just_be
Hiya! I'm Justin, I'm a Brooklyn, NY based engineer working here at Val.town! I co-host devtools.fm and am interested in the future of personal computing, malleable software, and anything we can build to make folks' lives easier.
Likes
6
maxm
valSession
HTTP
Val Session import { ValSession } from "https://esm.town/v/maxm/valSession";
// Generate a token from your valtown api key.
const token = await ValSession.newSession(Deno.env.get("valtown"));
// Other services can use it to authenticate
const user = await ValSession.validate(token); Fork it, provide your own VT_SESSION_PRIVATE_KEY, and update the hardcoded public key. You can generate your own keys like so: import { crypto } from "https://deno.land/std@0.198.0/crypto/mod.ts";
// Generate a key pair for JWT signing and verification
const { privateKey, publicKey } = await crypto.subtle.generateKey(
{
name: "RSASSA-PKCS1-v1_5",
modulusLength: 2048,
publicExponent: new Uint8Array([1, 0, 1]),
hash: "SHA-256",
}, true, ["sign", "verify"],
);
function formatPEM(b64: string, type: "PRIVATE KEY" | "PUBLIC KEY"): string {
const lines = b64.match(/.{1,64}/g) || [];
return `-----BEGIN ${type}-----\n${lines.join("\n")}\n-----END ${type}-----`;
}
const privateKeyPem = formatPEM(
btoa(String.fromCharCode(...new Uint8Array(exportPrivateKey))),
"PRIVATE KEY",
);
const publicKeyPem = formatPEM(
btoa(String.fromCharCode(...new Uint8Array(exportPublicKey))),
"PUBLIC KEY",
);
console.log(privateKeyPem, publicKeyPem);
3
peterqliu
selfDestruct
Script
💥 Deletes the val using it, or whatever url is fed it. Handy for sharing single-use scripts without cluttering people's accounts. If used in an HTTP val, this will run immediately when anyone forks it, making for a zero-click execution as long as ValTown immediately serves a preview of the forked val. Add this to your code last and then do not run it, or you will lose your work. Recommend first checking that the val owner isn't you, so that only forked copies will self-destruct. Usage selfDestruct(import.meta.url) Returns a promise of successful deletion, though the val would not be around to read it.
3
jxnblk
test
HTTP
A test utility and badge to put in your val readme to show test suite status Example: Usage: Make a val Make a separate test suite HTTP val for the val you want to test Import describe and it utilities Write tests Add the badge to your readme, with the url parameter pointing to the test suite val's endpoint. Tests run whenever the test suite val or the badge is fetched import { describe, it } from "https://esm.town/v/jxnblk/test";
import { assertEquals } from "jsr:@std/assert@1";
export default describe("my test suite", () => {
const sum = (a, b) => a + b;
it("sums it up", () => {
assertEquals(sum(1, 2), 3);
})
}) Badge: [![][badge]][url]
[badge]: https://jxnblk-test.web.val.run?url=YOUR_TEST_SUITE_ENDPOINT
[url]: YOUR_TEST_SUITE_URL Example [![][badge]][url]
[badge]: https://jxnblk-test.web.val.run?url=https://jxnblk-tunatestsuite.web.val.run
[url]: https://www.val.town/v/jxnblk/tunaTestSuite
4
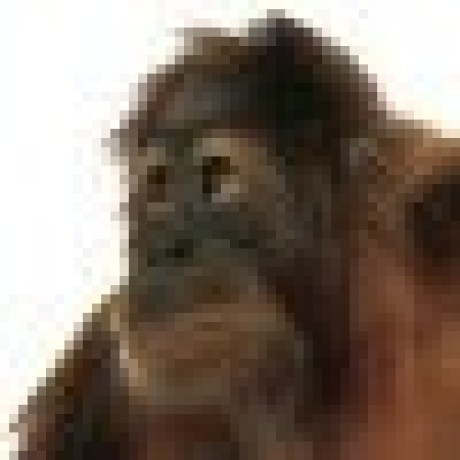
pomdtr
lastlogin
Script
Lastlogin Authentication for val.town Looking for an hono integration ? See @pomdtr/lastloginHono Support login in trough: Email Link QR Code Google Oauth Github Oauth Gitlab Oauth Facebook Oauth Demo You can try a demo at https://pomdtr-lastloginhonoexample.web.val.run (see @pomdtr/lastLoginHonoExample for code) Usage Wrap your http handlers in a lastlogin middleware (sessions will be persisted in the lastlogin_session table on your sqlite account). If you want to be the only one able to access your val, you can use @pomdtr/verifyUserEmail. import { lastlogin } from "https://esm.town/v/pomdtr/lastlogin";
import { verifyUserEmail } from "https://esm.town/v/pomdtr/verifyUserEmail";
export default lastlogin((req) => {
return new Response(`You are logged in as ${req.headers.get("X-LastLogin-Email")}`);
}, {
// check that the user email match your val town email
verifyEmail: verifyUserEmail
}); If you want to customize how is allowed to signup, you can set the verifyEmail option: import { lastlogin } from "https://esm.town/v/pomdtr/lastlogin";
export default lastlogin((req) => {
return new Response(`You are logged in as ${req.headers.get("X-LastLogin-Email")}`);
}, {
verifyEmail: (email) => { email == "steve@valtown" }
}); You can allow anyone to signup by returning a boolean from the verifyEmail function: import { lastlogin } from "https://esm.town/v/pomdtr/lastlogin";
export default lastlogin((req) => {
return new Response(`You are logged in as ${req.headers.get("X-LastLogin-Email")}`);
}, {
verifyEmail: (_email) => true
}); Public Routes import { lastlogin } from "https://esm.town/v/pomdtr/lastlogin";
import { verifyUserEmail } from "https://esm.town/v/pomdtr/verifyUserEmail";
export default lastlogin(() => {
return new Response("Hi!");
}, {
verifyEmail: verifyUserEmail,
public_routes: ["/", "/public/*"],
}); See the URLPattern API for reference. Logout Just redirect the user to /auth/logout
10
jxnblk
tuna
Script
🐟 Simple CSS library for Val Town import { css } from "https://esm.town/v/jxnblk/tuna";
const styles = css({
body: { // special keyword for <body> element
fontFamily: "system-ui, sans-serif",
margin: 0,
backgroundColor: "#f5f5f5",
},
container: {
padding: 32, // numbers are converted to pixel units
margin: "0 auto",
maxWidth: 1024,
},
input: {
fontFamily: "inherit",
fontSize: "inherit",
lineHeight: "1.5",
padding: "2px 8px",
border: "1px solid #ccc",
borderRadius: "4px",
},
}); // JSX example
const html = render(
<div className={styles.container}>
<style {...styles.tag} />
<h1>Tuna Example</h1>
<input
type="text"
defaultValue="hi"
className={styles.input}
/>
</div>
); // get raw CSS string
styles.css Nested selectors and pseudoselectors const styles = css({
button: {
background-color: "tomato",
"&:hover": {
background-color: "magenta",
},
"& > svg": {
fill: "currentColor",
},
},
}); Media queries const styles = css({
box: {
padding: 16,
"@media screen and (min-width: 768px)": {
padding: 32,
"&:hover": {
color: "tomato",
},
},
}
}); Limitations Does not support HTML element selectors (other than body ) Tests: https://www.val.town/v/jxnblk/tuna_tests
3