town/v/iamseeley/valTownUser";
export const getBlob = async (key: string) => {
const blobData = await valTownUser.getBlob(key);
return new TextDecoder().decode(new Uint8Array(blobData));
};
// Example usage:
const blobContent = await getBlob('myFile.txt');
console.log('Blob content:', blobContent); deleteBlob(key) Deletes a specific blob. Use case: Removing old or unnecessary files. import { valTownUser } from "https://esm.town/v/iamseeley/valTownUser";
export const deleteBlob = (key: string) => valTownUser.deleteBlob(key);
// Example usage:
await deleteBlob('myFile.txt');
console.log('Blob deleted successfully'); Val Management listVals(options) Lists the user's vals. Use case: Displaying a dashboard of the user's created vals. import { valTownUser } from "https://esm.town/v/iamseeley/valTownUser";
export const listVals = (options = {}) => valTownUser.listVals(options);
// Example usage:
const userVals = await listVals({ limit: 5 });
console.log('User vals:', userVals.data); createVal(valInfo) Creates a new val. Use case: Programmatically creating vals based on user input. import { valTownUser } from "https://esm.town/v/iamseeley/valTownUser";
export const createVal = (valInfo: any) => valTownUser.createVal(valInfo);
// Example usage:
const newVal = await createVal({ name: 'myNewVal', code: 'console.log("Hello, World!");' });
console.log('Created val:', newVal); updateVal(valId, updates) Updates an existing val. Use case: Implementing an "edit val" feature in your application. deleteVal(valId) Deletes a specific val. Use case: Allowing users to remove their vals through your interface. SQLite Database Operations executeSqlite(statement) Executes a SQLite statement. Use case: Running custom queries on the user's database. listTables() Lists all tables in the user's SQLite database. Use case: Displaying the structure of a user's database. getTableSchema(tableName) Retrieves the schema of a specific table. Use case: Showing the structure of a particular table. getTableContent(tableName, limit) Retrieves the content of a specific table. Use case: Displaying the data stored in a user's table.
3